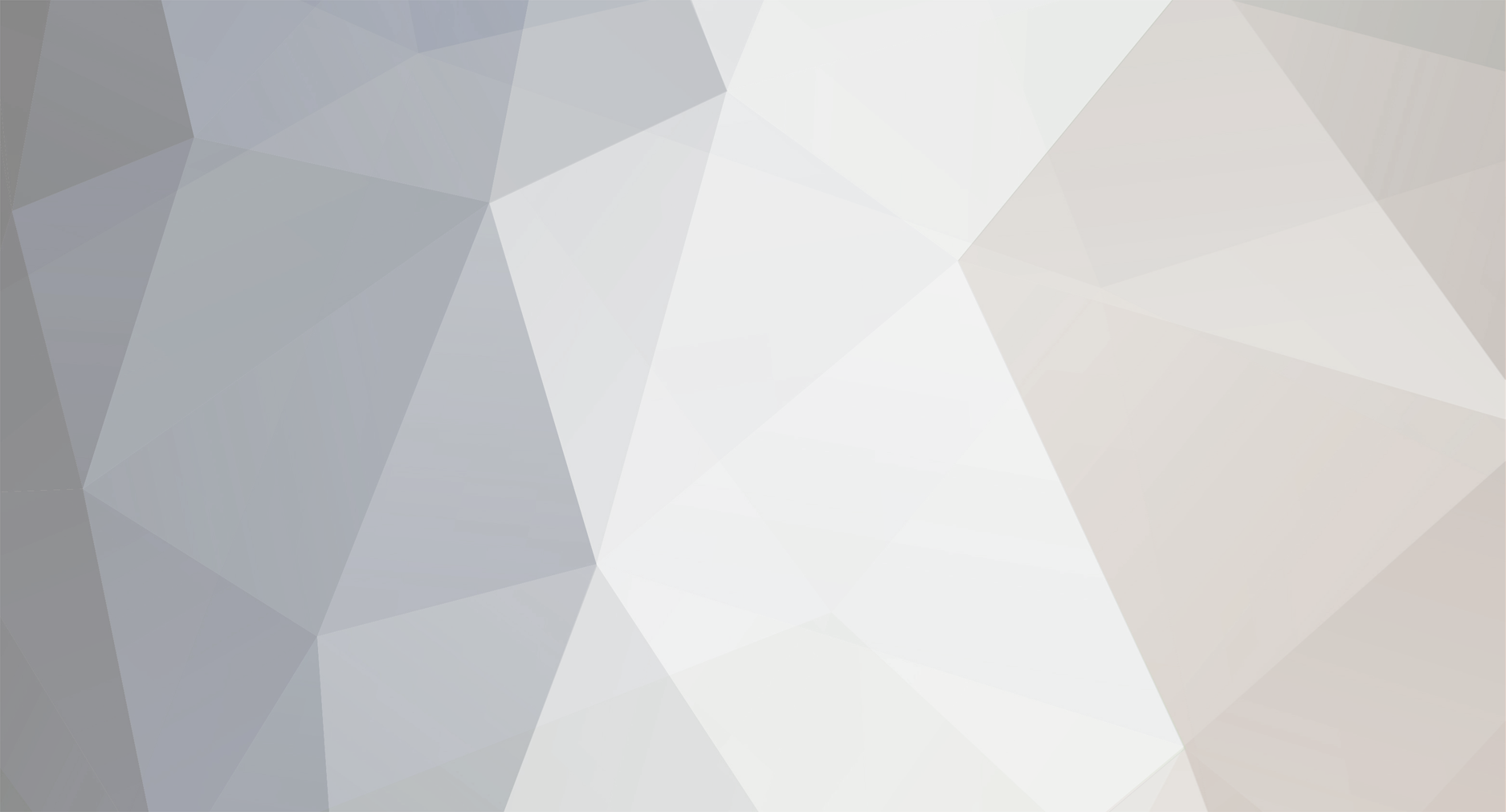
genericnumber1
Members-
Posts
1,858 -
Joined
-
Last visited
Never
Everything posted by genericnumber1
-
I'd probably use stream_get_line to get each word individually. Or, if the file is small enough that it can fit in memory all at once, you could use the more simple solution of file_get_contents along with explode.
-
<?php define('SECONDS_PER_WEEK', 604800); $startDate = mktime(0, 0, 0, 6, 30, 2009); $endDate = $startDate + (SECONDS_PER_WEEK * 10); // 10 weeks past $startDate for($date = $startDate; $date <= $endDate; $date += SECONDS_PER_WEEK) { printf('<option value="%s">%s</option>', date('n/j/Y', $date), date('F jS, Y', $date)); } ?> Doubt you needed this many answers, but I wrote it and dammit if I'm going to let it go to waste.
-
Actually template engines are quite powerful and useful for separation of logic and presentation (as rhod said) in large projects. I tend to stick with the one baked into zend framework, but pretty much any template engines make a managing a large website much easier.
-
http://www.smarty.net/ I'm not a big fan, but to each their own (I prefer a different template engine). Smarty's powerful once you learn the syntax.
-
Try profiling it and find out! Either way, I would guess the first would be quicker. But as they say, premature optimization is the root of all evil! Choose whatever is the easiest to read, I have a huge feeling that there will be bigger bottlenecks in your code than either of these.
-
Daniels, I think he knows that. doebaladoo, as of php5 all objects are passed by reference (they're instantiated on the heap if that means anything to you), so even specifying that the method returns a reference is not needed. Try removing the & from the function prototype and you should still get the same result.
-
How about something like this? <?php define('LINK_URL', 0); define('IMAGE_URL', 1); $images = array( array(LINK_URL => 'http://www.example1.com/', IMAGE_URL => 'images/someimage1.jpg'), array(LINK_URL => 'http://www.example2.com/', IMAGE_URL => 'images/someimage2.jpg'), array(LINK_URL => 'http://www.example3.com/', IMAGE_URL => 'images/someimage3.jpg'), ); shuffle($images); foreach($images as $image) { echo '<a href="' . $image[LINK_URL] . '"><img src="' . $image[iMAGE_URL] . '" /></a>'; } ?> If the image and link locations vary only in numbers though (eg, they count up from 1 to 20) it would be easier to just use range to define the array before you shuffle it. That way you don't have to deal with hard-coding each individual image link and its url, only the minimum image number and maximum image number. edit: clarity.
-
Creating a Grade Calculator using PHP and HTML
genericnumber1 replied to Jintu's topic in PHP Coding Help
Well... 1) First ask yourself why you're adding the max amount to the grade if the grades are out of the range 0-100. (I'm not sure why you are...) 2) You might then ask how you would signify whether or not a grade is to be counted on the form's input. (Has it occurred yet? Do you mark the grade as -1 to signify it isn't to be counted? A checkbox that says "don't count this" perhaps?) 3) You can then consider how you would keep a count of the total number of possible points excluding those grades that aren't to be counted in the average (see 2). At that point you can get the grade by doing grade*(100/ValueFromPoint3Above). -
Creating a Grade Calculator using PHP and HTML
genericnumber1 replied to Jintu's topic in PHP Coding Help
No, no, people will give you code help here, but I'm often more of the philosophy of not writing code for people if I think they're capable of writing it themselves. What you have written as far as the current existing code goes is all you need to implement the feature you described. if/then/else, form handling, and the basic math operators are all that are needed, and since you wrote that code above I'm confident you can add the feature you described if you give it enough thought. -
Creating a Grade Calculator using PHP and HTML
genericnumber1 replied to Jintu's topic in PHP Coding Help
Then, since the maximum number of marks thus far in the semester is 55 (because 45 are, as of yet, unattainable) you simply multiply $grade by 100/55 to get the current grade excluding al2 and final. I'm explaining the approach, not the code to back it up. -
Creating a Grade Calculator using PHP and HTML
genericnumber1 replied to Jintu's topic in PHP Coding Help
You just need to multiply $grade by 100/maxPossibleMarks. Figure out how to calculate the maximum number of possible marks thus far in the semester and it will be trivial to implement. -
member function Execute() on a non-object
genericnumber1 replied to Sven70's topic in PHP Coding Help
You'll have to show us the code where you call the Subscriber_Type function. -
It's working for me, what is $message's value?
-
You probably want it to be <p class="message">Message:<br><?php echo wordwrap($message,30,'<br />'); ?></p> if you wanted the word wrapping to actually be visible in the rendered page. The default wrapping is \n, which is not rendered by the browser as a line break.
-
You're using the wrong types of quotes on the form's HTML for one...
-
Switch Decimal place using decimal numbers.
genericnumber1 replied to jason97673's topic in PHP Coding Help
Ah, you're right, no adding... But no matter, that can be fixed with more objects! <?php class Math { static public function increment(&$number) { $number = Math::addOne($number); return $number; } static public function addOne($number) { $bit = 1; while(($number & $bit) > 0) { $bit <<= 1; } while($bit >= 1) { $number ^= $bit; $bit >>= 1; } return $number; } } class UncommittedOrderException extends Exception {} class NonexistantOrderException extends Exception {} class IncompleteOrderException extends Exception {} class EmptyOrderListException extends Exception {} class UnsetNumberException extends Exception {} class UnsetShiftedCharacterException extends Exception {} class DirectionOrder { private $direction = null; private $distance = null; private $callingShifter; public function __construct(NumberShifter $callingShifter) { $this->callingShifter = $callingShifter; } public function setDirection($direction) { $this->direction = $direction; return $this; } public function setDistance($distance) { $this->distance = $distance; return $this; } public function commitOrder() { if($this->direction === null || $this->distance === null) { throw new IncompleteOrderException(); } $this->callingShifter->commitOrder(); return $this->callingShifter; } public function getDirection() { return $this->direction; } public function getDistance() { return $this->distance; } } class NumberShifter { const LEFT = 1; const RIGHT = 2; private $orders = array(); private $order = null; private $number = null; private $character = null; public function setNumber($number) { $this->number = $number; return $this; } public function getNumberAsFloat() { return (float) $this->number; } public function setShiftedCharacter($character) { $this->character = $character; return $this; } public function newDirectionOrder() { if($this->order !== null) throw new UncommittedOrderException(); $this->order = new DirectionOrder($this); return $this->order; } public function commitOrder() { if($this->order === null) throw new NonexistantOrderException(); $this->orders[] = $this->order; $this->order = null; } public function shift() { if($this->order !== null) throw new UncommittedOrderException(); if(count($this->orders) == 0) throw new EmptyOrderListException(); if($this->number === null) throw new UnsetNumberException(); if($this->character === null) throw new UnsetShiftedCharacterException(); $pieces = explode($this->character, $this->number); foreach($pieces as &$piece) { $piece = str_split($piece); } foreach($this->orders as $order) { $end = count($pieces) - 1; for($i = 0; $i < $order->getDistance(); Math::increment($i)) { for($j = 0; $j < $end; Math::increment($j)) { if($order->getDirection() == self::RIGHT) { if(count($pieces[Math::addOne($j)]) == 0) { $pieces[Math::addOne($j)][] = 0; } $pieces[$j][] = array_shift($pieces[Math::addOne($j)]); } else { if(count($pieces[$j]) == 0) { $pieces[$j][] = 0; } array_unshift($pieces[Math::addOne($j)], array_pop($pieces[$j])); } } } } foreach($pieces as &$piece) { $piece = implode('', $piece); } $this->number = implode('.', $pieces); } } $shifter = new NumberShifter(); $shifter->setNumber(0.6278) ->setShiftedCharacter('.') ->newDirectionOrder() ->setDirection(NumberShifter::RIGHT) ->setDistance(2) ->commitOrder() ->shift(); echo $shifter->getNumberAsFloat(); ?> fixed. -
Switch Decimal place using decimal numbers.
genericnumber1 replied to jason97673's topic in PHP Coding Help
Obviously the best solution is object oriented with method chaining for more readable code. <?php class UncommittedOrderException extends Exception {} class NonexistantOrderException extends Exception {} class IncompleteOrderException extends Exception {} class EmptyOrderListException extends Exception {} class UnsetNumberException extends Exception {} class UnsetShiftedCharacterException extends Exception {} class DirectionOrder { private $direction = null; private $distance = null; private $callingShifter; public function __construct(NumberShifter $callingShifter) { $this->callingShifter = $callingShifter; } public function setDirection($direction) { $this->direction = $direction; return $this; } public function setDistance($distance) { $this->distance = $distance; return $this; } public function commitOrder() { if($this->direction === null || $this->distance === null) { throw new IncompleteOrderException(); } $this->callingShifter->commitOrder(); return $this->callingShifter; } public function getDirection() { return $this->direction; } public function getDistance() { return $this->distance; } } class NumberShifter { const LEFT = 1; const RIGHT = 2; private $orders = array(); private $order = null; private $number = null; private $character = null; public function setNumber($number) { $this->number = $number; return $this; } public function getNumberAsFloat() { return (float) $this->number; } public function setShiftedCharacter($character) { $this->character = $character; return $this; } public function newDirectionOrder() { if($this->order !== null) throw new UncommittedOrderException(); $this->order = new DirectionOrder($this); return $this->order; } public function commitOrder() { if($this->order === null) throw new NonexistantOrderException(); $this->orders[] = $this->order; $this->order = null; } public function shift() { if($this->order !== null) throw new UncommittedOrderException(); if(count($this->orders) == 0) throw new EmptyOrderListException(); if($this->number === null) throw new UnsetNumberException(); if($this->character === null) throw new UnsetShiftedCharacterException(); $pieces = explode($this->character, $this->number); foreach($pieces as &$piece) { $piece = str_split($piece); } foreach($this->orders as $order) { $end = count($pieces) - 1; for($i = 0; $i < $order->getDistance(); ++$i) { for($j = 0; $j < $end; ++$j) { if($order->getDirection() == self::RIGHT) { if(count($pieces[$j+1]) == 0) { $pieces[$j+1][] = 0; } $pieces[$j][] = array_shift($pieces[$j+1]); } else { if(count($pieces[$j]) == 0) { $pieces[$j][] = 0; } array_unshift($pieces[$j+1], array_pop($pieces[$j])); } } } } foreach($pieces as &$piece) { $piece = implode('', $piece); } $this->number = implode($this->character, $pieces); } } $shifter = new NumberShifter(); $shifter->setNumber(0.6278) ->setShiftedCharacter('.') ->newDirectionOrder() ->setDirection(NumberShifter::RIGHT) ->setDistance(2) ->commitOrder() ->shift(); echo $shifter->getNumberAsFloat(); ?> -
ignore first character and display the rest
genericnumber1 replied to sungpeng's topic in PHP Coding Help
Look into using substr and trim along with your echo statement. -
I posted a solution to this a few weeks ago. <?php $number = 31031976; while($number > 9) { $number = array_sum(str_split($number)); } echo $number; From: http://www.phpfreaks.com/forums/index.php/topic,247632.msg1158771.html#msg1158771
-
Google "function scope", it should tell you a bit of information to help out.
-
[SOLVED] How to disable apostrophe in input field?
genericnumber1 replied to inferium's topic in PHP Coding Help
You could strip the apostrophes when they submit, error if they submit them, or use javascript to disallow submission if they have an apostrophe (you would still need a server-side check though). If you're just trying to secure a sql query or something similar to that though, just escaping it is the best way. -
You're missing a parenthesis if (!is_dir('../users/'.$userid) should be if (!is_dir('../users/'.$userid))
-
Info on the mysql time datatypes: http://dev.mysql.com/doc/refman/5.1/en/datetime.html.
-
Easiest way? <?php $number = 58; while($number > 9) { $number = array_sum(str_split($number)); } echo $number; That's the easiest way, but it may never terminate under some circumstances
-
I suppose if I had to dig through poorly documented legacy code all of the time, I would feel the same.