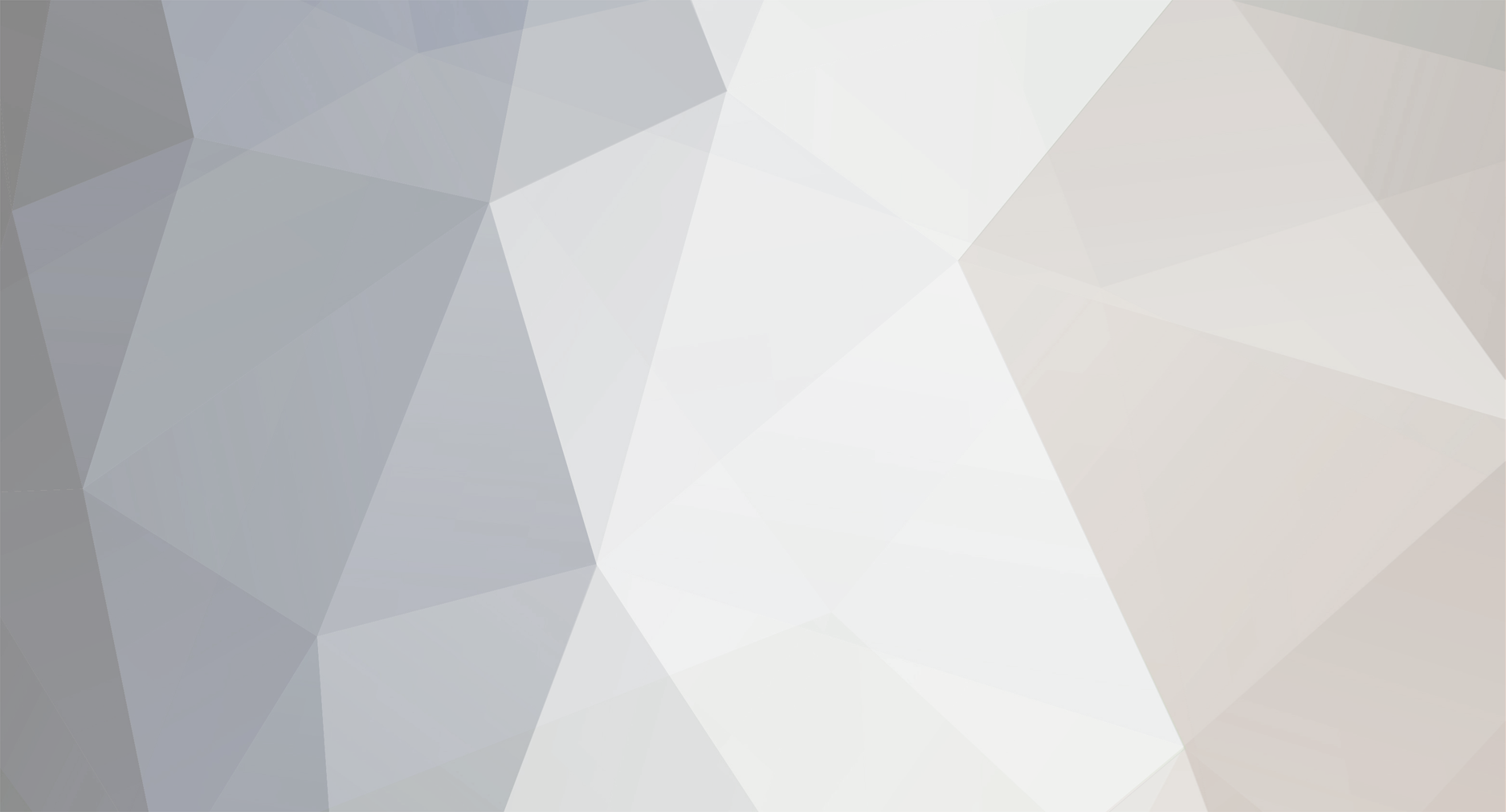
pocobueno1388
Members-
Posts
3,369 -
Joined
-
Last visited
Never
Everything posted by pocobueno1388
-
Delete query that deletes from 3 tables
pocobueno1388 replied to pocobueno1388's topic in MySQL Help
No, thats not it. I don't want the threadID OR the categoryID to be 3, I only want the categoryID to be 3. -
Delete query that deletes from 3 tables
pocobueno1388 replied to pocobueno1388's topic in MySQL Help
Is that because I took one of the tables out of the DELETE clause? I did that because I didn't want it to actually delete the category, just the threads and replies that were in that category. Is there anyway to get it to work without that third table listed? -
This is called the ternary operator, it is the short way of writing an if/else statement. This: $sku_stock = (allowBackOrders) ? "" : " AND S.SKU_Stock > 0"; Is the short version of writing: <?php if (allowBackOrders){ $sku_stock = ""; } else { $sku_stock = " AND S.SKU_Stock > 0"; } ?>
-
You just need the number_format() function. <?php $amount = 1.9; $amount = number_format($amount, 2); echo "$$amount"; ?>
-
Delete query that deletes from 3 tables
pocobueno1388 replied to pocobueno1388's topic in MySQL Help
Okay, it's getting very close to working now. The problem now is it only deletes the threads and replies that have at least one reply. So if there are no replies on a thread it leaves that thread alone, and I want it deleted. Here is the query: DELETE forum_threads ft, forum_replies fr FROM forum_categories fc, forum_threads ft, forum_replies fr WHERE ft.categoryID = fc.categoryID AND fr.threadID = ft.threadID AND fc.categoryID = 3 I'm assuming I have to add something else to the WHERE clause, but I have already linked each table together...am I missing something? -
Yes, that sounds like a good way to do it. You should start coding and ask specific questions about your code when you get stuck. If you are completely lost to how to even start, I recommend reading basic tutorials. www.tizag.com www.w3schools.com
-
Delete query that deletes from 3 tables
pocobueno1388 replied to pocobueno1388's topic in MySQL Help
Okay, I just ran this query: DELETE FROM forum_categories fc, forum_threads ft, forum_replies fr WHERE ft.categoryID = fc.categoryID AND fr.threadID = ft.threadID AND fc.categoryID = 1 Error: #1064 - You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'WHERE ft.categoryID = fc.categoryID AND fr.threadID = ft.threadID AND ' at line 4 -
Run this query: UPDATE table_name SET Filetype='WORD.jpg' WHERE Type='application/msword'
-
Delete query that deletes from 3 tables
pocobueno1388 replied to pocobueno1388's topic in MySQL Help
I'm sorry, I don't follow... I attempted to follow the manual, and this is what I came up with: DELETE forum_categories, forum_threads, forum_replies FROM forum_categories fc, forum_threads ft, forum_replies fr WHERE ft.categoryID = fc.categoryID AND fr.threadID = ft.threadID AND fc.categoryID = 1 I get the error (the table "forum_categories" DOES exist): #1109 - Unknown table 'forum_categories' in MULTI DELETE Am I not supposed to put the table names I want to delete rows from after the DELETE part? -
Delete query that deletes from 3 tables
pocobueno1388 replied to pocobueno1388's topic in MySQL Help
Ah, thanks. Thats seems to cover everything now. I could have sworn I tried a LEFT JOIN, maybe I was thinking that JOIN was the same as LEFT JOIN, but it's INNER JOIN. Now how would I turn this into a delete query? I'm baffled by the syntax in the manual. DELETE FROM forum_categories fc LEFT JOIN forum_threads ft ON ft.categoryID = fc.categoryID LEFT JOIN forum_replies fr ON fr.threadID = ft.threadID WHERE fc.categoryID = 1 -
Delete query that deletes from 3 tables
pocobueno1388 replied to pocobueno1388's topic in MySQL Help
Bump -
Have you even tried Google? http://www.dustindiaz.com/add-and-remove-html-elements-dynamically-with-javascript/
-
Just change this: for ($i = 0; $i < count($month); $i++) { To: for ($i = 1; $i < count($month); $i++) {
-
This will require JavaScript.
-
Getting, saving, displaying and editing data.
pocobueno1388 replied to Braveheartt's topic in PHP Coding Help
You can use PHP and HTML on the same page. Just make sure the extension is .php (unless you have your site configured to read HTML files as PHP). -
Getting, saving, displaying and editing data.
pocobueno1388 replied to Braveheartt's topic in PHP Coding Help
Well then, here are some specific tutorials for you. Working with forms - http://www.tizag.com/phpT/phpsessions.php Insert form data into database - http://www.tizag.com/mysqlTutorial/mysqlinsert.php Get information from database - http://www.tizag.com/mysqlTutorial/mysqlquery.php -
passing variables values using POST
pocobueno1388 replied to sudhakararaog's topic in PHP Coding Help
To pass it via post you need to have a form. Then you can set it up as a hidden input. If your not dealing with a form, you could just set a session with the information in it... <?php session_start(); //remember to call this for every page you want the session on $_SESSION['firstname'] = $firstname; -
Someone isn't going to just code all these for you (unless they have absolutely nothing else to do). You should take it one question at a time and actually supply us with an actual attempt, and the problem with your supplied code. So, lets start with question one. Thats basically just working with forms...then all you need to know other than that is how to register a session. Working with forms - http://www.tizag.com/phpT/forms.php Sessions - http://www.tizag.com/phpT/phpsessions.php
-
Getting, saving, displaying and editing data.
pocobueno1388 replied to Braveheartt's topic in PHP Coding Help
You need to just search Google for basic PHP/MySQL tutorials. What your asking is very broad and your not going to be able to do it without basic knowledge. It wouldn't hurt to read through all the PHP/MySQL tutorials on this site: www.tizag.com or www.w3schools.com After that, you should start figuring out how to do what your wanting. -
Your logic was messed up. Your going to have to do something like this: <?php if(isset($_POST['submit'])) { // general vars // $maximum_filesize = 3145728; // vars POST // $username = mysql_real_escape_string($_POST['username']); $pass_1 = mysql_real_escape_string(trim($_POST['pass_1'])); $pass_2 = mysql_real_escape_string(trim($_POST['pass_2'])); $email_1 = mysql_real_escape_string(trim($_POST['email_1'])); $email_2 = mysql_real_escape_string(trim($_POST['email_2'])); $gender = mysql_real_escape_string($_POST['gender']); $birth_month = mysql_real_escape_string($_POST['birth_month']); $birth_day = mysql_real_escape_string($_POST['birth_day']); $birth_year = mysql_real_escape_string($_POST['birth_year']); $country = mysql_real_escape_string($_POST['country']); $state_county = mysql_real_escape_string($_POST['state_county']); $city = mysql_real_escape_string($_POST['city']); $zip_post_code = mysql_real_escape_string($_POST['code']); $smoke = mysql_real_escape_string($_POST['smoke']); $drink = mysql_real_escape_string($_POST['drink']); $height = mysql_real_escape_string($_POST['height']); $weight = mysql_real_escape_string($_POST['weight']); $eyes = mysql_real_escape_string($_POST['eyes']); $hair = mysql_real_escape_string($_POST['hair']); $children = mysql_real_escape_string($_POST['children']); $marital_status = mysql_real_escape_string($_POST['marital_status']); $seeking_gender = mysql_real_escape_string($_POST['seeking_gender']); $profession = mysql_real_escape_string($_POST['profession']); $interests = mysql_real_escape_string($_POST['interests']); $description = mysql_real_escape_string($_POST['description']); // some vars for the image // $image_file_name = $_FILES['image']['name']; $image_file_size = $_FILES['image']['size']; $image_file_temp = $_FILES['image']['tmp_name']; $image_file_type = $_FILES['image']['type']; // error checking // if(empty($username)) { standard_error("Error","You never entered a username."); $error = TRUE; } if(empty($pass_1)) { standard_error("Error","You never entered a password."); $error = TRUE; } if(empty($pass_2)) { standard_error("Error","You never confirmed your password."); $error = TRUE; } if(empty($email_1)) { standard_error("Error","You never entered an e-mail address."); $error = TRUE; } if(empty($email_2)) { standard_error("Error","You never confirmed your e-mail address."); $error = TRUE; } if(empty($zip_post_code)) { standard_error("Error","You never entered your zip/post code."); $error = TRUE; } if($image_file_size > $maximum_filesize) { standard_error("Error","Your uploaded image is bigger than <b>3mb</b> please upload another."); $error = TRUE; } if($image_file_type != 'image/jpeg' || $image_file_type != 'image/pjpeg' || $image_file_type != 'image/gif' || $image_file_type != 'image/png') { standard_error("Error","Your uploaded image is not one of these allowed file-types only <b>.jpg</b>/<b>.gif</b> and <b>.png</b> are allowed."); $error = TRUE; } if (!isset($error)){ // ========================================= // // some working for the image //$renamed_image = $username // ========================================= // // if there is no errors then insertion // $q_insertion = "INSERT INTO `cmd_users` (`id`, `username`, `password`, `email`, `gender`, `birth_month`, `birth_day`, `birth_year`, `country`, `state_county`, `city`, `zip_post_code`, `smoke`, `drink`, `height`, `weight`, `eye_color`, `hair_color`, `children`, `marital_status`, `seeking_gender`, `profession`, `interests`, `description`, `image`, `image_thumb`, `registered`) VALUES ('', '$username', '$pass_1', '$email_1', '$gender', '$birth_month', '$birth_day', '$birth_year', '$country', '$state_county', '$city', '$zip_post_code', '$smoke', '$drink', '$height', '$weight', '$eyes', '$hair', '$children', '$marital_status', '$seeking_gender', '$profession', '$interests', '$description', '$image_fullsize', '$image_thumbnail', now())"; $r_insertion = mysql_query($q_insertion); // print success and send the e-mail // if($r_insertion) { standard_error("Success","You have been successfully registered. we have sent an e-mail to <b>$email_1</b> with your login details."); // send the e-mail // } else { standard_error("Error","There was an error inserting your details please contact the site admins."); } } // end isset // ?> <?php include("inc/inc_arrays.php"); ?> <?php print("<form action='join.php' method='POST' name='join_form' enctype='multipart/form-data'>"); print("<table class='form_table' cellpadding='5' cellspacing='0' >\n"); print("<tr>\n"); print("<td colspan='2' align='left'><h1>Join Up</h1></td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td colspan='2' align='left'><h1>Your Profile Details</h1></td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Username:</b></label></td><td class='form_style' align='left'><input type='text' name='username' class='textfield' size='40'> <span class='star'>*</span> Required.</td>"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Password:</b></label></td><td class='form_style' align='left'><input type='password' name='pass_1' class='textfield' size='40'> <span class='star'>*</span> Required.</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Confirm Password:</b></label></td><td class='form_style' align='left'><input type='password' name='pass_2' class='textfield' size='40'> <span class='star'>*</span> Required.</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>E-Mail:</b></label></td><td class='form_style' align='left'><input type='text' name='email_1' class='textfield' size='40'> <span class='star'>*</span> Required.</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Confirm E-Mail:</b></label></td><td class='form_style' align='left'><input type='text' name='email_2' class='textfield' size='40'> <span class='star'>*</span> Required.</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Gender:</b></label></td><td class='form_style' align='left'>\n"); print("<select name='gender'>"); foreach($gender_array as $key => $gender_value) { print("<option value='$key'>$gender_value</option>"); } print("</select>"); print("</td>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Birthdate:</b></label></td><td class='form_style' align='left'>\n"); print("<select name='birth_month'>\n"); foreach($birth_month as $key => $birth_month_value) { print("<option value='$key'>$birth_month_value</option>\n"); } print("</select>\n"); print("<select name='birth_day'>\n"); for($i = 1; $i <= 31; $i++) { print("<option value='$i'>$i</option>\n"); } print("</select>\n"); print("<select name='birth_year'>\n"); for($year = 1930; $year <= 2008; $year++) { print("<option value='$year'>$year</option>\n"); } print("</select>\n"); print("</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Country:</b></label></td><td class='form_style' align='left'>\n"); print("<select name='country'>\n"); foreach($countries_list as $key => $country_value) { print("<option value='$key'>$country_value</option>\n"); } print("</select>\n"); print("</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>State / County:</b></label></td><td class='form_style' align='left'><input type='text' name='state_county' class='textfield' size='40'></td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>City:</b></label></td><td class='form_style' align='left'><input type='text' name='city' class='textfield' size='40'></td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Zip / Post Code:</b></label></td><td class='form_style' align='left'><input type='text' name='code' class='textfield' size='40'> <span class='star'>*</span> Required.</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td colspan='2' align='left'><h1>Your Personal Details</h1></td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Do You Smoke?</b></label></td><td class='form_style' align='left'>"); print("<select name='smoke'>"); foreach($smoking_array as $key => $smoking_value) { print("<option value='$key'>$smoking_value</option>\n"); } print("</select>"); print("</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Do You Drink?</b></label></td><td class='form_style' align='left'>"); print("<select name='drink'>"); foreach($drinking_array as $key => $drinking_value) { print("<option value='$key'>$drinking_value</option>\n"); } print("</select>"); print("</td>\n"); print("</tr>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Your Height?</b></label></td><td class='form_style' align='left'>"); print("<select name='height'>"); foreach($height_array as $key => $height_value) { print("<option value='$key'>$height_value</option>\n"); } print("</select>"); print("</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Your Weight?</b></label></td><td class='form_style' align='left'>"); print("<select name='weight'>"); foreach($weight_array as $key => $weight_value) { print("<option value='$key'>$weight_value</option>\n"); } print("</select>"); print("</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Your Eye Colour?</b></label></td><td class='form_style' align='left'>"); print("<select name='eyes'>"); foreach($eyes_array as $key => $eyes_value) { print("<option value='$key'>$eyes_value</option>\n"); } print("</select>"); print("</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Your Hair Colour?</b></label></td><td class='form_style' align='left'>"); print("<select name='hair'>"); foreach($hair_array as $key => $hair_value) { print("<option value='$key'>$hair_value</option>\n"); } print("</select>"); print("</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Do You Have Children?</b></label></td><td class='form_style' align='left'>"); print("<select name='children'>"); foreach($children_array as $key => $children_value) { print("<option value='$key'>$children_value</option>\n"); } print("</select>"); print("</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Your Marital Status?</b></label></td><td class='form_style' align='left'>"); print("<select name='marital_status'>"); foreach($marital_status_array as $key => $marital_status_value) { print("<option value='$key'>$marital_status_value</option>\n"); } print("</select>"); print("</td>\n"); print("</tr>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>I'm Looking For A</b></label></td><td class='form_style' align='left'>"); print("<select name='seeking_gender'>"); foreach($seeking_gender_array as $key => $seeking_gender_value) { print("<option value='$key'>$seeking_gender_value</option>\n"); } print("</select>"); print("</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Profession:</b></label></td><td class='form_style' align='left'><input type='text' name='profession' class='textfield' size='40'></td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td colspan='2' align='left'><h1>Your Interests</h1>(Optional, you can fill this in later.)</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><textarea name='interests' class='textfield' rows='20' cols='80'></textarea></td><td class='form_style' align='left' valign='top'><div id='tip'>Tell everyone all about your interests and hobbies.</div></td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td colspan='2' align='left'><h1>Describe Yourself</h1>(Optional, you can fill this in later.)</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><textarea name='description' class='textfield' rows='20' cols='80'></textarea></td><td class='form_style' align='left' valign='top'><div id='tip'>Please include as much information as possible about yourself, you will recieve far more messages.</div></td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td colspan='2' align='left'><h1>Upload An Image Of Yourself</h1></td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Main Profile Image:</b></label></td><td class='form_style' align='left'><input type='file' name='image' size='40'> <span class='star'>*</span> Required.</td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td class='form_style' align='left'><label><b>Enter The CAPTCHA Numbers:</b></label></td><td class='form_style' align='left'><img src='captcha.php'></td>\n"); print("</tr>\n"); print("<tr>\n"); print("<td colspan='2' align='right'><input type='submit' name='submit' value='Join Up!' /></td>\n"); print("</tr>\n"); print("</table>\n"); print("</form>"); ?>
-
Delete query that deletes from 3 tables
pocobueno1388 replied to pocobueno1388's topic in MySQL Help
Okay, here is my attempt at a select query that would select all threads within a category, and all replies to those threads. SELECT fc.name as category_name, ft.threadID, ft.subject as thread_subject, fr.replyID, fr.body as reply_body, fr.threadID as reply_to_threadID FROM forum_categories fc JOIN forum_threads ft ON ft.categoryID = fc.categoryID JOIN forum_replies fr ON fr.threadID = ft.threadID WHERE fc.categoryID = 1 It was close, but it only selects the threads and replies to the threads that have replies. I would also like to select threads that don't have any replies on them. Am I using the wrong join? I tried others, but got the same results. Thanks for the help -
Change this line: if($validforum) == 0) { To: if ($validforum == 0) {
-
What are you trying to set? You maybe be able to do it with the function ini_set().
-
Your missing a semi-colon after this line mysql_select_db("$db_name")or die("cannot select DB")