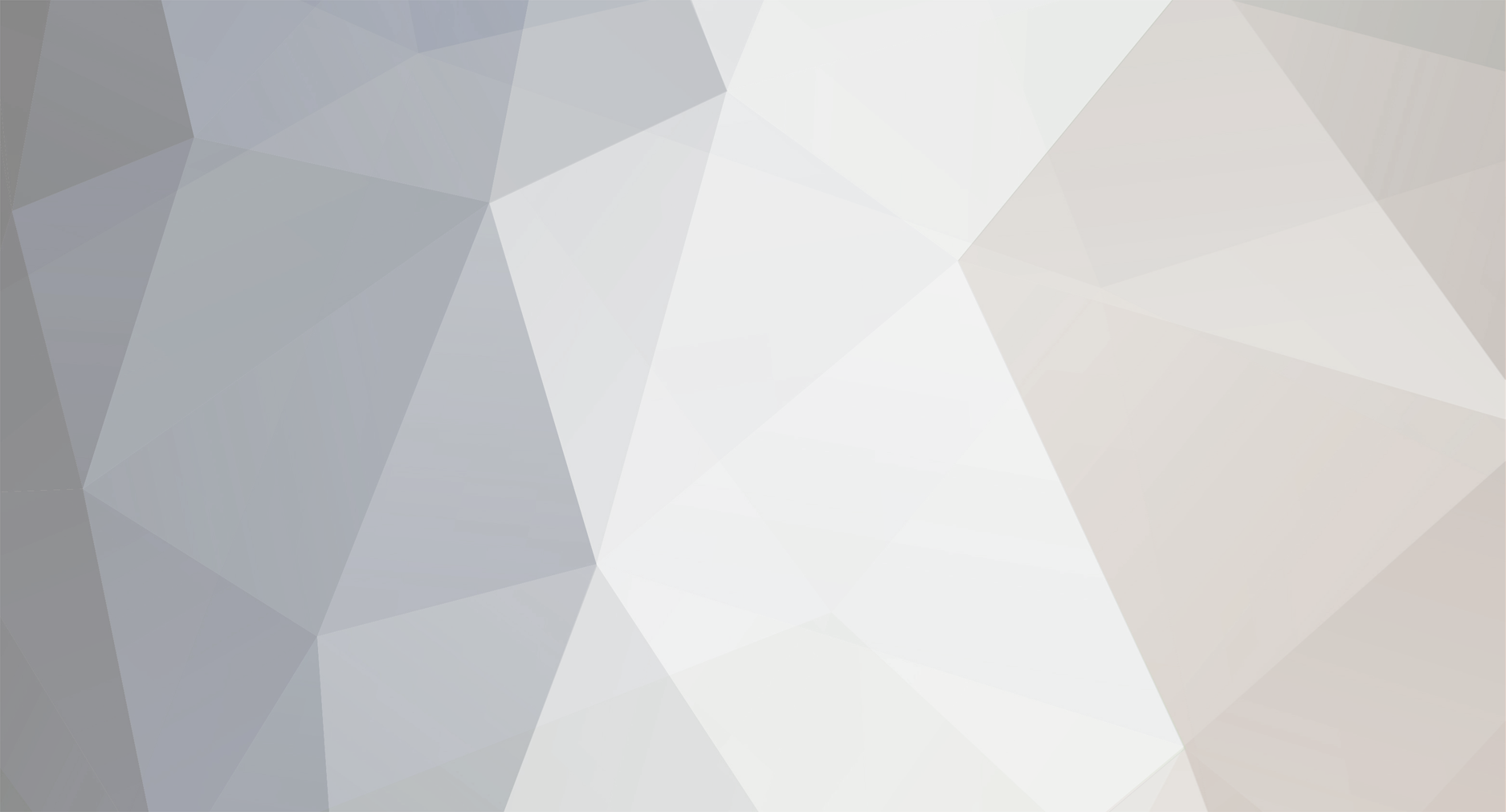
pkSML
Members-
Posts
191 -
Joined
-
Last visited
Everything posted by pkSML
-
-
Just alter this line: imagejpeg($image); to // $newdir is the new folder where you want to save watermarked images -- relative to where the script is (and make sure it ends in a trailing slash) $newdir = "../../new_folder/"; // JPEG quality is from 0-100 -- 90-95% will give you approximately your original filesize for the watermarked image $quality = 90; // Save the file to disk imagejpeg($image, "{$newdir}{$_GET[path]}", $quality); Make sure you place the script in the folder where your images are. Then call watermark.php?path=image.jpg The watermarked image will be saved in the folder that $newdir points to. You'll have to manually call each file through this script to get it watermarked.
-
Just use an absolute URL then. It'll never steer you wrong. Example: http://you.edu/~you/path/css/file.css Just use the full URL because it won't change.
-
If you just need to hit some sites in order to keep it from inactivity, just do this: <?php $a = file_get_contents("http://yoursite1.com"); echo "Site#1 is " . strlen($a) . " bytes long.<BR><BR>"; $b = file_get_contents("http://yoursite2.com"); echo "Site#2 is " . strlen($b) . " bytes long.<BR><BR>"; ?> You get the point on how to do this for multiple websites...
-
I believe you're saying you're putting the link to the CSS file in each page of your site. And some of these pages reside in a different folder, so the path to the CSS is wrong. If so, your URL just needs to be spruced up a little. From the root of your domain, trace the path. If CSS is a directory under the root directory, your code would be: <link href="/css/default.css" rel="stylesheet" type="text/css"> Notice the slash beginning the URL. That will make all the difference.
-
What exactly are you trying to do with the script? Just trying to run through a directory and save each image that has the watermark? If so, are you trying to replace the files that are there already, or save the watermarked images with a different name?
-
<?php echo webcopy("http://calvarybucyrus.org/CBC-50.jpg", "./"); function webcopy($source, $destdir) { $filename = $destdir . basename($source); $a = file_put_contents($filename, file_get_contents($source)); if ($a > 0) {return "{$a} bytes written to {$filename} from {$source}";} else {return "We had a problem with this query.";} } ?> This will preserve the original filename and is more similar to your code.
-
If you're using PHP5 and fopen wrappers enabled, try this: <?php $url = "http://calvarybucyrus.org/CBC-50.jpg"; $filename = "./savedfile.jpg"; $a = file_put_contents($filename, file_get_contents($url)); if ($a > 0) {echo "{$a} bytes written to {$filename} from {$url}";} ?> You could turn it into a function easily. It simply fetches a remote image and saves it locally.
-
No. It dynamically adds the watermark. The image it outputs isn't saved to disk. If you want it to, you'd need to modify the source code, which is fairly easy.
-
Something else to think about... You can set the length of the session by using a persistent session. This length is from the end of the last logged-in request. This will effectively log them off after xx minutes of inactivity. Note that the length before expiry is user-side. It's up to their browser to comply with the length of the cookie. <?php $length = 3600; // length in seconds of session --> 3600 seconds = 1 hour session_set_cookie_params($length, "/", ".yourdomain.com"); session_start(); //Start session // Your PHP code here ?> Be sure to replace yourdomain.com with your domain name.
-
$dr=preg_replace('/modify\.php.+/', '', $_SERVER['PHP_SELF']); $filename=str_replace($dr, './', $_SERVER['PATH_INFO']); Here's the problem: It's not getting a filename properly. If you absolutely don't want users downloading the file without the watermark, the images need to go in a folder that is not web-accessible. Then you need to format how the script will get a filename. You might use a query string from a hard-coded path. Example: Images in this folder: /images $directory = "/images/"; $filename = "{$directory}{$_SERVER[QUERY_STRING]}"; Image file: /images/test.jpg HTML code: http://yoursite.com/path/modify.php?test.jpg (Of course, you'd want to rename modify.php to something like image.php)
-
http://code-bin.homedns.org/62 This what you're looking for?
-
session_start(); --> Do this at the beginning of all your PHP pages. That's what gerkintrigg was saying. It should solve your problem immediately.
-
I have PHP5. File_put_contents must be a PHP5 command. Your problems are probably related to line breaks when you copy the code. If you forget just one of them (there may be extras at the end of the file), it won't match. But I see you got it working! That's great.
-
This is what you got (from your last post) and it matches mine. So you just have an old url.txt. Delete it and re-run the script, and you should be good to go.
-
That's very strange. Here's what I get: Delete your url.txt and re-run the script. Do you get what I get?
-
I tried this and it worked perfectly. This script will see if there were any changes --> http://code-bin.homedns.org/61
-
Your code needs to look something like this: $result = mysql_query("SHOW TABLE STATUS FROM table_name;"); while($array = mysql_fetch_array($result)) { print_r($array); // Will print information about each table stored in database } $result returns a resource. You need to use one of the mysql php functions to use it. BTW, I'm using InnoDB for my tables, and unfortunately it doesn't record a last update time.
-
My idea: 1. Get config.php file into string variable using file_get_contents 2. Perform a preg_replace on the string 3. Write the string to file using file_put_contents
-
http://www.getid3.org/ will retrieve all the ID3 information. Put this script in the root folder of the extracted download. <PRE> <?php $filename = "/path_to_mp3_file.mp3"; require_once('getid3\getid3.php'); $getID3 = new getID3; $fileinfo = $getID3->analyze($filename); echo "Bitrate: {$fileinfo[bitrate]} <HR>"; print_r($fileinfo); ?> </PRE>
-
Put this on the new page. <?php $news_link = "http://yoursite.com/link_to_your_news.php"; // This function reads all available news function getNewsList(){ $fileList = array(); // Open the actual directory if ($handle = opendir("news")) { // Read all file from the actual directory while ($file = readdir($handle)) { if (!is_dir($file)) { $fileList[] = $file; } } } rsort($fileList); return $fileList; } ?> <?php $list = getNewsList(); echo "<table>\n"; // Do your output however you like $newsData = file("news/" . $list[0]); $newsTitle = $newsData[0]; $submitDate = $newsData[1]; unset ($newsData['0']); unset ($newsData['1']); $newsContent = ""; foreach ($newsData as $value) { $newsContent .= $value; } echo "<tr><th align='left'>$newsTitle</th><th align='right'>$submitDate</th></tr>"; echo "<tr><td colspan='2'><br>".$newsContent."<br/><br><hr size='1'/><br></td></tr>"; echo "<tr><td colspan='2'>See more news at: {$news_link}</td></tr>"; echo "</table>\n"; ?>
-
As for the blank entries, replace the code below: // Output your records for this page echo "<table>\n"; for ($s = $start_key; $s <= $end_key; $s++) { if (file_exists("news/" . $list[$s])) { // Do your output however you like $newsData = file("news/" . $list[$s]); $newsTitle = $newsData[0]; $submitDate = $newsData[1]; unset ($newsData['0']); unset ($newsData['1']); $newsContent = ""; foreach ($newsData as $value) { $newsContent .= $value; } echo "<tr><th align='left'>$newsTitle</th><th align='right'>$submitDate</th></tr>"; echo "<tr><td colspan='2'><br>".$newsContent."<br/><br><hr size='1'/><br></td></tr>"; } } echo "</table>\n";
-
Maybe you forgot the mp3 extension on the filename??? upload/PhilCollins-AnotherDayInParadise
-
Just put the // Print Pagination section under the article display and put echo "<center>"; // Print pagination section goes here echo "</center>";