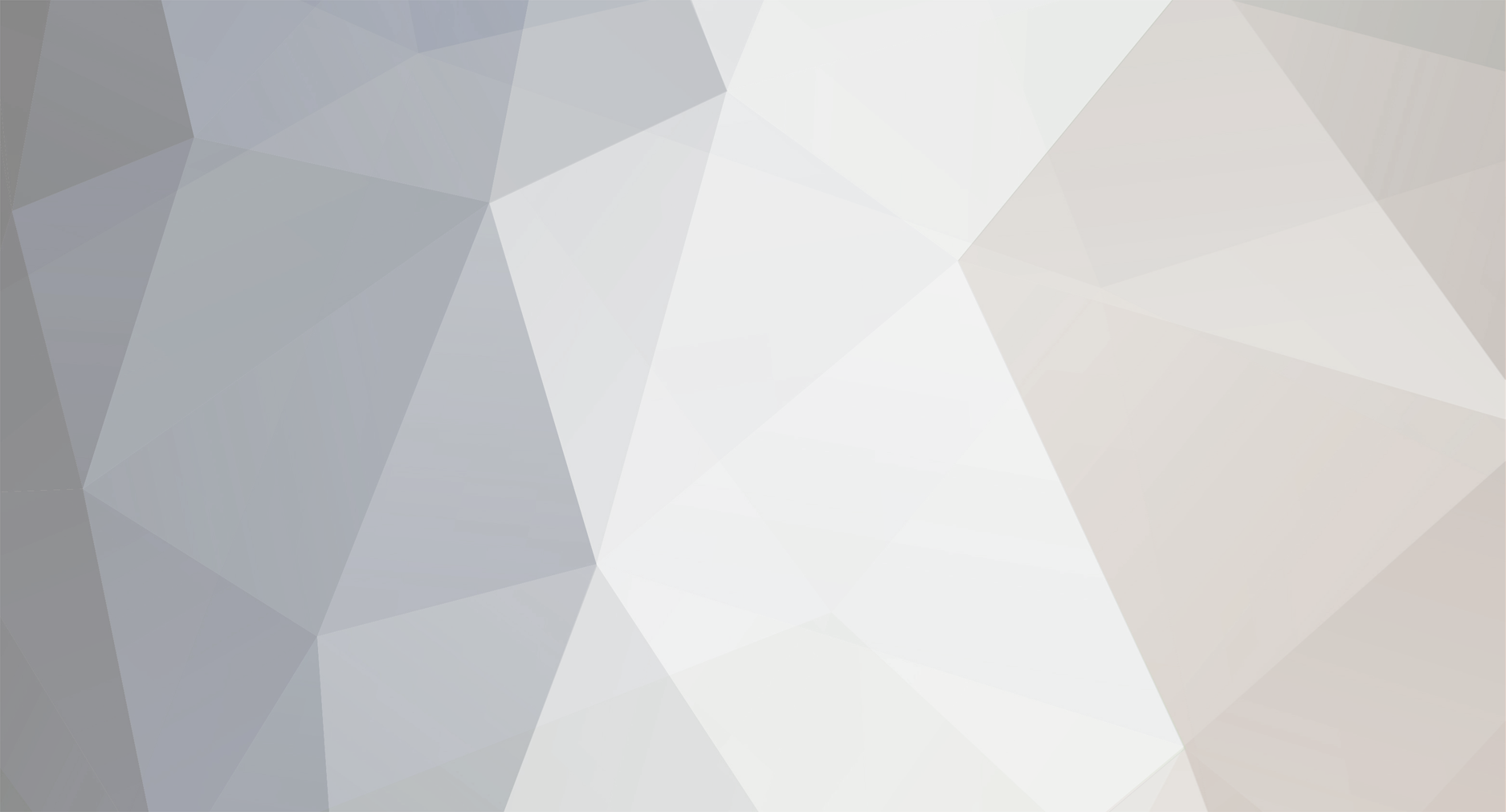
HuggieBear
Members-
Posts
1,899 -
Joined
-
Last visited
Everything posted by HuggieBear
-
Can I give you some advice... Always echo the error out when developing... [code]<?php $sql = "INSERT INTO pets_owned VALUES('', $userid, $pet, 1, 1, 1)"; $result = mysql_query($sql, $c); if (!$result){ echo "Couldn't execute $sql:" . mysql_error(); } ?>[/code] Regards Huggie
-
Try a semicolon after this line: [code=php:0]date1=date("d F Y")[/code] Regards Huggie
-
My advice would be to echo your code for the previous mysql query as the problem isn't with the call to [color=green]mysql_num_rows()[/color] it's actually more than likely with [color=green]mysql_query()[/color]. You probably have something like this: [code=php:0]$result = mysql_query("SELECT column_name FROM table_name");[/code] try changing to this: [code=php:0] $sql = "SELECT column_name FROM table_name"; $result = mysql_query($sql); if (!$result){ echo "Couldn't execute query: $sql\n\n" . mysql_error(); } [/code] This will tell you why your result isn't valid. Regards Huggie
-
[quote author=tracy link=topic=117288.msg478546#msg478546 date=1165252878] I would not want each dealer to be logging into the main site database for obvious reasons. Say an employee of one dealer got mad and took down the site? Say there was some error by a dealer and he deleted his database. I know it might be rare, but these things happen. [/quote] This indicates poor coding and database design, with the correct permissions for database users and well designed queries in the php, you can prevent this from happening. Regards Huggie
-
I'd suggest going the other way. Have one database (the big one you mentioned) with all the data in it. Then have all the websites only select the content they need for their individual site. It's a lot easier to do it this way than in reverse the way you're doing it, and it means only one database to maintain. The disadvantage is may have to modify your database structure a little, carry out some normalisation on it to make it a feasible solution. Regards Huggie
-
The code's almost correct... The problem is that the submit button doesn't have a name... This should work. [code]<?php session_start(); if (!isset($_POST['submit'])) { echo "<form method='post'>"; echo " your name?<input type='text' name='uname'>"; echo " <input type='submit' name='submit'>"; echo "</form>"; } else { $_SESSION['uname'] = $_POST['uname']; echo "Welcome {$_SESSION['uname']}, your session id is ". session_id(); } ?>[/code] Regards Huggie
-
Can you post your code? Regards Huggie
-
Why not give this a try, it's using preg_match(). [code]<?php // Test data as provided $data1 = "audun is doing his homework;;http://www.audun.com/test.php;whenever he feels like it"; // Match the data and stick it into the matches array preg_match("/(.*?);;(.*?);(.*)/ms", $data1, $matches); // Delete the first element in the array (the full match) array_shift($matches); // Dump the data to show what was captured echo "<pre>\n"; var_dump($matches); echo "</pre>\n"; ?>[/code] Regards Huggie
-
It looks OK, so long as the user you connect to the server with has the correct permissions. Regards Huggie
-
It's not that much of a security risk, I did say minor. Even with what you have people won't know your database name or table name. Regards Huggie
-
[quote author=JJohnsenDK link=topic=117282.msg478348#msg478348 date=1165231794] Thats not working... hent_pos isnt a row in the table... [/quote] That's what I suspected, when you say rows, you mean columns... Give what I posted a try. Regards Huggie
-
I got the impression that your terminology was wrong. When you say row names, do you really mean column names, as rows don't have 'names'. If you meant column names, then give this a try. [code]<?php // Connect to your database include('dbconnect.php'); // Run the query to get the field names $sql = "SHOW COLUMNS FROM wof_best"; $result = mysql_query($sql); // Start to echo the select code echo "<select name='columns'>\n"; while ($row = mysql_fetch_array($result, MYSQL_ASSOC)){ echo "<option value='".$row['Field']."'>".$row['Field']."</option>\n"; } echo "</select>\n"; ?>[/code] There are certain to be some minor security issues with this, as I can't see that it's good practice to make your column names visible to the public, but the code should work in theory. Regards Huggie
-
I think phpMyAdmin is probably the way to go here. Install it your server and then connect to the database. Assuming the user you're using has the correct privileges, you should be fine. Regards Huggie
-
Very confusing conditional statement problem
HuggieBear replied to unitedintruth's topic in PHP Coding Help
Based on your original layout, I'd go with the following, but that's just because I like using variables as opposed to just echoing the code out. [code]<?php if ($cmd =="List"){ $align = "center"; } elseif ($cmd == "Details"){ $align = "center"; } else { $align = "left"; } ?> <table> <tr> <td align="<?php echo $align; ?>"> Your content goes here </td> </tr> </table>[/code] Regards Huggie -
No problem, this is of course liable to break the moment MySpace make any changes, but it should just be a case of changing the regular expression. I found that it worked OK for normal alphanumeric characters, but when someone had a username that had special characters in, the XML didn't like it. You'll have to figure that one out for yourself. Regards Huggie
-
The website didn't really help, but I did come up with the following code, so give it a try. You may have problems with the XML but you can probably fix them better than I can. Regards Huggie P.S. Admins/Mods: I didn't use [nobbc][code][/code][/nobbc] tags for this as it throws the syntax colouring out. I would normally use it ;) [code=php:0]<?php // Turn on all error reporting error_reporting(E_ALL); // Create a new CURL handle $ch = curl_init(); // Set the CURL options curl_setopt($ch, CURLOPT_URL, "http://www.myspace.com/tom"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); // Put the data received from the page into a variable $data = curl_exec($ch); // Output an error to the browser if execute errors if (curl_errno($ch)){ echo "Error: " . curl_error(); } // Close the object curl_close($ch); // Regular expression to capture the data into the comments array $pattern = '/bgcolor="FF9933" style="word-wrap: break-word">.*?id=(\d+)".*?>(.*?)<.*?src="(.*?)".*?text10">(.*?)<.*?<br>.*?<br>(.*?)<\/td>/ims'; preg_match_all($pattern, $data, $comments, PREG_SET_ORDER); // Dispose of the full pattern matches foreach ($comments as $k => $v){ array_shift($comments[$k]); } // Strip out all the white space from 'userName', 'postedDate' and 'commentText' foreach ($comments as $k => $v){ $comments[$k][1] = trim($comments[$k][1]); $comments[$k][3] = trim($comments[$k][3]); $comments[$k][4] = trim($comments[$k][4]); } // Echo the XML echo "<?xml version=\"1.0\" encoding=\"UTF-8\"?>\n"; echo "<first_child>\n"; foreach ($comments as $k => $v){ echo " <commentData>\n"; echo " <userID>{$comments[$k][0]}</userID>\n"; echo " <thumbnail>{$comments[$k][2]}</thumbnail>\n"; echo " <postedDate>{$comments[$k][3]}</postedDate>\n"; echo " <userName>{$comments[$k][1]}</userName>\n"; echo " <commentText>{$comments[$k][4]}</commentText>\n"; echo " </commentData>\n"; } echo "</first_child>\n"; ?>[/code]
-
Do you have the code live somewhere that I can test it? Regards Huggie
-
OK, I'm still looking, I like this little challenge :) Huggie
-
Try: [code]SELECT * FROM `members` WHERE `username` LIKE '%?%';[/code] See what that brings up, as far as I'm aware, the '?' isn't a wild card in MySQL, however, the '%' and '_' are. Regards Huggie
-
I'll take a look at this for you this evening. Just a quick question, you have to be logged into MySpace to be able to access that content, how do you expect to get around that? Regards Huggie
-
Try this: [code]<?php $result = mysql_query("SELECT links.*, linkcats.linkcattext FROM links INNER JOIN linkcats ON (links.linkcat = linkcats.linkcat) ORDER BY linkcats.linkcat",$connect); $category = null; while($row = mysql_fetch_array($result)) {//begin loop if (is_null($category || strcmp($category, $row['linkcattext']) != 0) $category = $row['linkcattext']; // New category, break it up! echo "<h1>$row[linkcattext]</h1>\n"; } echo "<a href=\"$row[linkurl]\">$row[linktext]</a><br />\n"; }//end of loop ?>[/code] Regards Huggie
-
Are you sure this isn't a caching issue? Regards Huggie
-
Add the following to the top of your code... [code=php:0]error_reporting(E_ALL);[/code] Regards Huggie