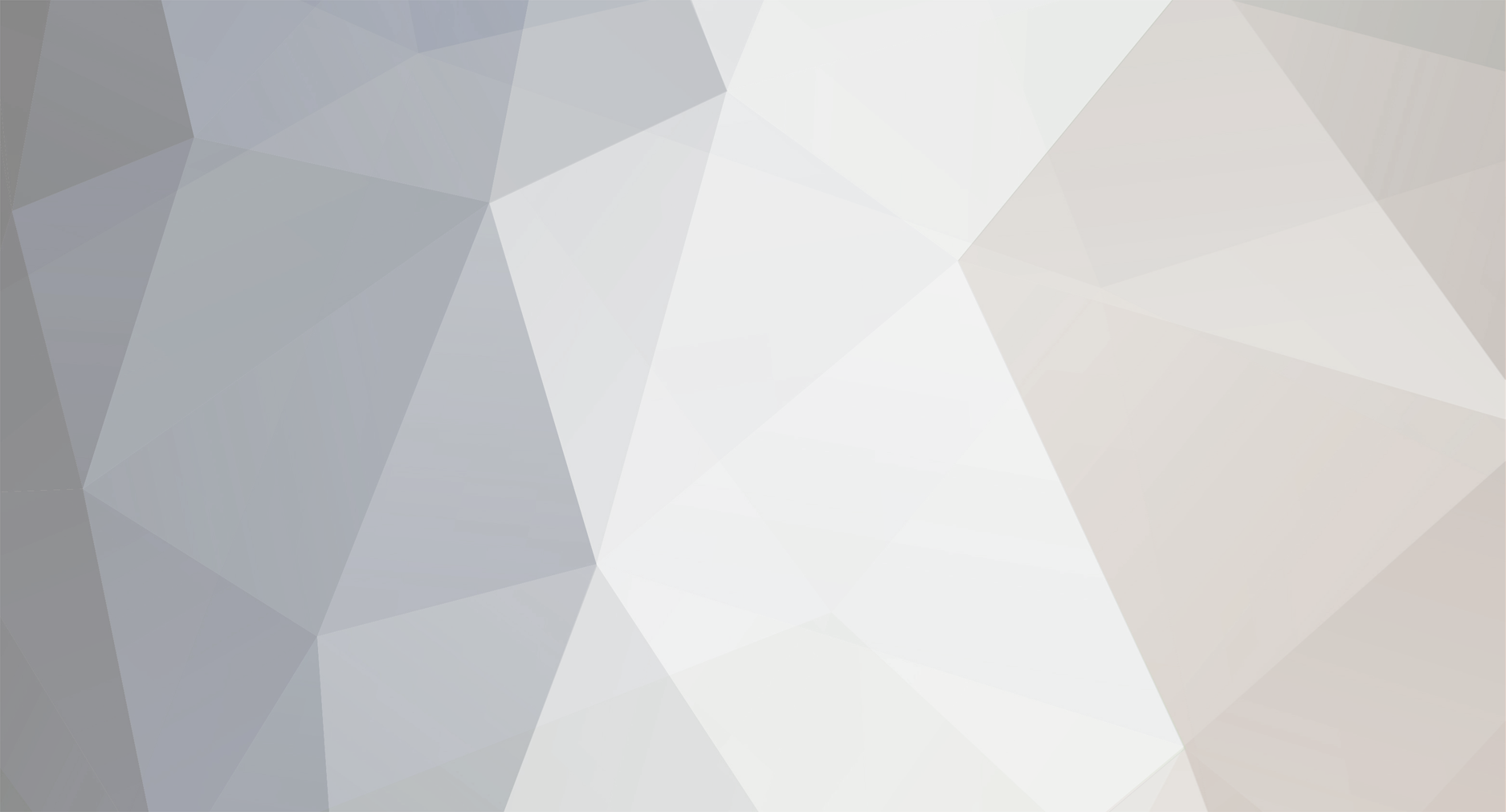
pocobueno1388
Members-
Posts
3,369 -
Joined
-
Last visited
Never
Everything posted by pocobueno1388
-
Okay, here is an example. <?php $query = mysql_query("SELECT username FROM table"); $row = mysql_fetch_assoc($query); //put username into link to profile page echo "<a href='profile.php?user={$row['username']}'>Go to {$row['username']}'s Profile</a>"; ?> Now, on the profile page, the code should look like this: <?php //get username from the URL $username = mysql_real_escape_string($_GET['user']); //Get information about that user from database to display profile information $query = "SELECT * FROM users WHERE username='$username'"; $result = mysql_query($query); $row = mysql_fetch_assoc($result); //Now you can start displaying their profile information with the info from the database. echo "This is {$row['username']}'s Profile page"; ?>
-
check out this site plz and provide feedback
pocobueno1388 replied to jasonc's topic in Website Critique
I couldn't agree more with GuiltyGear. -
You need to use a unique identifier in the URL for that user, such as their username or user ID. Then if the URL looks something like this: www.domain.com/profile.php?user=bob Then you can do a query to the database getting the information you need by using their username, which in this case would be "bob". Let me know if you need further explanation.
-
[SOLVED] help cannot spot syntax error on page.
pocobueno1388 replied to gammaman's topic in PHP Coding Help
Try changing this line: if(("{$_SESSION['admin']['admins']}" == "root") && ("{$_SESSION['admin']['adminpass']}"=="pwd123")){ To if (($_SESSION['admin']['admins'] == "root") && ($_SESSION['admin']['adminpass'] == "pwd123")){ Also, you should be calling session_start() at the TOP of your script. -
$_SESSIONS being passed to one page but not the other, help!!
pocobueno1388 replied to gammaman's topic in PHP Coding Help
You need to call session_start(); at the top of all your pages. -
Need help with a tutorial - syntax error
pocobueno1388 replied to sanderphp's topic in PHP Coding Help
You didn't put the "or" before the "die". So change this line: $sql = mysql_query("SELECT * FROM students") die(mysql_error()); To $sql = mysql_query("SELECT * FROM students")or die(mysql_error()); -
http://www.tizag.com/mysqlTutorial/
-
Use a foreach loop. <?php foreach ($_GET as $key => $val){ echo $key .' - '. $val .'<br>'; } ?>
-
Like this: <?php // OPEN THUMBNAILS DIRECTORY $handle = opendir ('./thumbnails/'); $count = 0; while (false !== ($file = readdir($handle))) { // RECOGNISE FILES if($file != "." && $file != ".." && $file != basename(__FILE__)) { // PRINT THUMBNAIL IMAGE AND LINK TO EACH LARGER IMAGE FOR EVERY IMAGE FOUND print "<a href='./uploadedimages/{$file}' target='_blank'> <img src='./thumbnails/{$file}'></a>"; if ($count == 5){ echo '<br />'; $count = 0; } $count++; } } ?>
-
With HTML? Instead of using <ul> use <ol>.
-
Good places to learn are: www.tizag.com www.w3schools.com
-
Your code looks like this: <option value"4"> You need to put an equals sign in there: <option value="4">
-
UPDATE quests SET ReqItemId1='$last', ReqItemId2='$last', ReqItemId3='$last' WHERE ReqItemId1='{$items['entry']}' Just follow that pattern.
-
[SOLVED] MySQL Record set to array()
pocobueno1388 replied to AndrewJ1313's topic in PHP Coding Help
mysql_fetch_array() -
There was a similar question asked on a different forum, here are the answers:
-
Are you calling session_start() at the top of ALL your pages?
-
Try this: <?php function productList($prod_id, $id) { $str = '<p style="margin-left: 15px; font-size: 14px;"><a href="ticket.php?id='.$id.'&p=prod_3">'.$prod_id.'</a></p>'; return $str; } echo productList('2', $_GET['id']); ?> All I did was assign what you had printing inside of the function to a variable, and I returned it.
-
You could use a delimiter for the select boxes value, that way you can store the ID and email. Like this: <option value="ID-Email">Teams Name</option> Then on the next page, you can just use the explode() function to separate the ID and email by the hyphen.
-
Like this: <?php if (isset($_POST['submit'])){ if (isset($_POST['check'])){ echo "Checked"; } else { echo "Not Checked"; } } ?> <form method="post"> <input type="checkbox" name="check" /> <input type="submit" name="submit" value="Submit" /> </form>
-
Give us your table structures. Also, which field ties table1 and table2 together?
-
Oops, I completely looked over the "AND" that was there. They are right, you need to use "OR".
-
Did you try my code? What didn't work about it? You have this line just by itself: WHERE `id` = '1546' AND `id` = '1' You have to put that in an actual query. That is only part of a query, look at the example I gave you above.
-
<?php $query = mysql_query("SELECT id FROM table WHERE id=1 and id=1546"); while ($row = mysql_fetch_assoc($query)){ echo $row['id'].'<br>'; } ?>