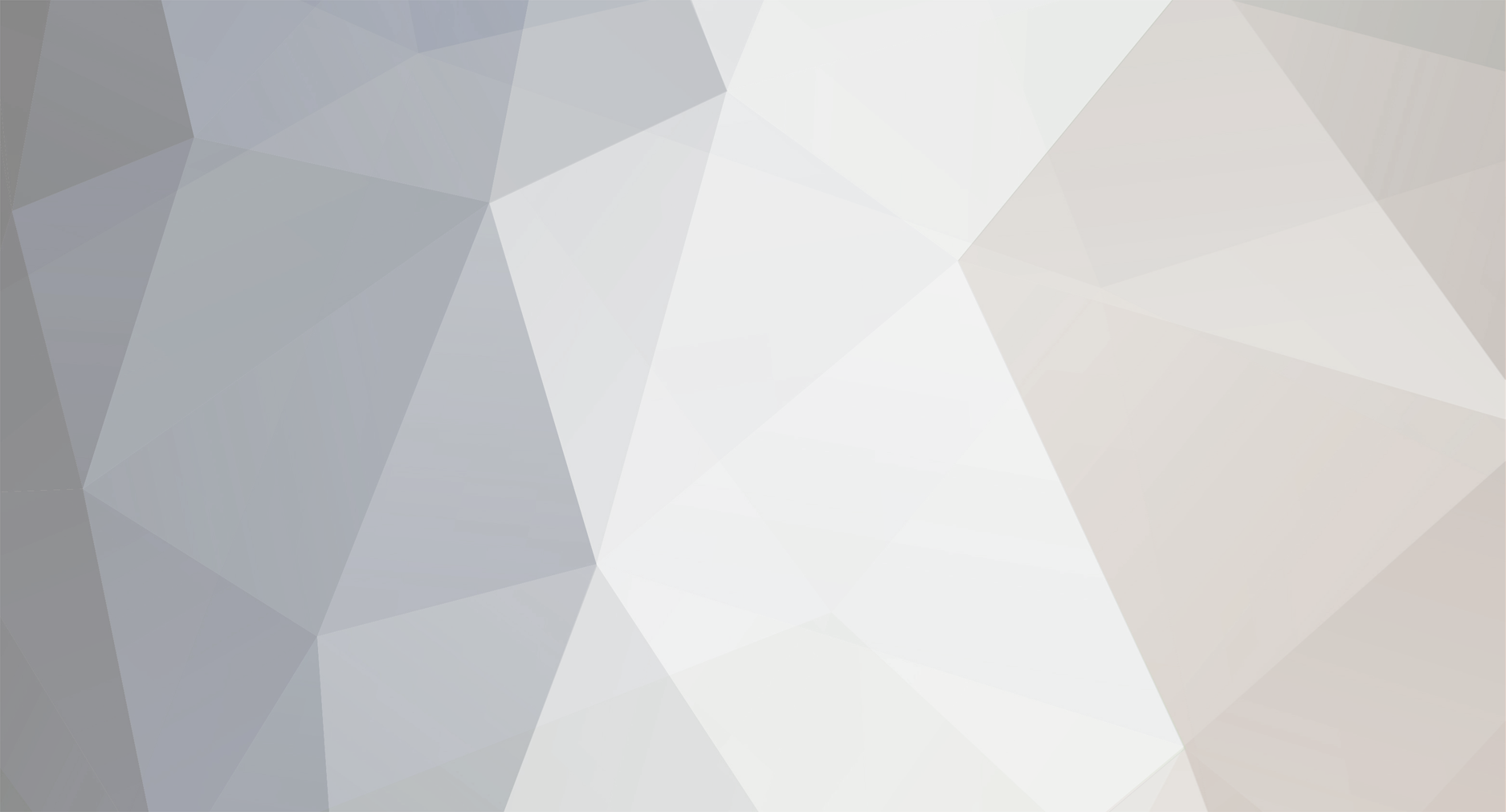
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
That is not OOP, sorry. Try to read the tutorials we have on the main site.
-
Well, imagine something simple like this: <?php session_start(); function checkBoundary($value, $add, $max, $min = 0) { $value += $add; if ($value < $min) { $value = $min; } else if ($value > $max) { $value = $max; } return $value; } $map = 'http://www.phpfreaks.com/media/images/forums/bg_header.png'; $width = 950; $height = 133; $stepValue = 10; // reset on invalid position or non-existant position if (empty($_SESSION['pos_x']) || empty($_SESSION['pos_y']) || $_SESSION['pos_x'] > $width || $_SESSION['pos_x'] < 0 || $_SESSION['pos_y'] > $height || $_SESSION['pos_y'] < 0) { $_SESSION['pos_x'] = round($width / 2); $_SESSION['pos_y'] = round($height / 2); } if (isset($_GET['op'])) { switch ($_GET['op']) { case 'up': $_SESSION['pos_y'] = checkBoundary($_SESSION['pos_y'], -$stepValue, $height); break; case 'down': $_SESSION['pos_y'] = checkBoundary($_SESSION['pos_y'], $stepValue, $height); break; case 'left': $_SESSION['pos_x'] = checkBoundary($_SESSION['pos_x'], -$stepValue, $width); break; case 'right': $_SESSION['pos_x'] = checkBoundary($_SESSION['pos_x'], $stepValue, $width); break; } } ?> <style type="text/css"> #mapCanvas { width: <?php echo $width ?>; height: <?php echo $height ?>; background: url(<?php echo $map ?>); position: relative; } #player { width: 5px; height: 5px; background: #F00; position: absolute; top: <?php echo $_SESSION['pos_y'] ?>; left: <?php echo $_SESSION['pos_x'] ?>; } </style> <div id="mapCanvas"> <div id="player"></div> </div> <ul id="controls"> <li><a href="?op=up">Move up</a></li> <li><a href="?op=down">Move down</a></li> <li><a href="?op=left">Move left</a></li> <li><a href="?op=right">Move right</a></li> </ul> <p>Current position: <?php printf('%d,%d', $_SESSION['pos_x'], $_SESSION['pos_y']) ?></p>
-
If you just want today +90 days you can just do strtotime('+90 days'). No need for a date to add it to.
-
http://lmgtfy.com/?q=whois+lookup+php&l=1
-
That's going to be difficult unless you can teach PHP to speak English.
-
No, drop the idea about tiles. Just store two real numbers: x and y. These represent a point (their position) in the plane (the map). You would obviously like to set an upper and lower bound on the x-axis and y-axis. When moving people around on the map you could allow them to use arrows and move them according to a predefined step value. You could also allow them to click on the map to move.
-
Sweet! I got +50 gigs on my already infinite plan! They mean "unmetered", their is a limit of usage but its not revealed, its just sale talk. Unlimited mean unlimited. You cannot put a limit on something that is unlimited. This has been discussed numerous times though.
-
Why do you want to frames? That's been outdated for several years.
-
It's the aliens. Finally, we've made contact!
-
Routing is just a collective term for mapping a request to some sort of resource in your business logic. In fact, it means what you call "bootstrapping" here. Specifically how you do it is not so important (to some extent). You will obviously want some sort of way that has some degree of modularity. E.g., having to alter a switch all the time would be cumbersome, and it would eventually result in a rather large switch; you would want something dynamically defined.
-
OOP is not just using "classes". OOP is about objects and how these naturally interact with each other. Each object has a state represented by it's internal properties, and they can be manipulated using the public methods they expose (also called the interface). You need to think about objects not classes. A class is merely a blueprint for an object, so to speak. You needn't use OOP if you don't want to. You can use procedural programming. You just need to know that OOP and procedural are not the same thing even though they're both part of the imperative paradigm.
-
OOP Question -> Object to contain a collection of objects
Daniel0 replied to enoch's topic in PHP Coding Help
Not if you're like me and your books are all over the place at least. -
OOP Question -> Object to contain a collection of objects
Daniel0 replied to enoch's topic in PHP Coding Help
That would be okay, with a few exceptions: 1) Your properties shouldn't be public. 2) What is up with $this->obj = $obj; all over the place? 3) A width is a numeric value. Why are you storing it as a string? Use a float or int depending on your needs. -
lkdjflksjdflkajsdflajsdfk ^^^ what does that say? Now, what do you think will happen if a browser received a load of binary junk?
-
I don't want to sound demeaning, but looking at your topics, maybe you should reconsider your hobby/career choice? Or maybe you should just stop for a while and take a look at what you're doing. You are failing at really elementary logic all the time. Before someone says I can't say that, I'm saying this as Daniel, not as PHP Freaks admin.
-
I don't see session_start() anywhere on that page...
-
Ah, sorry. I could have sworn I saw the word "checkbox" somewhere. Looks so. You could try it out and see if it works You'll need to use isset to make sure you aren't accessing an invalid index though.
-
First of all, remember that enumerated arrays are zero indexed! Secondly, $_POST is an array, as you know, so if you need to access an array inside $_POST you do like $_POST['some_array']['some_index']. As for the form, you can do like you did, but you can also explicitly specify the index like this: <input type="text" class="text" name="score[5]" id="score_5" /> You'll need to set a value for the checkboxes though. To make it checked when the page loads you will have to add checked="checked".
-
You can just do this: if(!empty($_POST['checkbox'])) { // checkbox is checked } It's impossible to have isset($_POST['checkbox']) == false and !empty($_POST['checkbox']) == true simultaneously.
-
Performance-wise it's irrelevant, but I would recommend using an array. It's much easier to work with, and it's a data structure specifically designed to hold groups of data.
-
Actually, don't bump at all unless you've shown some sort of progress or are able to provide additional information:
-
Actually, you can fully administrate an IIS server using the command line. The commands aren't exactly straightforward to remember though.