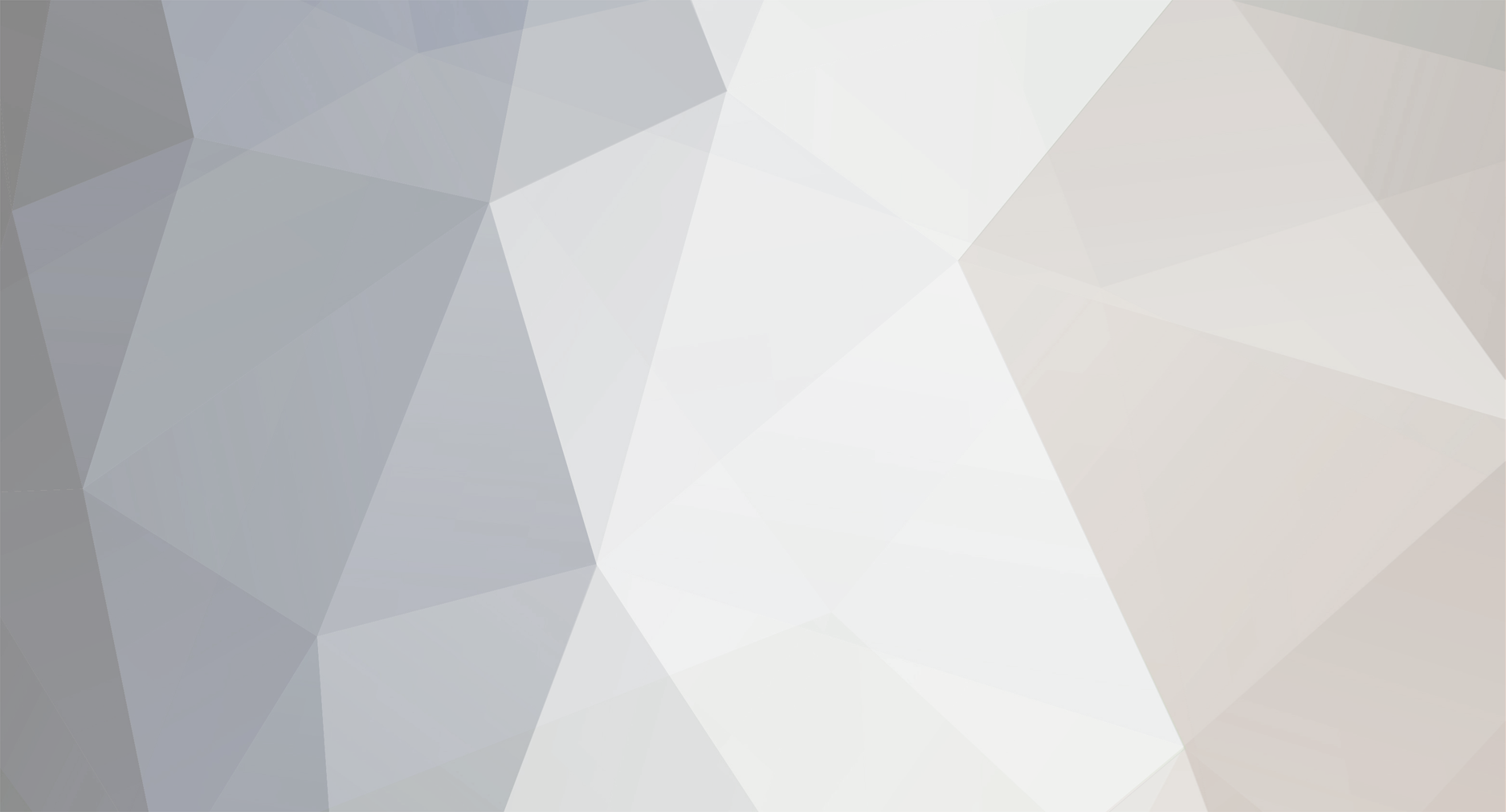
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
[quote author=Crayon Violent link=topic=107129.msg429390#msg429390 date=1157564770] And don't be afraid to use comments. [/quote] Don't comment the obvious, however. E.g.: [code]echo $username; // echo'es the username[/code] or [code]if(is_array($var)) // check if $var is an array[/code]
-
[code]mysql_query("query 1; query2; query 3;");[/code] If you don't understand what I mean, then I mean that you just have to seperate the queries with semi-colons ( [tt];[/tt] ).
-
I agree with wildteen. Additionally I prefer to write [tt]else if[/tt] instead of [tt]elseif[/tt] like some people do. And instead of [code]echo "Your name is ".$name."!";[/code] I prefer to use [code]echo "Your name is {$name}!";[/code]
-
You could use [url=http://www.zend.com/products/zend_guard]Zend Guard[/url] or [url=http://www.ioncube.com/sa_encoder.php]ionCube PHP Encoder[/url].
-
[code]<?php $dir = "/var/www"; $dh = @opendir($dir); $content = array(); // to prevent an E_NOTICE while($item = @readdir($dh)) { if($item != '.' && $item != '..') { $content[] = $item; } } if(count($content) < 1) { echo "Directory is empty or do not exist"; } else { foreach($content as $item) { if(is_dir("{$dir}/{$item}")) { echo "[DIR] "; } echo "{$item}<br />\n"; } } ?>[/code] Note that I put it in an array in the while loop so you could manipulate the array. Then I ran them through a loop again later to show you how to output it. You could of course just echo them directly from the while loop.
-
[b]Installing Apache2/PHP5/MySQL5 on Debian/Ubuntu[/b] Note: Since Ubuntu is based on Debian I suppose that this will work on Debian too, but I haven't tested it. This has been tested on Ubuntu 6.06. You will have to do this as root or run the commands using [tt]sudo[/tt]. [b]Installing the software[/b] Run this command to install Apache2 PHP5 and MySQL5: [code]aptitude install apache2 php5-mysql libapache2-mod-php5 mysql-server[/code] [b]Set root password for MySQL[/b] Run the following command on the terminal to get into MySQL's console: [code]mysql -u root[/code] When in the console run this query: [code]SET PASSWORD FOR 'root'@'localhost' = PASSWORD('your_root_password');[/code] Type [code]exit[/code] to exit MySQL's console. [b]Starting Apache[/b] Run this command as root to start Apache: [code]apache2ctl start[/code] Replace [tt]start[/tt] with [tt]stop[/tt] or [tt]restart[/tt] to stop or restart Apache, respectively. [b]phpMyAdmin[/b] Additionally you may run this command as root to install phpMyAdmin: [code]aptitude install phpmyadmin[/code] [b]Files/Directories:[/b] Apache configuration file: /etc/apache2/apache2.conf PHP configuration file: /etc/php5/apache2/php.ini MySQL configuration file: /etc/mysql/my.cnf Webpage root directory (what to call this?): /var/www
-
[code]<?php $items = scandir("/path/to/whatever"); if(count($items) < 2) { echo "No files found"; } else { foreach($items as $item) { if($item != '.' && $item != '..') { echo "{$item}<br />\n"; } } } ?>[/code]
-
[quote author=effigy link=topic=106885.msg428651#msg428651 date=1157483067] You can try the negative margin trick: [code] div { position: absolute; top: 50%; left: 50%; height: 200px; width: 600px; margin: -100 -300; text-align: center; } [/code] [/quote] Awesome! It works in both IE and Firefox.
-
I don't know if it's easier or if Dreamweaver supports it, but there is also Subversion (SVN).
-
[code]<?php print_r(scandir("/etc")); ?>[/code]
-
Creating instance of xmlhttprequest object
Daniel0 replied to Ninjakreborn's topic in Javascript Help
Taken from an AJAX Freaks tutorial: [code]function createRequestObject() { var req; if(window.XMLHttpRequest){ // Firefox, Safari, Opera... req = new XMLHttpRequest(); } else if(window.ActiveXObject) { // Internet Explorer 5+ req = new ActiveXObject("Microsoft.XMLHTTP"); } else { // There is an error creating the object, // just as an old browser is being used. alert('Problem creating the XMLHttpRequest object'); } return req; } // Make the XMLHttpRequest object var http = createRequestObject(); [/code] -
[quote author=Wintergreen link=topic=106962.msg428481#msg428481 date=1157468583] While we're on the subject of security type things, if I run my input through addslashes and mysql_real_escape_string before inserting into the DB, is it reasonably safe? [/quote] It's perfetcly safe if you escape it using that function first.
-
I'm not really good with JavaScript, but I think that you can use the focus() function. Something like: [code]blabla.document.focus();[/code]
-
http://www.cssplay.co.uk/ie/exampletwo.html It only works in IE however.
-
You are trying to make some sort of a warez search engine?
-
Could you provide us with a link and source codes?
-
Are you sure? Try sending a header like: [code]Content-type: text/html; charset=windows-1251[/code] (both on the page your script calls, and the page containing the script)
-
No, I don't feel special about it. I just mentioned it. About adding more work for them, then think about a bug tracker... this would be given a priority like low, but it still has to be fixed eventually.
-
http://validator.w3.org/check?uri=http%3A%2F%2Fphpfreaks.com
-
Actually you didn't.
-
Put [code]<?php session_start(); ?>[/code] at the top of image.php, then store the variable as a session variable ($_SESSION['picture1']) on the page containing the iframe and call the session variable in image.php. Remember to use session_start() in both pages (before outputting anything).
-
[code]<?php // ... send_message(189); sleep(10); send_message(16067); sleep(10); // ... ?>[/code] Edit: you obvisouly need to create the send_message() function.
-
[quote author=wildteen88 link=topic=106847.msg427790#msg427790 date=1157383516] Also when ever you use the sesison_start or setcookie function make sure you are not putting anything to the browser, otherwise you'll either get a blank screen or a nasty header cannot be sent error message. [/quote] That can be fixed using [url=http://www.php.net/manual/en/ref.outcontrol.php]output control[/url].
-
Strange From output sending an email ***SOLVED***
Daniel0 replied to Humpty's topic in PHP Coding Help
Change [code]$uFromWho = "From:$frmName\r\nReply-to: $frmEmail\r\nX-Mailer: PHP/" . phpversion();[/code] to [code]$uFromWho = "From: {$frmName} <{$frmEmail}>\r\nReply-to: {$frmName} <{$frmEmail}>\r\nX-Mailer: PHP/" . phpversion();[/code] Note that it's not the curly brackets that do it, I just think it looks nicer. -
[code]UPDATE products SET product_format='Book' WHERE product_code LIKE 'A%';[/code] mod edit: code A is books