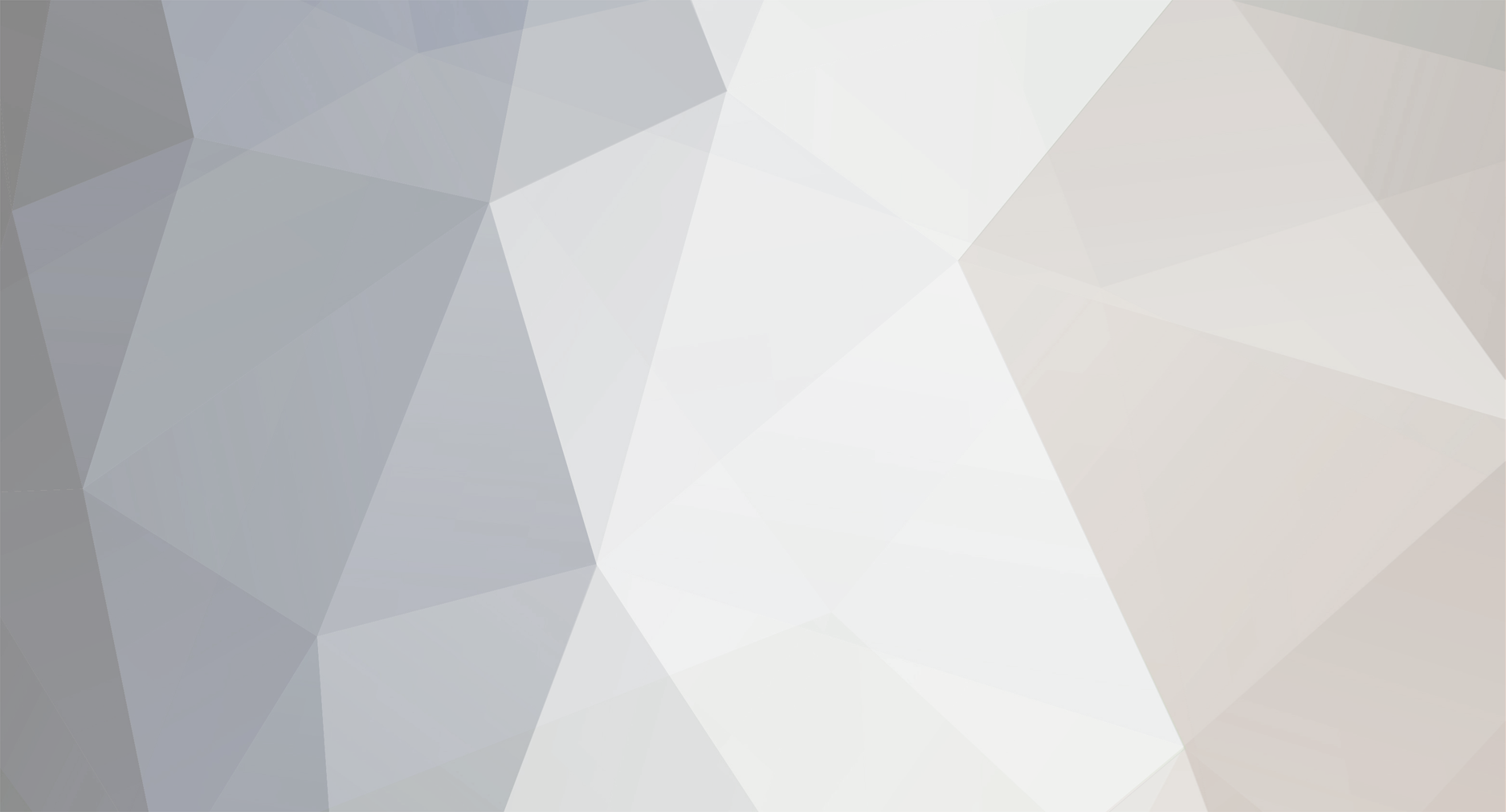
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
The following works for me: <html> <head> <title>Table row count</title> </head> <body> <table id="myTable"> <?php for($row = 0; $row < 4; ++$row) { echo "<tr>"; for($col = 0; $col < 4; ++$col) { echo "<td>$row-$col</td>"; } echo "</tr>"; } ?> </table> <button id="myButton">Click to count rows</button> </body> <script type="text/javascript"> var oButton = document.getElementById('myButton'); var oTable = document.getElementById('myTable'); var numRows = oTable.getElementsByTagName('tr').length; oButton.onclick = function() { alert("Number of table rows: " + numRows); } </script> </head>
-
Exactly right. What does: $API_UserName = "seller_1256141718_biz_api1.hotmail.com"; $API_Password = "1256141861"; function beees($userName, $userPass) { echo $userName; echo $userPass; echo "1234567<br><br>"; } echo "1 $API_UserName<br><br>"; beees($API_UserName, $API_Password); echo "3 $API_UserName<br><br>"; die(); Give you?
-
To get a hold of a form's values, the input name needs to be in quotes. Example: $firstValue = $_POST['val1']; Also, you have a couple of typos where you have two ending brackets (]]) at the end of your post values.
-
Why does it have to be either a 1 or 0? Why not if($successChance >= $target) { //success } else { //failure } Where $target is the finalized (meaning tweaked so that for large point differences it wouldn't be nearly impossible to fail) target percentage that would grant a success on the play? To me, the simplest thing to do would be to randomly pick a number between 1 and 100, and test it against the percentage derived from the current game's score. It keeps the random element of the play, and accurately follows the probability of success based on the score differential.
-
Instead of creating a huge array, why not deal with percentages? Sports logic dictates that the team in the lead has the advantage. This advantage increases the larger the score gets. So why not simply use the percentage of points (out of the total number) scored by the leading team to help determine success or failure? That should save you from needing to deal with huge arrays. A couple of syntax things I noticed: 1. Your scores are strings, not integers. They may not behave the way you want them to (I don't know if automatic conversion will take place if both operands are strings). 2. Your for-loop uses irregular syntax. It's normally: for($t1 = 1; $t1 <= $team1_complete_total; $t1++) { array_push($passage_success_or_fail_array, 1); }
-
$fullName = $firstName . " " . $lastName;
-
To get back to the OP, you could have something like: class UserManager { private $sessionData; private $userList; public function __construct($sessionArray) { //do any security processing to ensure that the session data hasn't been compromised $this->sessionData = $sessionArray; } public function validateUser() { $userId = $this->sessionData['user_id']; //access the db, which may also be an object if(/* everything checks out */) { $legitUser = new User($ip_address, $session_id, $user_agent, $activity); $this->addUser($legitUser); return true; } else { return false; } } private function addUser(User $user) { $this->userList[$user->getSessId()] = $user; } public function getUser(User $user) //could also write this to accept the session id as an argument... it'd look cleaner that way { if($this->userList[$user->getSessId()]) { return $this->userList[$user->getSessId()]; } else { //error } } } class User { private $ipAddress; private $sessId; private $userAgent; private $activity; public function __construct($ipAddress, $sessId, $userAgent, $activity) { $this->ipAddress = $ipAddress; $this->sessId = $sessId; $this->userAgent = $userAgent; $this->activity = $activity; } public function getSessId() { return $this->sessId; } //other similar functions } One of the key concepts behind OOP is that objects (like my hastily written UserManager) can contain other objects (Users). That tends to be the core concept that many have difficulty grasping, so I figured I'd give you a taste of that early. This was just a very rough idea of what you could do. Your best bet is to start at the beginning and slowly work your way up to being able to build and manipulate objects yourself.
-
I admit I thought about whether or not to hit 'Post' after writing that. Ultimately, I feel it needed to be said. I have no problem with people putting library code in classes. I don't necessarily agree with the practice, but if it works for the coder in question, then they should go for it. What I do take exception to is someone trying to tell a beginner that stuffing functions related by theme into classes is OOP. It is not, and it gives the beginner the wrong idea of what OOP is and the point behind it. Writing reusable code and understanding the separation of concerns - which is essentially all that ialsoagree described - is not OOP in and of itself. Was my remark conceited? Probably. But I find it to be accurate as well. Fake edit: I wasn't addressing ialsoagree's opinion on OOP. I could care less if they loathe it or think it's the bee's knees. I was merely making an observation based on their stated misunderstanding on what OOP actually is.
-
These conversations go down this road simply because people who don't fully grasp OOP but think they do attempt to tell those who actually know what they're doing that they're wrong.
-
You simply need to put the filepath of the second page as the first page's form's action attribute, and grab a hold of the correct form inputs. Simple example: page1.html: <html> <head> <title>Something</title> </head> <body> <form name="myForm" action="page2.php" method="post"> Name: <input name="userName" type="text" /><br /> Password: <input name="userPass" type="password" /><br /> <input name="submit" type="submit" value="submit" /> </form> </body> </html> page2.php - <?php if(isset($_POST['submit'])) { $user = $_POST['userName']; $pass = $_POST['userPass']; //continue processing } else { //form wasn't submitted...handle this error } ?>
-
Agreed, unless you're: In which case it's not really OO since you're not using objects, but it employs the same methodology. That's called creating library code. Again, there's more to OOP than migrating code to a group of similarly themed functions and including them in the global scope.
-
Merely stuffing code into collections of functions != OOP.
-
Also, I think it's difficult to attempt to translate code from procedural to OOP as your first attempt. There's often an implicit assumption that beginners have where they think there's a 1:1 correlation between the two styles of coding. There's not. Your best bet is to start at the beginning. Learn the basics - syntax, how to combine objects, how to extend them, etc.
-
The error means what it says - $adHTML is undefined and you try to concatenate a value to it. This doesn't make any sense, logically. Define $adHTML as an empty string (''), then try adding to it.
-
I agree with Daniel - Google Wave is not immediately very useful. It's in the midst of a rough beta phase, currently. It slows down to a crawl after 30 or so messages. It's not surprising - every message can contain a wide variety of media, be edited or replied to in place, and all interaction done by one member of the wave is immediately broadcast to all other members. All using JavaScript. It's a bit amusing to see how well people can type in real time. On top of all that, the UI isn't very friendly, messages don't indent the way you'd expect them to, and there's at least one gaping security flaw (JavaScript injection). I still think there's potential here, but it won't be realized anytime soon. There are too many bugs, performance issues, and security flaws needed to be addressed to consider Wave a viable technology for the masses. I expect it'll take at least a year to make it usable. Probably longer. Until then, it's a fancy tech demo.
-
True, but I like getting that look from people. It's incredibly fun behaving in a way that goes against the stereotype. My college career - aside from my studies and homework - was an exercise in trying to get a rise out of random people. It was a damn funny portion of my life. hmm...so if you are saying that your response is not the (stereo)typical response, what is the (stereo)typical response? In regards to the Bible pusher, either a polite "No, thanks" or wordlessly taking the Bible and most likely tossing it away, or otherwise conveniently losing it somewhere (New England isn't the most religious area of the country). For more general things, it's hard to say as it depends on the context of the situation.
-
True, but I like getting that look from people. It's incredibly fun behaving in a way that goes against the stereotype. My college career - aside from my studies and homework - was an exercise in trying to get a rise out of random people. It was a damn funny portion of my life.
-
please help, im not grasping a basic concept so well?
KevinM1 replied to crashgordon's topic in Javascript Help
Well, stuffing things into variables can be more efficient in some cases, the most frequent being when you need to grab a hold of an HTML element. A lot of people code in the style of: function someFunc() { var myVar = document.getElementById('someElem').value + 5; var myVar2 = document.getElementById('someElem').style.border; if(myVar2 == "1px solid black") { document.getElementById('someElem').style.border = ""; } } Multiple document calls to find the same element, which is horribly inefficient. So, using variables wisely will make the code better overall: function someFunc() { var elem = document.getElementById('someElem'); var newValue = elem.value + 5; var elemBorder = elem.style.border; if(elemBorder == "1px solid black") { elemBorder = ""; } } Why is this more efficient? It's due to the fact that JavaScript makes heavy use of references, and it's far more efficient to put a reference to an element in a variable (elem, in this case) and manipulate it through that reference than it is to tell JavaScript to search through the HTML document and retrieve the same reference repeatedly. In other words, my second example essentially caches the result of the document search, and uses that to work. So, with that canned example out of the way, I can't really comment on your code specifically without seeing it. -
please help, im not grasping a basic concept so well?
KevinM1 replied to crashgordon's topic in Javascript Help
Quotes (single and double) denote strings, or groups of characters that are supposed to be processed as-is. T_hit isn't true or false because of this...it is literally im_dis.y <= cropArea.y. You should have: var T_hit = im_dis.y <= cropArea.y; function findImageEdge() { if(T_hit == true) { TPos.setOpacity(1); } else { TPos.setOpacity(0.5); } } An even simpler version would be: function findImageEdge() { if(im_dis.y <= cropArea.y) { TPos.setOpacity(1); } else { TPos.setOpacity(0.5); } } -
<-- atheist. I don't generally have a problem with people who are spiritual. I consider them delusional, but mostly harmless (notable exception being fundamentalists). I do have problems with the idea of organized religion. It's just absurdly illogical, and more than a bit creepy IMO. I do take joy in needling believers now and then. I love this story, so I figure I might as well share, if I haven't already: When I was in college/university, there would always be a group of local Bible pushers hanging around campus at the start of each semester. Most were nice, but some were annoying. Being the egotistical, sarcastic jerk that I am, I decided to screw with one of them on my way to class. Now, to get the full impact of the joke, you have to understand that I'm physically disabled and use an electric wheelchair to get around. So, I'm on my way to class and one of the pushers standing on the sidewalk asks, "Do you want a Bible?" I reply, "No thanks. Your god broke my legs" and continue to drive on. The look on the kid's face was priceless.
-
[SOLVED] Dynamic content does not show up in IE (what else is new?)
KevinM1 replied to jordanwb's topic in Javascript Help
If you're accessing by dev server (http://99.224.34.94) then yeah it'll be a little slow. Judging by what I can see, it looks like it's functioning properly for both IE7 and IE8. -
[SOLVED] Dynamic content does not show up in IE (what else is new?)
KevinM1 replied to jordanwb's topic in Javascript Help
Well, it looks like you got it working, at least in IE8 (I was about to copy some of your code so I could run a test of my own when I noticed the changes). One problem remains, however - the help window takes forever to load when clicking on the '?'. I'm not 100% sure what's causing the slowdown. Also, you don't need the 'javascript: void(0)' bits. It's easier/cleaner to put a hash (#) as the value of the href attribute and/or have the event handler return false. Example: <html> <head> <title>Blah</title> </head> <body> <a id="link1" href="http://google.com">Google</a> <br> <a id="link2" href="#">Someplace else</a> </body> <script type="text/javascript"> var oLink1 = document.getElementById('link1'); var oLink2 = document.getElementById('link2'); oLink1.onclick = function() { alert("First link has been clicked"); return false; } oLink2.onclick = function() { alert("Second link has been clicked"); } </script> </html> -
[SOLVED] Dynamic content does not show up in IE (what else is new?)
KevinM1 replied to jordanwb's topic in Javascript Help
Instead of trying to set those onclick functions as an attribute of the links, can you try the following? mount_type_help_link.onclick = ShowMountHelp; -
[SOLVED] Dynamic content does not show up in IE (what else is new?)
KevinM1 replied to jordanwb's topic in Javascript Help
I just figure that with all of the other oddities JavaScript contains, it's better to be safe than sorry and always put the opening brace on the right. FWIW, I do code with the opening brace to the left in other languages. I think it makes things easier to read, but I digress.