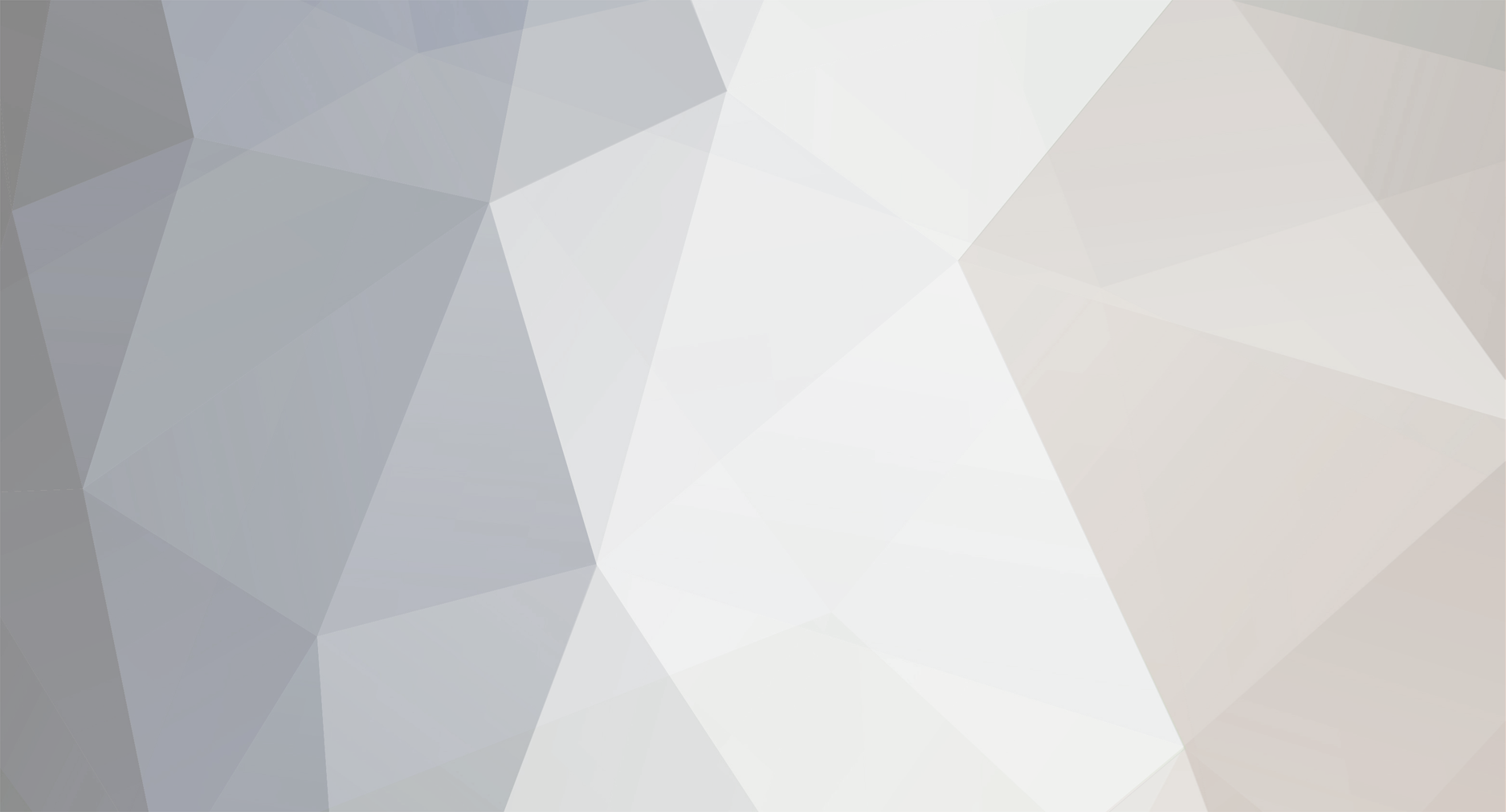
Cagecrawler
Members-
Posts
247 -
Joined
-
Last visited
Never
Everything posted by Cagecrawler
-
When the player clicks on a link, I want to have a popup that checks to see if they want to delete their character. This is the script I have at the moment: <script language="JavaScript"> <!-- function confirm_delete() { var input_box=confirm("Your player will be DELETED from the game. Are you sure you want to do this?"); if (input_box==true) { // When OK is clicked window.location="deleteplayer.php"; } else { // When Cancel is clicked window.location="preferences.php"; } } --> </script> This is my link: <a href=\"deleteplayer.php\" onClick=\"confirm_delete()\">[Harakiri!]</a> It's in PHP, hence me escaping the quotation marks. It works if it's a button, but not if it's a normal link. Any idea why?
-
Yes I am using mysql, and that link explained it all. Thanks.
-
For that example, put LIMIT 1 on the end of the query so it only returns the oldest. In fact, you could use this query: "DELETE FROM last_5 WHERE userid=$userid ORDER BY car_id ASC LIMIT 1" I'd still add in a date column, like was earlier mentioned. Then you can use this: "DELETE FROM last_5 WHERE userid=$userid ORDER BY date ASC LIMIT 1"
-
It works, thanks. I double checked and my database column type is timestamp, so why does it output a datetime?
-
Try: foreach($array as $value) { foreach($value as $value2) { if($value2="-") unset $value; } }
-
I am trying to show a particular date which is stored in my database as a timestamp. If I echo just the timestamp variable, i get the following: 2007-01-28 04:38:23 However, I put it into the date function like so: date('j.n H:i',$transactions['time']) This should display 28.1 23:38, but the timestamp isn't recognised and it displays it as if the timestamp was 0 (ie. 1.1.1970). How can I get it to use the right timestamp?
-
With textareas, using value doesn't work. You'll need to do this: <TEXTAREA ROWS=5 COLS=65 NAME="Description3"><?php echo "$Description3"; ?></TEXTAREA>
-
[code] <?php if($_SESSION['user']) { echo $_SESSION['user']; } else { //INSERT FORM HERE } ?> [/code]
-
Ignore me, as thorpe pointed out, you can use mysql_insert_id() which is much easier...
-
If you've just inserted it, then you can do this: [code] <?php $query=mysql_query("SELECT COUNT(*) FROM table_name"); $result=mysql_result($query,0); echo $result; ?> [/code] As it'll be the last row, just find out how many rows there are. If you want to do it at a later time, then this won't work because other rows may have been inserted. You could use: [code] <?php $query=mysql_query("SELECT id FROM table_name WHERE something=$something"); $result=mysql_fetch_assoc($query); echo $result; ?> [/code] This'll work all the time, but only if you have one of the pieces of data stored somewhere, like in a SESSION variable.
-
Thanks. It's similar to what I was thinking, and it'll give me a good start. I'll no doubt be back saying how it never works... :P
-
Display text on the x and y axis of the bar graph
Cagecrawler replied to rajbal's topic in PHP Coding Help
A series of imagettftext() functions? -
I have a fantasy stock market that I am in the process of creating, and what I want to do is give the user data about changes over a 6hr and 24hr period. The prices update every 5 mins, so every 5 mins the last value would be discarded and a new one added to the front end. My qustion is this - what is the most efficient way of doing this? I was going to store it in a database and then move all of the values, but I'll need almost 300 columns to do it (and with over 100 companies, thats a lot of data). That seems really inefficient, but I can't think of any other way to do it. Any ideas?
-
Try putting a php.ini file in the folder where your files are situated. It will sometimes override the shared host main one, if your hosts allow that.
-
Adding a session variable to the Log In Server Behavior, HELP.
Cagecrawler replied to SauloA's topic in PHP Coding Help
If you want to take a value from a query, you'll need to turn it into an array with mysql_fetch_array(). I've edited the code below, although it's untested so it might need something else. Also, when posting your code, stick it in [ CODE ] tags. [code]<?php require_once('../Connections/connBlog.php'); ?> <?php // *** Validate request to login to this site. if (!isset($_SESSION)) { session_start(); } $loginFormAction = $_SERVER['PHP_SELF']; if (isset($_GET['accesscheck'])) { $_SESSION['PrevUrl'] = $_GET['accesscheck']; } if (isset($_POST['username'])) { $loginUsername=$_POST['username']; $password=$_POST['password']; $MM_fldUserAuthorization = "al_levelid"; $MM_redirectLoginSuccess = "home.php"; $MM_redirectLoginFailed = "failed.php"; $MM_redirecttoReferrer = true; mysql_select_db($database_connBlog, $connBlog); $LoginRS__query=sprintf("SELECT user_id, user_sname, user_pass, al_levelid FROM user_tbl WHERE user_sname='%s' AND user_pass='%s'", get_magic_quotes_gpc() ? $loginUsername : addslashes($loginUsername), get_magic_quotes_gpc() ? $password : addslashes($password)); $LoginRS = mysql_query($LoginRS__query, $connBlog) or die(mysql_error()); $LoginArray= mysql_fetch_array($LoginRS); //Creates the array $loginFoundUser = mysql_num_rows($LoginRS); if ($loginFoundUser) { $loginStrGroup = mysql_result($LoginRS,0,'al_levelid'); //declare three session variables and assign them $_SESSION['MM_Username'] = $loginUsername; $_SESSION['MM_UserID'] = $LoginArray['user_id']; //Stores the user_id in a session variable. $_SESSION['MM_UserGroup'] = $loginStrGroup; if (isset($_SESSION['PrevUrl']) && true) { $MM_redirectLoginSuccess = $_SESSION['PrevUrl']; } header("Location: " . $MM_redirectLoginSuccess ); } else { header("Location: ". $MM_redirectLoginFailed ); } } ?>[/code] -
Solved... I wasn't using <img src="key.php">
-
The $_SERVER['HTTP_REFERER'] method: [code=statement.php] <?php if($_SERVER['HTTP_REFERER']=="disclaimer.php") //You might need the full address in here, but this should be ok. { //Insert statement.php here. } else { header("Location:disclaimer.php"); ?> [/code] You shouldn't really use this though. [quote=http://uk.php.net/manual/en/reserved.variables.php] 'HTTP_REFERER' The address of the page (if any) which referred the user agent to the current page. This is set by the user agent. Not all user agents will set this, and some provide the ability to modify HTTP_REFERER as a feature. In short, it cannot really be trusted. [/quote] The checkbox method, which is better: [code=disclaimer.php] <?php <form action="statement.php" method="post"> <div style="width:640px" align="left"> <fieldset class="fieldset"> <legend>Disclaimer</legend> <table cellpadding="0" cellspacing="3" border="0" width="100%"> <tr> <td>In order to proceed, you must agree with the following rules:</td> </tr> <tr> <td> <div style="border:thin inset; padding:6px; height:175px; overflow:auto"> //DISCLAIMER </div> <div><input type="checkbox" name="agree" id="agree" value="1" /><strong>I have read, and agree to abide by the Disclaimer.</strong></label></div> </td> </tr> </table> </fieldset> </div> <input type="submit" class="button" value="Register" accesskey="s" /> </form> [/code] You've probably got most of that, all you need to really add is "<input type="checkbox" name="agree" id="agree" value="1" /><strong>I have read, and agree to abide by the Disclaimer.</strong>" [code=statement.php] <?php $agree=$_POST['agree'] if($agree!==1) { header("Location:statement.php"); } else { //Show statement.php stuff } ?>[/code]
-
Try putting ob_start(); at the beginning and ob_end_flush(); at the end of the script.
-
Here's key.php: [code]<?php function genText($length) { $p_chars=md5(time()); // can be any set of characters while(strlen($out_str)< $length) { $out_str.= $p_chars{rand()%(strlen($p_chars))}; } return $out_str; } $rand_string = genText(8); header ("Content-type: image/png"); $im = imagecreate(60, 20); $bg = imagecolorallocate($im, 0, 255, 255); $text_color = imagecolorallocate($im, 0, 0, 255); imagestring($im, 2, 5, 3,$rand_string, $text_color); imagepng($im); imagedestroy($im); ?>[/code]
-
The cookie only becomes active after the next page load. If you load your page without any cookies, then refresh, it'll work fine. To get around it, have just this code on index.php, and change your current index.php to main.php (or something else you like). [code] <?php if(!isset($_COOKIE['template'])) { setcookie('template', '2_3.css'); } header("Location:main.php"); ?> [/code]
-
I'm trying to create an image to use for registration to stop bots. I've managed to create the image, but when I try to insert anything into the file, or insert it into another file, I get the following error: [code] The image “register.php” cannot be displayed, because it contains errors. [/code] In this case, the image code is in key.php, and the file I'm trying to insert it into is called register.php. Can anybody help please?
-
print_r displays information about a variable. For example, if you use print_r($_POST), it'll display all of the array variables currently set and show their values.
-
ahh... or die(mysql_error()) should be posted after mysql fuctions. Use the code I posted a couple of posts ago. EDIT: Here it is: [code]// Open database... $db = mysql_connect("$localhost", "$databaseuser", "$databasepasswd") or die(mysql_error()); mysql_select_db("$databasename",$db) or die(mysql_error()); // Check and Update affiliate data... $sql="SELECT userName FROM user WHERE userName='$user'"; $result = mysql_query("$sql",$db) or die(mysql_error()); $numrows=mysql_num_rows($result) or die(mysql_error()); if ($numrows != 0) { header("Location:error2.html"); exit; }[/code] [quote] [quote]Thorpe, surely you need to define $result to use it in an if statement?[/quote] Why would you? $result = mysql_query($sql,$db) is an expression, expressions return true or false.[/quote] Isn't = not a comparison expression. If you want it to return TRUE or FALSE, then wouldn't you have to define $result and use $result == mysql_query($sql,$db)? $result = mysql_query($sql,$db) makes $result equal to the query, so it will always equal.