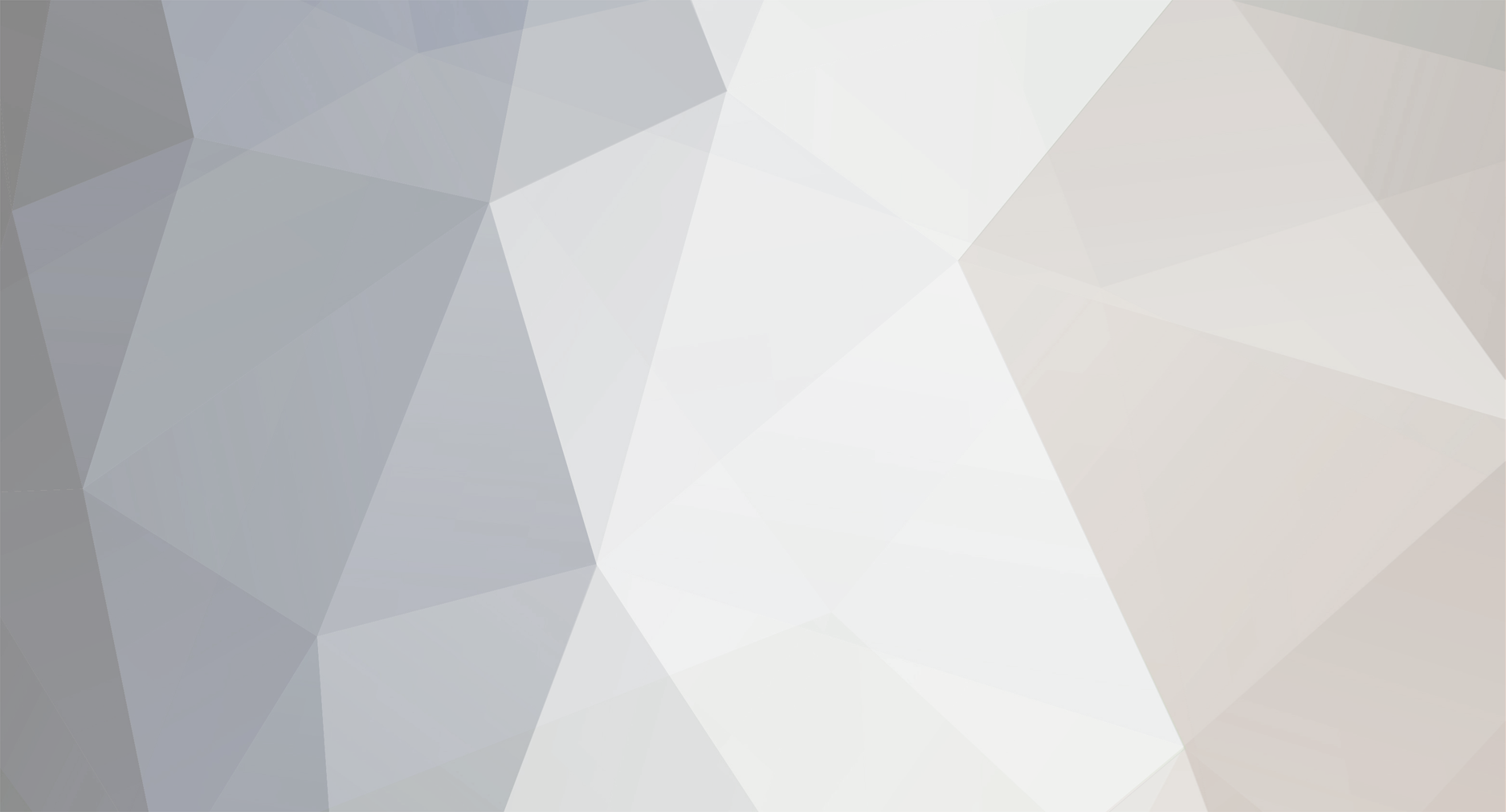
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
This would not be a PHP problem and should be in the HTML forum (Moving). I do see that you don't appear to have a closing TR tag for the data rows. That could be the problem. Plus, you can run the page through an HTML validator to find errant/invalid tags.
-
Looks like you are storing the date as a string in the DB and not a date value - that's a bad idea and makes this a little more difficult. Plus, based on your specific needs this could be simpler, but this will get you started <?php //Create array with current date as initial value $dateOptions = array(date('m/d/Y')); //Add dates from DB to array while($row_gdate= mysql_fetch_assoc($result_gdate)) { $dateOptions[] = $row_gdate['pat_date']; } //Remove duplicates (current date) $dateOptions = array_unique($dateOptions); //Sort the dates sort($dateOptions); //Create the options list foreach($dateOptions as $date) { $selected = ($exam_date == $date) ? ' selected="selected"' : ''; echo "<option value='?exam_date={$date}'{$selected}>{$date}</option>\n"; } ?>
-
There is no right answer here. No solution will be perfect because no matter what you do now you have no control over how those feeds may change over time. I think you should go into this with the expectation of getting a "good enough" solution. Getting 90% of the products into the right category will probably take little effort. Getting the remaining 10% would be a lot of work. Start by analyzing the input data to determine what attributes you can use to make a determination about the category. And, as you've pointed out this is probably different for each source of the data. I don't know how many different sources you are using. If you are using a relatively small number of sources I would look at each source individually and maybe create different logic for each one. But, if there are hundreds, then it probably isn't worth the effort. Bottom line is I can't really suggest how to go about this without doing the analysis. But, let;s take the one example you provided where they put laptops under the category notebook. Do, an analysis of the incoming data to see if all "notebooks" are in fact laptops. If so, you can create a rule that anything indicated as a "notebook" will go into the "laptop" category. As for laptop bags being in the laptop category, you can analyze those to see if there is something in common that you can use. For example, do they all have "bag" in the name or some other piece of data that distinguishes them from laptops? If all else fails, you could use the price (although I wouldn't want to resort to it) since laptops bags "should"generally cost a lot less than an actual laptop.
-
Warning: mysql_num_rows() expects parameter 1 to be resource
Psycho replied to JoshuaDempsey's topic in PHP Coding Help
ALWAYS include proper error handling. By not including it you could potentially leak information that someone could use to hack your application. I also see plenty of errors in that script. For example, your header() redirect will never work because you are sending output to the page before it would run. For that matter, why do you output the form before you process the data that may have been submitted? Also, right now, a user could use that form to completely compromise your database. -
I can't find your previous post, but the solution is to simply not output anything to the page until you are done processing. Here is a simple example: Wrong way: <head> <title>Test Page</title> </head> <body> <?php if(isset($_SESSION['username'])) { echo "Hello {$_SESSION['username']}"; } else { header("Location: login.php"); } echo "Today is " . date('m-d-Y'); ?> </body> </html> Right way <?php if(isset($_SESSION['username'])) { $output = "Hello {$_SESSION['username']}"; } else { header("Location: login.php"); } $output .= "Today is " . date('m-d-Y'); ?> <head> <title>Test Page</title> </head> <body> <?php echo $output; ?> </body> </html>
-
Never user regular expressions when you can accomplish the same thing with a simple string function. In this case you could use strrchr() to return the end of the file name after the last period to check the extension. Or, you could use pathinfo() to get the extension as well. But, I agree with PaulRyan completely. You need to create a script that will process the files and extract the necessary information you need and populate it into a database. Trying to read thousands of files on a page load will kill the server.
-
Aside from the fact that the code you posted has syntax errors and is invalid (see the array in the first execution statement), you state that $stmt1 is generating the duplicate data. But, $stmt1 is a SELECT query, $stmt (with no number) is the INSERT query and it only appears once. So, assuming your code doesn't have those syntax errors and the INSERT is only performed once in that function, then my guess is that the problem is not with the function, but that your script is calling the function twice.
-
<< Moving to MySQL Forum >> What you are doing doesn't make sense, so I'm not sure what you are trying to accomplish. The STDDEV_SAMP() is a GROUP_BY (aggregate) function which assumes a list of values. Since $percent is also stored into the field 'percent', I assume it is a single value. So, there will not be a standard deviation. Please explain what you are trying to do and we might be able to help.
-
How to get End Date from Adding Start Date with Duration Count?
Psycho replied to uncat_myself's topic in PHP Coding Help
I need to correct a statement above. I think I had a typo and then used auto-correct, but selected the wrong value: -
How to get End Date from Adding Start Date with Duration Count?
Psycho replied to uncat_myself's topic in PHP Coding Help
Why do you have the fields Duration Count & Duration Name AND an End Date field? Are you wanting to use PHP to determine the end date for the purpose of populating a value for that field? If so, you don't need it since you can always get the end date when you query the table as Muddy_Funster stated. It's a bad idea to try and store applicative information since it creates the opportunity for the data to get out of sync since you have to always make sure you update both sets of values. But, even if you are going to store that value, you should still use MySQL to populate the value, which can be done very simply in the INSERT query. INSERT INTO table_name (start_date, duration_count, duration_name, end_date) VALUES ('$startDate', '$durationCount', '$durationName', DATE_ADD($startDate, INTERVAL $durationCount $durationName)) -
What data EXACTLY are you wanting from that content? All you said is you want to "filter certain information". Also, I just realized you are doing this in JS, not PHP. So, you can try to build the functionality in JS OR you could do a 2nd AJAX call and pass the content to a page you create that will parse the data from the first call and send it back in the format you need.
-
PHP/MySql: Show parent and childrens from a table
Psycho replied to LBernoulli's topic in PHP Coding Help
Agreed, it definitely is not. But, based upon the OP being "new" I didn't want to cloud the solution with PDO. I don't want to encourage using mysql_, but it is still the most understood DB process of PHP use and most typical in current tutorials. -
And, if this is returned from an AJAX response that you do not control, then the better approach would be to use DOM parsing.
-
Yeah, it's not clear which one you think is correct or not. The one on the right looks correct based upon the images that Google brings up for that font. It looks terrible in pink, but those artifacts are meant to give the font characters a 3d appearance: http://t0.gstatic.com/images?q=tbn:ANd9GcTgq6BU9gmxlArNxmNqD8dFA8pBoBip8War-Qs2mG1sYNQsvpM8-g But, this is a non-standard font and probably doesn't have the available character sets to be displayed in italic or other modified states. So, when you try to apply those properties, the computer is trying to dynamically adjust the font based upon its best "guess" resulting in the unintended output. If that's the case, not much you can do unless you can find a similar font with italic characters in it.
-
PHP/MySql: Show parent and childrens from a table
Psycho replied to LBernoulli's topic in PHP Coding Help
I think an improved approach would be to order the results using the query - then there will be less code needed to create the output: $query = "SELECT Parent, Child FROM Test ORDER BY Parent, Child"; $result = mysql_query($query); $current_parent = false; while($row = mysql_fetch_assoc($result); { if($current_parent != $row['Parent']) { $current_parent = $row['Parent']; echo "<span style=\"font-weight:bold;margin-top:12px;\">{$current_parent}</span><br>\n"; } echo "{$row['Child']}<br>\n"; } -
Use DISTINCT or a GROUP BY. Since you are only wanting to get the warning types use DISTINCT. Something like: SELECT DISTINCT warning_type FROM warnings Also, why did you post this in the PHP forum and not the MySQL forum? << Moving >>
-
I'm not sure what X represents in your example: the reps or reps/100 (i.e. "+1 power per 100 reps you have"). I think it is the latter. So, the simplest solution would be something like this: function getPower($reps) { $divVal = $reps/100; $logVal = max(100 * log($divVal), 1); //Max is needed to prevent 0 being returned @ 100 reps return min($divVal, $logVal); } //Test loop for($rep=1; $rep<800; $rep++) { $reps = $rep * 100; $power = getPower($reps); echo "Reps: {$reps}, Power: {$power}<br>\n"; } Here is the part of the output from the test loop where you can see the transition take place: Reps: 64500, Power: 645 Reps: 64600, Power: 646 Reps: 64700, Power: 647 Reps: 64800, Power: 647.389069635 Reps: 64900, Power: 647.54327167 Reps: 65000, Power: 647.697236289
-
What do you mean "it is not working"? Exactly what are you getting? The code you have above does exactly what I expect - it outputs the array returned from the debug_backtrace() function. EDIT: If your issue is that the array output is not "pretty" then the issue is an HTML problem and not a PHP problem (and this is in the wrong forum). There are many options to make the output pretty. The most straightforward is putting it within PRE tags so white-space and line-breaks are rendered in the HTML display. function d ($arg) { echo "<pre>"; var_dump(debug_backtrace()); echo "</pre>"; } Otherwise you can create your own code to loop through the array and format the output any way you want.
-
FYI: You can make your life a little easier and pass a reference to the select field to the function <select name="changehull" size="1" onChange="hullchangeimage(this);"> You can then simplify your function like so function hullchangeimage(selectObj) { if (!document.images) { return; } var imgName = selectObj.options[selectObj.selectedIndex].value; document.images.hullpicture.src = 'build_pics/' + imgName + '.png'; }
-
Total off by penny - can't figure out how
Psycho replied to learningcurve's topic in PHP Coding Help
I'm not going to bother with reading through all that code (as mac_gyver stated, you have too much code). But, I will provide this: 1. If you need to do a calculation (such as for a shopping cart), create ONE process to do that calculation. Never rewrite the logic to do something. 2. NEVER rely upon any client-side calculations or validations. It is fine to use them to give the user some quick feedback - but don't rely on them. You should, typically, create your pages to not use any JS validations. Once everything is working - then you can add them in on top of any server-side validations you need. In this case, I would not use JS at all to do any cart calculation. I would force the user to submit a page to see the total. Or, at the very least, use AJAX to make a server-side call to do the calculation. -
Isn't that just splitting hairs? I will say I should have stated it was poorly "described". Nonetheless, if a description (definition) is not useful then isn't it, by its very purpose, poorly described (defined)? To be fair, the description for the Return Values is absolutely clear So any misunderstanding is the fault of the reader - not the manual. But, as we all know, people don't always read the manual - and when they do - rarely read it in its entirety. Based upon that knowledge, it is my opinion that the description could be worded to provide a more intuitive description of what the function does.
-
This has a much more logical flow. Not tested, so there may be a few issues to address <?php session_start(); $username = isset($_SESSION['username']) ? $_SESSION['username'] : false; $currentPassword = isset($_POST['c_password']) ? $_POST['c_password'] : false; $newPassword = isset($_POST['n_password']) ? $_POST['n_password'] : false; $confirmPassword = isset($_POST['rn_password']) ? $_POST['rn_password'] : false; $errorMsg = false; if(!$username) { //Username not set in session $errorMsg = 'You must be logged in to change your password!'; } elseif($_SERVER['REQUEST_METHOD'] == 'POST') { //Form was posted if(!$currentPassword || !$newPassword || !$confirmPassword) { //All fields not posted $errorMsg = 'Please fill in all the fields!'; } elseif($newPassword != $confirmPassword) { //Passwords do not match $errorMsg = 'Your new password and confirmation do not match!'; } elseif(strlen($newPassword) < 6) { //Password too short $errorMsg = 'The lengh of the new password must be longer than 6 characters!'; } else { //Check current password submitted $connect = mysql_connect("localhost", "**********", "**********"); mysql_select_db("**********"); //Create and run query to verify current password $usernameSQL = mysql_real_escape_string($username); $query = "SELECT password FROM users2 WHERE username='{$usernameSQL}' and password='{$currentPasswordSQL}'"; $result = mysql_query($query); if(!$result) { //Error running query $errorMsg = "Error retrieving user info"; } elseif(!mysql_num_rows($result)) { //Username not in DB $errorMsg = "Username is not recognized"; } else { //Extract and check password $currentPasswordCheck = mysql_result($result, 0); if(sha1($currentPassword) != $currentPasswordCheck) { //Password not correct $errorMsg = "Current Password is incorrect!"; } else { //Password correct, save new PW $newPasswordSQL = sha1($newPassword); $query = "UPDATE users2 SET password='{$newPasswordSQL}' WHERE username='{$usernameSQL}'"; $result = mysql_query($query); if(!$result) { //Error running query $errorMsg = "Error changing password"; } else { //Replace with a redirect (followed by an exit() statement) to a confirmation page with a valid HTML page die("Your password has been changed. <a href='member.php'>Return</a>"); } } } } } //End for post processes ?> <html> <body> <?php echo $message; ?> <form action='changepass.php' method='POST'> Current password: <input type='text' name='c_password'><br /> New password: <input type='password' name='n_password'><br /> Re-enter new password: <input type='password' name='rn_password'><br /> <input type='submit' name='submit' value='Change password'><br /> </form> </body> </html>
-
Well, first off, you need to have a COMMON process for validating password content. So, you should apply the same process when the users first creates their password as well as when they change their password. Second, your logic appears to be haphazard. Why are you even running the first query before you check to see if the user submitted the necessary data? Change your conditions so the error message is not separated from the condition. How you have it now makes it difficult to see what errors line up with what conditions. There is no need to verify the content of the new password AND the confirm password. Just verify they are both the same, then do verifications on the content of one. But, the problem you are currently facing is that you are hashing the password BEFORE you check the length.
-
Did you read the manual for substr()? It's pretty straightforward for what you want. substr($str, 1, 1); The first number represent the index of the character yuo want to start at (first character is at index 0, so the 2nd is at index 1). The second number represents the number of characters you want to obtain.
-
@ami_liz85: To add to Requinix's response, I think the confusion is that you may not be aware that there is a root folder that the host provides you. But, you can create webroot(s) that are at a lower level. You do NOT have to (nor should you) put your site content at the root of the folder that they give you. Create a logical folder structure (such as Requinix showed) and make your webroot for your domain at one of the lower levels. Anything above that would not be accessible to that domain.