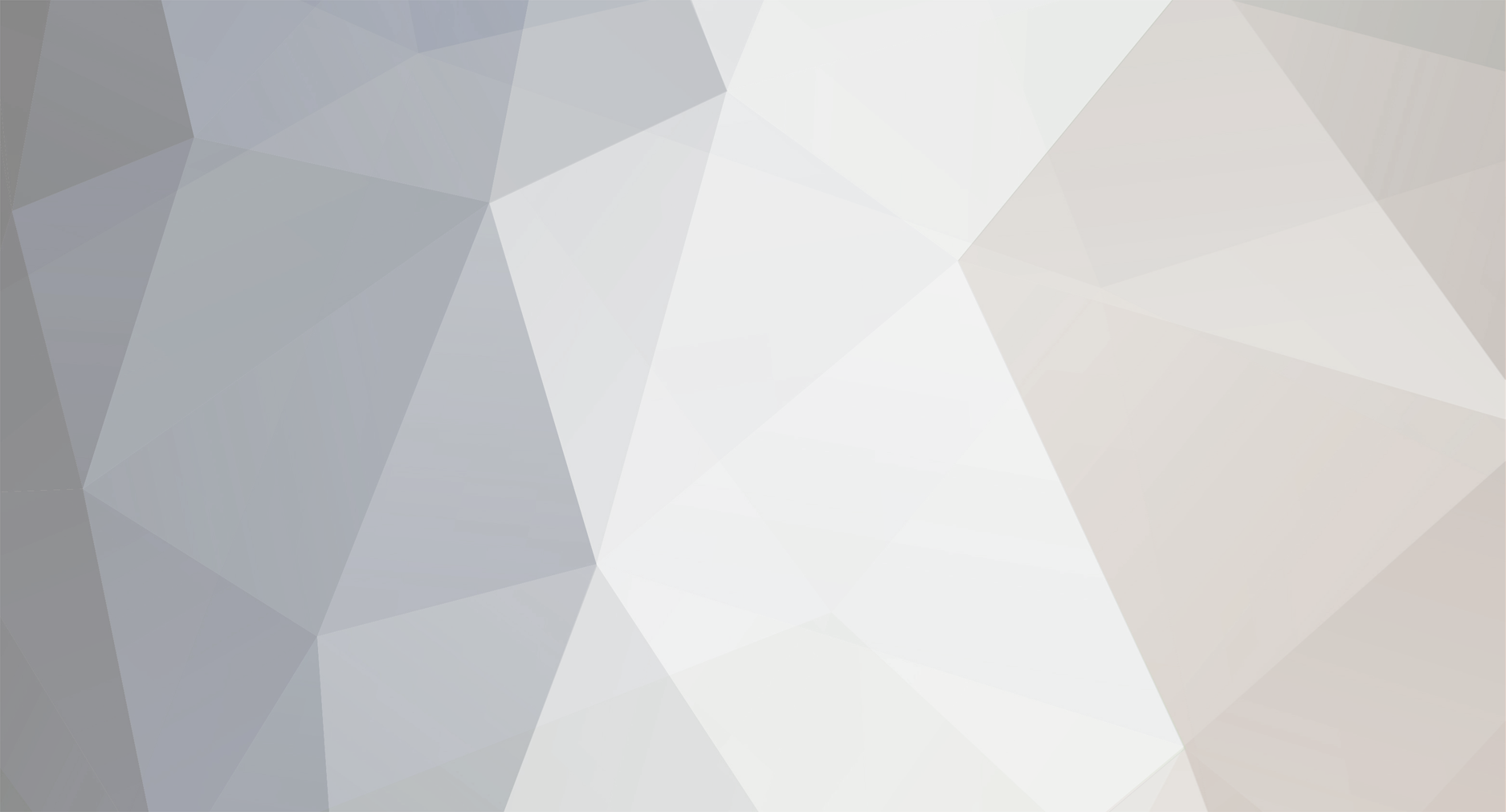
The Little Guy
-
Posts
6,675 -
Joined
-
Last visited
Posts posted by The Little Guy
-
-
Give this a go:
select * from deals d join handsets h on(d.handset = h.handet_numeric) where h.manufacturer_id = 3 order by d.popularity desc limit 20;
you would want to index:
deals.handset
handset.handet_numeric
you may even want a doube index on:
handset.handet_numeric, handset.manufacturer_id
-
Try this, it looks like you are missing a pair of parenthesis, and a limit
I am not sure if you can have a sub select inside of an in, but if you can I would assume it would look like this.
UPDATE x_orders SET OrderStatus = 1 WHERE OrderID IN( ( SELECT * FROM x_orders INNER JOIN x_payments ON x_orders.OrderID = x_payments.OrderID WHERE (x_payments.PaymentComplete = 1) AND (x_orders.OrderStatus = 0) limit 1 ) );
-
Do you have any heavy queries running?
mysql> show full processlist;
Do your servers get too much traffic to handle?
tail -f /usr/weblogs/access.log
Do an explain on all your queries, and make sure they are using an index, if not modify the query or create an index that the query can use. if it is using an index, can you make the index better?
-
you don't change max size through phpmyadmin, it is done in your ini file, you need to change your php ini file to allow for larger imports, and you will need to change the php max memory in there too.
Once you save your ini file, shut down Apache and start it back up, your settings should not take affect.
-
Then you need to wrap this is yet another SELECT.
or a temporary table
-
You can, it is not possible. Auto Increment increments, it does not deincrement.
-
Because an update query is in this format:
$lyhene = mysql_real_escape_string($_POST['lyhene']); $nimi= mysql_real_escape_string($_POST['nimi']); $id = (int)$_POST['id']; $query = "update tolkit set lyhenne = '$lyhene', nimi = '$nimi' ... where id = $id;";
-
here is basically how I like to do it:
(untested)
<?php function between($min, $max, $str){ if(strlen($str) >= $min && strlen($str) <= $max) return true; return false; } function post($key, $default = ""){ if(isset($_POST[$key])) return $_POST[$key]; return $default; } $title = post('title'); $comment = post('comment'); $description = post('description'); $errors = array(); if(!between(3, 20, $title)){ $errors[] = "Title is an invalid length)"; } if(!between(10, 1000, $comment)){ $errors[] = "Comment is an invalid length)"; } if(!between(3, 150, $description)){ $errors[] = "Description is an invalid length)"; } if(count($errors) > 0){ implode("<br />", $errors); }else{ $title = mysql_real_escape_string($title); $descr = mysql_real_escape_string($description); $comment = mysql_real_escape_string($comment); mysql_query("insert into comments (title, descr, comment) values ('$title', '$descr', '$comment')"); header("location: /comments.php#new"); exit; } ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <input type="text" name="title" maxlength="20" value="<?php echo $title; ?>" /> <input type="text" name="description" maxlength="150" value="<?php echo $description; ?>" /> <textarea cols="100" rows="5"><?php echo $description; ?></textarea> </form>
-
by running the following code, you will create two datetime columns.
1. is the time it was create
2. is the time it was last updated.
When you insert a new row, you need to use MySQL's now() function for the date_add column, the date_update column will automatically create a timestamp
alter table some_table add column date_add datetime null, date_updated timestamp NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP;
-
You would/could use it like this:
$q = "INSERT INTO blah(username, password) VALUES('$username', '$password', 1);"; $q .= "INSERT INTO blah2(first_name, last_name, user_id) VALUES('$first_name', '$last_name', last_insert_id());"; $r = mysqli_multi_query($db, $q);
-
btw, mysql and php both have the LAST_INSERT_ID() function, they both return a last insert id from an auto increment field.
-
Save it to a mysql variable like this:
$q = "INSERT INTO blah(username, password) VALUES('$username', '$password', 1);"; $q .= "set @user_id = (SELECT user_id FROM blah WHERE login_name='$login_name' limit 1);"; $q .= "INSERT INTO blah2(first_name, last_name, user_id) VALUES('$first_name', '$last_name', @user_id);"; $r = mysqli_multi_query($db, $q);
-
You should store the phone numbers as int's, and format the data when displaying it.
-
your queries need semi colons at the end of them, notice my code. Also your first query has more values than columns
$q = "INSERT INTO blah(username, password) VALUES('$username', '$password', 1);"; $q .= "SELECT user_id FROM blah WHERE login_name='$login_name';"; $q .= "INSERT INTO blah2(first_name, last_name, user_id) VALUES('$first_name', '$last_name', '$user_id');"; $r = mysqli_multi_query($db, $q);
-
insert into all (firstname, lastname, '2011-09-10', info) SELECT firstname, lastname, date, info FROM all WHERE date = '2011-08-30'
Just realized the above has a tiny error
insert into all (firstname, lastname, date, info) SELECT firstname, lastname, '2011-09-10', info FROM all WHERE date = '2011-08-30'
-
Something like this:
mysqli_multi_query($db, " insert into mytable(col1) values ('awesome'); select * from mytable where col1 = 'awesome'; insert into anothertable (col1) values ('last query'); ");
-
It outputs 1 chunk that is sent to the browser.
It actually outputs one thing, but that can make multiple requests, such as: images, css, javascript, flash, audio, video, xml, etc.
Steps (I believe, correct me if I am wrong or missed something):
- Client goes to your website - Browser makes request to the dns server - The dns sever converts to ip - the ip is found and it is considered an http request - your web server (probably Apache) intercepts the request since it is listening on port 80 - Apache uploads data (when using: <input type="file">) it is then put into a tmp folder - When Apache loads the file requested, it checks for the file extension - If there is a if there is something associated with that extension, and the plugin is installed Apache sends the request to the program to do the work - The program (php in our case) takes the file and runs it through php doing what ever the php script tells it to do. - When the script is complete html is sent back to Apache, and Apache then sends it back out to the web to the requesting client
-
Nightslyr
for inserting data into your database I am using strip_tags and addslashes, but do u think mysql_real_escape_string() is best?
of course mysql_real_escape_string is best
It was't added for kicks and giggles...
Always, Always, Always, Always, Always, Always, Always, Always, Always, Always, Always, Always, Always, Always use mysql_real_escape_string when inserting strings into a database.
When not using strings you can do this:
<?php echo $int = (int)"12.123's"; // $int = 12 echo "<br />"; echo $float = (float)"34.234"; // $float = 34.234 echo "<br />"; echo $bool = (bool)"kitty"; // $bool = 1 echo "<br />"; echo $bool = (bool)null; // $bool = 0 ?>
-
First, I can see that your checkboxes are not inside the form.
-
You want to do a insert into ... select from.
It would look something like this:
insert into all (firstname, lastname, '2011-09-10', info) SELECT firstname, lastname, date, info FROM all WHERE date = '2011-08-30'
-
Always good to add an extra column, test that (with an equality, make sure there all the rows "match"), and then drop the old one.
That is exactly what I did (b4 I read this post)!
Thanks!
-
Come to think of it, I would create a temporary table (mostly because I like them)
The query would looks something like this:
create temporary table temp_dates (the_date, temp); insert into temp_dates (the_date, temp) select Date, TempMax FROM wx_daily ORDER BY TempMax DESC LIMIT 10; select * from temp_dates order by the_date desc limit 1;
-
first convert your dates to real mysql dates (YYYY-MM-DD).
second, your query would then look like this:
SELECT Date, TempMax FROM wx_daily ORDER BY TempMax DESC, Date DESC LIMIT 10
This probably won't work for the dates format you have.
-
After doing those stress test, my data says the two small queries ran 2 times faster then the one larger query.
Results:
Been to every forum on the internet, no one has a clue. Please help!
in PHP Coding Help
Posted
Not sure if I under stand 100%, but give this a try: