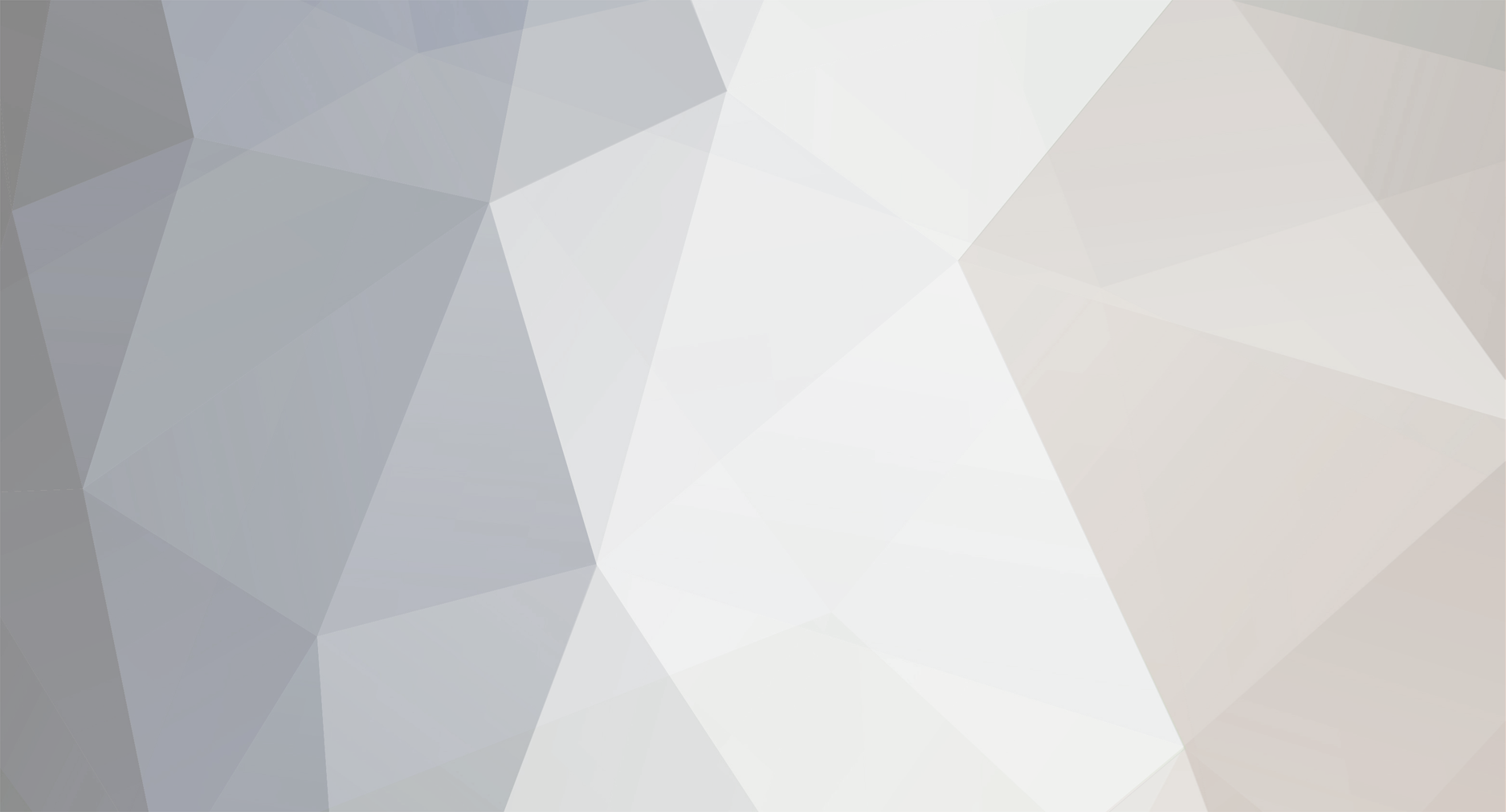
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
http://www.php.net/manual/en/function.array-slice.php
-
Try constructing it with double quotes: protected $ConnectionString = "username={$this->user};password={$this->pass};database={$this->server};";
-
Well, one of the things masking the problem is the use of a 3rd party template engine to insert PHP code within the markup. There's nothing wrong with using one, but it does make it harder for someone that doesn't use one (like me ) to figure out what's going on. My two compatriots are correct in the structure of how to check the price, assuming it's a number and not a string. if($price > 0) { echo "£" . $price; } else { echo "FREE"; } You should double-check that you're referencing the price(s) correctly. Try outputting it to the screen as is.
-
Not seeing anything when visiting that file is bad. Something went wrong somewhere. Do me a favor and add the line: error_reporting(E_ALL); To the file after the line with require_once(). Upload it to your web root, and then visit it again. Hopefully it'll output the error(s) causing the problem. Also, check the database itself to see if anything's been inserted into it. There should be at least one row with information in it. Are any of the other columns not-null?
-
[SOLVED] help please, add phone number to the code to work.
KevinM1 replied to madox's topic in PHP Coding Help
You need to add the phone number to the mail message rather than as an extra parameter to the mail() function. In otherwords: $message .= "Phone number: $phone"; mail("email_address", $subject, $message); -
Hey, sorry about that. Some personal things have come up, so my time's been limited. I'll be able to help you more fully tomorrow, but in the meantime, a couple of things: 1. Create a new database column named 'id'. Make it an unsigned int (4) non-null auto-increment primary key. I always like having a generic id field, just in case. 2. You'll need to test your PHP script database connection. So, in notepad, copy the following and name it dbtest.php: <?php require_once('../../dbconnect.php'); $dbc = mysql_connect(HOST, USER, PASS) OR die("Unable to connect to database: " . mysql_error()); mysql_select_db(MYDB) OR die("Unable to select database: " . mysql_error($dbc)) $query = "INSERT INTO jason_gold (name, email_address) VALUES ('Kevin', 'kevin@gmail.com')"; $result = mysql_query($query); if(mysql_affected_rows() == 1) { echo "Insert successful!"; } else { echo "Something went wrong!"; } ?> Upload that into your web root folder, then access it through your web browser by visiting that file (it should be at www.jasongold.org/dbtest.php). Report back all the output and/or errors displayed on the screen.
-
Well, the simplest way to do the skater park thing would be to: 1. Figure out all of the database fields you need. Town, county/province/state/whatever, street address, name of the park, etc. 2. Normalize the database. I'm not too skilled at database normalization myself, but I'm sure a Google search will yield the info you need. 3. Create a form for users to add new parks to the mix. Not all that difficult, although you'd probably want to verify that the submitted park exists before adding it to the list. You probably don't want skate parks referencing genitalia in the list, for example. 4. Create a search form, like the one on that site. That's probably the easiest form to create. The dropdowns are created by the info that's already in the DB, like so: $query = "SELECT state IN skate_parks ORDER BY state ASC"; $result = mysql_query($query); echo "<select name='states'>"; while($row = mysql_fetch_assoc($result)) { echo "<option value='{$row['state']}'>{$row['state']}</option>\n"; } echo "</select>"; But yeah, that's the basic process. There may be better ways to store such a volume of info, but this is the easiest, quickest way I can think to do it. The key really lies in normalizing the database. That will make it easier (possible?) to do various searches and result ordering.
-
You opinion on what makes a good web developer
KevinM1 replied to WebHostGurl's topic in Miscellaneous
I can't. Quod erat demonstrandum, I am not an idiot. Yay Okay, I'll leave now... You can administrate like the dickens, though. The dickens. -
You opinion on what makes a good web developer
KevinM1 replied to WebHostGurl's topic in Miscellaneous
Everyone knows that PERL doesn't exist. Perl does though. I've used PHP, Javascript, Ajax, and PB&J extensively while writing web apps. (If you figured out the subtle humor, congrats.) *snicker* So...strawberry? Raspberry? Grape? -
ASP.NET isn't too horrible. I like how easy it is to generate form structures (drop down lists, checkboxes and radio buttons) from database resources, its calendar control, and its validation controls are okay. And, to be honest, I'm loving C# at the moment. But the rest is pretty 'meh.' Especially how ASP.NET renders ids. Makes it nearly impossible to use ids with CSS. I can only imagine the headaches associated with trying to use JavaScript out of the box with it. I guess that's why they strongly suggest registering client side scripts with the rest of the application, although that itself is a bit of a nightmare process too. And yeah, Boxerman, what you're trying to build is a pretty big undertaking. Certainly more complicated than I think you grasp at the moment. My advice is to start small -- get some web hosting and start playing around. It'll be a while before you can make a full-fledged dynamic site.
-
You opinion on what makes a good web developer
KevinM1 replied to WebHostGurl's topic in Miscellaneous
To expand on what DarkWater said, it's of vital importance to know what constitutes professional work which utilizes what most in the industry considers to be best practices. OOP, unobtrusive JavaScript, familiarity with popular frameworks and/or libraries (jQuery, Code Igniter, Smarty, etc.), CSS for layout, etc. It's incredibly easy for any crappy, wannabe developer to impress the non-technical. After all, this industry is basically alphabet soup. It's not hard to impress most people by spitting out a few names of web technology ("Why yes, all my sites are Web 2.0 compatible. All my sites are PERL, PHP, JavaScript, AJAX, and PB&J based.")*. Moreover, crappy work tends to be buried underneath the hood. Any idiot can create a decent looking site using just Photoshop and Dreamweaver. But can they construct it in such a way that it's easy to maintain, edit, and expand? Probably not. My advice is to make sure you have someone with quality technical knowledge sit in on your interviews. Someone who can read code, and understand what the potential employee is actually writing. And, more importantly, someone who themselves engages in professional standards. If you have someone like that acting as a filter, you'll get talented employees. *Extra points if you know which one of those technologies isn't real. -
You're pretty close. A couple of things: Addslashes() is not secure. You should use mysql_real_escape_string() instead. Secondly, and more importantly, you can't use logical AND (&&) in the manner in which you did. Instead, you're going to have to run each query individually: $result1 = mysql_query($query1); $result2 = mysql_query($query2); if($result1 && $result2) { //everything okay } else { //something went wrong with the insert }
-
Like pretty much everyone else out there, I'm attempting to write my own web-based RPG. I'm currently writing it in ASP.NET/C#, but would like to port it over to PHP if I can get it working. Anyhoo, I've hit a bit of a design snag when it comes to coming up with a reasonable way to handle a character's special attacks. I've decided that each character will get a special attack at level 1, 3, then every three levels after that (6, 9, 12, etc). I'm just not sure how to implement them. Currently, I'm employing the strategy pattern with my characters. Each PlayerCharacter object contains a CharacterClass object, like so (translated to PHP): class PlayerCharacter { private $charClass; public setCharClass(ICharacterClass $charClass) { $this->charClass = $charClass; } } ICharacterClass is an interface that all character classes implement. I'm thinking that each special attack should be an object in and of itself, deriving from a base class/interface related to the character class which they (logically) belong. So, all soldier attacks would derive from the same base soldier attack info, all hacker attacks would derive from the same base hacker attack info, etc. I could 'unlock' the new attacks by adding them to an array/list when the character reaches the right level. My main question is whether or not this is an ideal way to approach this. I'm trying to keep my systems as flexible as possible, so I can add new classes and attacks easily. The one bad 'smell' I'm getting from this approach is determining which attack to add. I mean, I don't want to have a gigantic switch statement along the lines of: public function levelUp() { if($this->currentLevel % 3 == 0) { $this->addNewAttack(); } } private function addNewAttack() { switch($this->currentLevel) { case 1: $this->attacks[] = new SimpleAttack(); break; case 3: $this->attacks[] = new ModerateAttack(); break; case 6: $this->attacks[] new DevestatingAttack(); break; //etc } } I mean, I could pass the PlayerCharacter object into some sort of AttackFactory, but I'm not sure if that would really remove the conditional. I think it would simply move it to another (albeit, more logical) location. Any ideas?
-
Hmm... I think it may depend on how your hosting is set up. Let me check with my phpMyAdmin.
-
Actually, you should probably put it in /var, just to be safe. I have the feeling that /var/www is still publicly readable.
-
In all likelihood, this hacker has probably spoofed their IP address anyway. Either that, or they're a very stupid script-kiddie.
-
Yeah...nice..... >_> To be honest, I kinda expected it. The Phins always play the Pats hard, and Cassel doesn't seem comfortable throwing down field. The O-line has been a bit...off since the Super Bowl, which is disconcerting as it's the same group as last year, minus Stephen Neal who's out with an injury. My biggest concern is Randy Moss. He's not even being thrown to, and so far, this looks like a wasted year for him. Will he still be a good soldier, or will the old Randy come back? And, more importantly, what will he have left in the tank for next year? He's not getting any younger. But, hey...at least we're not Jets fans. ;-P
-
Use this: http://www.php.net/manual/en/function.urlencode.php
-
Looks pretty good. I'd change the phone numbers to VARCHAR's, though, as that way you can save any hyphens or parentheses that the user enters in order to divide up the area code from the rest of the number. Either that, or we could change your form so that each component of the telephone numbers is their own field, then create the phone number from that. In other words: Day phone: <input type="text" name="dayPhone[]" /> - <input type="text" name="dayPhone[]" /> - <input type="text" name="dayPhone[]" /> I'm not sure if that code snippet will make much sense for you, as I'm not sure how much PHP (if any) you know. What that bit of code does is store each phone number component (area code, etc.) as a different element of an array. Inside of the script that will process this info, we can just go through each element and construct our phone number: $dayPhone = "({$_POST['dayPhone'][0]}) {$_POST['dayPhone'][1]}-{$_POST['dayPhone'][2]}"; If none that makes any sense, then don't worry about it. To get you started on your way, create a file with notepad named dbconnect.php. Then, write the following inside: <?php DEFINE ('HOST', 'localhost'); DEFINE ('USER', 'user'); DEFINE ('PASS', 'password'); DEFINE ('MYDB', 'mydb'); ?> And replace the lowercase values of 'user', 'password', and 'mydb' with your database login info (your actual database username and password) and the name of the database you're trying to connect to (in your case, most likely jason_gold). Once that's written, upload it to your web host, but put it in the directory above your web root, if possible. This will help secure this critical information from prying eyes. Okay, the next step is to actually do some more web design. You probably want to have a page saying something along the lines of "Thank you for submitting your information" along with a way for them to continue to navigate through your site. So, create that page like you normally would, but name the file processContact.php. This page will be where all the magic happens. Let me know when you're ready for the next step.
-
Yeah, unfortunately, today isn't a good day for me. I'll try for tomorrow. Quick question: is your database setup yet? If not, that'll be the next step.
-
Okay, cool. I'll see if I can write something up for you by the end of the day.
-
Hmm...try removing lines 287 - 289. It looks like it's trying to end a few elements that don't actually exist. That should, hopefully, move your Flash banner up a bit. As far as processing the info goes, you do have options. The easiest thing I could do is set it up so the script e-mails you the info after each submission. I could also save it to a database, but you'd need to have another script to access and display that info. Another option is to save it to a text file. There's also the option of combining some of these ideas. You could get an e-mail from the site telling you someone submitted info, but have the actual info saved to the DB or file.
-
Patriots - born, raised, and still reside in NH. I'm not one of those obnoxious bandwagon fans. I remember watching Grogan and Tippett as a kid. So, I'm an obnoxious longtime fan. The injury to Brady sucks. Nothing quite like wasting a great potential season.
-
Here are a few... Don't lie on your resume Don't accept a job then scramble to learn how to actually do it Don't buy a 3rd party script at the last moment to try to save yourself when that deadline looms ever closer May not apply to you, but good suggestions nonetheless.