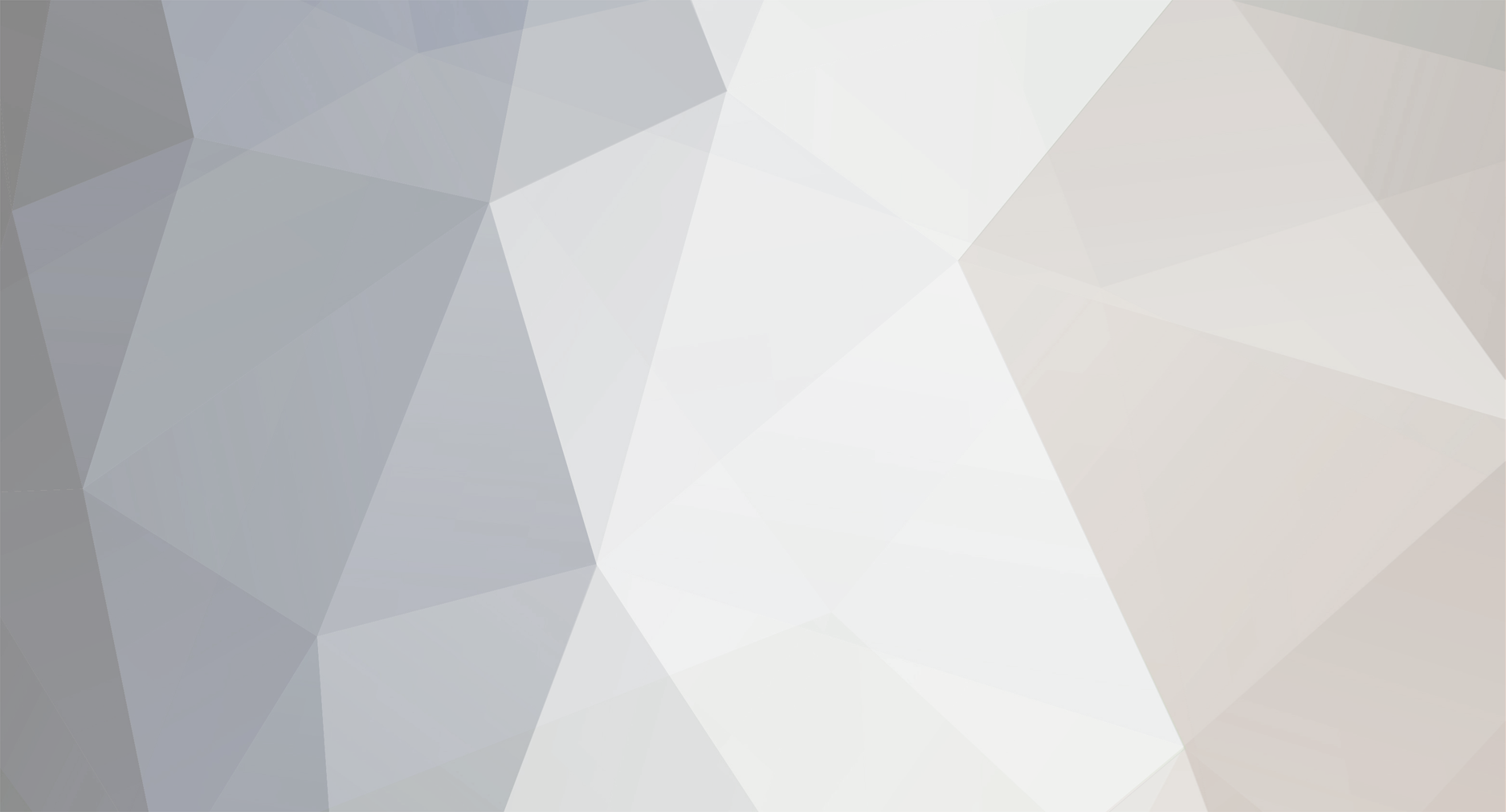
The Little Guy
-
Posts
6,675 -
Joined
-
Last visited
Posts posted by The Little Guy
-
-
what does your source code show?
but...
A. you don't have quotes around your attribute values.
B. why are you even using those, when there are css alternatives?
C. the font tag is deprecated.
-
Real men get Android, where we can do things like mysql, and run a web server
-
This Converts Pacific time to Central time:
SELECT CONVERT_TZ( NOW( ) , 'US/Pacific', 'US/Central' ) ;
-
Options +FollowSymlinks RewriteEngine on RewriteBase / RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d RewriteRule (.+?)$ someFolder/cat.php?id=$1
-
My cousin came up with this, now I don't know if it would work or not, but what you do is create a text field and give it this css:
"display: none;"
Then when you validate the form, you check to see if that field is filled in or not. if it is not filled in, it was probably submitted by a human (due to the fact that they did not see it); if it is filled in then it was probably submitted by a robot. You would probably also give the field a common name, for example if it was a login, maybe the name "url". A robot would more than likely put a url in there and submit it making that field filled out and we now assume a robot put that in there.
-
First off, a database isn't really made to be a file system, so I wouldn't store the images in the database. Instead you should store what you would need to access the file. So, your database may be different depending on how you plan on storing the images.
usually I have a root image directory, in there other directories, for example:
sub1
sub2
sub3
This will spit the load on each directory. If you do that you would need this (example):
directory, image, extension
- directory will contain sub1, sub2 or sub3.
- image will be the image name "My_Cool_Image"
- extension will be the extension of the file: jpg, gif, png, etc.
now if you store the images all over the place, such as different servers you would probably do something like this:
server, directory, image, extension
- server would be the server saved the image on such as "images.srv1"
- directory would be the location on the server "/downloads/user"
- image would be the image name
- extension would be the extension
When you build this it would look like so:
http://images.srv1.site.com/downloads/user/My_Cool_Image.jpg
or
http://images.srv5.site.com/usrData/user/1234/profile_pic.jpg
Their are lots of ways to store images in the database, and all ways are different, it just depends on what your needs are.
-
At work... No, I get too many questions to have time for music, and sometimes for even doing the coding.
At home, I usually listen to music while I code, but lately I just don't feel like coding when I get home, so I play StarCraft or watch Netflix instead, and don't usually listen to music then.
The good stuff
-
I don't know if you have read this, but maybe it will help:
What it sounds like to me, is that if it was coded before being used in the project, (basically was never made for the project but will work with the project) that you can reuse the code as much as you would like. But if it was made for the project, you can not or should I say should not.
-
mother-trucker.org
mother-trucker.co
-
When doing a site that has lots of images, what would be a good technique for storing them, to keep things organized, and the server fast? I have heard that storing all the images in one directory can slow things down when apache has to grab an image to server that is with say 50,000 other images in the same directory.
Right now the only plan that I have is to store the images in a sub-domain's directory, in 10-20 separate directories.
-
want to make a left/right scroller where when you click a button the div's scroll left/right depending on what button you click.
This example has it under the header "What's Hot" you just click the arrows left/right and it slides one div off the screen and the next one shows up.
another example:
I can't find anything on making something like this, anyone have any info on doing this?
-
Hacker.... Cracker....
-
-
Yeah, I saw that, but it isn't a full enough list. I can't find any other list though.
-
do string values like these have a name, and is there a list of them anywhere?
Examples:
\0
\n
\r
-
I am making a php flat file database, and I am trying to think of a way to separate columns and rows from each other. I don't want to CSV style, but more of a database style. NULLs can not be used as column separators because the flat file accepts that as a column value.
So basically what should I do to separate fields from one another and separate one record from another record. Any suggestions on an approach to this?
-
Never mind, I got it.
public function update_settings(){ file_put_contents("$this->pluginLocation/databases/settings.txt", serialize($this->settings)); } public function get_settings(){ $this->settings = unserialize(file_get_contents("$this->pluginLocation/databases/settings.txt")); }
-
I have an array, and I would like to write it to a php file for later use. Any suggestions on how I could write the array to file?
Here is an example array. When the array gets modified in my code, I want to have a method that saves it to a file when I call it. Any suggestions of how can I save it into a file?
$arr = array( "opt1" => 1, "opt2" => array( "opt2.1", "opt2.2" ), "opt3" => array( "opt3.1", "opt3.2", "opt3.3" ) );
-
-
I have this method, I haven't tested it yet, but before I attempt to, do you have any thoughts on it?
It is supposed to take a dir path and remove all the files and directories within that path.
private function delete_directory_structure($path){ $path = rtrim(trim($path),"/"); $files_and_dirs = glob($path."/*"); foreach($files_and_dirs as $fpath){ if(is_file($fpath)) unlink ($fpath); if(is_dir($fpath)) $this->del_dirs[] = $fpath; } $key = array_search($path, $this->del_dirs); if($key != false){ unset($this->del_dirs[$key]); } if(count($this->del_dirs) > 0){ $this->delete_directory_structure($this->del_dirs[0]); } return true; }
-
That is basically a php pointer to your result so php can tell mysql what resource to use.
Example:
$query1="SELECT site_name FROM mtr_control_panel WHERE site_url='".$this->site->url."'"; $query2="SELECT site_name FROM mtr_control_panel"; $result1=mysql_query($query1); $result2=mysql_query($query2);
With that you will have two resources to choose from, by passing that resource to one of the mysql functions php/mysql knows what query you want to work with. Now you can work with 1+ queries, it is similar to the file functions if your familiar with any of those.
-
Like this:
<?php class project0 { private $result; public function mysql_connection() { $host = 'localhost'; $user = 'root'; $password = ''; $db = 'om'; $dbtbl = 'omtbl'; $connetion = mysql_connect($host,$user,$password); $select = mysql_select_db($db); $this->result = mysql_query("SELECT * FROM $dbtbl"); } public function show_my_users(){ while ($row = mysql_fetch_array($this->result)) { print $row['id']; } } } ?>
-
??
can you re-word this:
how can i call a method if the class is an extended classI don't understand what you mean.
-
Another few things:
1. include isn't a function and shouldn't have parenthesis they are allowed but it isn't good practice.
2. if the file has functions/classes defined within it, you should use "require_once" this way you won't get an error for having something more than on time in your code such as a "SomeFunction has already been defined" error.
3. includes only show a warning if the file is not found, whereas require will show a fatal error. I would recommend using require and require_once over include and include_once.
Image database table
in MySQL Help
Posted
don't worry about database speed, I have a database table with about 950,000,000 rows and it can do a query in under a second.
So, I would make a table with at least 4 columns:
image_id, directory, image, extension
you can then add any other fields you would like to that.