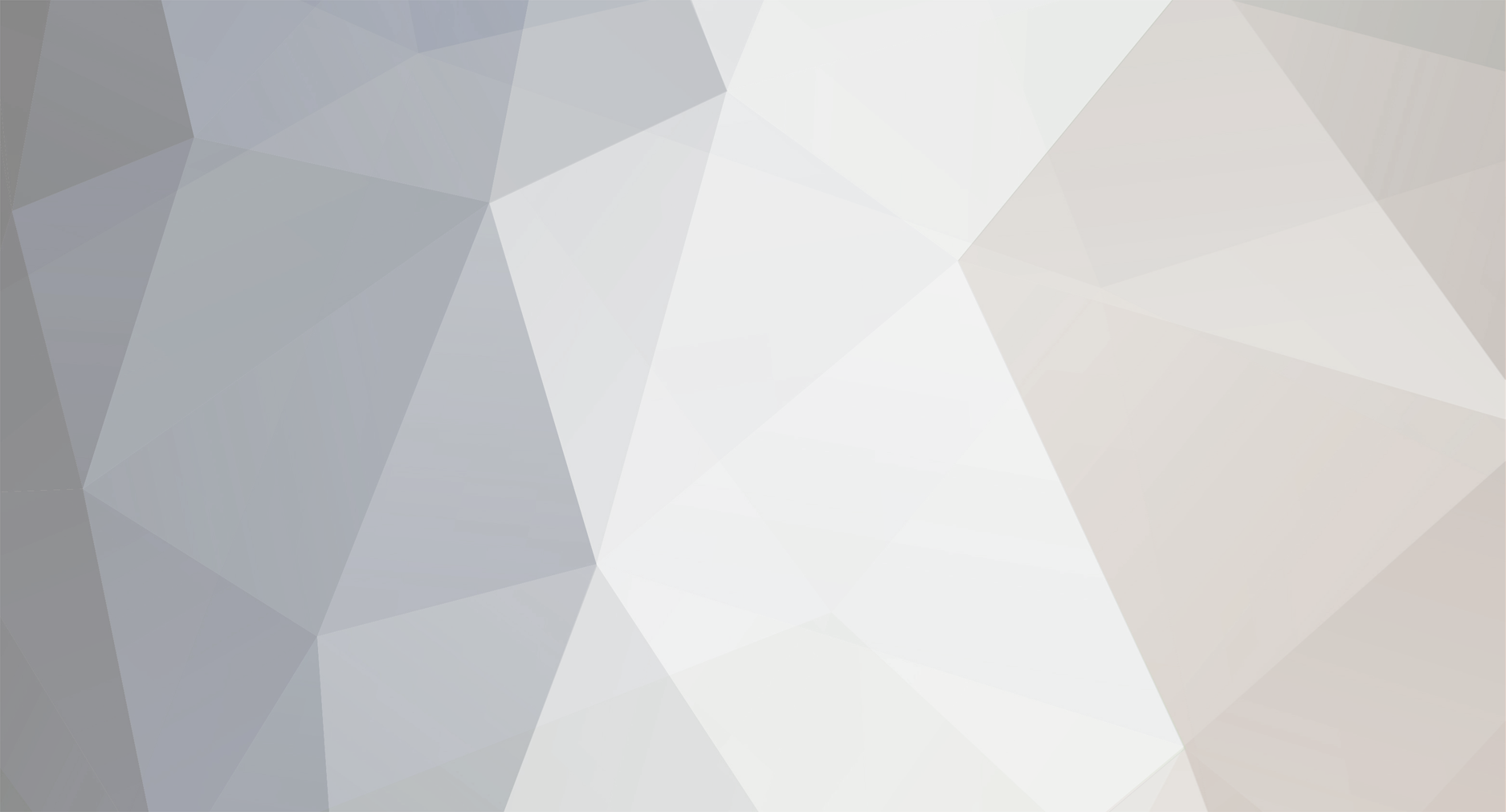
The Little Guy
-
Posts
6,675 -
Joined
-
Last visited
Posts posted by The Little Guy
-
-
Then it must be one of the others, and I think that it is the 4th one messing up the comments...
$tag = '#0000ff'; $att = '#ff0000'; $val = '#8000ff'; $com = '#34803a'; $find = array( '~(\s[a-z].*?=)~', // Highlight the attributes '~(<\!--.*?-->)~s', // Hightlight comments '~("[a-zA-Z0-9\/].*?")~', // Highlight the values '~(<[a-zA-Z!?].*?>)~', // Highlight the beginning of the opening tag '~(</[a-zA-Z].*?>)~', // Highlight the closing tag '~(&.*?~', // Stylize HTML entities ); $replace = array( '<span style="color:'.$att.';">$1</span>', '<span style="font-style:italic;color:'.$com.';">$1</span>', '<span style="color:'.$val.';">$1</span>', '<span style="color:'.$tag.';">$1</span>', '<span style="color:'.$tag.';">$1</span>', '<span style="font-style:italic;">$1</span>', );
-
$html = '<?xml version="1.0" encoding="UTF-8"?> <root> <!-- People who are 25 years old --> <names age="25"> <name>Ryan</name> <name>Bill</name> <name>Bob</name> </names> <!-- People who are 45 years old --> <names age="45"> <name>Roy</name> <name>Jill</name> <name>Jimmy</name> </names> </root>'; $com = '#34803a'; echo preg_replace('~(<\!--.*?-->)~s', '<span style="font-style:italic;color:'.$com.';">$1</span>', $html);
I have the above regexp and it works for these lines:
<!-- People who are 25 years old -->
but it doesn't work for this one:
<!-- People who are 45 years old -->
What can I do to fix this so it works for both?
Visual Example: http://tests.phpsnips.com/phpLive/examples/misc/highlight.php
-
I have this method that puts files into a zip file from an array list, it looks like this:
public function zip($files = array(), $maxMB = 2, $flag = ZIP_NEW){ $this->memory_size = $maxMB * 1024 * 1024; $zip_id = $this->zip_id; $this->zip[] = new ZipArchive(); $this->zip_id++; if($flag == ZIP_NEW){ $open = $this->zip[$zip_id]->open("php://temp/maxmemory:$this->memory_size", ZipArchive::CREATE); $files = (array)$files; if(!empty($files)){ foreach($files as $file){ if(is_file($file)){ $this->zip[$zip_id]->addFile($file); } } } $this->zip[$zip_id]->close(); } $this->function_name = __FUNCTION__; return $this; }
I then download them like this:
public function download($download_data, $download_name, $type = DOWNLOAD_FILE){ header("Pragma: public;"); header("Expires: 0;"); header("Cache-Control: must-revalidate, post-check=0, pre-check=0;"); header("Content-Type: application/force-download;"); header("Content-Type: application/octet-stream;"); header("Content-Type: application/download;"); header("Content-Transfer-Encoding: binary;"); header("Content-Disposition: attachment; filename=$download_name;"); if($type == DOWNLOAD_FILE){ header("Content-Length: ".filesize($download_data).";"); if($download_data == PHP_MEMORY){ $handle = fopen(PHP_MEMORY."/maxmemory:$this->memory_size", 'r+'); echo stream_get_contents($handle); }else{ readfile($download_data); } }elseif($type == DOWNLOAD_STRING){ header("Content-Length: ".mb_strlen($download_data).";"); echo $download_data; } $this->function_name = __FUNCTION__; return $this; }
When I run this code:
$files = array("file1.php","file2.php"); $live->zip($files)->download(PHP_MEMORY, "download.zip");
It downloads the file, the only problem is, is that when I open the file I get an error saying the file can not be opened. I know when I actually save it to a file instead of in memory it works. So, I am not sure if it isn't working in the download portion or the create file portion, as I have never worked with php://temp before. Any thoughts on why this doesn't work when using php://temp?
-
I thought something like this would be sported in php:
php -l myfile.php
And then you could throw in the option to check different version, and then it would return which versions had errors and which did not.
-
Found this:
http://pear.php.net/package/PHP_CompatInfo
It seems to work, IDK if it is out dated or not though...
-
Is there any script or command line program that anyone knows of that can analyze a PHP script and recommend a minimum PHP version to be used?
Thanks!
-
I am trying to run the function "age", using the last line, I thought that is how I could to access that method, but that doesn't work.
<?php require_once '../phpLive/phpLive.php'; $live->myClass = (object)array( "name" => "Billy Bob Joe", "age" => function($dob){ // Run some code } ); echo $live->myClass->age("1986-07-22"); ?>
I get the following error when trying to do that.
Fatal error: Call to undefined method stdClass::age() in /xxx/new.plugin.php on line 9Any Ideas how I can access that method?
Thanks!
-
This may not be the best way, but it works.
$max = 15; $sql = mysql_query("select rand_num from staff"); $nums = array(); while($row = mysql_fetch_assoc($sql)){ $nums[] = $row['rand_num']; } $staff_number = false; if(count($nums) < $max){ while(true){ $num = mt_rand(1, 15); if(!in_array($nums)){ $staff_number = $num; break; } } } var_dump($staff_number);
-
I have a chain of methods, is there anyway to end the chain half way through if some particular event happens?
Here is an example (not the best but still an example):
<?php $age = $class->post("age")->callback(funciton(){ global $class; if($class->string() < 18){ // end the execution of the chain, doesn't execute toInt() } })->toInt(); ?>
-
-
Or, for $100 I will search Google for you.
-
haha, thanks, but I just figured it out!
<?php function something(&$var){ $var = "hello"; return true; } something($cat); echo $cat; ?>
-
I am making a function it returns one thing, but I would the like to put something into a variable to use outside the function.
for example, the third parameter in preg_match() sets a variable that you can then use later in your code, how can I create a parameter like that?
-
Needed to save the new client to an array, and read from that client
-
I have a socket server that is running on a while loop, the server listens for something to write to the stream, when something does write to the stream the server prints out the following:
Warning: socket_read(): unable to read from socket [107]: Transport endpoint is not connectedIt fails on this line: $read = socket_read($socket, $length); with the above error (found in method "hear")
What does the error mean, and how can I fix it? any thoughts would be great!
Server:
require_once 'phpLive.php'; $live->listen(function(){ global $live; if(($heard = $live->hear())){ echo "I heard: $heard\n"; } });
Class:
public function hear($socket_id = 0, $length = 1024){ if(!isset($this->sockets[$socket_id])) $socket_id = $this->socket_connect(); $socket = $this->sockets[$socket_id]; if(is_resource($socket)){ $this->allow($socket_id); $read = socket_read($socket, $length); if(is_string($read)){ $this->quick_string = $read; $this->function_name = __FUNCTION__; return $this; } } return false; } public function allow($socket_id = 0){ if(!isset($this->sockets[$socket_id])) $socket_id = $this->socket_connect(); $socket = $this->sockets[$socket_id]; socket_accept($socket); $this->function_name = __FUNCTION__; return true; }
Thanks!
-
I have been thinking about how a live radio stream works, and I have come to this conclusion:
A client software (Flash for example) sends a live stream to the server, the server writes it to a file, such as an mp3, then a second client software (again Flash for example) reads from the end of the file (or a few seconds before the end). If the max file size, say 50MB is reached old stuff from the stream is deleted while the new stuff is written.
does this sound like how a live radio stream works, or am I completely off?
Any thoughts are appreciated!
-
Wonderful!
Works like a charm!
Thank you!
-
Are you planning on accessing this script from a browser? HTTP doesn't work like that.
Nope, from a cli.
-
I am using shell_exec, and it runs a ping on a server, is there any way to flush the buffer of the shell_exec as the data is getting written to the buffer?
Because I sometimes ping a site 10 times and nothing get written until the function finishes, so is there anyway to write out the output as soon as it is available?
btw, this isn't only for doing a ping it is for other things as well.
-
how are 3 and 8 the same thing?
$something << 3;
$something * 8;
-
I was looking over some php code, and came across this:
$c=($c*$j)<<2;
I have never see << before, what does it mean/do?
-
any ideas?
does it maybe have to be a php.ini setting?
-
I have this in a method:
<?php public function hear($socket_id = 0, $length = 1024){ $fp = fsockopen("localhost", 5565, $errno, $errstr); if(!$fp){ echo "$errno: $errstr";exit; } $heard = fread($fp, $length); fclose($fp); return $heard; } ?>
It is then called like this:
<?php require_once '../phpLive/phpLive.php'; $live->listen(function(){ global $live; if(($heard = $live->hear())){ echo "I heard: $heard"; } }); ?>
listen is a while loop that calls the passed function over and over.
The problem I am having is when I run it I get this error:
10061: No connection could be made because the target machine actively refused it.
I open the port, on my computer and it still doesn't work! What is causing this?
-
OMG, Sorry I found it!
echo htmlentities("<span>hello</span>", ENT_COMPAT, "ISO-8859-1", false);
multi line and single line.
in Regex Help
Posted
what about doctype?
<!DOCTYPE