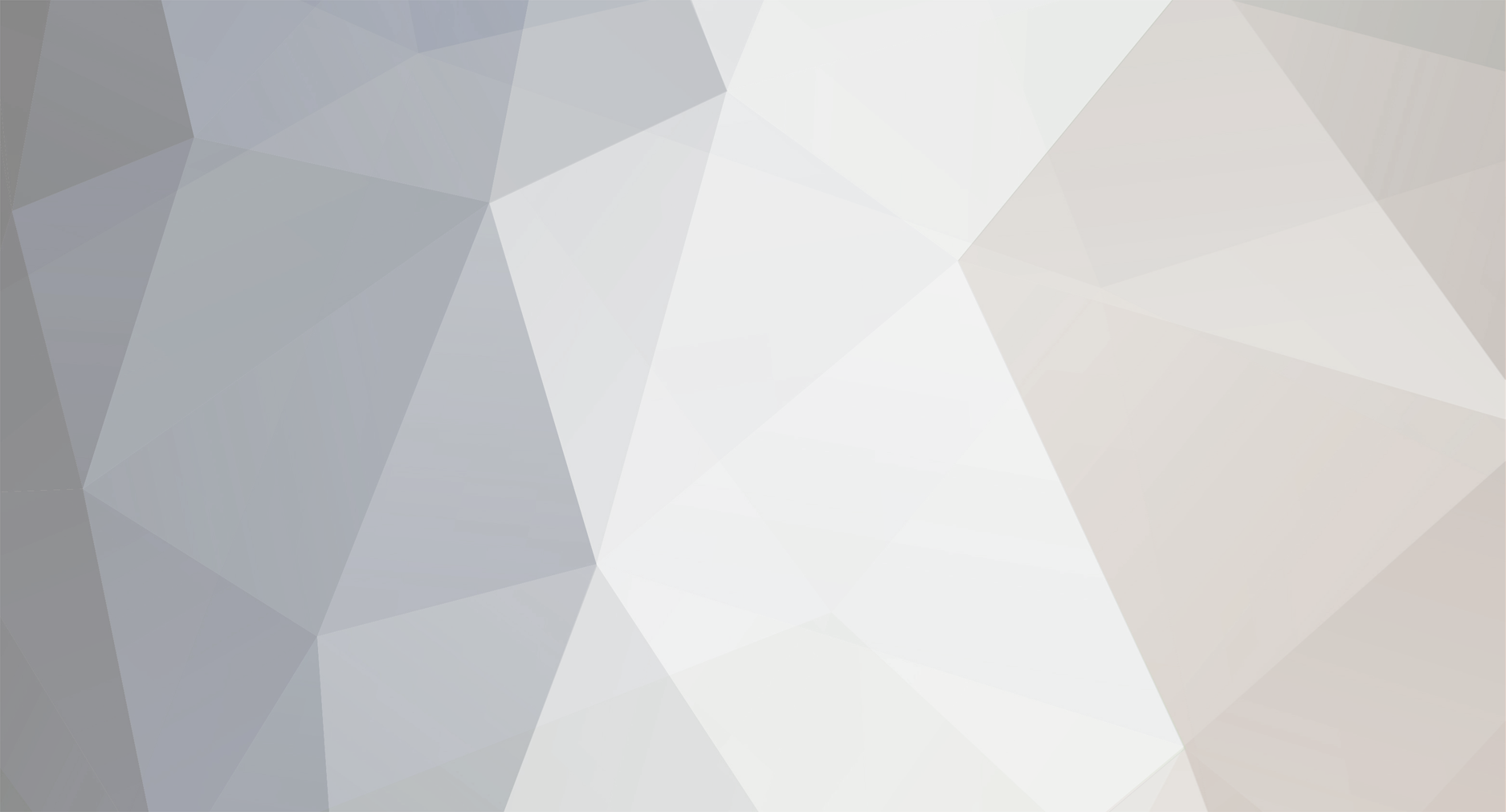
Jenk
-
Posts
778 -
Joined
-
Last visited
Never
Posts posted by Jenk
-
-
Use a static variable for counting, not global :)
-
whoops... that was my bad (I recognise the script :p)
change to:
[code]<?php if (preg_match('/[' .preg_quote('\/:*?"<>|', '/') .']+?/', $file)) { ?>[/code]
obviously without the <?php ?> tags.
ditto for the preg_replace. -
What's stopping you using the constructor?!
[code]<?php
class Foo
{
protected $bar;
public function __construct ()
{
$this->bar = 'foobar';
}
}
?>[/code] -
in a word.. No.
Besides, PHP uses C directly for all primitives, only exception to the rule is string key is allowed. (Uses a secondary array for the keys)
Why is it such a concern? What exactly are you doing with arrays to make them so huge? -
dude.. echo the value of $sql and paste it here.. for the love of god..
-
The form:
[code]<form action="page.php" method="post">
<textarea name="mytextarea" cols="50" rows="5"></textarea>
<br />
<input type="submit" />
</form>[/code]
The php for page.php:
[code]<?php
$link = mysql_connect('host', 'user', 'pass');
mysql_select_db('db', $link);
if (!empty($_POST['mytextarea'])) {
$sql = "INSERT INTO `table`(`column`) VALUES('" . mysql_real_escape_string($_POST['mytextarea']) . "'";
} else {
echo 'Input something you numpty!';
}
//mysql_query etc down here.
?>[/code] -
Dude, Think about it..
Everytime a user clicks the add to basket button, your code need to add that product..
So therefore you already know that you'll be inserting a record, or adding another item to an array, or writing another line to a flat file etc.
Vice versa for removing the item.
A very quick untested example:
[code]<?php
class Basket
{
private $userId;
private $db;
public function __construct (DataBaseClass $db, $userId)
{
$this->db = $db;
$this->userId = (int) $userId;
}
public function addItem ($prodId, $quantity)
{
try
{
$this->db->parseQuery
(
'REPLACE INTO `basket` (`userId`, `prodId`, `quantity`) '
.'VALUES(:1, :2)'
,$prodId
,$quantity
);
$this->db->commit();
}
catch (DataBaseException $e)
{
throw new BasketException('Error adding item');
}
catch (Exception $e)
{
throw $e;
}
}
public function removeItem ($prodId, $quantity)
{
try
{
$this->db->parseQuery(
'SELECT `quantity` '
.'FROM `basket` '
.'WHERE `prodId` = :1 '
.'AND `userId` = :2'
,$prodId
,$this->userId
);
$this->db->commit();
$quant = (int) $this->db->fetchField('quantity');
if ($quant <= $quantity)
{
$this->db->parseQuery
(
'DELETE FROM `basket` '
.'WHERE `prodId` = :1 '
.'AND `userId` = :2 '
,$prodId
,$this->userId
);
}
else
{
$this->db->parseQuery
(
'UPDATE TABLE `basket` '
.'SET `quantity` = `quantity` - :1 '
.'WHERE `prodId` = :2 '
.'AND `userId` = :3'
,$quantity
,$prodId
,$userId
);
}
$this->db->commit();
}
catch (DataBaseException $e)
{
throw new BasketException('Could not remove item.');
}
catch (Exception $e)
{
throw $e;
}
}
}
?>[/code] -
actually, use the keyowrd LIMIT in your statements, not mysql_data_seek.
-
first, learn to sanitise your user input for mysql (hint: read up on mysql_real_escape_string)
2nd, search for Pagination, plenty of reading material. -
sanitise all input for mysql with mysql_real_escape_string();
-
[quote author=wildteen88 link=topic=106833.msg427692#msg427692 date=1157372216]
Use
[code=php:0]$calc = "\$rate = str_replace(array(\"L\",\"E\",\"F\",\"I\"), array(\$l, \$e, \$f, \$I), \$field1);";[/code]
If you are using eval.
However i see no use for eval here. Just use:
[code=php:0]$rate = str_replace(array("L","E","F","I"), array($l, $e, $f, $I), $field1);[/code]
[/quote]it appears that $field1 contains an equation, the OP is trying to replace Keywords with values, and then execute the equation. eval() will be needed if the OP wants that equation to execute in PHP :) -
in that case: $field1 == ('' || null);
you need to look at where $field1 is declared and defined. -
dude.. you've been given an example. From that example you can tell what needs doing..
[code]<?php
if ($user->isLoggedIn()) {
if ($user->isOperator()) {
$image = 'pink with yellow pokadots';
} else {
$image = 'green'; //display green image
}
} else {
$image = 'red'; //display red image
}
?>[/code] -
change the eval to echo, what do you get?
-
[quote author=nickholt1972 link=topic=106411.msg425569#msg425569 date=1157054505]
thanks sasa.
I need to get myself a finer toothcomb I think.
Regards.
[/quote]You just need a text editor with highlighting, as you can see on this board - it helps :) -
[code]<?php
$calc="\$rate=".str_replace(array("L","E","F","I"), array('$l', '$e', '$f', '$I'),$field1).";";
eval($calc);
?>[/code] -
Well you've got your $result array returned by the function (which you've stowed away in var $a)
so with $a, assign to the template.
the template then iterates and decorates the data, ala my previous example where I assumed Smarty. -
just a note.. your accessing your arrays incorrectly..
[code=php:0]$array['index'];[/code]
not
[code=php:0]$array[index];[/code] -
use file() and foreach()
[code]<?php
foreach (file('urls.txt') as $line) {
WHOPPHIE($line); //whatever 'WHOPPHIE' does..
}
?>[/code] -
ok, well it looks like you are going majorly overkill with your arrays then.
Instead of creating so many arrays and doing all the shennanigans with them, just extract from the DB what you need - the image source link and the text by the looks of it.
Then assign that directly to your template engine, then have the template iterate over the values outputting the HTML. -
$a is an array, where is this declared and defined? (i.e. can you post the loop that creates this array)
-
recursion is what you need, and a lot of patience.
[code]<?php
function filelist($dir)
{
if (!$dir = realpath($dir)) return null;
static $files = array();
static $dirs = array();
$handle = opendir($dir);
while (($file = readdir($handle)) !== false)
{
if (!in_array($file, array('.', '..')))
{
if (is_dir($path = ($dir . DIRECTORY_SEPARATOR . $file)))
{
$dirs[] = $path;
filelist($path);
} else {
$files[] = $path;
}
}
}
return array('dirs' => $dirs, 'files' => $files);
}
echo nl2br(print_r(filelist('.'), true));
?>[/code] -
Which 'number'? The index is specified by the $key variable, do you mean something else, like item ID or similar?
-
righto.
[code]<?php
exec('ping www.urlofthesiteyouwantoping.com');
?>[/code]
Dynamic Gallery... is this possible in PHP?
in PHP Coding Help
Posted
Take a look at [url=http://robm.me.uk/projects/simplegal/]SimpleGal[/url]