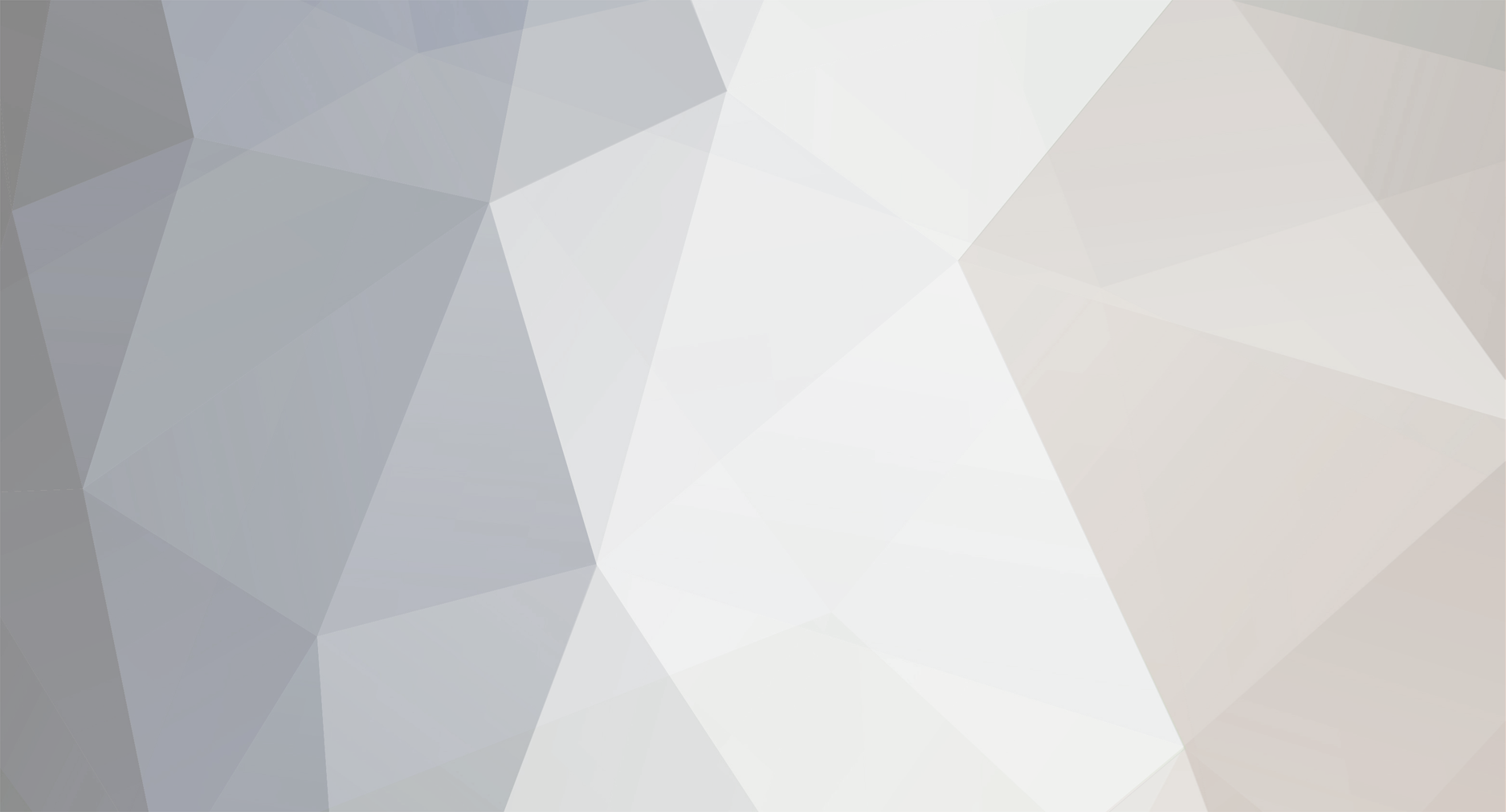
HeyRay2
Members-
Posts
223 -
Joined
-
Last visited
Never
Everything posted by HeyRay2
-
To have PHP do this, you would have to submit the form and refresh the page each time the button was clicked to increase your counter. You would also have to include the current value of [b]$static[/b] as a hidden element in your form: [code=php:0] <?php // Get the current value of $static if(isset($_POST['static'])){ $static = $_POST['static']; } else { $static = 0; } // Check if the form was submitted if($_POST['button']){ $static++; } ?> <html><body> <form method="POST" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <input type="hidden" name="static" value="<?php echo $static; ?>"> <input type="submit" name="button" value="CLICK"> </form> </body></html> [/code] As already mentioned, another option would be javascript. Create an "OnClick()" event that will increment the variable each time.
-
Glad to hear it started working again. If you're still interested in investigating why this happened, maybe answer the following: Have you recently changed anything in your forum code? Have you switch to a new web host? Have you upgraded to a new type / version of database? Have you upgraded to a new version of PHP? Also, a snippet of your "reply" code could still prove useful in this situation... ;)
-
You didn't answer the question I posed. Are you performing any modifications on the reply text before it's submitted to the database? If so, we need to know what those modifications are so we know what state the text is in. Only then will we know what needs to be done to that text to get rid of your "rn" problem. My code will only work if you have not yet stripped the slashes from your reply text. Perhaps posting a snippet of your "reply" code would be a step in the right direction.
-
The MySQL manual on JOINS is located here: [url=http://www.mysql.org/doc/refman/4.1/en/join.html]http://www.mysql.org/doc/refman/4.1/en/join.html[/url]
-
Placing a delimited list of groups in your user table invites data anomalies. For instance, if you deleted a group then the user could be tied to a non-existant group. To avoid this, you would have to: [list] [*]Parse through each user [*]Extract the list of group IDs for that user [*]Break the list of group IDs into an array [*]Remove the group ID from the array [*]Group the remaining IDs back into a delimited list [*]Submit the change the back to the database [/list] Normalizing your database, and breaking this information into a new table would be more consistent and easier to manage changes. In this instance, you would need 3 database tables, with structures similar to the following: [b]users_table[/b] user_id user_name ...etc... [b]groups_table[/b] group_id group_name ...etc... [b]group_relations[/b] relation_id user_rel_id group_rel_id The [b]users_table[/b] will be the table you already have. The [b]groups_table[/b] will be the unique IDs and names of all groups in the database. The [b]group_relations[/b] will be a list of User IDs and the Group IDs that are associated. This will allow you associate an unlimited amount of groups to one user, and unlimited users to a group. Additionally, when you remove a group or a user, you'll only need to run one small query to remove all entries in [b]group_relations[/b] that pertain to that given user or group. The one big hurdle in this method is working with JOINS, which can take a little bit of effort to learn to use correctly. However, once you learn this skill, you'll find that your MySQL queries are MUCH more powerful.
-
If you set your variables in a session, you don't need to pass them in a URL as well. Instead of trying to get your variable from $_POST, then assigning them to $_SESSION, followed by moving them to $_GET, just change your search form to use the $_GET method and keep it that way, like so: [b]form.php[/b] [code=php:0] <form action="http://localhost/queries/query.php" method="GET" name="form"> Searching for players which play these games... <br /> <br /> <input name="football" type="checkbox" value="x">Football <br /> <input name="basketball" type="checkbox" value="x">Basketball <br /> <br /> <input name="reset" type="reset" value="Reset"/> <input name="submit" type="submit" value="Submit"/> </form> [/code] [b]query.php[/b] [code=php:0] <?php $page=$_GET["page"]; if(!isset($page)) { $page=1; $pageNo = $page +1; } $pageNo = $page; echo "Page Number: <b>$pageNo</b>"; $page=$page-1; //connecting to my database.. and for sure it's working..(hidden for now) //.... //.... //.... $football = $_GET['football']; $basketball = $_GET['basketball']; // YOUR QUERIES AND RESULT PRINTING ... ... // YOUR URL TO THE NEXT PAGE OF RESULTS echo "<a href='query.php?page=$pageNo&football=$football&basketball=$basketball'>$font $num $unfont</a> "; // YOUR MySQL SESSION CLOSING, ETC... ... ... ?> [/code]
-
The query I wrote already compares the database to the search text ([code=php:0]$_POST['search'][/code]), and it only returns the matches. There's no need to do any other comparison, all you have to do from that point is print out the results.
-
Regarding your query: [code=php:0] $result = mysql_query("SELECT*FROM search WHERE text"); [/code] What data is contained in the "search" table? You specify "WHERE text", but you don't tell MySQL what to check "text" against. This would only work if you did something like: [code=php:0] $result = mysql_query("SELECT * FROM search WHERE text = '$somevar'"); [/code] What you'll need to do is something like this. Start by mapping out what tables in the database you want to search. For example, let's say your website has news articles, with the following database structure: [b]news_table[/b] news_id news_title news_text You would want to structure your query simiarl to this: [code=php:0] $sql = "SELECT * FROM news_table WHERE news_title LIKE '%".$_POST['search']."%' OR news_text LIKE '%".$_POST['search']."%'"; $result = mysql_query($sql); [/code] You would need to perform a "LIKE" statement for each field in the database you want to check.
-
Are you performing a [b]stripslashes()[/b] on the reply text before it's added to the database? If so, that would change "\r\n" to "rn". Run a [b]str_replace()[/b] or a [b]preg_replace()[/b] instead of stripping slashes, change "\r\n" to "\n", then change "\n" to HTML line breaks, and you should be good to go: [code=php:0] $text = nl2br(preg_replace("/\r\n/", "\n", $_POST['text'])); [/code]
-
What are you printing to the browser in [b]page1.php[/b]? If you're printing anything out to the browser on [b]page1.php[/b], you won't be able to use the [b]Header()[/b] method, as not other output can be made to the browser. This method works well for pages that only process data, not display it. If you want to print something out to the first page, and then carry the variables to another page, you can use a similar method with a hyperlink: [b]page1.php[/b] [code=php:0] <?php // Define variables $var1 = "foo"; $var2 = "bar"; // Link to a new location, with the vars carried over echo "<a href=\"page2.php?var1=".$var1."&var2=".$var2."\">Page2</a>"; ?> [/code] [b]page2.php[/b] [code=php:0] <?php // Grab the variables the from the $_GET superglobal array $var1 = $_GET['var1']; $var2 = $_GET['var2']; // Echo the variables echo "Var 1: ".$var1."<br />"; echo "Var 2: ".$var2."<br />"; ?> [/code]
-
;) Sounds like you're slowly working it out. Good luck... :)
-
[b]page1.php[/b] [code=php:0] <?php // Define variables $var1 = "foo"; $var2 = "bar"; // Go to a new location, with the vars carried over Header("Location: page2.php?var1=".$var1."&var2=".$var2.""); ?> [/code] [b]page2.php[/b] [code=php:0] <?php // Grab the variables the from the $_GET superglobal array $var1 = $_GET['var1']; $var2 = $_GET['var2']; // Echo the variables echo "Var 1: ".$var1."<br />"; echo "Var 2: ".$var2."<br />"; ?> [/code]
-
Impossible to stop completely, because once the user has the file, it's future travels are up to that user. However, using some of the methods mentioned in this thread, you can at least ensure that when people are getting the file from you, you are getting your due compensation. Good luck... :)
-
Help - Wondering how to input a variable, analyze it, then output
HeyRay2 replied to smc's topic in PHP Coding Help
Depending on how many levels you allow, that could be one [b]HUGE[/b] switch statement. I still say an algorithm would be the way to go. The source code for [url=http://phprpg.org/]phpRPG[/url] is available as well. You could get some ideas from the way they calculate experience, skills etc... ;) -
Help - Wondering how to input a variable, analyze it, then output
HeyRay2 replied to smc's topic in PHP Coding Help
If you don't want to hard code what XP values each level has, then you'll need to create an algorithm that will have an exponential increase for each level. This could work experience, skills, etc... I came across a web page where someone dissected the XP mechanics for FF7, which may help you get started. Check it out [url=http://astro.berkeley.edu/~dperley/ff7/expmechanics.html]HERE[/url] -
Very simple search script not working. I'm Baffled. **[SOLVED]**
HeyRay2 replied to Lyricsride's topic in PHP Coding Help
:D, haha you beat me to the punch! ;) -
Short answer: It's not possible. Checking the referrer may work, but you can't be 100% sure why or how they came from a certain site to yours. In the case of my suggestion, to ensure a user can fully download a file, you could: [list] [*]Give the user the ability to send a form or click a link to "request" another chance to download the file. You could then reenable their download link manually or with a "link request" script [*]Give the user a certain time period in which they can download the file, giving them plenty of chances to download it correctly, and disable the link after that time period. This, however, gives the user a certain window of time to share out the link. [/list]
-
Sounds like you already have the ability to do what you want to do. I don't understand how developing a new method for protecting certain parts of site is would be less expensive to a client than something you already have the skill to do "blind folded", but I digress... Perhaps you could have a database table that will track the PayPal transaction ID or email address, the associated file, and whether or not the user has downloaded the file. Once they've downloaded the file it will mark the database entry and "disable" the download link for further use. ;)
-
[url=http://www.phpfreaks.com/tutorials/28/0.php]http://www.phpfreaks.com/tutorials/28/0.php[/url] PHPFreaks.com, is there anything you can't do??? ;)
-
The best method would be to create a membership system that will keep track of what user has access to what files. There are some good "membership system" tutorials on this site [url=http://www.phpfreaks.com/tutorial_cat/7/Membership-Systems.php]HERE[/url]
-
The most you'll be able to accomplish would probably be: [list] [*]Import your Access database to another Linux-compatible database system, like MySQL or similar [*]Access your Access database from a Windows machine using your Linux machine (Info[url=http://www.phpbuilder.com/columns/timuckun20001207.php3?page=1]HERE[/url]) [*]Run PHP on a Windows machine with Access installed (Info[url=http://www.phpbuilder.com/columns/timuckun20001207.php3?page=1]HERE[/url]). [/list] Unless you have some specific reason for making Access work with a Linux webserver, I would say dump Access and use MySQL or something similar.
-
[quote]I can copy and paste this same code, and jsut check different fields in a different table for it to do the same thing[/quote] Then copy and paste the code, make the changes and try it out. Making multiple database calls will not hurt your database, and as long as you have a decent server, it should be able to handle the load with multiple users. [quote]I was given advice on the script earlier, tbu I don't want to take the chance of it breaking the script it took me awhile to get this covering all angles.[/quote] If you're not willing to try things out for fear of breaking your script, then I would suggest making a "test" copy of your database and script and trying different things out there. Otherwise, no amount of suggestions from anyone here will help you if you're not willing to give them a try... ;)
-
Does hardcoding the column name work? [code=php:0] $sql = "SELECT first, last, email FROM student_involvement WHERE acapella = 1"; [/code] Let us know if the query is successful.
-
What is the best way to link an image with a record in mysql
HeyRay2 replied to AdRock's topic in PHP Coding Help
Uploaded files are contained in the $_FILES superglobal array. If your upload form has this field: [code]<input type="file" name="thumbnail">[/code] Then access to the elements of that file upload would be contained in: [code=php:0]$_FILES['thumbnail'][/code] You are correct that you are going to "INSERT" the content of the form into a database. Each element in your form that you want it's value preserves will need a field in the database. You are also correct that the first "INSERT" statement will not include the image reference, because in this case we are relying on the ID number of the database entry for the file name, so we have to wait until that is assigned, then we can "UPDATE" (which I made a mistake on in my code, I apologize) the last entry with this file name, like so: [code=php:0] // Update the DB entry $sql2 = "UPDATE news SET image = '$image_filename' WHERE news_id = $last_news_id"; mysql_query($sql2) or die("Error updating image name: ".mysql_error()); [/code] This handles the database reference to the file. However you still need to save the file in a place that your webserver has access to, and can be read from when you print out your database entries, which is where the following line comes in: [code=php:0] // Save the image move_uploaded_file($_FILES['thumbnail']['tmp_name'], $image_fullpath); [/code] This take the uploaded file from it's temporary location and saves it on your webserver in a folder you specify. You can then reference that file by pointing to that path and filename when you print our your entries.