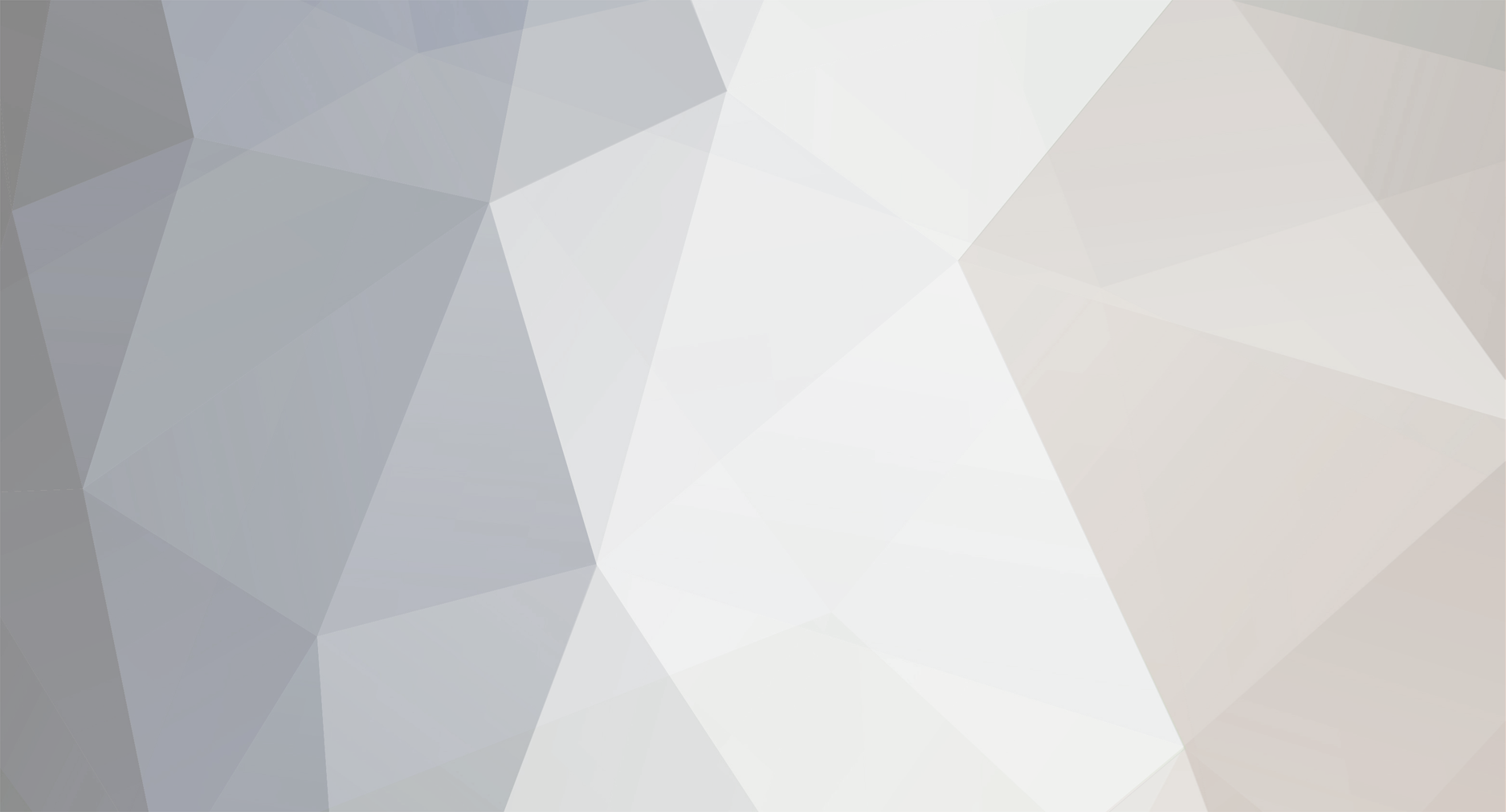
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
[Beginner Question] accesing outer variables inside a class
KevinM1 replied to elnaz1010's topic in PHP Coding Help
Simply pass the outer variable to the object via a function: class Person { private $name; public function setName($name) { $this->name = $name; } public function getName() { return $this->name; } } $test = "test" $person = new Person(); $person->setName($test); echo $person->getName(); -
A couple things - This: $s =& $session; Isn't necessary. PHP 5 automatically uses references when dealing with object assignment. Also, do you realize why using the 'global' keyword is, as wildteen said, a dirty fix? I actually strongly suggest you pass your session object to the function through its argument list.
-
Also, do you have your own array named $request, or are you trying to use the superglobal array $_REQUEST?
-
I think I speak for everyone here when I say I don't know what you're asking. A better explanation of what you're trying to do, with relevant context, may make it easier for you to get help.
-
Not only that, but what are you trying to click? Showing us two JavaScript function definitions without also showing where/how they're invoked isn't helpful.
-
Well, that's why I'm here.
-
Ah, okay. Yeah, the language barrier can make things a bit more difficult to get through. You're going to have 5 tables in total: The user/character table, which contains fields that relate to what a user/character should be. So, this would include their name, hit points, and/or whatever else you need. The items table, which will contain fields that describe the attributes of the items in your game. Again, things like the item's name, its value, how much it would cost in a store, and/or other attributes. The items-to-user/character table. This is a very simple table. Ideally, you'd have three fields - the primary key/id for each row, the user/character id, and the item id. With this table, you map characters to items (and vice versa) via their ids. A couple of example rows: ItemsToUserId UserId ItemId 0 45 55 1 45 98 2 45 2 3 23 55 4 23 49 5 52 9 6 77 37 User 45 has items with the ids of 55, 98, and 02. User 23 has items 55 and 49. I hope this makes sense. A table for abilities. This should be straightforward. A table that maps abilities to users/characters, which should essentially be the same as the ItemsToUsers table. So, with this sort of setup, instead of adding fields to your existing tables when a user gets a new item or skill, you add records (rows of data) to these new tables that exist solely for the purpose of maintaining these relationships. It keeps your data intact and your table structure sound while giving you the flexibility to add/edit/remove skills and items. This is, in fact, why MySQL is called a relational database.
-
Don't add fields, add records. Every item should have a uniform structure, correct? Something like name, cost, value, etc. Make one table with that, and populate it with all your items. Now, make another table. This one will link the character to their items. How? By matching item ids with character ids. Why? Because the relationship is many-to-many. Many different characters can own the same items. Many items can be owned by the same character. Do the same thing for abilities - one table that contains ability data, and another that links characters to them. Also, reread the article. If you go through the normal forms, you'll see these kinds of relationships occur naturally as you go through the progress.
-
Yeah, it should work out of the box. I guess the only thing left to do is create a small test script and slowly add the functionality you want to it to see where it breaks. See if you can get a function to execute after page load, then see if it can perform the AJAX-y stuff you want, etc. Dumb question - you are attempting to invoke your function within $(document).ready() or at the end of the HTML, right? Sorry i'm fairly retarded when it comes to Javascript >< I thought that I could invoke the function in the body section of the html? Is this wrong? I thought before when I echoed it in php I didn't need anything else but the javascript function. There are generally two '100% safe' places/ways to get JavaScript to run immediately after page load: 1. In the head element, with all executing code inside of the window.onload() event, or, in jQuery's case, inside of $(document).ready(). In other words: <!DOCTYPE html> <html> <head> <script type="text/javascript"> window.onload = function() { // all executable code goes here } </script> </head> <body> </body> </html> OR <!DOCTYPE html> <html> <head> <script type="text/javascript" src="where/you/have/your/jQuery.js"></script> <script type="text/javascript"> $(document).ready() { // all executable code goes here } </script> </head> <body> </body> </html> 2. At the very end of the HTML, like so: <!DOCTYPE html> <html> <head> <!-- if you need a title, css, whatever --> </head> <body> </body> <script type="text/javascript"> // executable code goes here </script> </html> OR <!DOCTYPE html> <html> <head> <script type="text/javascript" src="where/you/have/your/jQuery.js"></script> </head> <body> </body> <script type="text/javascript"> // executable code </script> </html> The reason for this is that JavaScript tends to get loaded, and thus tends to execute, before the HTML is fully rendered. This, in turn, leads to the script attempting to access elements that literally don't exist when the script runs, resulting in errors. You can put script elements within the body of your document, but there's no guarantee you'll be able to access the elements that occur after those script blocks. That's why it's best to wait until the entire HTML document is loaded before attempting to execute code, or otherwise obtain references to HTML elements. Regarding method 1 vs. 2, 2 is a bit easier/less cluttered to write. It may also be a bit faster, as you don't need to wait for an event to fire and execute an event handler to get your code to work. This sort of runtime error may not be the cause of your problem, but you should always keep it in mind when writing JS. It's the cause of a lot of headaches.
-
Yeah, it should work out of the box. I guess the only thing left to do is create a small test script and slowly add the functionality you want to it to see where it breaks. See if you can get a function to execute after page load, then see if it can perform the AJAX-y stuff you want, etc. Dumb question - you are attempting to invoke your function within $(document).ready() or at the end of the HTML, right?
-
Sounds like you didn't really spend enough time designing your game's data storage and representation. Ideally, something like this would be split into multiple tables, and maybe even some XML files. If you normalize your DB scheme, you can easily map player characters to skills and items (and vice versa). Here's a link to get you started: http://dev.mysql.com/tech-resources/articles/intro-to-normalization.html I find that game design is a bit easier if you use OOP. Many in-game entities (items, weapons, spells/attacks/abilities, the characters and enemies themselves, etc.) map naturally to objects, and from there, to db tables. It just seems clearer that way, but YMMV. Just throwing it out there.
-
Do you have a question or problem that needs to be addressed?
-
An extension for Firefox that is incredibly useful for debugging JavaScript (among other things). No serious developer should be without it. Link: https://addons.mozilla.org/en-US/firefox/addon/1843 My jQuery is a bit rusty, but shouldn't 'async' be set to true? EDIT: the function may not like the way you're passing the element to it... try doing it the vanilla JS way, like: var systemName = document.getElementById('systemName'); systemName.selectedIndex = 0; updateForm(systemName);
-
That's what I thought but I am using it like this <script type='text/javascript'> document.getElementById('systemName').selectedIndex=0; updateForm($('#systemName')); </script> It properly sets the selected index and then it's supposed to update the form. I know the function works the way it's supposed to because i have it in the onclick event as well. It just isn't working like this however. Are you getting any Firebug errors? What should be updated when the form executes correctly?
-
Sure you could, if you don't require the function firing when the user does some action.
-
Why on earth are you using global? Use the argument list! That's what its there for!
-
My first time OOP and I don't even know where to start
KevinM1 replied to jmayoff's topic in Application Design
IMO, user creation should be separate from a user class. It just has the potential to get too complicated if a user class is designed to both create users and model an individual user's info. Remember two things - the currency of OOP is the OBJECT, and K.I.S.S. It's okay (and more often than not, preferred) to have many small, singular purpose objects than large, complicated objects that try to do too much. Similarly, why should a user be bothered with form creation and validation? How does form handling tie into what a user is, logicality speaking? (hint: it doesn't) Some questions you should answer: What's the difference between a user and a client or a freelancer? How would you represent these differences in code? What's required to create a user, client, or freelancer? How flexible does your form validation need to be? What inputs are you expecting to use? What does 'valid' mean in those cases? Answering these questions will inform your design. They may not bring you to the most efficient or elegant solution, but it will get you started. -
Looking at your site won't tell us much. We need to actually see the PHP, which isn't visible in the HTML source code. Whoever told you this is a moron. If you see/hear from them again, tell them such. PHP is a server side scripting language. HTML is a markup language that cannot perform any logic. PHP can write HTML, but it has no similarity to HTML at all.
-
And therein lies the joke....
-
You're attempting to use $session as though it's an object. PHP is telling you it isn't an object. What is $session supposed to be? Also, never use global variables. Functions and methods have argument lists for a reason, one of which is ensuring that the parameters that are passed in and used in the function/method actually exist. Does $session and/or $form actually exist at the time when you attempt to use them?
-
how do i write mysql_real_escape_string in PHP ODBC
KevinM1 replied to worldcomingtoanend's topic in PHP Coding Help
If ODBC's prepared statements work like other db's, use that instead. -
Your first function seems to have some scope and default value issues. Try the following: function toEntity(str) { var return_str = ''; for(i = 0; i < str.length; ++i) { if (str.charCodeAt(i) > 127) { return_str += '&#' + str.charCodeAt(i) + ';'; } else { return_str += str.charAt(i); } } return return_str; } The reason being you already pass the str variable to the function...using the 'var' keyword in front of it designates a new str variable in local scope. Also, it's a good idea to initialize a variable to an empty string before concatenating other string data to it. If JavaScript behaves like some other languages, you're not guaranteed that the variable will be empty. Hopefully that will help somewhat. I'd look at your overall logic, too. If I'm reading things right, you're invoking toEntity on both the name and value fields of your JSON objects created by serializeArray. You may not want to do that.
-
Actually, my solution was based on the name of the ability attempting to be invoked. That way, each race and class could have a multitude of special abilities. This solution works well in ASP.NET/C# - which is what I'm constructing my game with - as each button can have a CommandName attribute. This attribute can be retrieved during an onclick event so the proper ability is invoked. Something similar can happen in PHP, with each button's name attribute mapped to a specific attack. My setup is essentially: Iterate through the PC's list of attacks, creating a button for each one with a CommandName matching that particular attack. | | V Create the field of buttons with that info | | V When clicked, have the page post back to itself. The results of the action depend on the name of the button that is clicked. Results are echoed out to a div that contains the combat info. It works pretty well.