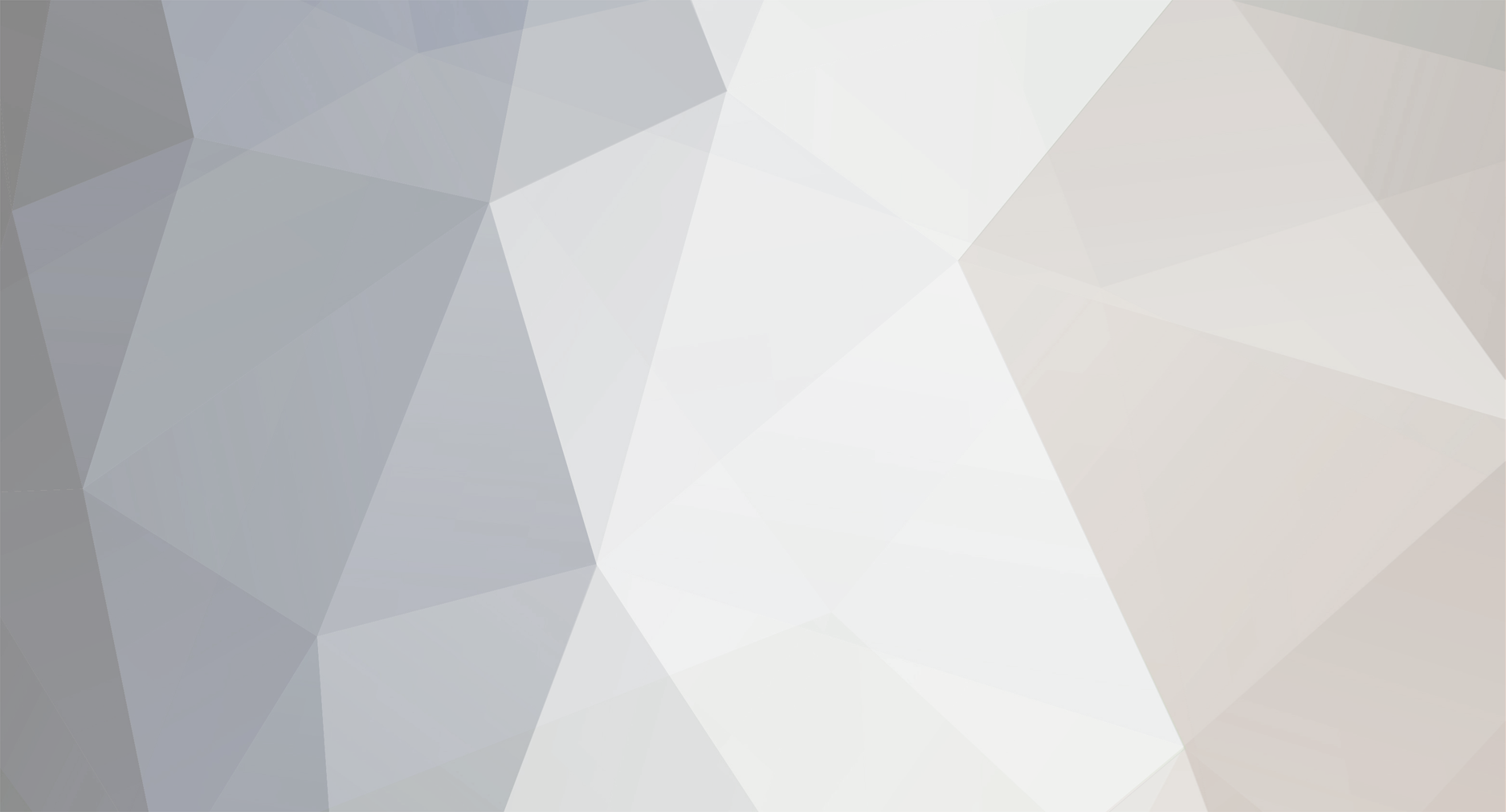
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
After some tests, it looks like innerHTML transforms the closing tag of the line break element from this: <br /> To this: <br> Automatically. So, if you're testing for the XHTML style element, the conditional will fail.
-
Try: <?php session_start(); include("inc/inc-dbconnection.php"); include("inc/inc-online.php"); include("inc/inc-functions.php"); if (isset($_POST['submitLogin'])) { // POST vars $user = $_POST['user']; $pass = $_POST['pass']; // Errors array() $errors = array(); $errMsg = ''; // Potential errors // Empty fields if (empty($user) || empty($pass)) { $errors[] = "You never filled in all the fields below."; } // Does user exist? $qU = "SELECT * FROM fso_users WHERE user_email = '$user' AND user_password = '$pass' LIMIT 1"; $rU = mysql_query($qU); if (mysql_num_rows($rU) < 1) { $errors[] = "We don't recognise those login details, have you typed them correctly?"; } // Count the errors and display if (count($errors) > 0) { errMsg .= '<div id="error">'; foreach($errors as $error) { errMsg .= "<b>$error</b><br />"; } errMsg .= "</div>"; } else { // Update the login timer and redirect $timer = mysql_query("UPDATE fso_users SET user_last_login = NOW() WHERE user_email = '$user' AND user_password = '$pass'"); // Array() $aU = mysql_fetch_assoc($rU); // $_SESSION['']; $_SESSION['loggedIn'] = 1; $_SESSION['user_id'] = $aU['user_id']; // Lastly redirect to the account page header("Location: account.php"); } } ?> <?php include("inc/inc-header.php"); ?> <div class="fcp-main-content-area"> <?php if ($errMsg != '') { echo $errMsg; } ?> <div class="fcp-breadcrumb"> <ul> <li><a href="index.php">Home</a></li> >> <li><a href="javascript:history.go(-1)">Previous Page</a></li> >> <li>Login in to your account.</li> </ul> </div> <div id="div-regForm"> <div class="form-title">Log In</div> <div class="form-sub-title">Login & see your points score!</div> <form id="regForm" action="login.php" method="post"> <table> <tbody> <tr> <td><label for="fname">Email:</label></td> <td><div class="input-container"><input name="user" id="user" type="text" /></div></td> </tr> <tr> <td><label for="lname">Password:</label></td> <td><div class="input-container"><input name="pass" id="pass" type="text" /></div></td> </tr> <tr> <td> </td> <td><input type="submit" class="greenButton" name="submitLogin" value="Login" /> </td> </tr> </tbody> </table> </form> </div> <?php include("inc/inc-footer.php"); ?> To reiterate - the consensus on what proper PHP structure is has form processing happening before form display. It's done this way due to the nature of the HTTP request cycle and the fact that PHP is essentially a fire-once language. Once a script is run, nothing else is happening in the background. It also helps remove those pesky "Headers already sent" errors, makes it easier to edit/maintain scripts, and is a smart way to structure code regardless as it separates concerns. This code isn't tested, but it should give you a better idea of the approach to take, while hopefully fixing the errors you have.
-
Once again, the sticky topic that describes the "Headers already sent" error and how to fix it: http://www.phpfreaks.com/forums/index.php/topic,37442.0.html
-
. is the string concatenation operator. .= is a shorthand operator. The following statements are equivalent: $var1 = $var1 . " and something added at the end."; $var1 .= " and something added at the end.";
-
Try taking out the ` marks.
-
Your logic is backwards. Always put form processing before displaying the form.
-
Besides having wrong syntax (Lacking a "}" at the end) what exactly is the reason you do not like that or is wrong to do in programming? The complete lack of indentation doesn't strike you as being hideous?
-
Browsers use different event models. This is why cross-browser errors tend to crop up. In order to ensure your code works well in all browsers, you'll need to equalize the event models: function functionName(evt) { evt = (evt) || window.event; // function processing here } This is one of the reasons why people use frameworks - they equalize the event models out of the box. Also, just for your own sanity, and for those of us who you ask to look through your code, you should really move your CSS to an external file. It's very difficult reading your code with a bunch of inline stylesheets in every element.
-
Or, have the following link bookmarked: http://www.php.net/quickref.php
-
It's JavaScript code to toggle the visibility of an element. The .style.display portion of the code refers to that element's CSS display attribute. The code literally says On a click of that particular hyperlink If the target element's display attribute value is set to 'none' Set it to 'block' to make it visible Else, it's already visible So set the target element's display attribute value to 'none' to hide it again For the rest, let's see... Return false is there to stop the hyperlink from attempting to bring the user to another page. The coder simply wants the link to act like a toggle button rather than a real hyperlink. Returning false stops the link's default behavior. The 'this' represents that particular element, in this case, that hyperlink. The toggle function apparently requires a reference to the actual link that invoked it, most likely because the page has several such links that toggle the visibility of elements, and it's necessary for the page to keep track of them. Hope this helps.
-
Remember that color codes are strings. Put quotes around them (onmouseover=set_colours("#FFFFFF")).
-
I think you're getting the wrong idea of what's actually going on. The constructor creates an object. If you don't specify an explicit constructor, a default parameterless constructor is still invoked. As far as I know, the order of how the arguments are passed to the constructor doesn't matter. Constructors, in general, serve two purposes: 1. Actual object construction 2. Initial object initialization - that is, supplying initial values for its data members In your question, using my example with the two properties, nothing would happen with the third argument passed into the constructor as the constructor itself doesn't have any code to handle a third argument. In fact, you may get an error, since the constructor's signature only contains two parameters. The best way to add another parameter to a class is to redefine the class itself, and code it into the definition. The 'this' refers to the context of which object is currently being accessed, and nothing more. It allows us to have many different instances of a class, all sharing the same structure (which is what the class provides) while having different values. It's what allows us to have one class that can have derived objects represent both Forrest Gump and Lt. Dan.
-
Absolute path for images and how it affects load time...
KevinM1 replied to webmaster1's topic in PHP Coding Help
Just tested it. I'm right. See here, and look at the source: http://www.helgaseibert.com/test/blah.html EDIT: the second attempt - without the leading slash - looks for closets.jpg within the test folder. It doesn't exist, so it displays the image's alt attribute value instead. Are you familiar with *nix file systems? Most servers run on an Apache server on a *nix system. The way paths work is exactly the same as the *nix file system because, well, that's what you're using under the hood. -
Absolute path for images and how it affects load time...
KevinM1 replied to webmaster1's topic in PHP Coding Help
foo/img.jpg - path is relative to the directory of the content the user is looking at. I.e., if you have a page at the following path: http://mydomain.com/news/today.html foo/img.jpg would actually be the path of http://mydomain.com/news/foo/img.jpg /foo/img.jpg - path is relative to the web root. All instances of this are actually http://mydomain.com/foo/img.jpg -
You need to echo the $id variable. I.e.: <li><a href="prueba.php?id=<?php echo $id; ?>"><img src="images/menu.gif" align="middle" alt="menu" width="100%" height="22" /></a></li>
-
That's correct. Like I said earlier, you only declare three (I originally said four...miscounted) non-static properties: id, username, and password. Your instantiate method receives a database row as an argument, and iterates over it, checking to see if your object has a property with the same name as the current column name you're at at that point of the loop. If so, it assigns the value that's stored in that db column into the variable. If not, it does nothing. So, since you didn't declare '$exp' in your object, you get an error when you try to access it since it doesn't exist within your object. Factories are ridiculously easy to implement. You can make them entirely abstract, as there often isn't a reason to actually have an instance of one. Instead, you can rely on static methods to handle everything. A quick example: abstract class UserFactory { private static $dbc = /* whatever database object you'll use */; private static $tableName = "cf_users"; public static function findById($id = 0) { $result = self::findBySql("SELECT * FROM " . self::$tableName. " WHERE id = $id LIMIT 1"); return $result; } public static function findBySql($sql = '') { $users = array(); $result = $dbc->query($sql); while($row = $dbc->fetchArray($result)) { $users[] = self::instantiate($row); } return $users; } private static function instantiate($record) { $user = new User($record[0], $record[1], $record[2] /* until all the properties are filled */ ); return $user; } } class User { private $id; //may not be necessary if you only use it in db queries private $userName; private $password; //ditto /* continue declaring all of the property fields a User object should have */ public function __construct($id, $userName, $password /* etc */ ) { $this->id = $id; $this->userName = $userName; $this->password = $password; /* etc */ } } $singleUser = UserFactory::findById(24); $multipleUsers = UserFactory::findBySql("SELECT * FROM cf_users"); Instead of hard coding the database information into the factory, you could have the class obtain that info by reading it from a config file or by accessing an application registry.
-
I'm suicidal over Endor. I want a pet midget in a Teddy Ruxpin suit.
-
You're asking if the OBJECT has an attribute with the same name as one of your db column names. You only declare four non-static attributes. If your object doesn't have one of the attributes you're looking for, it doesn't magically get added to your object. Therefore, you're attempting to access something that doesn't exist. You may want to rethink your design. IMO, a user object shouldn't populate itself. That should be the job of an abstract user factory class, which would create and populate a user object through static methods much like what you already have. That would allow the user class to only be concerned with storing individual user data and manipulating that data.
-
Ack, yes, my bad. D'oh!
-
Actually, 'this' isn't in ANSI C, as the language has no concept of a class or object. For the OP, 'this' is an instance variable. It literally means the current instance of an existing class variable. Example: class ExampleClass { private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function getName() { return $this->name; } public function getAge() { return $this->age; } } $person1 = new ExampleClass("Forrest Gump", 34); $person2 = new ExampleClass("Lt. Dan", 39); echo "Person1's name is " . $person1.getName(); echo "Person2's age is " . $person2.getAge(); As you can see, 'this' is contextual. Its value depends on which instance of the class is being accessed.
-
Yes, your syntax is wrong. Mchl gave you the solution.
-
What do you mean by 'one dictionary'? One language across a page or one DOCTYPE across a site? I don't understand. Pick a doctype to use, code according to its rules, and use the validator to verily that your code adheres to that doctype's rules. If you're extending or editing code that conforms to a particular doctype, write your code accordingly. If you want to keep it simple, from what I've read HTML 5's doctype is sufficient to ensure the browser doesn't render the page in quirks mode. That doctype is simply <!DOCTYPE html> Some caveats - there's no way to validate your HTML's well-formedness using this doctype. HTML 5 is still in the planning stages, so this doctype declaration is essentially a hack. So, you shouldn't use it if you don't feel comfortable or confident in your ability to write markup correctly the first time.
-
how can I apply more than one function to a variable?
KevinM1 replied to co.ador's topic in PHP Coding Help
Your _getValue() method is gibberish, and is the cause of your error. It doesn't grab the value of the field (I have no idea why you thought putting the 'global' keyword there would do that). Instead, it puts the value of the variable named $field that resides in the global scope in the local $field variable. Since there's likely no such variable in the global scope, you're getting passed a null string, which would make your test fail. For more information: http://us.php.net/manual/en/language.variables.scope.php -
how can I apply more than one function to a variable?
KevinM1 replied to co.ador's topic in PHP Coding Help
Well, just chain your validation functions together, like I already suggested: $email = emailValidator(requiredValidator(lengthValidator($_POST['email'], 50))); Or, design your function to smartly handle all three requirements at once: function emailValidator($field, $maxLen, $req) { if (empty($field) && $req) { // error, field is required } if (strlen($field) > $maxLen) { // error, field value is longer than the max length allowed } //other email validation code (regEx) } $email = emailValidator($_POST['email'], 50, true); The first option is more flexible, as you can reuse the length and required functions on other form fields. Or, if you're comfortable with OOP, you could use the Strategy pattern to help you out (example: http://www.phpfreaks.com/forums/index.php/topic,188111.msg845281.html#msg845281). -
That's all you need to understand. The problem is with the text in the alert box - it does not correspond to the field in the array. It will always alert the last message in the array, because each element's onclick action is binded with alert(ar[ i ]), which is variable, and not alert("text for field*id*"), which is a simple string and which is what i want. So what's actually being displayed in the alert? ar['field3']? And is this the case for all the elements?