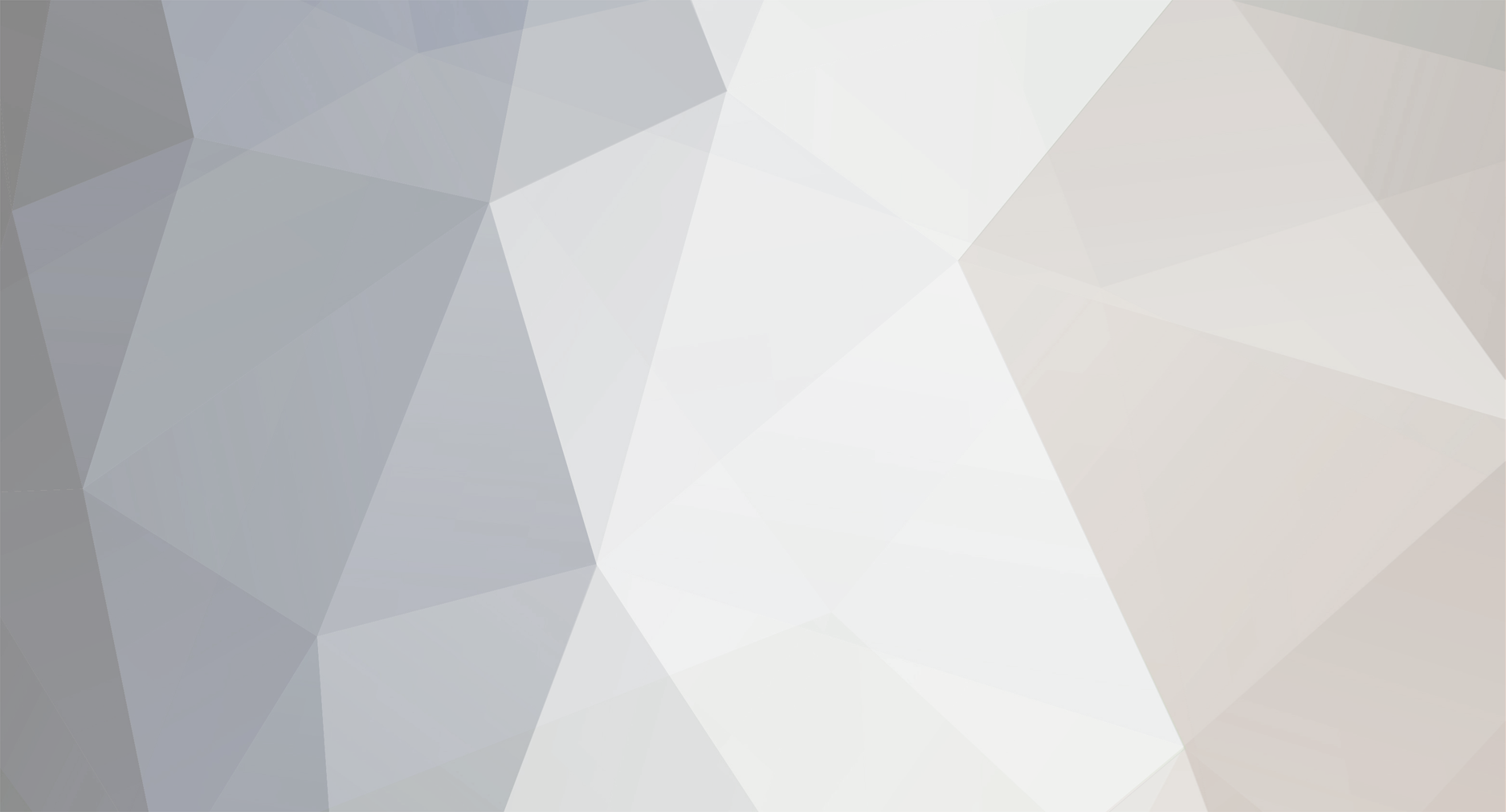
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
JavaScript is a bit different than other languages. Returning a value doesn't stick it in an element. Also, the way you're attempting to use events is a bit weird. You simply need: <script type="text/javascript"> window.onload = function(){ var quantity = document.getElementById('txtChar'); var amount = document.getElementById('amntChng'); quantity.onchange = function(){ amount.value = this.value * 2.50; //you may need amount.innerHTML = this.value * 2.50 instead } } </script> ... <td><input id="txtChar" /></td> <td><input id="amntChng" /></td> A couple of key ideas here: I don't like mixing JS and HTML. It's a style choice, but in my experience, things are easier to fix/manage/edit/maintain if markup and script are separate. So, my solution does away with inline JS calls in the HTML. To put it another way, you wouldn't need to add events (i.e., onchange) to the elements themselves within the HTML. The code isn't necessarily complete. It should show you how to approach the problem, however.
-
[SOLVED] Call to functions.php returns strange answer...
KevinM1 replied to DEVILofDARKNESS's topic in PHP Coding Help
It looks like a scope issue. $error is defined within your function's local scope, so it's unavailable to any higher level of scope. A quick fix would be to modify your function to return $error. You can then assign that error to a variable in the higher scope. Using code - The end of nation_check: return $error; Inside your if-conditional: $error = nation_check($nationname, $username); If that doesn't fix it, print_r $error so you can see what's inside of it. -
Haha, yeah, I hear you. I like Chrome as a concept, but it doesn't quite feel ready for primetime yet. It doesn't help that it's ugly. I don't like its aesthetic at all. Way too top-heavy for my tastes, and the tabs just don't look good. I managed to build myself a fairly minimalist UI for Firefox using a few addons (Personas and Tiny Menu do most of the work, with Remove New Tab Button, Organize Status Bar, and Download Status Bar doing the rest). Here's what I'm currently rocking: http://www.nightslyr.com/twitterfox.jpg My only complaint is that there are two buttons for refresh and cancel. Firefox does everything I need it to. It's still fast, despite the addons I've installed, and I can customize the hell out of it. What more do I need? Now I'm waiting for Thunderbird to reach version 3, so I can match themes.
-
A good rule of thumb is to keep all properties private and use accessor methods to manipulate them. Public properties run the risk of being overwritten.
-
is_bool isn't really a good fit here as it only checks whether or not the value is a boolean, not if it's true or false. Also, if the OP's type is a string, it won't generate the desired result. To the OP, what is $type, and what's stored in it?
-
Try it without the short tags, i.e.: <?php echo $buildTable; ?>
-
[SOLVED] JS not working with <!DOCTYPE html PUBLIC...... header!
KevinM1 replied to unistake's topic in Javascript Help
The problem is that 'text' has no context within the body of that function. It doesn't exist in the function's scope. You need to get a hold of 'text' - either by using document.getElementById, or document['formName'].elements['text'] (where formName is the name of your form) - before you can start manipulating it. -
Where are your <table> tags?
-
Good catch. If that doesn't fix it, print_r on man_rows, just so we can see if its being filled.
-
You're not pushing values onto the man_rows array. Instead, you're overwriting the value stored in man_rows because you're using simple assignment. Try instead: $man_rows[] = $man_db->loadObjectList();
-
Need code or a link to the site. Despite our many talents, telepathy isn't among them.
-
[SOLVED] JS not working with <!DOCTYPE html PUBLIC...... header!
KevinM1 replied to unistake's topic in Javascript Help
Just off the top of my head: function calculate(radioButtonVal, textFieldVal /*, ... */, outputField){ switch (radioButtonVal){ case /* button 1 value */: // do first kind of calculation break; case /* button 2 value */: // do second kind of calculation break; case /* button 3 value */: // do third kind of calculation break; default: // report error } } window.onload = function(){ var textVal1 = document.forms["formName"].elements["textField1"].value; //repeat for however many text field values you have var outputField = document.forms["formName"].elements["outputField"]; var radios = document.forms["formName"].elements["radioButtonsName"]; var numRadios = radios.length; for(var i = 0; i < numRadios; ++i){ radios[i].onclick = calculate(this.value, textVal1 /* , any other text field values */, outputField); } } This is just a skeleton of the way I'd go about it. It won't work as-is, but it should give you more than a head start on figuring it out. -
Look up 'HTML injection.' That should speed up your search efforts.
-
That's a good point. Also, now that I think about it more, the tests used in the link tested ASP.NET's background code. ASP.NET doesn't have native loops or file handling - that's all done with the code-behind languages. So, it's not really a PHP vs. ASP.NET battle, but a PHP vs. C#/VB battle. The ASP portion is essentially the server controls, which I'm betting would be slower to render than straight HTML, even if the PHP in question was using a template system, or some other form of OO for output. ASP checks the user agent of the browser currently accessing content, then decides how to best render the controls based on that info. That must incur a pretty hefty cost.
-
But, can you determine if it's ASP.NET that's causing the slowdown, or a chunky UI and/or db setup, which tend to be more of an issue with page loads? I ask, because the link you gave confirmed my own suspicions that ASP.NET is actually faster than PHP, which isn't surprising if you think about it - it's an issue of compiled vs. interpreted languages.
-
With ASP.NET, I've found the following page to be invaluable: http://69.10.233.10/KB/aspnet/ASPNET_Page_Lifecycle.aspx Page_Load() doesn't do what it looks like it should do. I've found that putting my code in Page_Init() and Page_PreRender() is a much better way to go. This is especially true when trying to figure out event handling - events are fired after Page_Load().
-
It's not a question of whether one language can do something that another cannot. The question, rather, is if one can do something better than the other. All programming languages have their pros and cons. PHP is no exception. It's how you leverage their pros and minimize those cons that matters. ASP.NET (which is what I'm assuming you're asking about, as there's not much demand for 'classic' ASP) is a decent platform. It has a lot of nice, powerful features built-in, and its close ties to Microsoft's various IDEs make it easy to use. Indeed, one of its benefits is that non-programmers can create fully functioning dynamic pages/sites by drag-and-dropping controls onto the design surface of the IDE and the various configuration wizards. There are drawbacks, too, of course. Given the way ASP.NET works, you have less control over everything. CSS can be a pain in the butt if you like using element ids to apply styles. There's the web.config and machine.config files you need to be aware of. And the ASP.NET page lifecycle can be difficult to grasp at first. As to whether or not it's 'worth' learning another language, of course it is. The more languages under your belt and the more skills you gain, the better your employment opportunities will be. You shouldn't lock yourself into one technology. There's no guarantee that PHP will remain as popular as it is, and there's no guarantee that the PHP of the future will resemble what we have today.
-
Ah, there is that.
-
Interesting. I'm curious as to why that decision was made in the HTTP spec. There has to be some technical reason for it, because at first blush it seems a bit restrictive/antiquated.
-
You're not relying only on JavaScript validation, are you? JavaScript can be turned off in the browser, rendering client-side validation useless. In any event, you should check to see if the form fields' values actually contain values. If not, then raise an alert.
-
To be honest, with modern browsers and computers, I wouldn't worry too much about speed. Unless you have a very large, or loop-intensive script, it probably won't matter where you put it. I, personally, like putting my code in the head and using either window.onload() or jQuery's ready() function to load it. It helps foster unobtrusive design while ensuring everything in the document is loaded before I attempt to manipulate elements. But, to each their own. As far as the people without JavaScript are concerned, they're why the noscript tag exists.
-
The following works: <?php $cars = array("car1", "car2", "car3", "car4", "car5"); $firstTest = "car2"; $secondTest = "car5"; for($i = 0; $i < count($cars); ++$i) { if ($cars[$i] != $firstTest && $cars[$i] != $secondTest) { echo $cars[$i]; } } ?> It outputs:
-
I have two... really more like one and a half. Gateway desktop, which more than does the job. iBook laptop. Yeah, yeah, I know. It's an old G3 pos. It worked just fine for what I used it for - taking notes in college - but it's essentially a computerized typewriter. I'll be getting a new laptop this fall, though. Just waiting for Windows 7 to come out.
-
Try: for($i = 0; $i < count($cars); ++$i) { if($cars[$i] != $var || $cars[$i] != $var1) { echo $cars[$i]; } }
-
After you obtain the $ulevel, but before you echo the data, do something like: $level = ""; if ($ulevel == 9) { $level = "admin"; } Then, use $level as the echoed data. And, you can have an infinite number of if statements, or if/else statements.