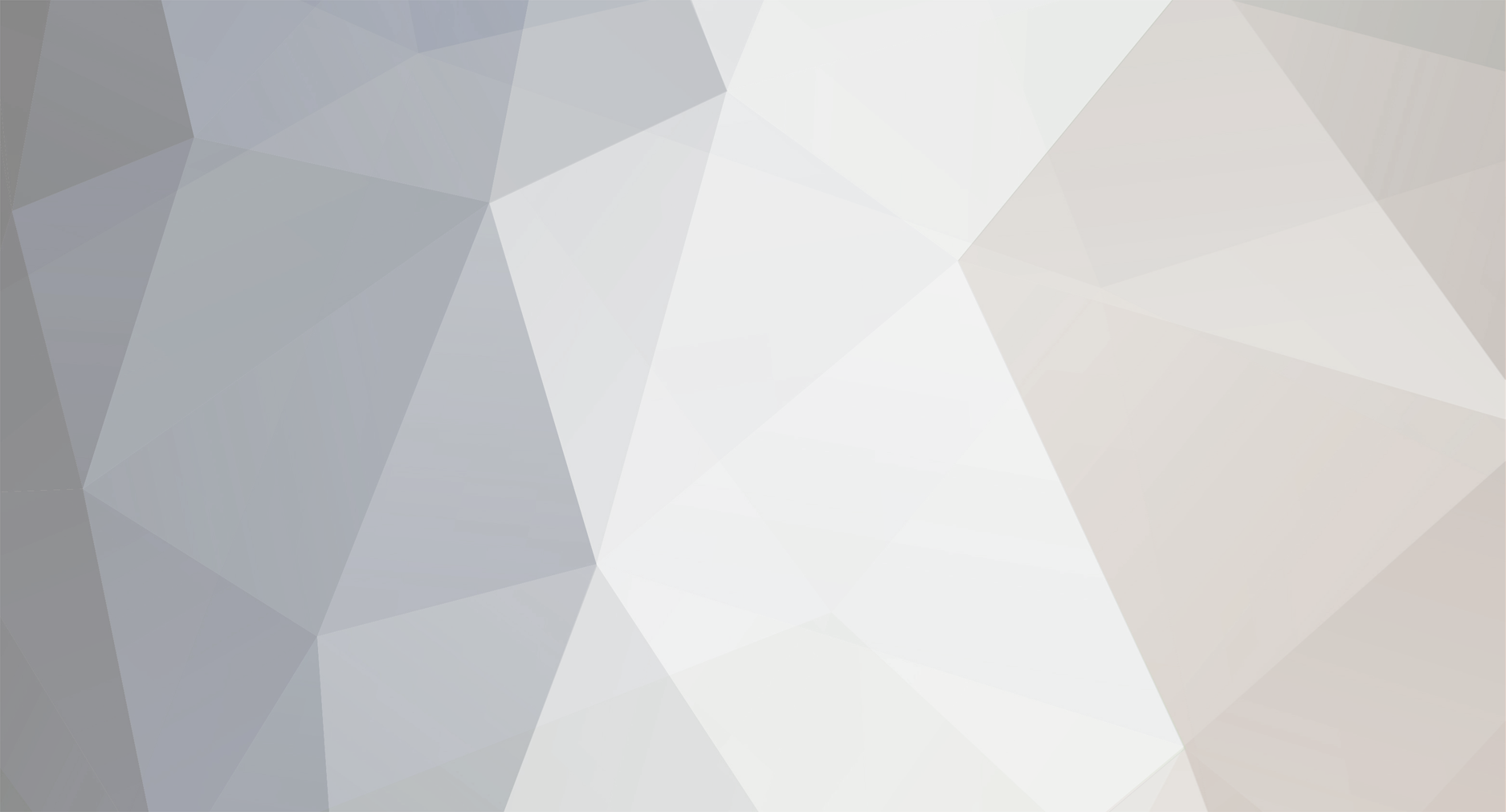
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Again, what makes "Cars", "Home" and "Home Garden" good and the others not good? If you are saying they are good because they are "words" then you would have to have a dictionary to compare the results against. (besides why isn't "car" on your list of 'good' values? It's a normal word as well).
-
Well, creating an echo to "duplicate" the query is a terrible way of debugging. Create the query as a string variable and echo that - the actual query - when there is a problem. All it takes is one simple missed character and your debug code will show perfect while the actual code is flawed. In this case you may not have any error at all. mysqli_error() show the last error. That could have occurred before this code was ever executed. You should check if the query failed first - then check what the error is. $query = "SELECT * FROM ".decrypt($_SESSION["name"], ENCRYPTION_KEY)."_table"; $sql = mysqli_query($con, $query); if(!$sql) { echo "Query: $query<br>Error: " . mysqli_error($con); } while ($row = mysqli_fetch_array($con)) { // displays data }
-
No need to rebuild the wheel: http://codefury.net/2008/07/klogger-a-simple-logging-class-for-php/
-
Why does this always give same result, even when table is empty?
Psycho replied to CGTroll's topic in PHP Coding Help
$query = "SELECT artist_id, artist_name FROM content_artists ORDER BY artist_name ASC"; $result = $mysqli->query($query); if(!$result) { //There was an error executing the query echo "There was a problem getting the list of artists"; } elseif(!mysqli_num_rows($result)) { //There were no results echo "<td>Name of artist:</td>\n"; echo "<td><input type=\"text\" name=\"artist_name\"></td> "; } else { //There were results echo "<td>Pick from existing artits:</td>\n"; echo "<td><select name='ac_artist_id'>\n"; while ($row = $result->fetch_array(MYSQLI_ASSOC)) { echo "<option value='{$row['artist_id']}'>{$row['artist_name']}</option>\n"; } echo "</select></td>\n"; } -
Why does this always give same result, even when table is empty?
Psycho replied to CGTroll's topic in PHP Coding Help
The result of a query will be false if the query failed - i.e. there was an error. An empty result set is still a valid result. -
Off topic. Pro tip. This is a waste of code // Check if file was uploaded if (is_uploaded_file($_FILES['myFile']['tmp_name'])) { $fileCheck = "1"; } else { $fileCheck = "2"; } and can be replaced with this $fileCheck = (is_uploaded_file($_FILES['myFile']['tmp_name'])); $fileCheck will now be a Boolean (true/false)
-
What is the EXACT value of the name field in the database that you think "peter smith" should match?
-
To expand on kicken's post, you need to make some additional changes. You use a while() loop to find the low card. But, that is inefficient to simply do a loop looking for a random value that is lower. Plus, and more importantly, if the high card pulled was a 1, you would get caught in an infinite loop since you could never find a lower card! The high card must have a minimum value of 3 and the low card must have a maximum value of the high card - 2. Your function to get a card needs to accept parameters so you can get a card you need the first time and avoid these problems. Since the high and low cards MUST be different, you don't need any logic when determining the suit to prevent duplicates, since the value will never be the same. So, I'd just randomly pick a suite for each. Here's my take <?php function deal($min, $max) { //Determine the suite $suits = array('Hearts', 'Spades', 'Clubs', 'Diamonds'); $suit = $suits[array_rand($suits)]; //Determine the value and 'face' $value = rand($min, $max); $faceCards = array(11 => 'Jack', 12 => 'Queen', 13 => 'King', 14 => 'Ace'); $face = (array_key_exists($value, $faceCards)) ? $faceCards[$value] : $value; //Return the results as an array $result = array('value' => $value, 'face' => $face, 'suite' => $suit); return $result; } function cardValue($cardAry) { return "{$cardAry['face']} of {$cardAry['suite']}"; } $highCard = deal(3, 14); //Min of 3, Max of Ace $lowCard = deal(1, $highCard['value']-2); //Min of 1, mMax of High card - 2 print "Your low card is " .cardValue($lowCard). " and your high card is " .cardValue($highCard); ?>
-
if(!preg_match($email_exp,$email)) { $email is not defined. The error you are getting with the first name has nothing to do with the first name. it is because of this: if(!preg_match($string_exp,$company_name)) { $error_message .= 'The First Name you entered does not appear to be valid.<br />'; } $company_name is not defined causing this validation to fail, but the error message states "first name"
-
When you execute a query, the return value is a resource identifier that points to the results of the query. You need to use one of the mysql_fetch functions to get the actual data. And, that data would be an array - so it would not be usable in JavaScript until you get the value you need from the array first. The less used method would be to mysql_result() to get a particular value from the resource identifier. <?php error_reporting(E_ALL); $connect = mysql_connect("dbinfo", "dbinfo", "dbinfo"); //select database mysql_select_db("dbinfo", $connect); $refNum = intval($_POST["refNum"]); $query = "SELECT JS_Status FROM JobStatus WHERE JS_ID = $refNum"; $result = mysql_query($query); if(!$result) { $message = "There was a problem getting the results"; } elseif(!mysql_num_rows($result)) { $message = "There was no matching record"; } else { $status = mysql_result($result, 0); } echo "<script language=\"javascript\" type=\"text/javascript\"> alert('{$status}'); </script>"; ?>
-
If you are getting a blank page, it's probably because it is not finding the files. I took the content you provided in reply #3 and put it in a file named test.subm and that file was located in a folder titled "submissions" that was at the same level as the folder in which the executed file was running. Do the files have "subm" as an extension? I assumed so and put the period in the pattern used to find the files.
-
His issue is not in creating the file - it is in processing the input data into the appropriate format for the output.
-
Ok, here you go. I could actually make this more efficient (i.e. not have to traverse over the $lines array), but doing it this way makes it so much easier to debug - just do a print_r() on the array to see what it contains. <?php //Set directory of the input files $directory = '../submissions/'; session_start(); if (!isset($_SESSION['user_email'])) { header( 'Location: login.php'); } //Get array of all files $files = glob("{$directory}*.subm"); //Process the files into a combined array $lines = array(); foreach($files as $file) { $record = json_decode(file_get_contents($file), 1); $content = json_decode($record['content'], 1); //Create flat array for this record $line = array( 'Date' => $record['added'], 'Form Id' => $record['form_id'], 'Name' => $content[13]['value'], 'Email' => $content[14]['value'], 'Phone Number' => $content[15]['value'], 'Best time to contact' => $content[16]['value'], 'City' => (!empty($content[11]['value'])) ? $content[11]['value'] : $content[10]['value'], 'Rating' => $content[3]['value'], 'Reason for rating' => $content[4]['value'] ); //Add current record to combined array $lines[] = $line; } //Create the output data //---------------------- //Build the header row $output = implode("\t", array_keys($lines[0])) . "\n"; //Add each record to the output foreach($lines as $line) { $output .= implode("\t", $line) . "\n"; } //Output the file header("Content-type: application/x-msdownload"); header("Content-Disposition: attachment; filename=form_data.xls"); header("Pragma: no-cache"); header("Expires: 0"); print "$output"; ?>
-
Are the fields in the records always in the same order/positions? For example, 'email' is at index #16 in the subarray for 'content'? If so, it's easy. If not, it will take a little more code. but, we'd need some way to determine which fields go with which values. I'd use the labels, but some are very long. Will the tooltips be consistent for each value? Also, I see you want to output first name and last name in different columns, but the input data only has a single value for "name". You can create a rule for parsing the name into different values, but need to decide how to handle when there aren't exactly two "name" parts in the values. Edit: one last question. I see there is a field for city and another for "if other city, please specify". They both have values. Do you want the "other" city if it has a value, else use the city value from the drop-down?
-
Looks like there are a few problems here, but it's impossible to determine why the output is the way it is from what you have supplied. We really need to see a sample of the input file.
-
Also, you should pass the value to the function as a parameter instead of having the function directly reference the POST value. That way you can re purpose the function as needed. I would also suggest giving the function a better name, such as insertVin() so it is obvious what it is for. Plus, it is considered poor form to echo within a function. Here is an example script. Note that INSERT IGNORE will not produce an error for any problems - not just duplicate keys. For a simple query such as this, it should be fine. But, for more complicated scenarios using it could make debugging very difficult. function insertVin($vin) { //Trim and escape the value $vinValue = mysql_real_escape_string(trim($vin)); //Verify value exists if(empty($vinValue)) { return false; } //Attempt to insert the record $query = "INSERT IGNORE INTO inventory (vin) VALUES ('$vinValue')"; $result = mysqli_query($this->myconn, $query); //Check if the record was inserted if(mysqli_affected_rows($this->myconn)>0) { return "added vin # {$vin}"; } else { return "{$vin} is already in the database"; } } //Usage $isertVinResult = insertVin($_POST['vin']); if(!$isertVinResult) { echo "There was a problem attempting to insert the vin '{$_POST['vin']}'"; } else { echo $isertVinResult; }
-
I don't know it would return anything useful. strtotime() expects a string that is in a date/time format. E.g. strtotime('5-15-2013'), strtotime('10:14:23'), strtotime('2010-8-23 22:35:10'), etc. What are you expecting an uppercase I to return? Are you maybe confusing the input parameter with what would be used for the date() function? For date(), the uppercase I would return 1/0 based upon whether the date/time is within daylight savings time. You can find more information regarding the supported formats here: http://us1.php.net/manual/en/datetime.formats.php EDIT: IT does look like you can use alpha characters for the input - but I don't see a single "I" as a valid value. There are references to double "I"s though
-
PHP/MySql: Show parent and childrens from a table
Psycho replied to LBernoulli's topic in PHP Coding Help
A forum post is not an appropriate medium for teaching you a new methodology for DB handling. There are plenty of tutorials out there to get you started. -
I was going to suggest that - but you still have the same problem of the text may not "fit" in the available space. So, the process to output to PDF would have to be able to measure the output and adjust it accordingly.
-
Each browser type (IE, FF< Opera) and each version can have differences in how it displays a web page. Using a web page for exacting printer layout/output is never going to be 100%. For labels you can probably format the structure of the output so it will meet your needs, but you can't cover every eventuality. If the content of some labels is bigger than the available space you can reduce the font size to cover those eventualities. heck, even MS Word will fail to fit content into a label template if there is too much text. EDIT: Here is a post on dynamically scaling text within a fixed area that might be of interest to you. http://stackoverflow.com/questions/687998/auto-size-dynamic-text-to-fill-fixed-size-container
-
1. Please use [ code ] tags when posting code. 2. You can use the syntax for foreach() as requinix showed, but (and this is just my opinion) I think it is better to use the curly braces to deliniate the start/end of the code blocks associated with the foreach loop. 3. The inner foreach() loop is creating the same content each time. That is a waste of resources. You should instead, run that foreach() loop first and create the content as a variable. Then use that variable inside the main foreach loop. 4. It's also a waste to create variables from the $preownedinfo object since you are only using them once. Give this a try <?php $preowned = simplexml_load_file('file.xml'); $photosHTML = ''; foreach ($AdditionalPhoto as $addphoto) { $photosHTML .= "<li>$addphoto</li>"; } foreach ($preowned as $preownedinfo) { $price = '$' . money_format($preownedinfo->Price, 2, '.', ','); echo " <div class=\"detailsTitle\"> <a href=\"#\">{$preownedinfo->Yrs} {$preownedinfo->Make} {$preownedinfo->Model} {$preownedinfo->ExtraField->ContentEN->ExteriorColor} {$preownedinfo->ExtraField->ContentEN->Doors} Doors</a> </div> <div> <div style\"float:left; width:400px;\"><img src=\"{$preownedinfo->MainPhoto}\"width=\"400\" border=\"0\" /></div> <div style=\"float:right;\"> <ul>{$photosHTML}</ul> </div> <div style=\"clear:both;\"> <div class=\"specsDetails\"> <div class=\"specsDetailsRow\"> <div class=\"leftDetailsSpec\">Our Price: </div> <div class=\"rightSpec\">{$price}</div> </div> <div class=\"specsDetailsRow\"> <div class=\"leftDetailsSpec\">Body Style: </div> <div class=\"rightSpec\">{$preownedinfo->Trim}</div> </div> <div class=\"specsDetailsRow\"> <div class=\"leftDetailsSpec\">Status: </div> <div class=\"rightSpec\">Used</div> </div> <div class=\"specsDetailsRow\"> <div class=\"leftDetailsSpec\">Engine: </div> <div class=\"rightSpec\">{$preownedinfo->ExtraField->ContentEN->Engine}</div> </div> <div class=\"specsDetailsRow\"> <div class=\"leftDetailsSpec\">Transmission: </div> <div class=\"rightSpec\">{$preownedinfo->ExtraField->ContentEN->Transmission}</div> </div> <div class=\"specsDetailsRow\"> <div class=\"leftDetailsSpec\">Ext. Colour: </div> <div class=\"rightSpec\">{$preownedinfo->ExtraField->ContentEN->ExteriorColor}</div> </div> <div class=\"specsDetailsRow\"> <div class=\"leftDetailsSpec\">Int. Colour: </div> <div class=\"rightSpec\">{$preownedinfo->ExtraField->ContentEN->InteriorColor}</div> </div> <div class=\"specsDetailsRow\"> <div class=\"leftDetailsSpec\">Klometres: </div> <div class=\"rightSpec\">{$preownedinfo->ExtraField->ContentEN->Odometer}</div> </div> <div class=\"specsDetailsRow\"> <div class=\"leftDetailsSpec\">Stock Number: </div> <div class=\"rightSpec\">{$preownedinfo->StockNumber}</div> </div> </div> <div class=\"detailsOverview\">{$preownedinfo->AdDescription}</div> <div class=\"detailsOverview requestInfo\"> <a href=\"#\">Request Info</a> </div> </div>"; } ?>
-
SELECT * FROM ( SELECT * FROM products JOIN categories USING (product_id) WHERE category_name IN ('Helmets', 'Forks', 'Foo', 'Bar') AND price_tier = 1 AND (Gender LIKE '%Mens or Unisex%' OR Gender = '') ORDER BY RAND() ) AS t GROUP BY category_name
-
Ah, if memory serves, that is because you can use ORDER BY on the entire result set from UNIONed queries. Try putting the queries in parens so the ORDER BY would only apply to the individual queries (SELECT * FROM products WHERE ProductID != 56305 AND 'Helmets' IN (Cat1, Cat2, Cat3) AND price_tier = 1 AND (Gender LIKE '%Mens or Unisex%' OR Gender = '') GROUP BY ProductID ORDER BY RAND() LIMIT 1) UNION (SELECT * FROM products WHERE ProductID != 56305 AND 'Forks' IN (Cat1, Cat2, Cat3) AND price_tier = 1 AND Brand = 'Affix' AND (Gender LIKE '%Mens or Unisex%' OR Gender = '') GROUP BY ProductID ORDER BY RAND() LIMIT 1) Also, as I stated, the structure not only limits you to three categories - it creates real problem in working with the data as is evident in the problem you are facing now. You could get all the data you need with a simple query if the structure was correct.
-
I can't speak for what it's like in the UK, but in the US there is no one right answer. I know some companies focus on the candidates' ability and look for years of experience and/or examples of their work and will follow up with specific tests or technical interviews. However, some will have a mandate that a degree is required. Personally, I think that is short sighted as very qualified candidates can be rejected over less qualified candidates just because they have a degree. But, it makes it easier for the hiring manager since they don't have to dig as deep in the candidates actual qualifications. But, if you are looking to get into the game, then by all means get a degree. Unless you have an extensive work history and have a strong-skill set, it is probably the single best thing you can do to get a job. Plus, some firms that require degrees do so with the intention of hiring "junior" developers. I will also add that, in my opinion, Web Development and Web Design are two completely different things and very few people can do both very well. In the early days, everyone who "built" web pages was considered a Web Designer because it was mostly static web pages. but, as times changed, the need to build complicated web-based software emerged. However, the original nomenclature of "Web Designer" still gets applied to the programmer/developers on a fairly regular basis.