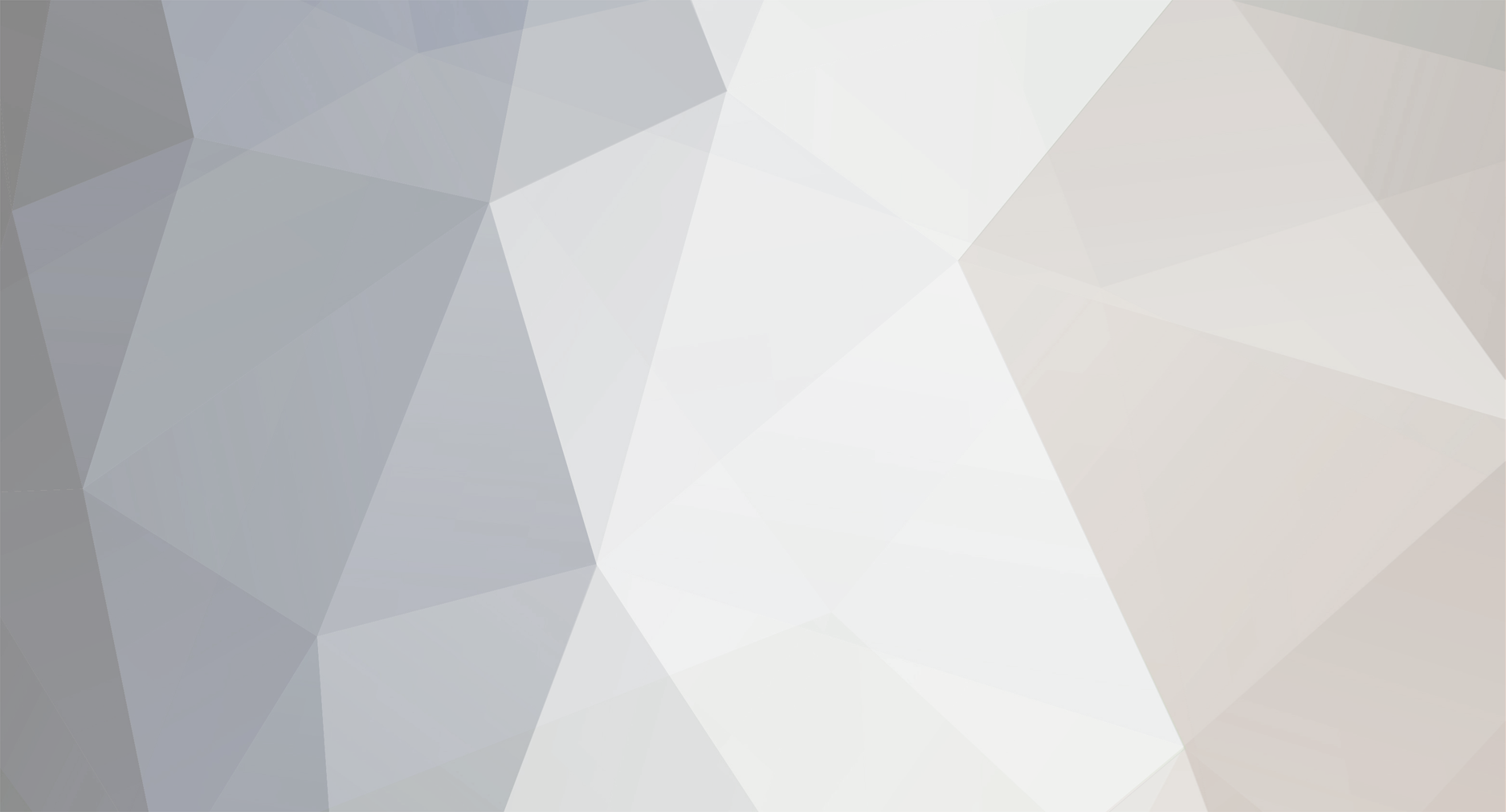
Jenk
-
Posts
778 -
Joined
-
Last visited
Never
Posts posted by Jenk
-
-
The error is exactly what it says on the tin.. you are not passing mysql resource handlers to the functions specified.
-
He might be using it for use in a query builder.. such as this method:
[code]<?php
public function parseQuery()
{
$num = func_num_args();
if ($num == 1)
{
$this->_query = func_get_arg(0);
}
elseif ($num > 1)
{
$query = func_get_arg(0);
for ($i = 1; $i < $num; $i++)
{
$arg = $arg = mysql_real_escape_string(func_get_arg($i), $this->_link);
if (!is_numeric($arg))
{
$arg = "'" . mysql_real_escape_string($arg, $this->_link) . "'";
}
$query = str_replace(':' . $i, $arg, $query);
}
$this->_query = $query;
}
else
{
throw new InvalidArgumentException('Must provide a query to be parsed!');
}
}
?>[/code]
usage..
[code]<?php
$db->parseQuery('SELECT * FROM `table` WHERE `col` = :1 AND `col2` = :2', $colVal, $col2Val);
?>[/code] -
Just a note.. this method of storage (delimeted list) is looked upon as 'sloppy' because, well, it is sloppy.
Use a table to store all the users entries with a foreign key for the user id and a foreign key for the book details. Essentiall 3 columns: index, userid, bookid.
Then select the rows from that table, with joins on users and books if necessary. It will be MUCH more efficient. -
[quote author=recset link=topic=107280.msg430332#msg430332 date=1157688533]
The database record just shows 'Array', but doesn't display what is in the array.
[/quote]you need to convert the array to a string before inserting to a database. A sloppy way is to implode() the array with a delimeter, then explode() it when you retrieve from the site, a better way is to create a new table for NOTES and use the foreign key of user ID or post ID or whatever it is that is the 'parent' entity of NOTES.
For example; the NOTES are child members of a form for a book review..
so;
[code]<?php
$link = mysql_connect('host', 'user', 'pass');
mysql_select_db('db', $link);
function magic_escape($value, $link)
{
if (get_magic_quotes_gpc()) $value = stripslashes($value);
return mysql_real_escape_string($value, $link);
}
if (!empty($_POST['NOTES']))
{
foreach ($_POST['NOTES'] as $note)
{
if (!mysql_query(
"INSERT INTO `book_reviews` (`notes`) VALUES ('"
. magic_escape($note, $link) . "') WHERE `bookid` = '"
. magic_escape($_POST['bookid']) . "'"
))
{
die('Error inserting note: ' . htmlentities($note));
}
}
}
?>[/code]
Then when selecting..
[code]<?php
$link = mysql_connect('host', 'user', 'pass');
mysql_select_db('db', $link);
function magic_escape($value, $link)
{
if (get_magic_quotes_gpc()) $value = stripslashes($value);
return mysql_real_escape_string($value, $link);
}
if (!empty($_POST['bookid']))
{
$result = mysql_query(
"SELECT `notes` FROM `book_reviews` WHERE `bookid` = '"
. magic_escape($_POST['bookid'], $link) . "'"
);
if (!$result) die('Error selecting notes for bookid: ' . htmlentities($_POST['bookid']));
while ($row = mysql_fetch_assoc($result))
{
echo '<div class="note">' . htmlentities($row['note']) . "</div>\n";
}
}
else
{
echo 'No notes';
}
?>[/code]
untested -
It's not reliable though :)
-
php?> is not a valid closing tag, fyi :)
-
it's a 404 message, file not found.. therefore the link you are clicking points to a file or directory that does not exist.
-
[quote author=redarrow link=topic=107327.msg430431#msg430431 date=1157708572]
rubbish correct way to format a form ok.
how brake it?
[/quote]what if short tags are off? ;) -
redarrow - that won't make any difference, and may well infact break it altogether..
-
can you also post the full error message please?
(view the page source if necessary) -
the file that the link points to does not exist.
-
don't use it.. just use a session variable, database table or plain old flat file to store the info.
If you are emailing someone a link to confirm registration or such, use the ID field from a database table. -
still experiencing problems?
echo all your queries to see if they are 'correct' -
[code=php:0]if ($do == Login)[/code]
should be:
[code=php:0]if ($do == 'Login')[/code]
and
[code=php:0]$do = stripslashes($_REQUEST['do']);[/code]
should be:
[code=php:0]function magic_strip($value)
{
if (get_magic_quotes_gpc())
{
$value = stripslashes($value);
{
return $value;
}
$do = magic_strip($value);
[/code] -
ask on phpbb forums, plenty of hacks and other modders there.
-
post code please.
-
However, your nested mysql_query()'s won't impress them at all :p
Learn to use JOIN's.. -
don't tak offence... I merely corrected a mistake..
-
use realpath() for stability.
-
If the billing site is that bad, which I strongly believe it not to be and you are mistaken, then find a new billing site. all billing needs to know is cost and payment method, so tally up your users cost and then submit that to the billing site.
-
If it's local, don't use $_POST.
There will be recommendations for $_SERVER['HTTP_REFERER'] and/or $_SERVER['REMOTE_ADDR'] but they are [b]very[/b] unreliable. -
[code=php:0]foreach(glob(realpath('smile') . '/*.gif') as $file)
{
echo '<option value="' . hmtlentities($file) . '">' . htmlentities($file) . '</option>';
}[/code] -
redarrow - it's [code=php:0]$_SESSION['username'][/code] not [code=php:0]$_SESSION[username][/code]
-
Page 1:
[code]<?php
session_start();
$_SESSION['variable'] = 'Hello world!';
header('Location: page2.php');
?>[/code]
Page2.php:
[code]<?php
session_start();
echo $_SESSION['variable'];
?>[/code]
explode() and arrays
in PHP Coding Help
Posted
[code]CREATE TABLE `favorites`(
`id` INT NOT NULL AUTO_INCREMENT,
`bookid` INT NOT NULL,
`userid` INT NOT NULL
) PRIMARY KEY(`id`);[/code]