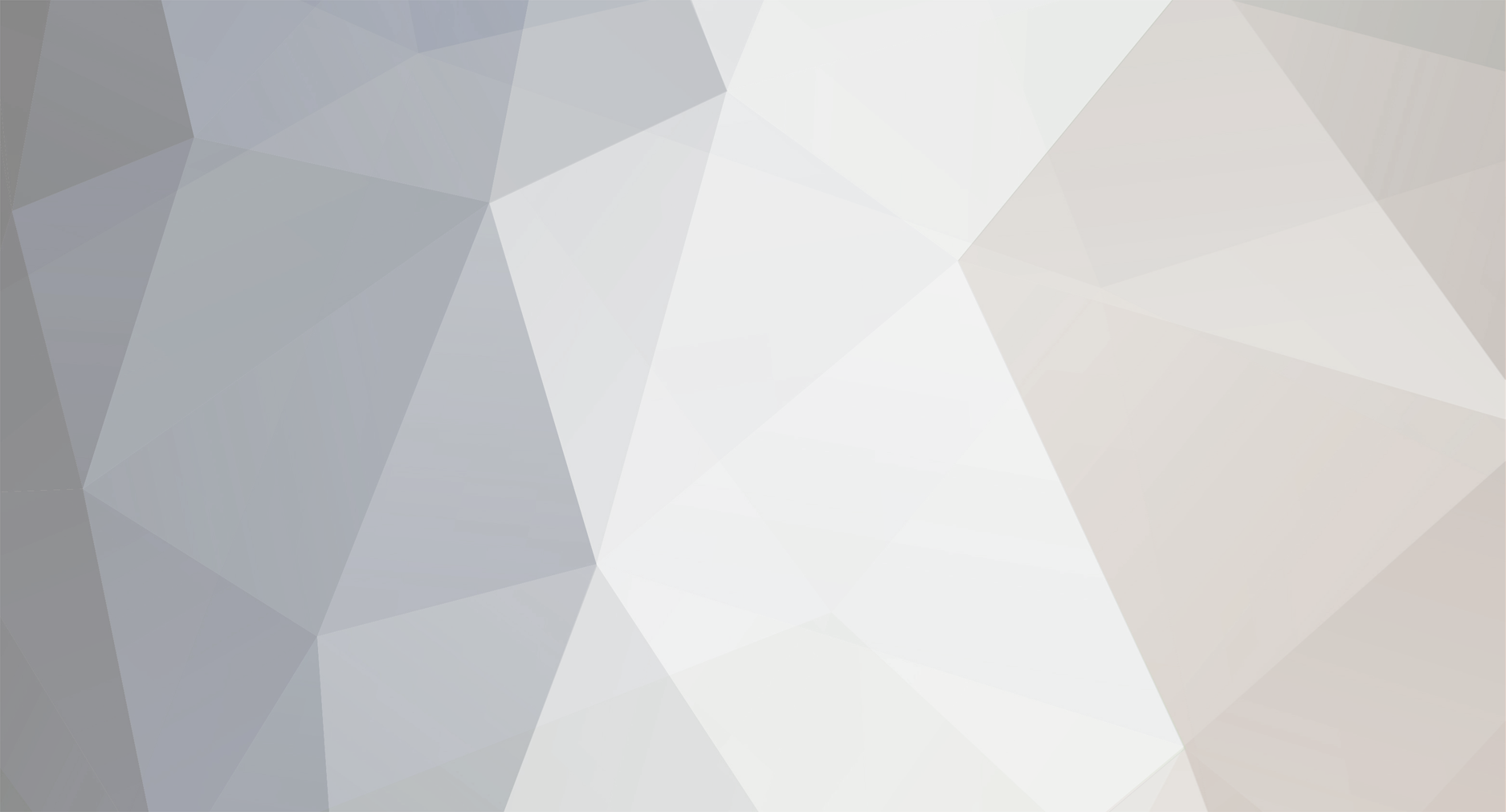
tomfmason
Staff Alumni-
Posts
1,693 -
Joined
-
Last visited
Everything posted by tomfmason
-
You should sanitize all user input. For example: <?php $name=mysql_real_escape_string($_POST['name']); $em=mysql_real_escape_string($_POST['mail']); ?> I would also suggest that you read up on sql injection
-
You should post some of the relevant code as I doubt many, if any, people will download your zip. If you are using JQuery there are several existing plugins that should make your life a lot easier. Otherwise you could use a hidden textfield to store the original date format for use in the db and then use something like this to convert that into a more user friendly version.
-
Trying to style buttons in JavaScript Slide-Rotator
tomfmason replied to doubledee's topic in Javascript Help
I checked it out in firebug and it would seem that the best way would be to simply change the css properties for .jshowoff-active (for active links),.jshowoff-slidelinks a (for inactive links) and .jshowoff-controls a (for the controls) -
a simple way could be something like <?php $data = "some data \n"; $file = '/path/to/file'; $data .= file_get_contents($file); file_put_contents($file,$data); ?>
-
I believe the problem is with the quotes around your arguments in the onclick. You have: <a href="#" onclick="removeCharacter(<?php echo $row['ID'] . ','. "'" . $row['characterName'] . "'"; ?>);">Remove</a> When it should be: <a href="#" onclick="removeCharacter(<?php echo "'{$row['ID']}', '{$row['characterName']}'"; ?>);">Remove</a>
-
You reference a function called removeCharacter in your onclick but the function posted is named remove. Also, I am not really sure why you are setting a new event handler in your remove function. The remove function should be the only event handler needed imo. Here is an example that should do what you are wanting. function remove(characterID, characterName) { event.preventDefault(); $(this).parent().remove(); var option = = $( '<option value=' + characterID + '>' + characterName + '</opton>' ); $( '#charactersDrop' ).append( option ); } You should also notice that I used event.preventDefault() which is a better common practice for preventing the default event handler(for links, forms ect.) than returning false.
-
you are still setting the value of PaymentMethods to Cheques instead of comparing the actual value. Once again, '=' assigning a value and '==' is used for comparison. Assigning a variable: foo = 1; Comparing the variable if(foo == 2) { alert('foo is equal to two'); } you have VarChequesCBtoTB = (document.getElementById("PaymentMethods").value="Cheques"); VarCreditCard = (document.getElementById("PaymentMethods").value="Credit Card"); if(VarChequesCBtoTB == 'Cheques') {} In that snippet you are assigning the value of to PaymentMethods 'Cheques' and then again on the next line to 'Credit Card' Assignment (document.getElementById("PaymentMethods").value="Cheques"); Comparison (document.getElementById("PaymentMethods").value=="Cheques"); In either case the variables you are assigning there would be boolean(true/false) and not a string as you expect. You may want to read over the w3schools documentation on assignment operators and javascript comparison operators Here is a simplified example of your code: pm = document.getElementById("PaymentMethods").value; if(pm=='Cheques') { //your Cheques related code } else if(pm == 'Credit Card') { //your Credit Card related code } Also, you may want to use proper indention(either tabs or spaces) when coding. It will make it easier to read, debug and work with in general.
-
the problem is the fact that you are using one '=' in your if statement which is setting the value of 'PaymentMethods' to 'Cheques'. use one '=' to set a value and two('==') for evaluation. for example: foo = 'something';//set the value if(foo == 'something') { //evaluated the value of foo }
-
can you post some of your code? Also, what autocomplete lib, if any, are you using?
-
you could simply use json instead. for example: var fields = {'game_type':'Game Type','game_abbreviation' : 'Game Abbreviation'};
-
Cron Job to check the creation date of a MySQL Database then delete it
tomfmason replied to spinner0205's topic in Linux
A simple shell script could be something like this #!/usr/bin/env bash #get the unix timestamp from 30 days ago ENDTIME=`date --date="30 days ago" +%s` #delete all records that are 30 or more days old. mysql -u user -p'password' -e "DELETE FROM your_table WHERE UNIX_TIMESTAMP(date_field) <= $ENDTIME;" You will obviously need to replace "your_table" and "date_field" with the relevant table name and date field. Be careful with the above script as it will delete all records that are 30 or more days old. You may want to edit the where clause to suit your specific needs. -
I just called another spammer on her home phone. Needless to say she was very surprised to be hearing from me directly. I may have found my purpose in life.... get rich screwing over spammers
-
I just got off the phone with my attorney regarding some sexually explicit emails that I have been receiving on a daily basis. I tried to opt out of their emails over 30 days ago and since that I date I have received at least one email a day. The attorney told me that my state allows up to $25k a day damages starting from the first opt out attempt. Needless to say he was very eager to pursue legal action
-
apparently Australia also has anti spam laws - http://www.dbcde.gov.au/online_safety_and_security/spam/ I am seriously considering doing this my self as well. The state that I live in has odd laws regarding "adult" emails and from the looks of it they will make it very easy to file a lawsuit against porn spammers.
-
A friend of mine pointed out an article where a man is actively filing law suits against spammers. I was not aware of this but apparently most states in the US have anti-spam laws in place with California being one of the most strict. Basically this guy wades through his spam box looking for US based companies that violate the California anti-spam laws and files a lawsuit against them in small claims court. Apparently the vast majority of these companies settle out of court and from what I gathered from the article above he has made a healthy sum of money from doing so.
-
This topic has been moved to PHP Coding Help. http://www.phpfreaks.com/forums/index.php?topic=319232.0
-
This topic has been moved to PHP Freelancing. http://www.phpfreaks.com/forums/index.php?topic=319234.0
-
HTML5 <audio> and why it will convince people to change browser.
tomfmason replied to ignace's topic in Miscellaneous
Great idea imo. -
what distro are you using? If you search "distro lamp setup tutorial"(obviously replace 'distro' with your distro - debian,CentOS etc) you should find plenty of detailed tutorials covering the setup.
-
This topic has been moved to Linux. http://www.phpfreaks.com/forums/index.php?topic=319197.0
-
Seriously? You have been given several different examples that should work with small modifications. Please don't be this guy -
-
Here is how I would do it first turn on the RewriteEngine RewriteEngine on To solve the problem with css and images don't rewrite existing files RewriteCond %{REQUEST_FILENAME} !-f Assuming your 'id' is an integer you would want to do something like this RewriteRule ^vest/([0-9]+)$ vesti.php?id=$1 [L] This should rewrite urls like /vest/123 to vesti.php?id=123 . I didn't test this but it should work without any problems
-
i think (off the top of my head) preg_match('/^\(?\d{3}\)?[\.\- ]?\d{3}[\.\- ]\d{4}$/',$phone): should capture: (xxx) xxx-xxxx (xxx)-xxx-xxxx xxx.xxx.xxxx xxxxxxxxxx or any combination thereof that is pretty much exactly what I posted except you don't have to escape the - e.g. '/^\(?(\d{3})\)?[-\. ]?(\d{3})[-\. ]?(\d{4})$/'
-
It is important to note that if the string matches the given validation a boolean is returned(true) and if it doesn't match an array is returned like this: <?php $validation = new Validation(); echo nl2br(print_r($validation->validate('phone','(555)---555.1212'),true)); ?> would return Array ( [phone] => is not a valid US phone number format. ) You can also use this for zip codes(XXXXX or XXXXX-XXXX etc) and email addresses.