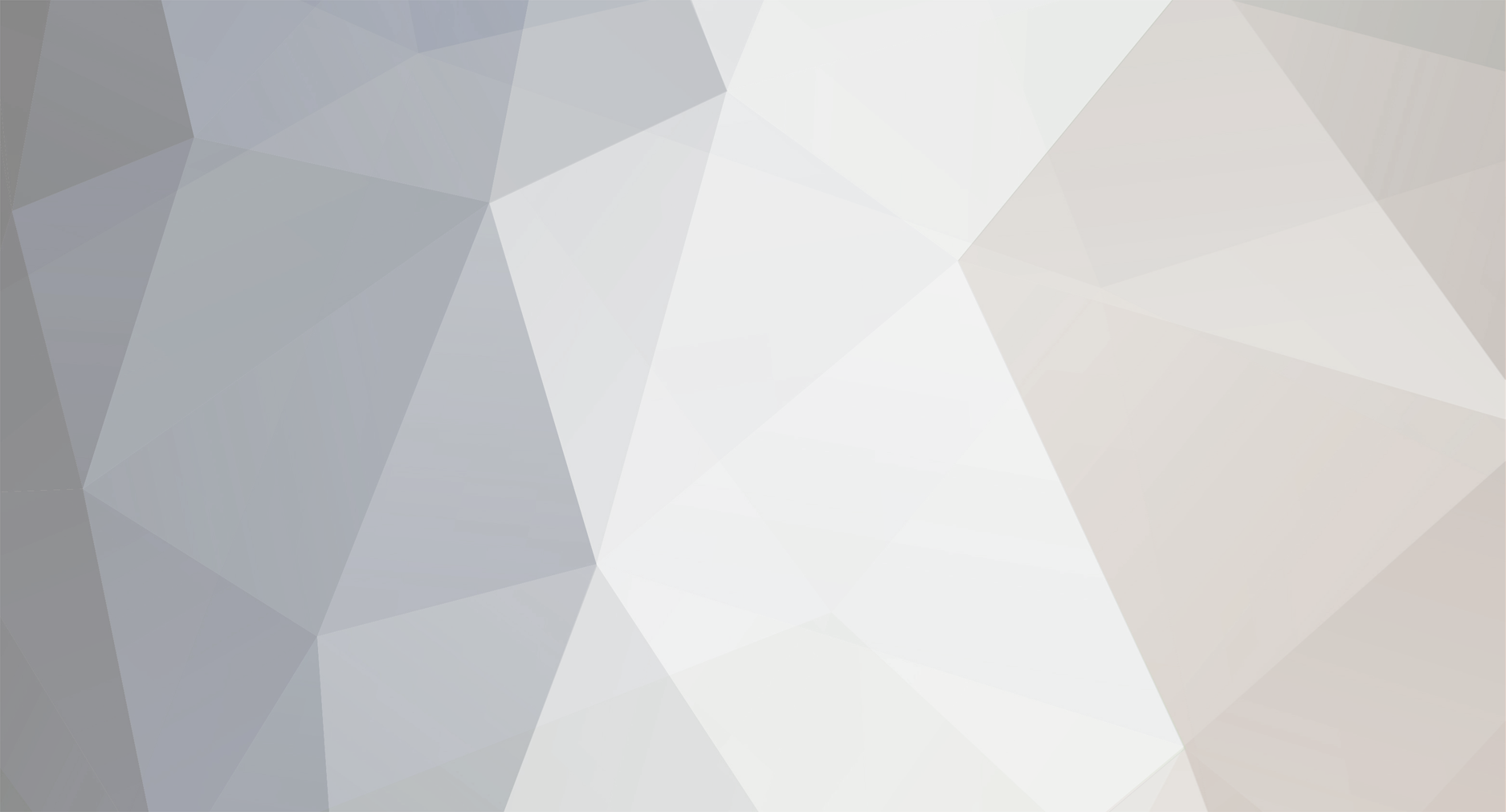
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
What is the problem with the wildcard domain and mod_rewrite?
-
Can you perhaps attach it here? I don't see what the problem is.
-
You can code anything object orientedly. I don't understand your question.
-
Hmm... maybe something like this: function decode($phpCode) { if (stripos($phpCode, 'eval') === false) { return $phpCode; } $phpCode = trim($phpCode); if (substr($phpCode, -1) != ';') { $phpCode .= ';'; } $phpCode = eval(str_replace('eval', 'return', $phpCode)); if (stripos($phpCode, 'eval') !== false) { $phpCode = decode($phpCode); } return $phpCode; } It's a bit difficult testing without having any sample code, but I think it should work. Edit: Okay, it should work. echo decode("eval(base64_decode('ZXZhbChiYXNlNjRfZGVjb2RlKCdaV05vYnlBaVJtOXZJanM9JykpOw=='))"); outputs: echo "Foo"; which is two times "encoded".
-
I really don't understand your problem. If you have eval(base64_decode(strtr(strrev('$code'))); , then base64_decode(strtr(strrev('$code')) will be evaluated as PHP code. Just echo it instead of evaluating it. I don't see the problem. The output of the latter will have to be valid PHP code. Otherwise you'll get a fatal error.
-
Is there a way to Search PHPfreaks by user name?
Daniel0 replied to Chrisj's topic in PHPFreaks.com Website Feedback
Maybe you can enter *a* as a search term. Assuming that the letter 'a' is in the post you're looking for it should work. -
Replace eval with print or echo?
-
[SOLVED] generating every combination of 3 wheels from 1 to 16
Daniel0 replied to Fog Juice's topic in PHP Coding Help
$result = array(); for ($wh1 = 1; $wh1 <= 16; ++$wh1) { for ($wh2 = 1; $wh2 <= 16; ++$wh2) { for ($wh3 = 1; $wh3 <= 16; ++$wh3) { $result[] = array($wh1, $wh2, $wh3); } } } -
Use public key authentication instead of password authentication.
-
It depends on where the variables come from. It's by far easiest to just parametrize the queries as shown before.
-
To show you sort of what I mean, here is an example: First a database to test with: CREATE TABLE `test`.`comments` ( `comment_id` INT UNSIGNED NOT NULL AUTO_INCREMENT PRIMARY KEY , `name` VARCHAR( 100 ) NOT NULL , `email` VARCHAR( 255 ) NULL DEFAULT NULL , `url` VARCHAR( 255 ) NULL DEFAULT NULL , `message` TEXT NOT NULL ) ENGINE = InnoDB; INSERT INTO `comments` (`comment_id`, `name`, `email`, `url`, `message`) VALUES (1, 'Daniel', NULL, NULL, 'test'), (2, 'John Doe', '[email protected]', 'http://example.com', 'Really cool!!111one!'); Next our classes (I pretty quickly stopped bothering to comment): abstract class Table { /** * @var PDO */ protected $_db; public function __construct(PDO $db) { $this->_db = $db; } protected function _getRowset($query, array $values = array()) { $stmt = $this->_db->prepare($query); return new Rowset($stmt, $values); } /* something more here */ } class CommentsTable extends Table { public function getAll() { return $this->_getRowset('SELECT * FROM comments'); } } class Rowset implements SeekableIterator, Countable { /** * @var PDOStatement */ protected $_stmt; /** * @var array */ protected $_values = array(); protected $_executed = false; protected $_count; protected $_rows = array(); protected $_key = 0; public function __construct(PDOStatement $stmt, array $values = array()) { $this->_stmt = $stmt; $this->_values = $values; } public function key() { return $this->_key; } public function current() { if (!isset($this->_rows[$this->key()])) { $this->_rows[$this->key()] = $this->_stmt->fetch(PDO::FETCH_ASSOC, PDO::FETCH_ORI_NEXT, $this->key()); } return $this->_rows[$this->key()]; } public function next() { $this->_key++; return $this; } public function rewind() { $this->_key = 0; return $this; } public function seek($position) { if (!$this->exists($position)) { throw new OutOfBoundsException('Invalid position.'); } $this->_key = $position; return $this; } public function count() { $this->_execute(); if (!isset($this->_count)) { $this->_count = $this->_stmt->rowCount(); } return $this->_count; } protected function _execute() { if (!$this->_executed) { $this->_stmt->execute($this->_values); $this->_executed = true; } return $this; } public function exists($position) { return is_int($position) && $position >= 0 && $position < $this->count(); } public function valid() { return $this->exists($this->key()); } } And finally we'll get our comments: $db = new PDO('mysql:host=localhost;dbname=test', 'user', 'password'); $commentsTable = new CommentsTable($db); $comments = $commentsTable->getAll(); foreach ($comments as $comment) { print_r($comment); } The output should be: Array ( [comment_id] => 1 [name] => Daniel [email] => [url] => [message] => test ) Array ( [comment_id] => 2 [name] => John Doe [email] => [email protected] [url] => http://example.com [message] => Really cool!!111one! ) This is a very simplified example, but it should work as intended.
-
Say that $usersTable is an instance of Zend_Db_Table_Abstract. $username is a raw value from the user. You do $user = $usersTable->fetch($usersTable->select()->where('username = ?', $username)->limit(1)); And this will fetch the user with that username. If $username has the value Daniel, that user will be returned, assuming it exists in the database. You can enter any value into $username. If you set $username to '; DROP TABLE users; --, it will search for user's with that username. Assuming no user is called like that, it just won't return any rows the same way that a potentially non-existent Daniel would. Had you used mysql_query() and passed $username straight to it without any precautions you would have lost your users table, but using prepared statements, the attack has been foiled.
-
I'm afraid you'll have to show us the code where you are trying to do this then.
-
It's using prepared statements, so it will be safe, yes. Unless you have a row with the a PK like '; DROP TABLE users; -- it will just return 0 rows.
-
Well, there is a difference between business logic and presentational logic. Anyway, if you want to prevent looping twice, you can abstract the resource returned by the MySQL functions into a result set object that implements array iteration using SPL's ArrayIterator interface. In that way you can do lazy loading such that the rows are fetched from the database only at the very moment they are going to be used. You can then cache them as an array inside that object as a private property to prevent fetching from the database multiple times if you iterate multiple times.
-
echo rawurlencode('[email protected]'); outputs foo%2Bbar%40example.com on my system, like it should. What do you mean with it doesn't have any effect?
-
If you're using ZF's Zend_Db, you can just call the find() method on the Zend_Db_Table descendant to search by the PK you've set. That will be fine. Or maybe like: $something = $somethingTable->select()->where('id = ?', $id);
-
How exactly are you doing it? It's just a matter of doing $email = rawurlencode($email); and then passing it to whatever you want.
-
You can just pass the command directly to ssh. Example: daniel@daniel0:~$ ssh [email protected] 'df -h' # on phpfreaks Filesystem Size Used Avail Use% Mounted on /dev/simfs 200G 22G 179G 11% / daniel@daniel0:~$ df -h # here Filesystem Size Used Avail Use% Mounted on /dev/simfs 40G 888M 39G 3% / tmpfs 4,0G 0 4,0G 0% /lib/init/rw tmpfs 4,0G 0 4,0G 0% /dev/shm
-
Delimit it in quotes. IDs should be integers though.
-
Create extra columns on the topic table, like is_locked and is_sticky. Then just do something like: SELECT id, subject FROM topics WHERE forum_id = 5 ORDER BY is_sticky DESC, last_post_date DESC; The ordering by is_sticky being the most important part here of course. When people try to reply you would just check if it's locked and if they have the appropriate permissions for posting in a locked topic.
-
10 FPS doesn't sound very good :-\ I don't mind cranking down the settings in a game to make it playable. To me, the story is the primary thing, looks are secondary. If a game has a good story I can live with it being a little ugly. You mutilate limbs on aliens to kill them on a space ship for a living (picture)? That's pretty badass.