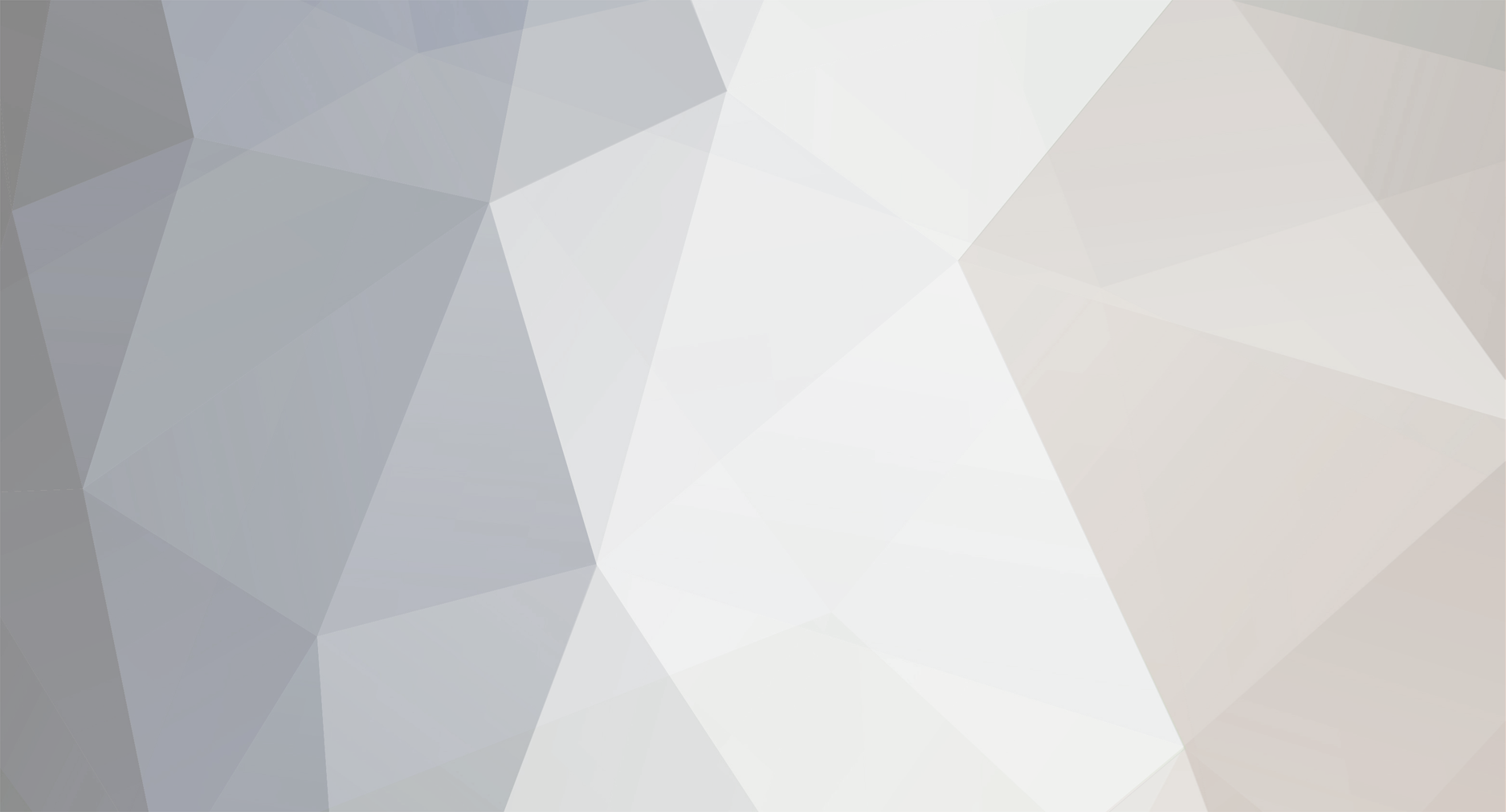
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
Why don't you ask and then we'll see if we can answer?
-
You're correct in saying that you wont have to bother with manually securing the data. An added benefit of using prepared statements instead is that prepared statements is a sort of template which has been parsed. This means that it'll decrease the execution time should you need to run the query multiple times as it only has to be parsed once, but executed multiple times with different arguments whereas using non-prepared statements the query will have to be parsed multiple times. Note that you can in fact use PDO::query() to run queries in the way mysql_query() does. If you're exclusively using prepared statements then you are also completely eliminating the aspects of SQL injection as it will all be taken care of automatically. If I remember correctly, then all vendor specific DBMS extensions will also be disabled by default in PHP 6 and PDO will be enabled by default (it is enabled by default in PHP 5.1+ as well). PDO being object oriented also makes it easier to extend and easier to encapsulate your code, which is in my opinion a great thing. I hope that answered your questions...
-
I haven't used that library, but seeing as it's written in PHP and PDO is written in C, PDO ought to be faster than the PEAR library you are referring to. I wouldn't use it if I were you unless it provides something in addition to PDO that would take long time for you to recreate.
-
Sure, PDO is "PHP Data Objects". It provides a sort of consistency across the different DBMSs (how do you pluralize that?). This has the advantage of being easily able to change the DBMS should you like to do that. When you create a prepared statement you'll create sort of like a template for a query and using either markers with names or just question marks as placeholders/variables. You'll then execute a prepared statement. By executing you are also passing the remaining data, i.e. the variables/placeholders, and thereby creating a complete query which will be run. When this is being executed you'll PDO will take care of adding quotes around the value if necessary and it will escape it and make it safe. On the statement object you can then call methods like fetch(), fetchAll(), etc. Here is a short example: <?php // connect $pdo = new PDO('mysql:host=localhost;dbname=test', 'username', 'password'); // create a prepare statement $statement = $pdo->prepare('SELECT * FROM users WHERE username = ? LIMIT 1'); // execute it and send the username to it. then fetch the row to an array $user_info = $statement->execute($_GET['username'])->fetch(); print_r($user_info); ?>
-
The MySQL extension has it's own function to deal with that: mysql_real_escape_string() Instead you should consider using PDO instead and prepared statements though: http://php.net/pdo
-
Yeah, I suppose that's a problem. One will either have to sacrifice performance or sacrifice complete data encapsulation. I find performance more important so I'd go with the latter approach.
-
This diagram describes the model's tasks pretty well and in general the MVC pattern pretty well.
-
IMO your second approach is fine. Regarding your first question about whether to use the orders or users model to get the latest orders... I'd say it depends on if you're trying to get a user's latest orders (then it would be the users model) or you'd get the latest orders overall (then it would be the orders model).
-
As Tom said, if you have a lot of traffic then it can increase the speed. Some large sites do that, e.g. SitePoint has sitepoint.com and then sitepointstatic.com for their static content (images, js, css).
-
No. The static server would only serve the static content, the db server would only have the db, etc... they would not take of each other's duties. You could make a solution where you would do that, but that is not what brown2005 is talking about as far as I can see.
-
An instance of the model doesn't necessarily have to represent one row. You can also use it as an API so you perhaps have UserModel::getLatestUsers() which would get the latest x users in an array. That's what I do and that's what I plan to do for the models I'll create when working on the main site here. The idea of a model is just to separate the data as much as possible from the business logic, nothing else.
-
As far as I'm concerned there is no legal proof that the owners of darkmindz are behind the attacks.
-
They're a bunch of losers and amateur programmers. Just take a look at their register script and their "CAPTCHA": <form name="registerfrm" action="" METHOD="post" onSubmit="return ValidateForm()"> Username: <br><input type="text" class="textbox" id="user_name" name="user_name"><br /> <br /> Password: <br><input type="password" class="textbox" name="password1"><br /> Confirm: <br><input type="password" class="textbox" name="password2"><br /> <p><small><font color="red">For your security, test your password strength <a href="pass_check.php">Here</a></font></small></p> Email: <br><input type="text" class="textbox" name="email"><br /><br /> <!-- Your Verification Code: 2bd5c --> <!-- Your Verification Code: TW96a --> <!-- Your Verification Code: GJ96n --> Your Verification Code: cflyk <br /> Please Enter The Verification Code: <br><input type="text" class="textbox" name="code"> <br /><br /> <input type="submit" name="cmdRegister" class="button" value="Register Now!"> </form> Morons... PS: What's the link to the post you created? I'd like to see it.
-
Lame script-kiddies having fun...
-
How to call a pre-existing object from within a class
Daniel0 replied to Naez's topic in PHP Coding Help
Furthermore, third party code might interfere with the global variables... -
[u][b]To people looking for the topic solved link:[/b][/u] That modification is currently not installed so the "Topic Solved" link will [i]not[/i] appear.
-
How to call a pre-existing object from within a class
Daniel0 replied to Naez's topic in PHP Coding Help
That's a bad idea. You'll eventually run into problems are you are coupling your things too tight and making all the things dependent on each other. -
If I buy something then I'll expect it to work properly, not be a piece of shit and be secure. I don't want to pay extra for those things. Who would want to purchase an insecure website?
-
Wouldn't it be easier to buy a small VPS and host it there? You can get those really cheap.
-
How to call a pre-existing object from within a class
Daniel0 replied to Naez's topic in PHP Coding Help
Then what if he would like another connection? You should only use a singleton on things that should only be instantiated one time. -
Something which could help you would be becoming a Zend Certified Engineer or perhaps studying computer science or a related field at a university. As Neal said, a PhD is in many countries the highest degree you can obtain, but that is certainly not necessary for "just" being a PHP developer. There are many great self-taught PHP developers that does not have any sort of formal education, but having some sort of degree related to your field of expertise might, however, help you get a job as you will have proof that you know something.
-
None... you just need to know PHP. But that differs from company to company of course.
-
How to call a pre-existing object from within a class
Daniel0 replied to Naez's topic in PHP Coding Help
If you're going to use the $db object a lot inside a class then it would perhaps be a good idea to store a reference in a property like you said. You could then pass it to the constructor and set it there. If you're just going to use it in one specific method then just pass it to that method. -
How to call a pre-existing object from within a class
Daniel0 replied to Naez's topic in PHP Coding Help
In PHP there are three scopes: global scope, class/object scope and function scope. The variable $db you define is in the global scope so you can only access it there, you can cheat and use the global keyword inside a function to bring it into the function scope, but you should not do that. Normally you cannot access things from a different scope that you are in (except for the class scope depending on the property's visibility (public, protected or private)). There are a few so-called superglobals which can be accessed from anywhere, an example could be $_GET and $_POST. To access things from the global scope within the function scope (and vice-versa) you'll have to find a way to bring the variable in there, that could be passing it as an argument. Also see: http://en.wikipedia.org/wiki/Scope_(programming) and http://php.net/variables.scope function getsomething($db) { /* ... */ } getsomething($db); -
See this: http://www.sitepoint.com/article/hierarchical-data-database