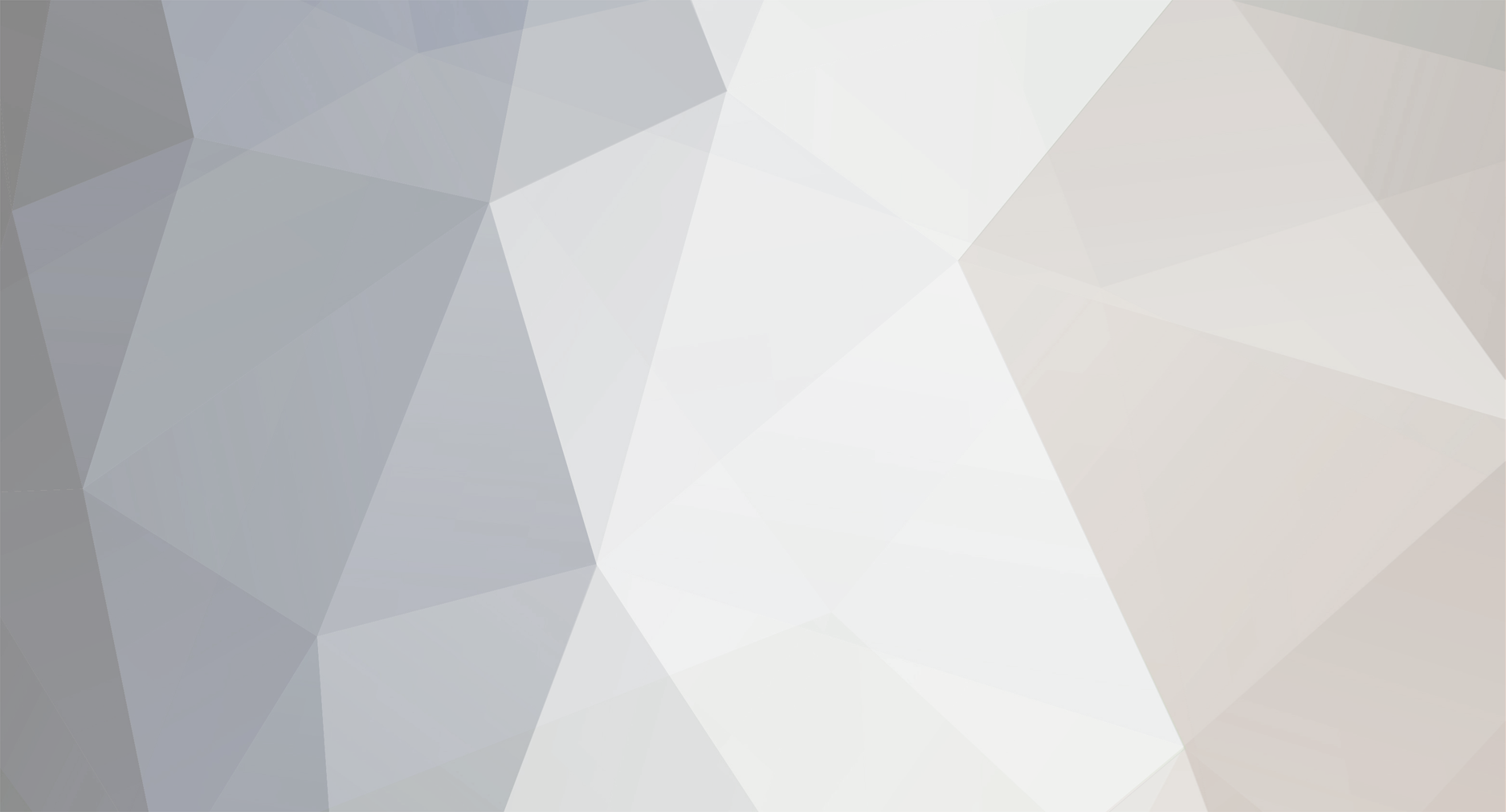
pocobueno1388
Members-
Posts
3,369 -
Joined
-
Last visited
Never
Everything posted by pocobueno1388
-
You can store that part of the URL into a session like this $_SESSION['url'] = $_SERVER['REQUEST_URI']; Do that before they post. Then to redirect them, you can do this: header("Location: {$_SESSION['url']}");
-
These lines shouldn't have two equal signs $error_email == "Invalid Email"; $error_name == "Name was empty"; EDIT Hah, yeah, I was just typing that out as you can see above. Did that fix the problem? Nevermind, I see that you pressed solved.
-
You have all the grids values labeled from v1-v24 which will be stored in the $_POST['R1'] variable. So you will know which part of the grid is selected because they all have different values. Now you would just do a query based on that to see if it matches the one in the database or whatever. If that isn't what your trying to do, you need to explain a little more.
-
$message = nl2br($_POST['message']); That should take care of it.
-
You just had to change all the $input variables to $output, to keep the variable changing. Don't forget to solve.
-
Give this a try <?php function parse($input) { $output = nl2br($input); $output = stripslashes($output); $output = preg_replace("`\[b\](.*)\[/b\]`sUi","<strong>\\1</strong>",$output); $output = preg_replace("`\[i\](.*)\[/i\]`sUi","<em>\\1</em>",$output); $output = preg_replace("`\[u\](.*)\[/u\]`sUi","<u>\\1</u>",$output); $output = preg_replace("`\[url\]([\w]*[:\/\/]*[\w\.\?\/&=\:;, \-@%\?]+)\[/url\]`sUi","<a href='\\1'>\\1</a>",$output); $output = preg_replace("`\[url\=([\w]*[:\/\/]*[\w\.\?\/&=\:;, \-@%\?]+)\](.*)\[/url\]`sUi","<a href='\\1'>\\2</a>",$output); $output = preg_replace("`\[img\]([\w]*[:\/\/]*[\w\.\?\/&=\;, \-@%\?]+)\[/img\]`isU","<img src='\\1' alt='Image' />",$output); return $output; } ?>
-
Post your code so we can see whats going on. Do all the options have the same name attribute?
-
Quickly looking at your code, I found a problem that might just be throwing everything off. In display.php, you have this code. $actualrating = $row2['AVG(rating)']; That isn't the correct way of doing that, you need to change your query to this: $query2 = "SELECT AVG(rating) AS avg_rating FROM ratings WHERE affid = '$affid'"; Now, change the first line of code I posted to this $actualrating = $row2['avg_rating']; See if that changes anything before I look at the code any further.
-
Both of your links are not working. The first one I think you just left out the 's' in options. The second one goes to your site, but gives a 404 error.
-
have you tried putting that query in your phpmyadmin to see what it brings up?
-
You can always test it. On the top of the page it is redirecting to, put this: <?php if (isset($_SESSION['fromurl'])){ echo "It wasn't unset"; } else { echo "The session was unset!"; } ?>
-
You will have to check for a completed answer per required question. Here is an example from your example <?php if (!isset($_POST['questionOne'])){ echo "You did not fill question 1 out!"; } else { //They filled everything out, do the next thing } ?> <body class="bg" style="margin:20px;"> <form action="<? echo $_SERVER['PHP_SELF']; ?>" name="userinfoform" method="POST"> <h2><p>Please enter the appropriate responses and then click on the submit button:</p></h2> <span style="color:#FF0000"></span> <table width="603" border="0"> <tr> <td colspan = 9>1. Are Company X complying with your original and ongoing brief (Score from 1-9)?</td> </tr> <tr> <td><input type="radio" name="questionOne" value="1">1<span style="color:#FF0000"></span> </td> <td><input type="radio" name="questionOne" value="2">2<span style="color:#FF0000"></span> </td> <td><input type="radio" name="questionOne" value="3">3<span style="color:#FF0000"></span> </td> <td><input type="radio" name="questionOne" value="4">4<span style="color:#FF0000"></span> </td> <td><input type="radio" name="questionOne" value="5">5<span style="color:#FF0000"></span> </td> <td><input type="radio" name="questionOne" value="6">6<span style="color:#FF0000"></span> </td> <td><input type="radio" name="questionOne" value="7">7<span style="color:#FF0000"></span> </td> <td><input type="radio" name="questionOne" value="8">8<span style="color:#FF0000"></span> </td> <td><input type="radio" name="questionOne" value="9">9<span style="color:#FF0000"></span> </td> </tr> </table> The answer they chose from that question will be stored in $_POST['questionOne']
-
Oh I see now. This line $q->insertUser($user,$pass,$email,$sid,$time); probably isn't in scope (the $q) since it is inside the defining of a function. So maybe create your $q object right before that line.
-
Try putting this: <?php if (isset($_COOKIE['lala'])){ setcookie("lala", "lalala", time() -3600); } else { echo "This cookie doesn't exist"; } ?>
-
[SOLVED] Add commas to numbers 1034 > 1,034
pocobueno1388 replied to papaface's topic in PHP Coding Help
<?php $num = "16546981"; echo number_format($num); ?> -
[SOLVED] Database value not the same as FORM value displayed
pocobueno1388 replied to paulrjacques's topic in PHP Coding Help
Try putting quotes around the variable, like this <input type="text" name="city" size=20 maxlength=20 align="center" value="<?php print $row['city']; ?>"> You also forgot the semi-colon to end the print statement. -
Regex isn't going to be able to detect existence. Just the format of the email. Such as it has to have the @ symbol.
-
Your probably never initialized the $db variable as an object. You use it here $db->query($sql); Before that did you ever do this? $db = new db_class;
-
but it is valid to have a email of me@something.somethingelse.somethingelse.com I guess in this case that regex doesn't do much good. I use it on my site for registration, but the users also must validate their email...so it works fine in my case.
-
Thats true. That regex only validates that the format is correct.
-
Here is the regex to check the email. <?php if (!eregi("^[_a-z0-9-]+(\.[_a-z0-9-]+)*@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$", $email)) die("Invalid Email!"); ?>
-
[SOLVED] T_CONSTANT_ENCAPSED_STRING ????? Where??
pocobueno1388 replied to tommyda's topic in PHP Coding Help
<?php if ($rating > 5){ die ("Error: Rating must be between 1 and 5 please contact the support team"); } ?> -
Yeah, that can do it =] The Broncos lost tonight, and I'm still angry
-
<?php $newtxt =explode(" ",$txt['bio']); for ($i=0; $i<15; $i++){ echo $newtxt[$i].' '; } ?> There could be a better way...but that works. Teng84 - They didn't say they wanted 15 words per LINE, they want 15 words total. At least from what I understand.