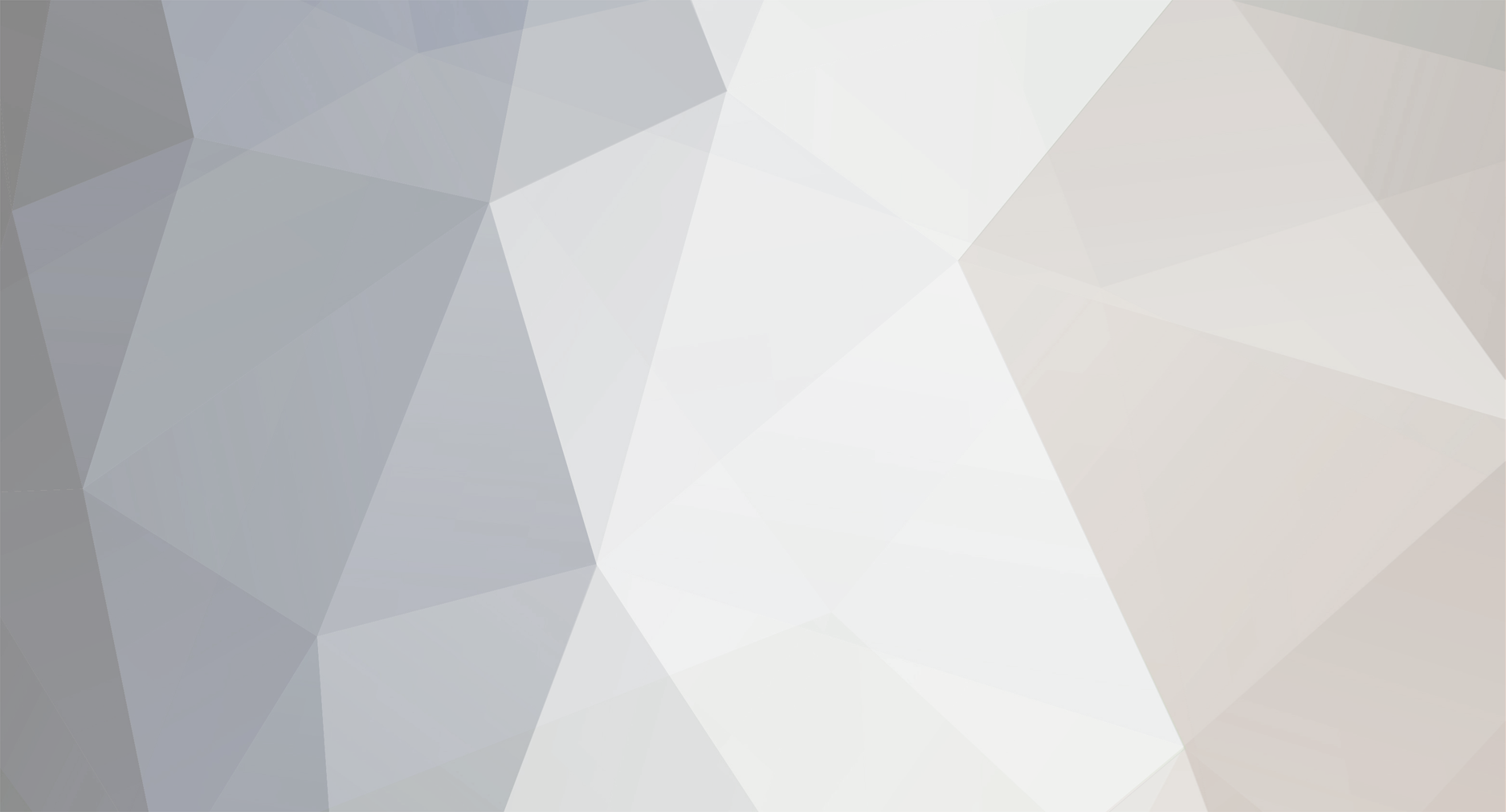
phil88
Members-
Posts
111 -
Joined
-
Last visited
Never
Everything posted by phil88
-
Hey guys. I've just uploaded my web development portfolio, what do you think of the design? Link I'm going to add another page that's sort of like a blog, but will be more of where I can re-post (and properly attribute) blog posts from elsewhere that are relevant to my field and that I find interesting. I'll probably write some original material for it too, but that won't be the main purpose. Once I've created that section, I'll probably have a "Latest Notes" or something on the featured work page underneath everything - but this is all probably a little way into the future.
-
This isn't really a regex problem. It sounds to me that the server is running in a different timezone to you or the server's time is just plain wrong. You can use date_default_timezone_set to set the timezone to whatever you want. As far as setting the server's clock, you'd have to get into contact with godaddy about that.
-
You could use APC to save the result of the query so that it doesn't have to be made on every page load, then whenever the table you're getting the global variables is updated just delete the cache so it doesn't have stale values and it'll rebuild with the new values on next page load. For example; // Getting the variables $cached = apc_fetch('global_vars'); if(!$cached){ // The variables aren't cached, so get them from the DB $query = "SELECT ... FROM global..."; $result = mysql_query($query); while($t = mysql_fetch_assoc($result)){ $cacheThis[] = $t; } apc_store('global_vars', $cacheThis); $useThis = $cacheThis; }else{ // The vars are cached, so just use from there $useThis = $cached; } // $useThis will have the variables you need // Then, where ever you have some code that updates the global values in the database, run this apc_delete('global_vars'); If your database is being updated so often that you're not getting many hits on the cache before the values change, it isn't really worth it.
-
How to make my website run on a non-standard port?
phil88 replied to kevk3v's topic in Apache HTTP Server
Out of curiosity, why do you want it to listen on a different port? -
Which version of Linux is best for webhosting server?
phil88 replied to shortysbest's topic in Linux
I always used Centos or Debian in the past, but recently I've been using Ubuntu more often. Despite being the 'noob desktop distro' - it still makes a pretty solid server OS. -
If the form used GET instead of POST to send the form (quite unlikely) you'll probably be able to get the email address from apache's access log (assuming your server is apache) If the script saves the info to a database (quite unlikely) you could get it from there.
-
If it's the link in the OP's sig, he has bigger problems than slow loading. The product's description has a glaring typo in it ...unless the plan was to use some psychological trickery to make me think you're less professional - in which case, you succeeded
-
You can have arrays of arrays. I can't remember the exact set up of $_FILES, but I suspect it's something like; $_FILES = array( 'filename.png' =>array( 'tmp_name' => 'sadfasdf.tmp', 'name' => 'filename.png', 'type' => 'image/png' ), 'filename2.jpg' =>array( 'tmp_name' => 'sadfasasdfwdf.tmp', 'name' => 'filename2.jpg', 'type' => 'image/jpeg' ) ); So $_FILE's elements can be accessed by string keys, but each element in $_FILE can also be accessed by their string keys because they're also associative arrays.
-
All the images.php file will do is create an image using GD/whatever, or read in the image from a file somewhere eg, using file_get_contents('something.png'); and then echo it with the appropriate header. For example; $imageContents = file_get_contents('animage.png'); header('Content-Type: image/png'); echo $imageContents; The header just tells the web browser to display what the script is sending it as a png image rather than trying to treat it as a text.
-
You could decrease the red value by x and increase green by x for every item from the 1st to the middle item. Then you decrease the green value by x and increase the blue value by x for every item until you reach the last item. x will have the same value throughout because you can work it out based on the number of items. Here's some psuedocode to help explain what I mean; $numItems = count($items); $middleItem = $numItems / 2; $red = 255; $green = 0; $blue = 0; $x = ?; foreach($items as $index => $item){ if($index < $middleItem){ $red -= $x; $green += $x; }else{ // $red should be 0 by now if $x is set correctly // $green should be 255 $green -= $x; $blue += $x; } echo $item in the colour rbg($red, $green, $blue); } I left you to work out how you figure out what the increment/decrement ($x) should be
-
Something along the lines of this? foreach($newarray as $item){ if($item['formtype'] == 'ContactUs'){ echo $item['screenloc']; # or whatever you want to do } }
-
I don't think there's much you can do without installing a server extension to run the encoded source because PHP is an interpreted language - so the source code has to be visible to the server it's running on. You could maybe do some kind of code obfuscation - but that's only going to discourage the mildly curious. It's by no means secure. If someone wants to crack your system, they'll do it.
-
Just as an extension to mattspriggs28's post - the main thing to know is never trust any data given to your website by the user. That data can be tampered with by the user and, if you're not careful, could cause havoc with your app. Running user supplied parameters through mysql_real_escape_string() like mattspriggs said is a good idea as it helps prevent the 'havoc' by escaping any characters that could modify the SQL query into something unplanned.
-
In the past I've written something that'll create thumbnails that are in proportion to the original image. This is the function I used, feel free to take it and modify it to fit your site. For my site, the parameters where, $id- Every image had a unique ID, the $url to the image and $selectedWidth was the maximum width that the image could be - this function doesn't care much for limiting the image's height. <?PHP function createLinkableThumbnail($id,$url,$selectedWidth){ $imageinfo = getimagesize($url); if(($imageinfo['mime'] == "image/jpeg") || ($imageinfo['mime'] == "image/pjpeg")){ $image = @imagecreatefromjpeg($url); }elseif($imageinfo['mime'] == "image/png"){ $image = @imagecreatefrompng($url); }else{ $image = @imagecreatefromgif($url); } $width = imagesx($image); $height = imagesy($image); $new_width = $selectedWidth; // Width can't be more than the width that has been given $factor = $width / $new_width; // Calc. the resize factor $new_height = $height / $factor; // Apply that factor to the image's height $image_resized = imagecreatetruecolor($new_width, $new_height); // Create blank canvas of the new dimensions imagecopyresampled($image_resized, $image, 0, 0, 0, 0, $new_width, $new_height, $width, $height); // Resize the image and put it on the blank canvas imagepng($image_resized,'images/thumbs/ext'.$id.'.png'); // Create the png file } ?> Hope this helps!
-
I managed to find this. It says its for going from HTML to PDF, but I don't know if it contains any method to go the other way or not.
-
Need help with displaying reults on same page please?
phil88 replied to roldahayes's topic in PHP Coding Help
D'oh! I totally misread the question. Apologies! -
Need help with displaying reults on same page please?
phil88 replied to roldahayes's topic in PHP Coding Help
You'd need to use javascript for that. -
[SOLVED] Visitor Voting System - Can only vote once - (W/ No Login)
phil88 replied to Steve Jabs's topic in PHP Coding Help
I think you need to aim for something less than bullet proof on this one. It's practically impossible to uniquely identify not just individual computers, but individual people on the internet - even with a login system. You could use HTTP_X_FORWARDED_FOR to get the LAN IPs through proxy servers to combat the problem of more than 1 machine accessing the site through the same internet connection - but that won't necessarily work as some proxies could block it, and even if it does work, more than 1 person might use the same machine in a day. What I think you need to do is analyse who are the end users of the system. Are they likely to be web savvy? Are they likely to be accessing the site from work? If not, simply setting a cookie or recording the IP is most likely going to be enough. If they are web savvy, it won't be enough. It's easy to change your IP or delete a cookie. If they are web savvy or access to the site from the same IP address is quite likely, maybe a login system would be more appropriate. -
The way I tend to check bugs with my code that are like this is to out put both things I'm trying to compare. In this case, one of those things is obviously False. So, put; var_dump(strtotime($_POST['date'])); before your if statement and see what comes out. If it's not what you're expecting, it's probably something wrong with $_POST['date'], ie, it doesn't contain what you're expecting it to - so try outputting that too. As far as syntax and logic goes, your code looks fine to me. Edit: Try using the strict comparison operator (===), might make a difference.
-
What is the exact output of the print_r($Comments) statement? Also, what exactly does $Comments have in it?
-
Ok, I managed to copy tasklist.exe off of another PC (that had XP Pro) and put it in my system32 folder and now it works Thanks for your help!
-
Either I have magical scripts, or it doesn't work on my set up. It always returns 0. Edit: I tried running the exec(...) stuff from a batch file, and it said: 'tasklist' is not recognised as an internal or external command, operable program or batch file. Is this because I have XP Home as opposed to Pro or a server edition?
-
[SOLVED] Display most common entry in a mysql column.
phil88 replied to kmutz22's topic in PHP Coding Help
$sql="select nm, count(nm) as total from users WHERE nm IS NOT NULL group by nm order by total desc"; I guess? -
I still don't understand what you're trying to do... what are you trying to update? and with what?