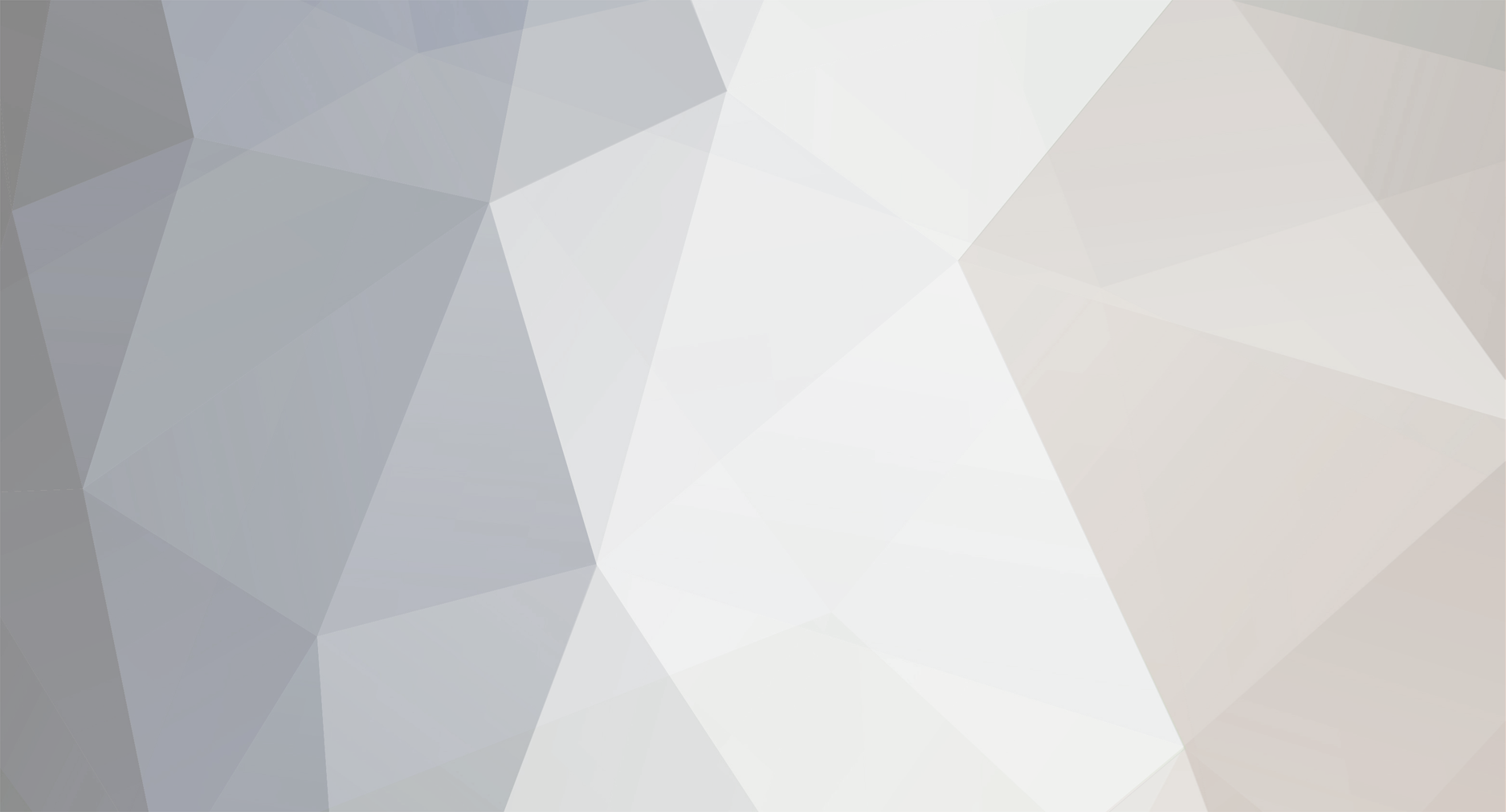
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
I was hoping my post describing the algorithm would have suggested an implementation at the same time. function caesarEncode($plaintext, $key) { for ($i = 0, $length = strlen($plaintext); $i < $length; $i++) { if (!ctype_alpha($plaintext[$i])) { continue; } $first = ord($plaintext[$i]) >= ord('a') ? ord('a') : ord('A'); $offset = ord($plaintext[$i]) - $first + $key; $plaintext[$i] = chr($first + $offset % 26); } return $plaintext; } function caesarDecode($ciphertext, $key) { return caesarEncode($ciphertext, -$key); } echo caesarEncode('This is a test', 3); // Wklv lv d whvw echo caesarDecode('Wklv lv d whvw', 3); // This is a test
-
Define "doesn't work". Also, throw out your book and buy a new one. $HTTP_COOKIE_VARS has been deprecated for a loooong time.
-
His previous wife became a zombie during a small outbreak. Ever since has he been very cautiously been making sure he is adequately protected from zombie attacks.
-
Improved version: function generateFraction($numerator, $denominator, $mixed = true) { if ((int) $denominator == 0) { throw new InvalidArgumentException('Cannot divide by zero.'); } if (!$mixed) { return (int) $numerator . '/' . (int) $denominator; } $num = (int) ($numerator / $denominator); $newNumerator = $numerator - $num * $denominator; if ($newNumerator < 0 && $denominator < 0) { $newNumerator *= -1; $denominator *= -1; } if ($num == 0) { return $numerator . '/' . $denominator; } else if ($newNumerator != 0) { return $num . ' + ' . $newNumerator . '/' . $denominator; } else { return $num; } } echo '5/3 = ' . generateFraction(5, 3) . PHP_EOL; echo '5/-5 = ' . generateFraction(5, -4) . PHP_EOL; echo '5/5 = ' . generateFraction(5, 5) . PHP_EOL; echo '-5/-5 = ' . generateFraction(-5, -5) . PHP_EOL; Changelog: 1) Made it into a function. 2) Made sure that the denominator cannot be zero. 3) Adds a plus sign between the integer part and fraction part to be more mathematically correct. 4) Now supports negative nominators and denominators. Output of above code: 5/3 = 1 + 2/3 5/-5 = -1 + 1/-4 5/5 = 1 -5/-5 = 1
-
<?php $numerator = 5; $denominator = 3; $num = (int) ($numerator / $denominator); $newNumerator = $numerator - $num * $denominator; echo $numerator . '/' . $denominator . ' = '; if ($num == 0) { echo $numerator . '/' . $denominator; } else if ($newNumerator > 0) { echo $num . ' ' . $newNumerator . '/' . $denominator; } else { echo $num; } ?> Adjust aesthetics as you see fit.
-
You learn PHP by using PHP. It's that simple, really. Find a small-scale project you think would be interesting, and try to figure out how you would do it using the tools you have available (PHP, SQL, HTML, CSS, etc.).
-
Here are a lot of exercises for you: http://www.phpfreaks.com/forums/index.php/board,1.0.html A lot of problems just waiting to be solved. You'll help them by solving their problems, and you'll benefit because you'll get insight in some real world problems.
-
Why not just use on of the existing products that already exist and work fine? If you are doing any serious work then having to pay something like $25-50/mo shouldn't be a major issue. That's like a couple of hours' work. You have to take into consideration the time you will need to build and maintain your own project management solution as well. If you charge $25/hr, which isn't even in the high end, and have to pay $50/mo then it's the same as maintaining your system 2h/mo. That doesn't even account for the initial overhead of having to use a lot of hours of building it in the first place. These are hours you don't get paid for, and hours you could have spent on working for a client. Sometimes the benefits of rolling your own doesn't outweigh the cost of having to purchase it from somebody else. Of course if there are none of the existing solutions you like, then you'll have to, but otherwise I think it would be overkill.
-
A sub query returns a result, like all queries do, and you can use that result as a variable in your query. That's it, essentially.
-
Imagine a woman with hemophilia. Infinite menstruation...
-
Why was this really old thread revived anyway?
-
Look up the word "scalability". It's mentioned a few times in this topic already. Yours will also be slower. You're running four queries instead of one.
-
Well, yeah you need some way to numerically represent the characters. You cannot say 't'+'c'. That doesn't make sense unless you've defined how these should numerically be represented. Anyway, no, this is not a secure cipher, but OP said this is "for class". It would be an excellent introductory cipher in a cryptology class. You could probably then move on to to Vigenère cipher, which is a bit more advanced, but still relatively simple to understand. Of course eventually you would learn about ciphers that are applicable today such as RSA.
-
That's probably one of the most common arguments on forums. Well, perhaps you could even say that ad hominem is the most popular fallacy. "What you're saying is invalid because you're an authority". That's logical nonsense. Being an authority doesn't change the truth value of a statement. It's two completely irrelevant things, or a non sequitur if you wish. Try again.
-
They would most certainly support it, but they might have called it something else. You'll have to consult your control panels' help pages to figure it out.
-
For site2.com, add CNAME records for site.site.com.
-
I would advice you to read this chapter: http://php.net/manual/en/langref.php It explains type conversion and the other syntactical aspects of PHP. Do feel free to ask if there is anything you do not understand
-
A shifting algorithm. Let the key k ∈ Z, let P be a set containing alphabetic characters (a-z) forming the plain text value, let S be a set for the cipher text. |P|=|S|. Case-insensitively, the letter 'a'=0, 'b'=1, 'c'=2, etc. The function f maps a letter to its numerical value, the function g does the opposite. ∀ pi∈P, si∈S : si = g((f(pi) + k) % 25) Good enough? Coincidentally, the last line should explain exactly how to implement it. Before anyone asks, the algorithm is named like that because Caesar supposedly used that algorithm to encrypt messages to his generals. Whether that's true or not we'll let historians figure out. ROT13 is the above algorithm with k=13.
-
Math in PHP works like the math you would normally use: -(-x) = x I suppose that it's a popular saying that you "move" it, but that's not really what's going on. You can perform the same operation on each side of the equal sign (except division and multiplication by zero). This means that if you have a=b, then a+x=b+x. 1=1 <=> 1+2=1+2 <=> 3=3. You just need both of the sides to evaluate to the same. So if you have 2x-3y+6=0, then you know that subtraction is the opposite arithmetic operation of addition, thus they negate each other. So because of the above rules, you are allowed to say 2x-3y+6-6=0-6 <=> 2x-3y-0=-6 <=> 2x-3y=-6. You knew that you had to do -6 to get the +6, because that would make it +/-0, which is redundant so you can just remove it. It helps to know that. echo is a language construct, and not a function. Either way, it takes a string as argument. PHP is weakly typed, so scalar non-string values will implicitly be converted to strings for usage in a string context. It's only converted for that purpose though and isn't stored anywhere.
-
That website is the most ridiculous site ever. Oh really? Then why is it they're having trouble guarding the Afghan-Pakistani border, which is considerably smaller than the alleged "Ice Wall", to prevent al-Qaeda from crossing? "If it is in fact"...? That's something YOU are saying. Easily countered by saying "If the Earth is in fact round, then you must be lying when you say it isn't." I can't take shit like that serious. The Earth is cylindrical, and we're living on the top. Things don't fall off because the edge is surrounded by a gigantic ice wall, which isn't affected by global warming because it's actually just a mountain range covered by snow and ice. Nobody has ever seen this wall because world wide governmental conspirators are, for unknown reasons, guarding it using military force. GTFO.
-
If you want to know how something works then I would recommend howstuffworks.com, so for this particular question: http://electronics.howstuffworks.com/gadgets/clocks-watches/digital-clock.htm
-
http://www.newsweek.com/id/184156/output/print
-
convert path to a unique number that is unique to that path?
Daniel0 replied to shedokan's topic in PHP Coding Help
Well, it's not a string though, it's a hexadecimal number which could easily be converted to base 10. -
Try this: http://sshwindows.sourceforge.net/
-
convert path to a unique number that is unique to that path?
Daniel0 replied to shedokan's topic in PHP Coding Help
You could use MD5. Won't have a length of 12, but it'll be numeric.