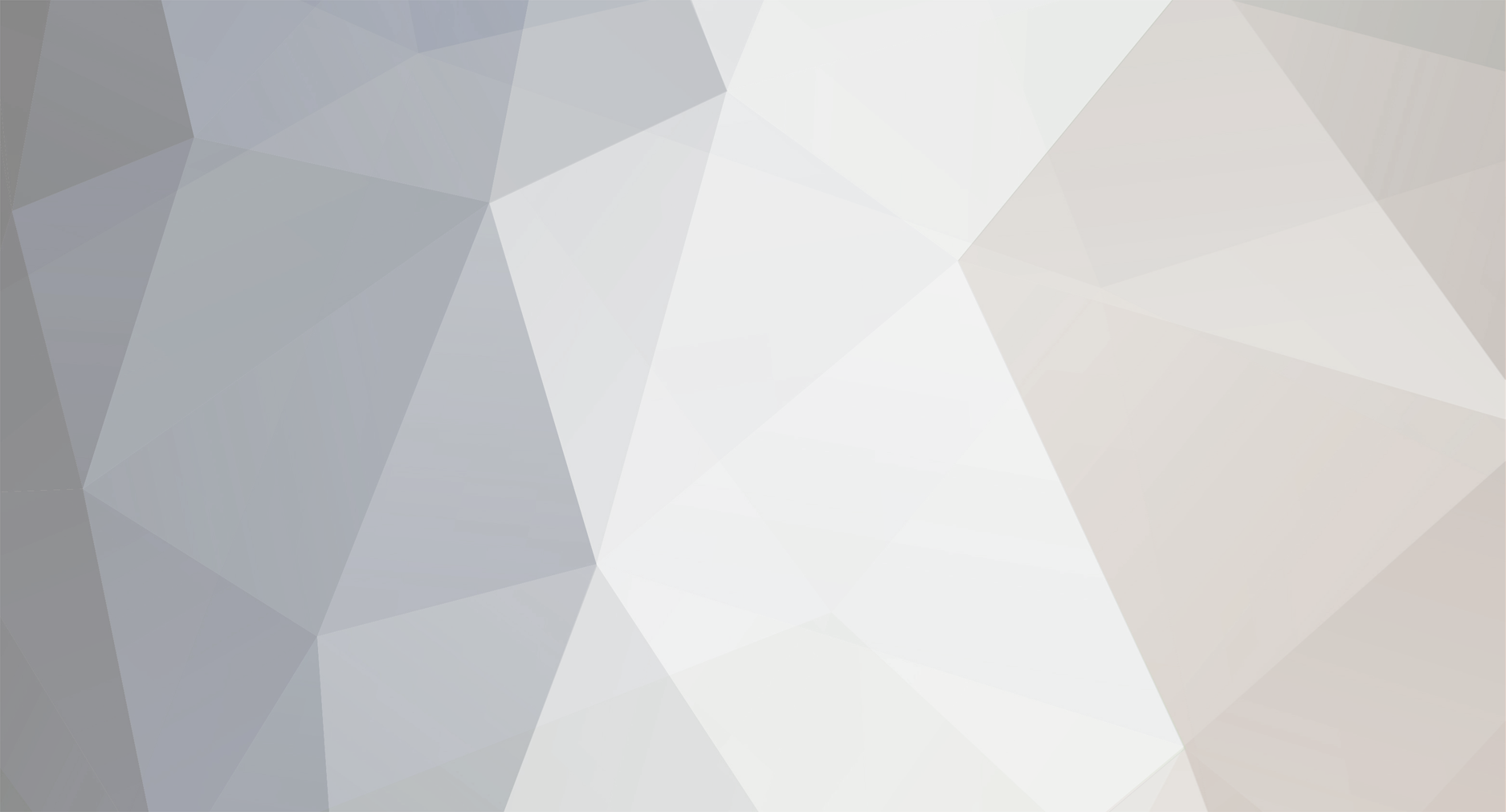
pocobueno1388
Members-
Posts
3,369 -
Joined
-
Last visited
Never
Everything posted by pocobueno1388
-
It's because you are using single quotes instead of double. Try this: <?php $query = "SELECT id, commenttext, username FROM comments LIMIT $offset, $rowsPerPage"; $result = mysql_query($query) or die('Error, query failed'); echo '<table div class="tborder">'; $username = $_SESSION['username']; while($row = mysql_fetch_array($result)) { echo '<tr class="tr' . $tr . '"><td>' . '<img src="http://example.com/main/images/post.gif">' . "<a href='userinfo.php?user=$username'>" . $row["username"] . '</a>' . '<br>' . ' posted on: ' . '<br><br>' . ' ' . $row["commenttext"] . '</td></tr>'; ?>
-
It looks like $bd_base is the name of the database. Change your connect line to: <?php $con = mysql_connect($bd_host, $bd_usuario, $bd_password)or die(mysql_error()); mysql_select_db($bd_base, $con)or die(mysql_error()); See if it gives you an error. Also, look in the manual for mysql_connect() and read a little.
-
86 WPM. Not as good as it used to be, but I'll settle for it :]
-
All the data is going to be in an array, so you will have to loop through it with a foreach loop. <?php if (isset($_POST['your_submit_button'])){ foreach ($_POST['type'] as $key => $val){ echo $key . '-' . $val . '<br>'; } } ?>
-
Dynamically populating a drop down list via PHP
pocobueno1388 replied to colafran's topic in PHP Coding Help
Try running this code and see what you get: <?php // Connect and select a database mysql_connect("localhost", "root", ""); mysql_select_db("addressBook"); // Run a query $result = mysql_query("SELECT * FROM colleague"); if (mysql_num_rows($result) > 0){ echo '<form action="action" method="post">' .'<select name="option">'; //for each row we get from mysql, echo a form input while ($row = mysql_fetch_array($result)) { echo "<option value='{$row['value']}'>{$row['title']}</option>\n"; } echo '</select>' .'<input type="submit">' .'</form>'; } else { echo "No results found" } ?> Also, make sure the rows "value" and "title" exist in the database. It sounds like it's returning the results, but there are no such rows. -
Why not just try it?
-
Site won't load for me.
-
This part: members_login <= (NOW() - INTERVAL $x DAY) means If the date members_login is less than or equal to x days ago. I think I got the symbol messed up and turned the wrong way So change it to this: SELECT COUNT(*) AS members_count FROM members WHERE members_login >= (NOW() - INTERVAL $x DAY)
-
Try changing the query to: SELECT COUNT(*) AS members_count FROM members WHERE members_login <= (NOW() - INTERVAL $x DAY)
-
<?php $x = "30"; $sql = "SELECT COUNT(*) AS members_count FROM members WHERE members_login < (NOW() - INTERVAL $x DAY)"; ?>
-
Redirecting to login page if session variable is not set?
pocobueno1388 replied to gtrufitt's topic in PHP Coding Help
<?php session_start(); if (!isset($_SESSION['sess_name'])){ header("Location: login.php"); } -
You can do it like this: <?php $myvar = 'value'; $$myvar = 'something'; echo $value; //prints "something" ?>
-
$url = $_SERVER['REQUEST_URI']; echo $url;
-
Okay, your master query is going to look like this SELECT oh.*, cm.*, oo.*, od.*, os.*, dm.* FROM order_header oh LEFT JOIN client_master cm ON cm.client_ID=oh.client_id LEFT JOIN order_origin oo ON oo.client_id=oh.client_id LEFT JOIN order_destination od ON od.client_id=oh.client_id LEFT JOIN order_status os ON os.client_id=oh.client_id LEFT JOIN driver_master dm ON dm.client_id=oh.client_id ORDER BY oh.id DESC LIMIT 10 I'm assuming all your tables are linked by client_id. Also, your selecting ALL (*) from every single table, are you really using every bit of that data? You should go through and actually only select the fields your going to use. Not positive if that query will give you what you want, but it's worth a try.
-
Oops, I forgot to select "Score" from the table in the query. Change it to this: <?php $resulta = mysql_query("SELECT s.userid, s.score, u.username FROM Score s LEFT JOIN users u ON u.userid=s.userid ORDER BY SCORE DESC LIMIT 5"); while ($row = mysql_fetch_array($resulta)){ echo '<b>'.$row['username'].'</b>: '.$row['Score'].'<br>'; } ?>
-
Are you asking how to combine these queries? If so...you can do it like this: <?php $resulta = mysql_query("SELECT s.userid, u.username FROM Score s LEFT JOIN users u ON u.userid=s.userid ORDER BY SCORE DESC LIMIT 5"); while ($row = mysql_fetch_array($resulta)){ echo '<b>'.$row['username'].'</b>: '.$row['Score'].'<br>'; } ?> If thats not what you want, explain a little more.
-
Try this: <?php $result = mysql_query("SELECT `1`,`2` FROM `friend_requests` WHERE `id`='{$id}'") or die(mysql_error()); $new_friends = mysql_fetch_array($result); $new_request1 = stripslashes($new_friends['1']); $new_request2 = stripslashes($new_friends['2']); if($new_request1 != 0){ if($new_request2 != 0){ Echo '<a href="friend_request.php">New Friend Requests</a>'; }else{ Echo '<a href="friend_request.php">New Friend Request</a>'; } }else{ $new_friends0 = 'none';// here? } $result2 = mysql_query("SELECT `id` FROM `messages` WHERE `to`='{$id}' and `read`='0'") or die(mysql_error()); $count = mysql_num_rows($result2); while($row = mysql_fetch_assoc($result2)){ if(strlen($row['id']) > 0){ echo '<br>You have '.$count.' new messages!'; }else{ $new_messages = 'none'; //here? } } if ((mysql_num_rows($result) < 1) && (mysql_num_rows($result2) < 1)){ echo 'Nothing =('; } ?>
-
I'm stumped on how to write a delete query that will delete rows from 3 different tables. I'm programming a forum and users can add/delete a category. If a category is deleted, I want to delete all threads/posts from that category. Here are the table structures: ----------------------- TABLE forum_categories ----------------------- categoryID +---------------------+ name | description | | ----------------------- | TABLE forum_threads | ----------------------- | threadID ---------------------------+ categoryID +---------------------+ | userID | subject | body | date | | ----------------------- | TABLE forum_replies | ----------------------- | replyID | threadID +--------------------------+ userID body date So here are the queries I would like to combine DELETE FROM forum_categories WHERE categoryID=$categoryID DELETE FROM forum_threads WHERE forum_threads.categoryID=forum_categories.categoryID DELETE FROM forum_replies WHERE forum_replies.threadID=forum_threads.threadID And here is my failed attempt at the query: DELETE FROM forum_categories fc, forum_threads ft, forum_replies fr USING fc, ft, fr WHERE fc.categoryID=ft.categoryID AND ft.threadID=fr.threadID AND fc.cateogryID=$categoryID I'm not sure that I understand the syntax, and what exactly the USING part is. Any help with this from the MySQL gurus would be greatly appreciated Thanks.
-
I'm assuming you have an auto-incrementing ID field in the table, so you would do a query like this: SELECT username FROM users ORDER BY ID DESC LIMIT 1
-
Don't forget the mysql_real_escape_string(). Other than that, it looks fine.
-
Yep, thats exactly how you use it. Although I would use mysql_real_escape_string() with it too. You have to make sure you escape the data AFTER the purifying though. Like this: $clean_html = mysql_real_escape_string($purifier->purify($dirty_html));
-
Download the "light" version, it cuts it down a lot. I use it and I've never noticed a performance issue.
-
You should look into this HTML purifier class: http://htmlpurifier.org/
-
is session_start() called in your header file? If not, then you need to call it at the top of the script.
-
SIMPLE form validation (Cant get it to work!!!!!!)
pocobueno1388 replied to mikebyrne's topic in PHP Coding Help
You need to put a semi-colon after this line: echo 'got no name<br>'