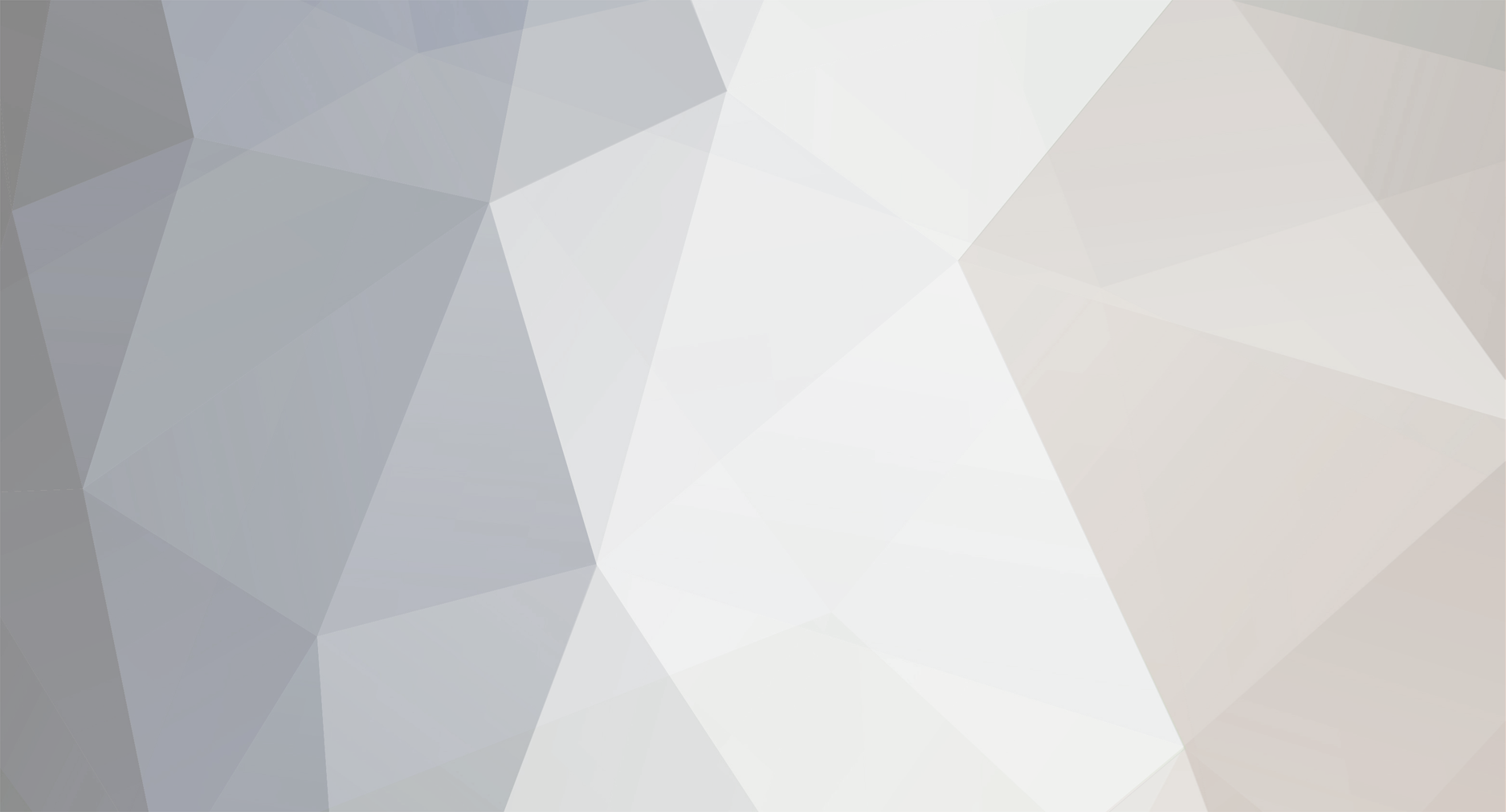
dptr1988
Members-
Posts
372 -
Joined
-
Last visited
Never
Everything posted by dptr1988
-
the word 'like' is a mysql keyword You need to quote that column name in backticks(`)
-
[SOLVED] Using HTML Buttons to update SQL Server
dptr1988 replied to digitalELP's topic in PHP Coding Help
You need to include the SQL ID code for that news item. It would be a lot easier of you didn't have to user buttons ( ie HTML forms ). Here is an example that just uses links. You will need to modifiy it to include the name of your row id field and your URLS. <?php if (isset($_GET['show'])) { $id = 0 + $_GET['show']; $query = "UPDATE news SET expiration_date_field = 0 WHERE id = " . $id; mysql_query($query); } elseif(isset($_GET['hide'])) { $id = 0 + $_GET['hide']; $query = "UPDATE news SET expiration_date_field = 1 WHERE id = " . $id; mysql_query($query); } $query="SELECT * FROM news ORDER BY news.newsID DESC"; $result=mysql_query($query) or die ("ERROR! ".mysql_error()); while($Row=mysql_fetch_array($result)) { echo "<tr><td>".$Row['title']."</td>" . "<td> <span class=\"h4\">" . date('F d\, Y', $Row['postDate']). "</span></td>" . "<a href='your_page.php?show=" . $Row['id'] . "'>Show</a><a href='your_page.php?hide=" . $Row['id'] . "'>Hide</a>"; } ?> -
There is a lot more to creating a HTTP post script then the url and post data. Could we see the code that you use to send the post request? Are you sure the the code the receives the post request 'http://tektek.org/gaia/worth.php' is working correctly?
-
Have your tried echoing the query and checking if it's correct? Example: <?php $query = "INSERT INTO users (id, first, username, password, second, address, email, job, salary, like, salarye, transport, contact) VALUES ($id, $first, $username, $password, $second, $address, $email, $job, $salary, $like, $salarye, $transport, $contact)"; echo $query; $result =mysql_query($query); ?> Also, why are you suppressing errors on the mysql_query() function? What error messages are you getting? Do you even have a mysql connection?
-
I have not done much from users side of OSCommrece, but I have made modifications to it for other people, and it's source code is a mess. Just recently, they were working on rewriting the code so that it didn't require register globals!! I would reccomend that you look for something else besides OSCommrece.
-
Send email at Specific Time ? Automatically
dptr1988 replied to Zyphering's topic in PHP Coding Help
If you have enough visitors on your website, you can use them as a 'cron' daemon! Each time somebody loads a page, check if it's 07:00 and if it is, you can send the email. It's not guranteed to send at the right time, but if you get a lot of traffic, it would be pretty accurate. But this is a last resort for people that don't have any type of cron system. Always use cron if you can. -
You can't unless you use AJAX. The server doesn't know when you close your browser window.
-
Check if the directory exists with file_exists () and then create the directory with mkdir() if needed. http://us2.php.net/file_exists http://us2.php.net/manual/en/function.mkdir.php
-
help echo different rows based on (if not null)
dptr1988 replied to gammaman's topic in PHP Coding Help
If a variable is 'NULL' in PHP it's almost like it doesn't exist. What you need to do is check for an empty string like this: <?php if ($grade != "") { $value='<form action="updateGrades.php" method= "post">'; echo "<br />" . '<input name = "grd" type="text" />'; }else{ $value = $grade } ?> -
Opps! Thanks for pointing that out.
-
Sessions should last for session.gc_maxlifetime or when the user closes his browser
-
I have a sample login system that I'm using as an example in a tutorial. I was wondering if somebody could go over it and see if they find any security related bugs or any other undesirable features. In fact, any type of criticism would be appreciated. The only reason I'm asking this is because I emphasize security in my tutorial, and it would be very embarrassing if my example code had a serious security flaw! Here is the code: <?php session_start(); // Make sure that these are stored in a secure place // where nobody will find them $database_salt = 'a@pu#qz$kv%!1&2*(2_3233l;k345{[}`235jsd '; // Who ever heard of putting salt on your cookies? $cookie_salt = 'adh;234509()_*!#$HKsdMN$35Dfh-9ihsdfk'; function hash_password($password, $salt) { $counter = strlen($password); $c2 = strlen($salt); if ($c2 < $counter) { $counter = $c2; } $salted_string = ''; for ($i = 0; $i < $counter; $i++) { $salted_string .= $password[$i] . $salt[$i]; } return md5($salted_string . $salt); } function no_login($error_message) { // Make sure that they are logged out. unset($_SESSION['login_id']); setcookie('login_username', '', time()-60*60, '/', 'example.com'); setcookie('login_password', '', time()-60*60, '/', 'example.com'); echo "<html><head><title>login required</title></head>" . "<body><p>{$error_message}</p>" . "<form action='{$_SERVER['REQUEST_URI']}' method='post'>" . "<p>Username: <input type='text' name='login_username' value='' ></p>" . "<p>Password: <input type='password' name='login_password' value='' ></p>" . "<p>Remember me <input type='checkbox' name='login_usecookie' value='' ></p>" . "<input type='submit' name='login_submit' value='Login' >" . "</form></body></html>"; exit(); } // Assumes that you have already setup the database connection // somewhere else in the file function db_mysql($username) { $login_info = array(); $username = mysql_real_escape_string($username); $query = "SELECT userid, username, password, user_permissions " . "FROM login_users WHERE username = '{$username}' LIMIT 1"; $result = mysql_query($query); if ($result === false) { $login_info['success'] = false; $login_info['message'] = "ERROR: Unable to access user information!"; return $login_info; } $data = mysql_fetch_assoc($result); if ($data === false) { $login_info['success'] = false; $login_info['message'] = "Invalid username."; return $login_info; } $login_info['success'] = true; $login_info['id'] = $data['userid']; $login_info['permissions'] = $data['user_permissions']; $login_info['password_type'] = 'db_hashed'; $login_info['password'] = $data['password']; return $login_info; } function db_array($username) { $users_passwords = array( array('joe_sample', 'password', 'r|w|e'), array('john_sample', 'password', 'r'), array('harry', 'asdf', 'r|w|e|d|c'), array('test', 'test_password', 'r|w|c') ); foreach($users_passwords as $id=>$info) { if ( $info[0] == $username) { return array( 'success' => true, 'id' => $id, 'permissions' => $info[2], 'password_type' => 'plain_text', 'password' => $info[1] ); } } // We didn't find anybody by that name in the list return array( 'success' => false, 'message' => 'Invalid username or password.' ); } // This loads a serialized array from a file and // passes it on to db_array() function db_serialized_array($username) { $password_file = '/path/to/password/file.dat'; $data = file_get_contents($password_file); if ($data === false) { return array('success' => false, 'message' => 'ERROR: User database not found.'); } $user_list = unserialize($data); if ($user_list === false) { return array('success' => false, 'message' => 'ERROR: Unrecognized user database format.'); } foreach($user_list as $id=>$info) { if ( $info[0] == $username) { return array( 'success' => true, 'id' => $id, 'permissions' => $info[2], 'password_type' => 'db_hashed', 'password' => $info[1] ); } } // We didn't find anybody by that name in the list return array( 'success' => false, 'message' => 'Invalid username or password.' ); } // This function takes a username/password combination and // checks it against the database If it's correct, it will // return information about the user, otherwise it will // print out the login form with an error message function do_login($username, $password_info) { global $cookie_salt, $database_salt; // Here you can select the database that you want // to use for the user logins //$result = db_serialized_array($username); $result = db_array($username); //$result = db_mysql($username); if (!$result['success']) { no_login($result['message']); } $db_pw_type = $result['password_type']; $usr_pw_type = $password_info['password_type']; $passwords_matched = ( ($usr_pw_type == 'plain_text' AND $db_pw_type == 'plain_text' AND $result['password'] == $password_info['password'] ) OR ($usr_pw_type == 'cookie_hashed' AND $db_pw_type == 'plain_text' AND hash_password($result['password'], $cookie_salt) == $password_info['password'] ) OR ($usr_pw_type == 'plain_text' AND $db_pw_type == 'db_hashed' AND $result['password'] == hash_password( $password_info['password'], $database_salt) ) OR ($usr_pw_type == 'cookie_hashed' AND $db_pw_type == 'db_hashed' AND hash_password($result['password'], $cookie_salt) == $password_info['password']) ); if (!$passwords_matched) { no_login('Wrong password'); } $permissions = explode('|', $result['permissions']); $_SESSION['login_id'] = $result['id']; $_SESSION['login_username'] = $username; $_SESSION['login_permissions'] = $permissions; } function check_permissions($permissions_required) { if (!in_array($permissions_required, $_SESSION['login_permissions']) ) { echo "You need permission {$permissions_required} " . "to access this page"; exit(); } } // Are they wanting to log out? if (isset($_GET['log_out']) AND $_GET['log_out'] == 1) { $_SERVER['REQUEST_URI'] = $_SERVER['PHP_SELF']; no_login('You are now logged out'); } // Always allow them to login, even if they are already logged in if (isset($_POST['login_submit'])) { $username = trim($_POST['login_username']); $password = trim($_POST['login_password']); do_login($username, array('password_type' => 'plain_text', 'password' => $password) ); // if do_login() returns, then the user has logged in // If the user wants a cookie, give him one if (isset($_POST['login_usecookie'])) { $cookie_password = hash_password(hash_password($password, $database_salt), $cookie_salt); setcookie('login_username', $username, time()+60*60*24*30, '/', 'example.com'); setcookie('login_password', $cookie_password, time()+60*60*24*30, '/', 'example.com'); } header("Location: http://{$_SERVER['HTTP_HOST']}" . "{$_SERVER['REQUEST_URI']}"); } // If not logged in if (!isset($_SESSION['login_id'])) { // Check for a cookie if (isset($_COOKIE['login_username']) AND isset($_COOKIE['login_password']) ) { do_login($_COOKIE['login_username'], array('password_type' => 'cookie_hashed', 'password' => $_COOKIE['login_password'])); } else { no_login("Please login to view this page."); } } check_permissions('d'); // Create an log out link that can be used later in the page $log_out_link = "<a href='{$_SERVER['PHP_SELF']}?log_out=1'>Log Out</a>"; echo "You are now in a protected page, logged in as {$_SESSION['login_username']} " . "and have the required permission 'd'\n\n\n{$log_out_link}"; ?>
-
PHP doesn't require any compilation. And most IDE's are slow to load, always have something popping up in your face and have the screen cluttered with useless junk. So just a good editor like VIM works for me.
-
This seems to work in my server. Try it out on your server <?php //$uri = $_SERVER["REQUEST_URI"]; //$ipserver = $_SERVER["SERVER_ADDR"]; //$resuelveserver = gethostbyaddr($ipserver); //$IP = getenv("REMOTE_ADDR"); //$host = gethostbyaddr($IP); // For debugging $host = "239-12-92-86.rdsnet.ro."; $banhosts = array(".us","email",".cc",".de",".hk", ".ro"); $x = count($banhosts); //$notfound = "<HTML><HEAD> //<TITLE>404 Not Found</TITLE> //</HEAD><BODY> //<H1>Not Found</H1> //The requested URL $uri was not found on this server.<P> //<HR> //<ADDRESS>Apache/1.3.34 Server at $resuelveserver Port 80</ADDRESS> //</BODY></HTML>"; var_dump($banhosts); for ($y = 0; $y < $x; $y++) { $result = strpos($host ,$banhosts[$y]); echo "Result for {$banhosts[$y]}: " . var_dump($result) . "\n"; if ($result !== false) { // echo $notfound; echo "Banned Host"; } } ?>
-
Yes, that looks correct.
-
Yes, that was the only error I found in the code, and that is why I think it was not working.
-
In the file 'index.php' your are only using 1 equals sign where you should be using 2 equal signs ('==') to do the comparision. <?php // BAD if($passwordFromCookie = $encryptedPass) //Good if($passwordFromCookie == $encryptedPass) ?> Also where is the variable $encryptedPass setup? It looks like a uninitialzed var.
-
Your need code does does not take the values from the $_POST variable like they should Example: // BAD "UPDATE `grpgusers` SET `avatar`='".$avatar."', WHERE `id`='".$user_class->id."'" // GOOD "UPDATE `grpgusers` SET `avatar`='".$_POST['avatar']."', WHERE `id`='".$user_class->id."'"
-
phpinfo(); will display the location of the PHP.INI that it is using.
-
Are you sure that you don't have duplicate data in the database? I don't see any reason why the code would be printing out duplicate data.
-
[SOLVED] Checkboxes to delete rows in members table...
dptr1988 replied to amsgwp's topic in PHP Coding Help
Or even better: <?php if (isset($_POST['delete'])) { $sql = "DELETE FROM Members WHERE Member_ID IN (" . implode(', ', $_POST['checkbox']) . ")"; $result = mssql_query($sql); } ?> -
That was my fault sorry. try using phpinfo(); to locate your php.ini
-
Search for 'error_reporting' in your PHP.ini. It could be anywhere
-
try something like this <?php for($i = 1; $i <= 10; $i++) { if ($isset($_POST["fieldcheck" . $i])) { // Get the data and process it $name = $_POST["name" . $i]; // etc } } ?>
-
[SOLVED] Checkboxes to delete rows in members table...
dptr1988 replied to amsgwp's topic in PHP Coding Help
Try this DELETE FROM members WHERE id IN (1,2,3,4,5,6)