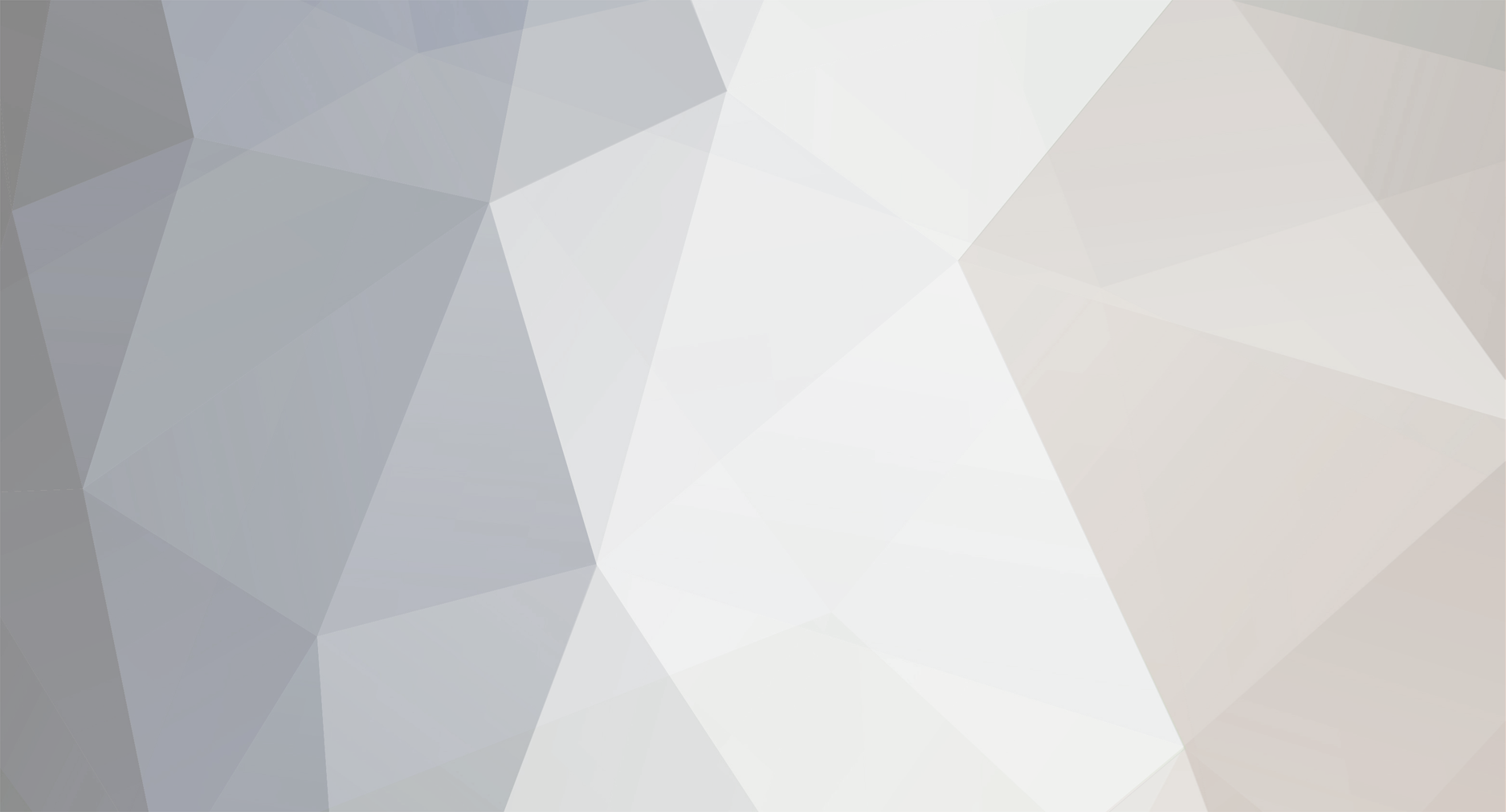
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
The following line: if (document.main_form.number_of_owners.value = -1) { Should be: if (document.main_form.number_of_owners.value == -1){ Note the double equal signs which designate a test for equality. Also, you're better of caching your values. In other words, instead of writing out long bits like document.main_form.number_of_values... Stick them in a variable. So, for your validateForm function, something like: function validateForm(){ var errors = []; var ownerName = document.main_form.owner_name.value; var numOwners = document.main_form.number_of_owners; if (numOwners.value == -1){ errors[errors.length] = "New Error"; numOwners.style.border = "1px solid red'; } else{ numOwners.style.border = ""; } if (!checkLength(ownerName)){ errors[errors.length] = "New Error"; ownerName.style.border = "1px solid red"; } else{ ownerName.style.border = ""; } if (errors.length > 0){ return false; } } You not only save typing, but your script is actually more efficient this way. Why? Because you're not asking it to find the same element over and over again. You already have a hold of it, so why would you want to force your script to make document.whatever calls to obtain the same data?
-
It would work, but that's a lot of extraneous markup to have on each page, especially if you have multiple languages in play (you'd probably want English, French, and Spanish as a bare minimum). For the user, the smoothest option would probably be to use AJAX. Have the various 'language packs' (for lack of a better term) reside on the server. When a button is clicked on the page, the client would then retrieve the correct language data from the server based on that button's value, and fill the appropriate elements with that language data. Even better, the process would be instantaneous for the user.
-
Ballot: IE or Lynx
-
[SOLVED] string represenation of number as number
KevinM1 replied to mikesta707's topic in PHP Coding Help
Ah, I didn't think you meant the word 'fifteen'. Nope, no built-in function. You'll most likely have to regex the string for a known number value, then return the integer equivalent. Fake EDIT: or use one of the functions found in Mark's link. -
[SOLVED] string represenation of number as number
KevinM1 replied to mikesta707's topic in PHP Coding Help
Have you tried casting it to an int? -
Ah, there it is. That's what happens when I rush.
-
After the else-clause in the Database class' constructor, write the following line: echo "The database is connected: {$this->isConnected}"; Actually, wait...is the id column of your rooms table set to NOT NULL?
-
No, it's is_resource(). I rushed while typing. Can I see how you're calling the function?
-
Okay, let's start with your database class, as that needs some work. Rule #1: Never use 'global'. Regardless of whether you're coding procedurally or using OOP, 'global' is bad. Argument lists exist for a reason. Rule #2: Classes have data members and constructors for a reason as well. Use them. Don't fight against convention. So, here's a re-imagined Database class: class Database { private $conn; private $isConnected = false; public function __construct($configData) { $this->conn = mysql_connect($configData['db_host'], $configData['db_user'], $configData['db_pass']); if (!isResource($this->conn) || !mysql_select_db($configData['db_name'], $this->conn)) { $isConnected = false; echo "Could not connect to the database."; } else { $isConnected = true; } } public function query($sql) { if ($this->isConnected) { $result = mysql_query($sql); return $result; } else { echo "Could not execute query"; } } } Now, for the DataManager: class DataManager { private $dbc; public function __construct($configData) { $this->dbc = new Database($configData); } public function addRoom($id = null, $roomName) { $sql = "INSERT INTO Rooms (Room_Id, Room_Name) VALUES ('$id', '$roomName')"; $result = $this->dbc->query($sql); return $result; } } Then, in the client code: require_once('config.php'); $DataManager = new DataManger($cfg); /* etc. */ Try that as a first step.
-
1. What is line 89? Is it the mysql_query call in your DataManager class? 2. Are you sure that the non-DataManager version is working? Are your rooms actually being added to the db? 3. What does your Database class look like?
-
1. Where's your while-loop? 2. Use code tags.
-
The simplest way to get what you want to work is to give both your toggle element and your div the same onmouseover behavior. That'll force the div to stay open while you navigate through it. I've written a demo that works in both IE 8 and FF 3.5. <html> <head> <title>Quick Links</title> <style> #linkbox { width: 350px; border: 1px solid black; display: none; } #linkbox a:hover { color: green; } </style> <script type="text/javascript"> window.onload = function(){ var toggle = document.getElementById('toggle'); var linkbox = document.getElementById('linkbox'); toggle.onmouseover = function(){ linkbox.style.display = 'block'; } linkbox.onmouseover = function(){ this.style.display = 'block'; } linkbox.onmouseout = function(){ this.style.display = 'none'; } } </script> </head> <body> <a id="toggle" href="#">Mouse over me</a><br /><br /> <div id="linkbox"> <a href="http://www.google.com/">Google</a><br /><br /> <a href="http://www.microsoft.com/">Microsoft</a><br /> <a href="http://www.apple.com/">Apple</a><br /> <a href="http://www.ubuntu.org/">Ubuntu</a> </div> </body> </html> One final note, and it's something I tell all aspiring JavaScript coders - you should always attempt to write your code in an unobtrusive manner. What that essentially means is that you shouldn't have JavaScript code embedded in your HTML. It makes it easier to debug existing code and add new code if your script is in one centralized location. More importantly, it makes it easy to write sites that degrade gracefully in older browsers. Further, JavaScript gives you plenty of tools to grab whatever element you want, so there's no real excuse not to. Also, to R4nk3d, you shouldn't be using multiple getElementById() calls to get a hold of the same element. That's very inefficient/slow. Instead, store that retrieved element in a variable. That way, you won't be asking JavaScript to repeatedly search for the element you already have.
-
[SOLVED] Don't understand what I'm doing wrong on this :/
KevinM1 replied to adamjones's topic in PHP Coding Help
You need to loop through your results when you use a SELECT query: while ($row = mysql_fetch_assoc($result)) { // do stuff } Without looping, you only get the first row. -
It still looks a bit ignorant to me. Essentially, he doesn't like Western women because he states his friends got shafted by them in divorces. He some how uses that anecdotal evidence as the basis for thinking that all Western women are bad. This just screams a lack of firsthand experience on the matter. I'm certain that there's an underlying cultural bias at play here, too, like you say. But his posts read as "I don't know, and I don't care to know. I'm right, and that's that." It's his right to believe such, but it certainly is a very small and willfully ignorant way to view the world IMO.
-
document.write starts writing at the beginning of the document, overwriting what was originally there. Use innerHTML when building your table instead. Also, your visibility toggle should be toggling between hidden and block, as a div is a block element.
-
We have a web hosting sticky for a reason. It's in this sub-forum.
-
Pretty spartan so far. The one thing I don't like is the 'About Me' box. I hate page items that scroll with me. It's just distracting. Of course, this is a personal pet peeve.
-
Western women aren't inherently evil. Neither are Eastern women, or African women, etc. There are assholes/bitches in every culture. Similarly, there are awesome people in every culture. Now, you may find more in common with someone from a particular culture, or you may be more attracted to them, but to dismiss/aim for one particular kind of person is pretty dumb, IMO. Keep your options open and you may be surprised at who you find yourself falling in love with.
-
Ah. Back when I was learning C++ in the late 90's, we only ever used the >> operator for input. If we wanted to terminate the loop, we'd feed it an end of line/file character, which was Ctrl-D on our linux machines. So, typically our loops would look like: while (cin >> someInput, cout << "=> ") { //do stuff } Unfortunately, my C++ is weak, so I'm not sure what is causing your problem.
-
Why are you using both >> and get()? Shouldn't you only be using the >> operator in this case?
-
How to use methods from the same 2 included files
KevinM1 replied to DexterR's topic in PHP Coding Help
Hrmm, is there a way I can accomplish what i'm looking to do? I've never played with Wordpress, but I'm sure there is. You'd just need to connect to the other db, pull the correct results, and post them. I can't tell you how to do that without seeing how Wordpress does it. -
It's really not very clear, though. Why is the 'Users" link more prominent than the 'Home' link? Why not have a login box right in the main page? Should things like users, ranks, and stats be visible to non-members? What does any of it mean in the context of the game? What is the game about, anyway? Everything is floating around without any context to put it all together. Your lack of page structure stems, in part, from that lack of content. Okay, this is some sort of post-apocalyptic game set in the aftermath of a nuclear holocaust. That's not really descriptive, though. It's a sentence, not a description. How do I play? What are the game's features? Is it a RPG? Is it a Civilization-esque game? Is it turn-based? Is there a way to win, or does it just progress infinitely? These are the things your site needs to address. No one is going to play a game they don't understand. Your home page should answer these questions briefly, with separate specialized pages for additional info if needed. Users shouldn't need to press 'Register' to get a taste of the game's story. In terms of making the site better looking, you really need to look critically at existing sites. The vast majority of sites share common design elements. Why? Because they work. Headers, footers, etc all serve a purpose. Not having them is a distraction. Keep in mind, you don't have to be a Photoshop expert to make a decent looking site. In fact, simple, clean designs are all the rage right now. But you should have some sort of overall structure and a look that will compliment your content rather than hide it.
-
How to use methods from the same 2 included files
KevinM1 replied to DexterR's topic in PHP Coding Help
Include isn't an assignable function. That is, you can try to stuff the contents retrieved from it in a variable. Instead, include and its related functions (include_once, require, and require_once) simply dump all of the included file's contents into the script at that point. -
function that calls a "class function" outsid itself
KevinM1 replied to gladideg's topic in PHP Coding Help
Ugh. Don't use global. Argument lists exist for a reason. Instead, use: function check($dbc, $uuid) { $row = $dbc->query("SELECT UUID FROM someTable WHERE UUID = $uuid"); if ($dbc->affected_rows > 0) { return true; } else { return false; } }