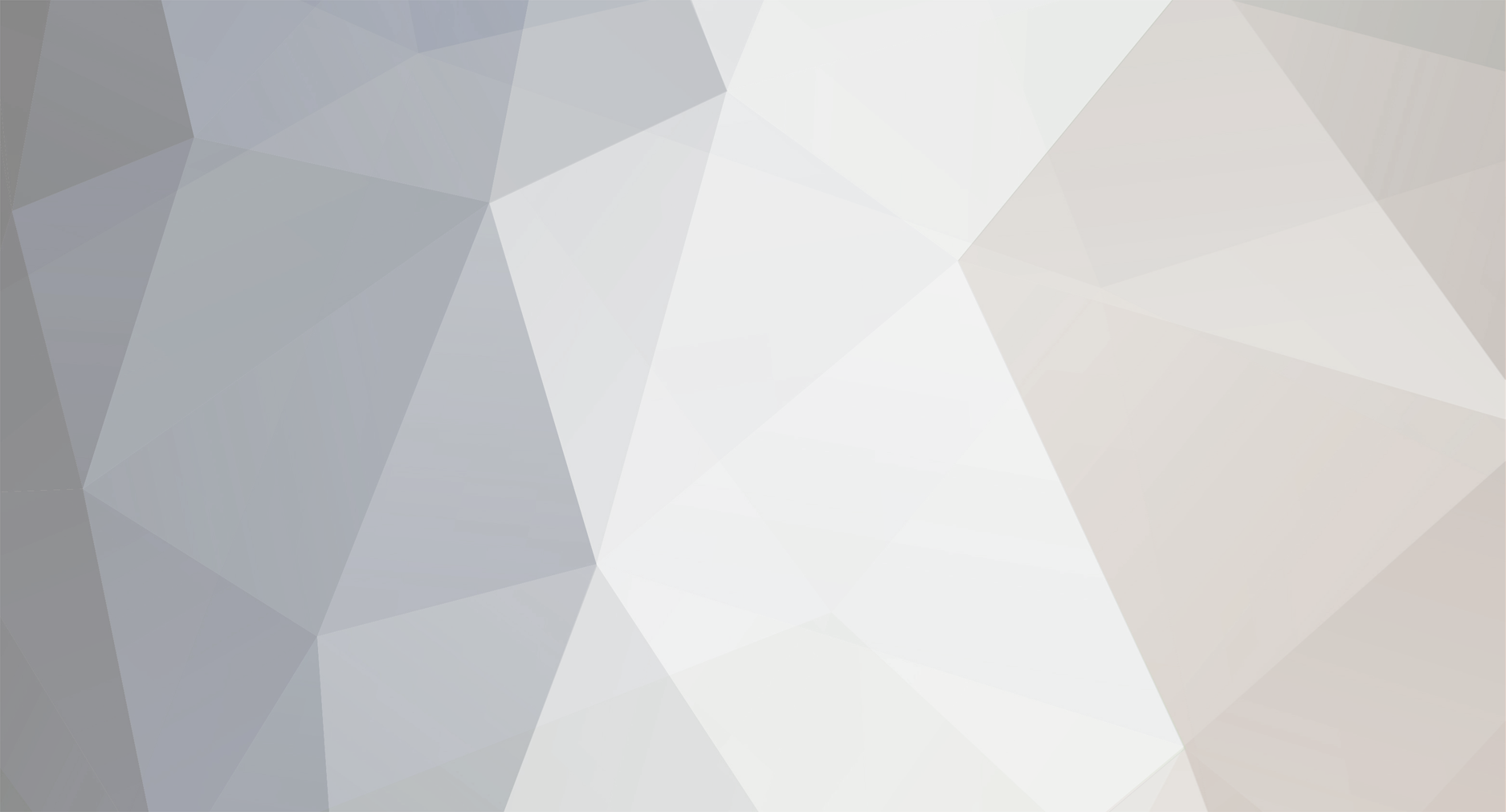
pkSML
Members-
Posts
191 -
Joined
-
Last visited
Everything posted by pkSML
-
Here's how I would do it: <?php $how_many_fields = $_POST['how_many_fields']; for ($i = 1; $i <= $how_many_fields; $i++) { echo "<input name=\"description[$i]\" type=\"text\" id=\"description[$i]\">". "<input name="price[$i]" type="text" id="price[$i]" />" } echo "<input type='hidden' value='{$how_many_fields}' name='fieldnums'"; ?>
-
There's no need to perform a foreach loop on $_POST data. There will always only be one value for each posted field.
-
[SOLVED] Calender script not working with session
pkSML replied to matt.sisto's topic in PHP Coding Help
Here's the answer: $row = mysql_fetch_row($result); If you change that to mysql_fetch_array, you should get your desired results. You can also change this line: $_SESSION['username'] = $row['first_name']; to this: $_SESSION['username'] = $row[2]; // assuming Malcolm is in the first_name column per your example -
[SOLVED] Calender script not working with session
pkSML replied to matt.sisto's topic in PHP Coding Help
if ($row != null) { $_SESSION['username'] = $row['first_name']; header("Location: index.php"); exit(); } You might change these lines of code to this (just for testing purposes): if ($row != null) { $_SESSION['username'] = $row['first_name']; echo "<PRE>" . var_dump($row) . "</PRE>"; /* header("Location: index.php"); exit();*/ } Post the output of that. -
[SOLVED] Calender script not working with session
pkSML replied to matt.sisto's topic in PHP Coding Help
Obviously, you're not properly logged in. Just for the moment, copy and paste this data at the VERY TOP of your cal.php script. <?php session_start(); echo "<PRE>"; var_dump($_SESSION); echo "</PRE>"; exit(); ?> What output does it give? -
You don't have many attractive options here. Here's what I can come up with. 1. Use javascript. It can complete an AJAX request with POST data. This requires the user to have javascript turned on in their browser. 2. Condense your download.php and antigenic.php into one script. That way, all variables would still be available. 3. Make a form with hidden elements to get the user from page 2 to page 3. They would have to click on a button to submit the form data. 4. Save the data that you need transferred as session data. This requires cookies to be turned on in the user's browser. 5. Save the important data as a text file on the server, and in your redirection (from download.php), put the filename in the query string. Have the script delete the file when you're done with it. If none of these options work for you, I think you're stuck. Sessions would be the easiest method in my opinion.
-
IMO, the easiest way to get variables into your function is to use the global command. <?php $user=$_POST['user']; $country=$_POST['country']; function indiv_user() { global $user, $country; // this is the first line of your function // ...... function all_user() { global $user, $country; // this is the first line of your function ?> Also, make sure your input data in sanitized! You're using raw POST data to enter into your database, which can lead to security issues. See the php manual on mysql_real_escape_string.
-
First thing: make sure your input data in sanitized! You're using raw POST data, which can lead to security issues. See the php manual on mysql_real_escape_string. So you're getting duplicate results for any address entered. Here's a 'hack' way to do it: if (mysql_num_rows($var) > 0) { // any further processing here // use mysql_fetch_array in a while loop to get each }
-
You can use a form to POST the pertinent data on the 3rd page. The third page would have to be PHP, not HTML (because you want dynamic content). Then, just read the appropriate POST data element into the textarea. You'll want to use this format: <?php echo "<textarea style=\"height: 500px; width: 500px;\">" . htmlentities($_POST['dataField']) . "</textarea>"; ?> Note: If you need more help, you'll need to be a little clearer in your explanation.
-
Here's the last few lines of your code: <?php if (preg_match('/jpg|jpeg|JPG|JPEG/',$ext)) { imagejpeg($newimg,$file); } if (preg_match('/gif|GIF/',$ext)) { imagegif($newimg,$file); } if (preg_match('/png|PNG/',$ext)) { imagepng($newimg,$file); }?> Shouldn't you use your $dest variable? <?php if (preg_match('/jpg|jpeg|JPG|JPEG/',$ext)) { imagejpeg($newimg,$dest); } if (preg_match('/gif|GIF/',$ext)) { imagegif($newimg,$dest); } if (preg_match('/png|PNG/',$ext)) { imagepng($newimg,$dest); }?>
-
<?php $data["History"] = "long value 1"; $data["Contact"] = " value text 2"; $data["Offer"] = "long value 3"; $data["Clients"] = "long value 4"; ?> What happens when this code is saved to about.php and included on your main page?
-
Glad to help
-
Here's a way to do tags that are nested. I've not seen this elsewhere, but I *think* it would work. Handle the QUOTE and /QUOTE conversions individually. Obviously, if someone had more opening tags than closing tags, this might cause a problem. But I think that would be rare. What do ya' think?
-
Silly me... It was greedy, and the U modifier turns that off.
-
I'm trying to get this regex to work, but wind up banging my head on the wall instead... I'm grabbing the NOAA zone forecasts for Ohio (from http://www.weather.gov/view/prodsByState.php?state=oh&prodtype=zone ) You can see from the source code of that page that all the forecasts from a certain time are in < pre>< /pre> tags. The next oldest forecast is in another < pre>< /pre> tag set. I want to grab each forecast as an item in an array. Here's my code: <?php preg_match_all('@<pre>(.*)</pre>@s', $source, $results); print_r($source); ?> Unfortunately, I'm getting everything from the first opening PRE tag to the last closing PRE tag. Preg_match_all is greedy by default, isn't it? Thanks for any help/explanation!
-
Glad to hear it's finally working for ya'!
-
1. You'll want to check out http://php.net/getimagesize 2. Simple example of text becoming an image: http://code-bin.homedns.org/91 :: Google search 3. http://pksml.net/search/php+resize+image 4. http://nyphp.org/content/presentations/GDintro/ (the next page link is in the upper-right)
-
OK. This is really not that complex. The uploading is the complex part. Your image.php script: (yes, ten lines is all this simple script needs) <?php $imageid = $_GET['file']; // *****Insert MySQL connection details here***** $query = "SELECT Image, Type FROM $Images WHERE ImageId='$imageid'"; $result= MYSQL_QUERY($query); $data = @ mysql_fetch_array($result); header("Content-type: image/jpeg"); echo $data["Image"]; ?> Your page with the image on it: <img src="http://www.******.com/imageupload.php?file=11"> BTW, what type of image have you uploaded? Is it a JPG, GIF, PNG??? That will affect the header in the image script. I think that since you're getting the red box with the X in it, you should re-upload the image to the database.
-
<?php header('Content-type: image/gif'); readfile("image.gif"); //sends binary information -- the image ?> Above is the barebones to displaying an image with PHP. Notice you need to send an HTTP header that tells the browser what kind of information it's receiving. That's why you're getting all the gobbly-gook. The browser doesn't know it's an image. Search image mime types for the correct mime type to use. Notice that all your code is missing is the header. //test viewing an image from the database $query = "SELECT Image, Type FROM $Images WHERE ImageId='11'"; $result= MYSQL_QUERY($query); $data = @ mysql_fetch_array($result); echo $data["Image"];
-
Can you paste the code to display your image on The Code-Bin and post the link? (I'm thinking the HTTP header has something to do with your problem.)
-
Try comparing what you have to what's in this tutorial: http://www.phpriot.com/articles/images-in-mysql/1 (this tutorial has 10 pages to look through) I'll have more time to look at this later today.
-
Are you sending out a proper header? I don't think you're understanding how to use the image script. You have a webpage that you want to place a database image in. We'll say your script is called image.php. In your webpage, you display the image as < img src="/image.php?id=5"> Then you should see an image like it's supposed to be.
-
You would call the image as so in your page: < img src="http://yoursite.comimage.php?id=6"> (without the space :-) In your image.php script, you send the image like this: // get the image from the db $sql = "SELECT image FROM testblob WHERE image_id=0"; // the result of the query $result = mysql_query("$sql") or die("Invalid query: " . mysql_error()); // set the header for the image header("Content-type: image/jpeg"); echo mysql_result($result, 0); Source: http://www.phpriot.com/articles/images-in-mysql/8
-
$uploaddir = 'http://funbox.wz.cz/'; You need to save the file locally with the filesystem. You can't save a file to a URL.
-
Also, please place your PHP code within code tags. Just remove the spaces from what you see below. [ code][ /code] OR you could post your code at The Code-Bin ( http://code-bin.homedns.org ), which is a site I run for such a purpose