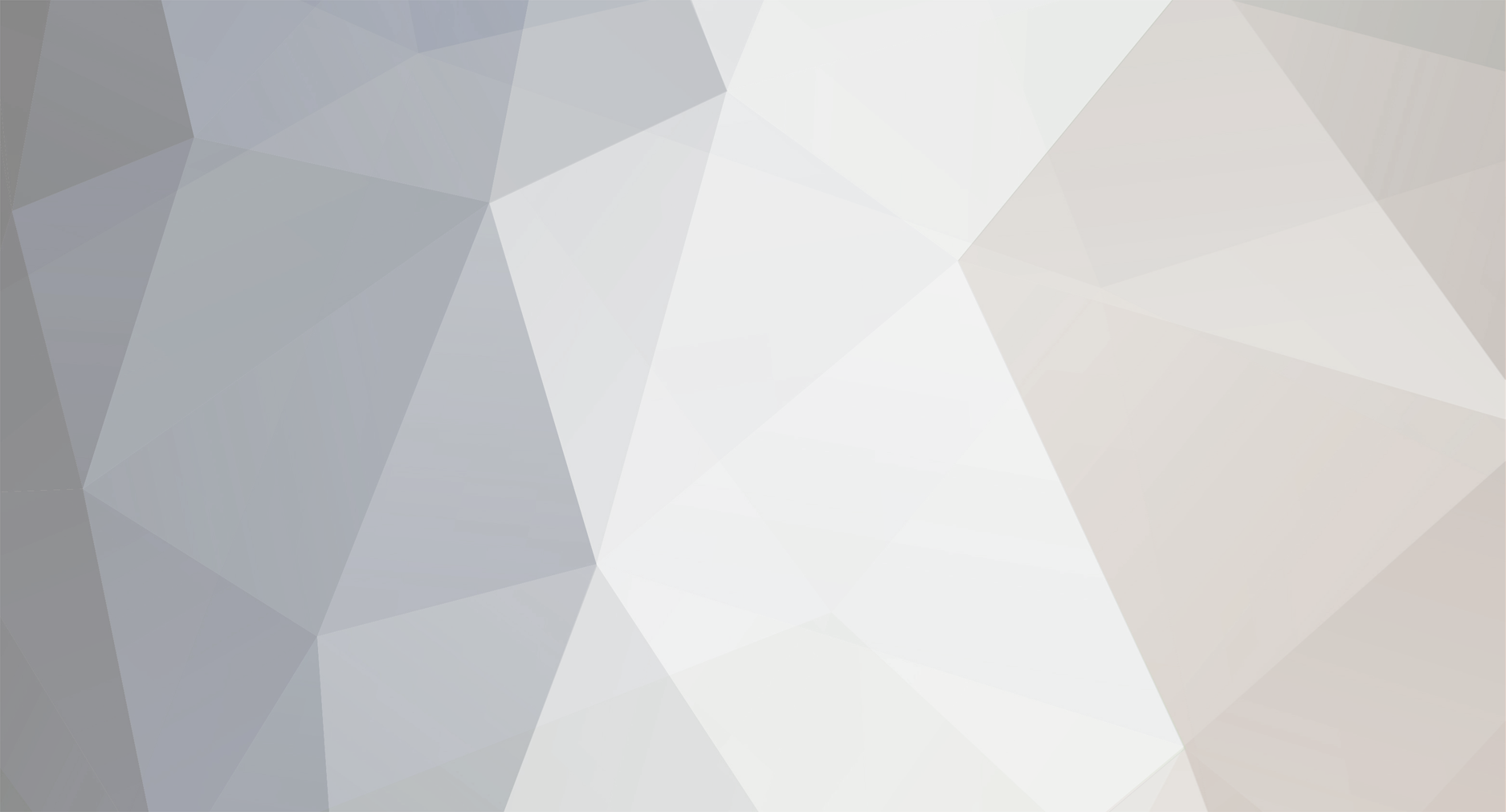
ozfred
Members-
Posts
20 -
Joined
-
Last visited
Never
Everything posted by ozfred
-
<URL: http://www.jibbering.com/faq/#FAQ4_15 >
-
Insert in a javascript array the elements of a php array
ozfred replied to worldworld's topic in Javascript Help
The syntax for a javascript Array literal is: var anArray = [ 'element 0', 'element 1', 'element 2', ... ]; And so on... -
You need to start by saying what you are testing for. You seem to be testing a phone number, but there are many different formats for that. What are you actually trying to do?
-
You are assigning the value true, rather than checking it (i.e. use "==" not "="). It is simpler and less error-prone to write: if (document.getElementById("check").checked)
-
<URL: http://www.paypal.com/cgi-bin/webscr?cmd=p/pdn/paypal-solutions-outside >
-
That's just the way they work. You can use window.onload to reset the buttons to the default using: <body onload="document.someForm.reset();" ... > which will reset the entire form. If you want to just set the first button to checked: <body onload="document.someForm.buttonSetName[0].checked = true;" ... >
-
Alerting a (yes or no) option before deleting?
ozfred replied to sync2007's topic in Javascript Help
You need something like this in your form: <form ... onsubmit="return confirmSubmit();" ... > And the confirmSubmit function is: function confirmSubmit(){ return confirm('Do you really want to submit the form?'); } -
<URL: http://www.jibbering.com/faq/#FAQ4_16 >
-
Yes, IE is being strictly standards compliant for a change. The onchange event is supposed to fire when the element loses focus, so when you click on the button, nothing happens until you click somewhere else and cause the button to lose focus. Firefox and other browsers do onchange (for radio buttons) as if it were an onclick. The solution is to use onclick and test the button status, something like: <input type="radio" onclick="if (this.checked){ /* do something */ }" ... > Or to test the status in the function called by onclick: HTML: <input type="radio" onclick="foo(this);" ... > Script: function foo(el) { if (el.checked) { /* el is checked, do something */ } else { /* el isn't checked, do something else */ } }
-
This should get you going: <url: http://www.javascripttoolbox.com/lib/dynamicoptionlist/ > Or search comp.lang.javascript for 'dynamic option list', a link is in my signature.
-
Wrap the code provided by mainewoods in a script element that is placed before the one that loads the script file. Remove the variables from the script file. <script type="text/javascript"> // mainewoods code here </script> <script type="text/javascript" src="ticker.js"></script>
-
If all you want to do is remove the second ?, use a regular expression before any other processing of the search string. try: var fullS = window.location.search.substring(1).replace(/\?/g,''); but be careful: that will replace all instances of "?" in the query string.
-
Which forum? comp.lang.javascript is very busy and they offer the best help you will get about javascript, bar none: <url: http://groups.google.com.au/group/comp.lang.javascript/topics?lnk=gschg&hl=en > That would appear to be invalid HTML - a tr must have a tbody or table parent, it can't have a form as its immediate parent. That code will run once and once only: when the page loads. You need to attach it to the submit event of the form, how does the form get submitted? e.g. <form name="form" action="https://www.paypal.com/cgi-bin/webscr" method="post" onsubmit=" this.amount.value = document.form1.amount.value; "> <input type="hidden" name="amount" value=""> Should do the trick, but without knowing how you are submitting the hidden form I can't guarantee that. -- Fred Javascript FAQ: <url: http://www.jibbering.com/faq/ >
-
If all you want is a reference back to the element that fired the event, you don't need to pass any paramters: ... thisrow.onclick = foo; ... function foo(){ var thisrow = this; } foo's this keyword will be set as a reference to the element that called it. -- Fred Javascript FAQ: <URL: http://www.jibbering.com/faq/ >
-
You meant only the "outer" rows: every table has a rows attribute that returns a collection of that table's rows, so: var theRows = document.getElementById('data').rows; Tough, eh? Try the W3C site and read the W3C DOM 2 HTML spec, or use the Mozilla DOM reference: W3C DOM 2 HTML Specification: <URL: http://www.w3.org/TR/DOM-Level-2-HTML/ > Mozilla DOM reference: <URL: http://developer.mozilla.org/en/docs/Gecko_DOM_Reference > -- Fred Javascript FAQ: <URL: http://www.jibbering.com/faq/ >
-
The default value of the display attribute for TR elements in CSS2 compliant browsers is "table-row". IE is not compliant, so it uses 'block'. Toggle your row's display attribut between 'none' and '' (empty string). That will allow it to return to its default, be it 'block' or 'table-row' or whatever. Some other tips: Store references in a local varaible and re-use them, that way you only get them once and significantly reduce the amount of code and its execution time (though you may not notice the time difference, your users may well appreciate the faster download times). Abbreviate the display function to: function evaluate() { var sel = document.getElementById("paidthrough"); var val = sel.options[sel.selectedIndex].value; var bankrow = document.getElementById("bankrow"); bankrow.style.display = (2 == val)? '' : 'none'; } Even better is if you change the onchange code to: <select id='paidthrough' name='paidthrough' onchange="evaluate(this);"> Your 'evaluate' function becomes: function evaluate(sel) { var val = sel.options[sel.selectedIndex].value; var bankrow = document.getElementById("bankrow"); bankrow.style.display = (2 == val)? '' : 'none'; } -- Fred Javascript FAQ: <URL: www.jibbering.com/faq/ >
-
There is no need to initialise temp with new Array() since the very next line initialises it as an array anyway. Whatever value you assign it befor then is irrelevant, so just use: var str = "this&is&exmaple&string" var temp = str.split('&'); //creates an array with each element split on & or even var temp = "this&is&exmaple&string".split('&'); -- Fred Javascript FAQ: <URL: http://www.jibbering.com/faq/ >
-
IE's support for the W3C DOM is patchy, especially in regard to select elements. Just set the select element's properties directly; select.name = 'compChoice'; To insert options, use new Option() . <URL: http://www.quirksmode.org/js/options.html > -- Fred Javascript FAQ: <URL: http://www.jibbering.com/faq/ >
-
Don't give the button a name unless you want its value to be submitted too. If you call it "submit" you will mask the form's submit method, naming it Submit is very close to doing that. -- Fred comp.lang.javascript FAQ:<url: http://www.jibbering.com/faq/ >
-
The content of a form control or similar HTML element is always returned as a string. That depends on what you mean by "validating the numbers". isNaN works exactly as described in the ECMAScript Language Specifiction. If you want to check that a string consists of only digits, use a regular expression: if ( /\D/.test(someField.value) { /* value contains non-digit characters */ } You will get better help in regard to javascript in a real javascript forum: news: comp.lang.javascript <URL: http://groups.google.com.au/group/comp.lang.javascript/topics?lnk=gschg&hl=en > -- Fred Javascript FAQ: <URL: http://www.jibbering.com/faq/ >