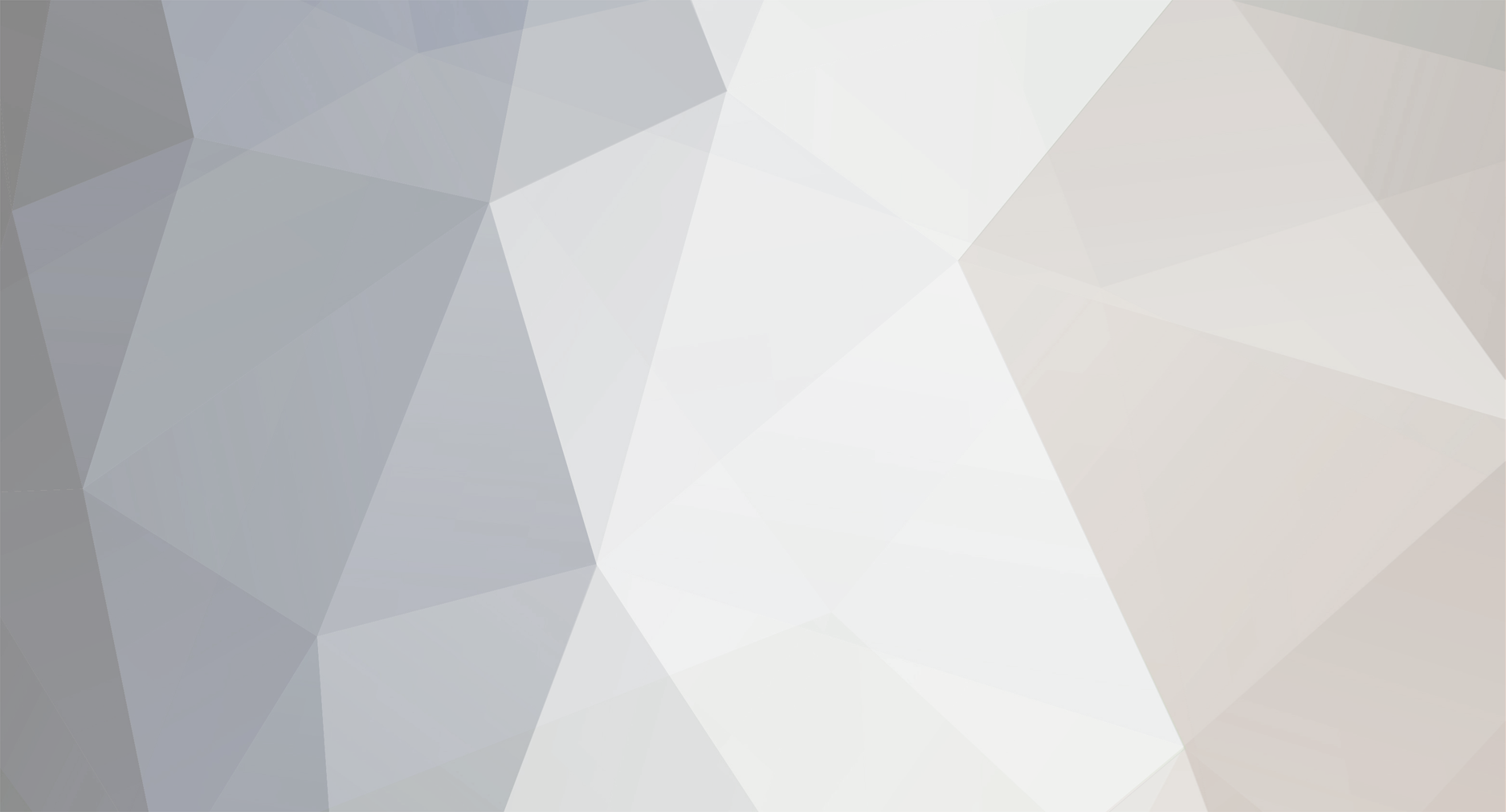
mpharo
Members-
Posts
221 -
Joined
-
Last visited
Never
Everything posted by mpharo
-
if($username==NULL{???????????? Won't work :( Thanks.
mpharo replied to Technex's topic in PHP Coding Help
<?php if(!$_POST['username']){ $errorusernamenull=1; echo 'Bad username'; } if (isset ($_POST['username']) AND ($_POST['password'])){ $username = $_POST['username']; ?> I tested and verified it is working on my end.. -
if($username==NULL{???????????? Won't work :( Thanks.
mpharo replied to Technex's topic in PHP Coding Help
can you post your entire page code? -
if($username==NULL{???????????? Won't work :( Thanks.
mpharo replied to Technex's topic in PHP Coding Help
hmm... after lookin at the code you used it should work. You are checking the POST and not the variable my mistake with the last post. I tested it on mine and it is working. -
if($username==NULL{???????????? Won't work :( Thanks.
mpharo replied to Technex's topic in PHP Coding Help
You need to take out the: $username = ''; $password = ''; Once you do that it is no longer a Null value. Variables in PHP do not need to be declared. -
if($username==NULL{???????????? Won't work :( Thanks.
mpharo replied to Technex's topic in PHP Coding Help
I see what the problem is, You have an nested If statement which sets the variable before it checks for a null value. if (isset ($_POST['username']) AND ($_POST['password'])){ $username = $_POST['username']; then you are checking for a null value, but if $POST['username'] is not set it just bypasses the rest of it...You need to close the first if statement and add an Else for the second one, or make it unnested. if(is_null($username)){ $errorusernamenull=1; echo 'Bad username'; } if (isset ($_POST['username']) AND ($_POST['password'])){ $username = $_POST['username']; } -
if($username==NULL{???????????? Won't work :( Thanks.
mpharo replied to Technex's topic in PHP Coding Help
your missing a closing } for the first If statement and a ) in the second one. it is prolly there just not posted in the code. Try doing this: $username = ''; $password = ''; if (isset ($_POST['username']) AND ($_POST['password'])){ $username = $_POST['username']; if(is_null($username)){ $errorusernamenull=1; echo 'Bad username'; } } -
Your question is worded kinda funny but I am assuming you want to grab the data between BEGIN and END and put it into an array? You can use the substr(); function to do this. <?php $var=BEGIN09840918END; $out=substr($var, 5, -3); echo $out; ?> This should get you the result you are looking for.
-
Ok I looked at your site. You need to do is create a session variable with the value of the user logged in. then you can take the session variable holding the username from page to page. <?php $query=mysql_query("SELECT * FROM users WHERE username='$_POST[username]' AND password='$_POST[password]' ") or die(); $sql=mysql_fetch_array($query); session_start(); $_SESSION['username']=$sql[username]; echo "you are logged in as: $_SESSION['username']"; ?>
-
this topic kinda got off topic and me lost...can you repost the problem your having? along with any errors?
-
[SOLVED] a problem with "php sessions" and W3C validity.
mpharo replied to flunn's topic in PHP Coding Help
try putting your textarea in its own line. print '<p> I am trying '; print '<textarea name="comments" rows="1" cols="5"></textarea> '; print 'write a valid form.</p>'; -
In order for you to use session variables you need to call the session_start(); function first. Also you can eliminate a step by checking if your session variable is initialized. <?php session_start(); ?> <title><?php echo "$name"; ?></title> <link rel="stylesheet" href="style.css" type="text/css" /> <body bgcolor="#64748B"> <center> <table width="850" border="0" cellspacing="0" cellpadding="0"> <tr bgcolor="#26354A"> <td width="514" nowrap="nowrap"><p align="right" class="logo">VirAir International <span class="tagline">Paving The Skies In Flight Simulator </span></td> <td width="50" height="70" nowrap="nowrap" class="logo"><p class="logo"> </td> <td width="286"><?php if (!$_SESSION['username']){ $_SESSION['username'] = $row['username']; ?> <form action="<?php print "$PHP_SELF"; ?>" method="POST"> <table border="0" cellpadding="2" cellspacing="2"> <tr> <td class="flights">Username: </td> <td><input type="text" name="username" value="" /> </td> </tr> <tr> <td class="flights">Password: </td> <td><input type="password" name="password" value="" /> </td> </tr> <tr> <td class="flights"> </td> <td><input type="submit" name="userlogin" value="Login" /></td> </tr> </table> <?php } else{ ?> <a href="index.php?page=modiinfo">Modify Information[/url] | <a href="index.php?page=modipass">Modify Password[/url] | <a href="index.php?page=logout">Logout[/url] <?php } ?> </form> </td> </tr> </table> <table width="854" border="0"> <td class="NavTop"> <?php if (!$_SESSION['username']){ //Non Logged In Members Below ?> <a href="index.php?page=signup">Sign Up![/url] <?php } else{ //Logged Members Below! ?> <a href="index.php?page=postflight">Post Flight[/url] <?php } ?> </td> you may need to modify it a little but you should get the picture.
-
Why even make it that complicated....leave it all on index.php.....after login create a session variable then check to see if it exists. If it does then the person is logged in if it isnt then display the login form.
-
What he is saying is that he wants to do a check to see if it is already set, if it is then update if not then insert a new record. You can use the following as some test code. <?php if(isset($_POST['save'])) { $heading = $_POST['heading']; $updates = $_POST['updates']; if(!get_magic_quotes_gpc()) { $heading = addslashes($heading); $updates = addslashes($updates); } $select=mysql_query("SELECT * FROM news WHERE heading='$heading' AND updates='$updates'"); $rows=mysql_num_rows($select); If($rows==0){ $query = " INSERT INTO news (heading, updates) ". " VALUES ('$heading', '$updates')"; mysql_query($query) or die('Error ,query failed'); include 'library/closedb.php'; echo "Inserted '$heading' added as a new record"; }Else{ $query = " UPDATE news updates='$updates' WHERE heading='$heading'"; mysql_query($query) or die('Error ,query failed'); include 'library/closedb.php'; echo "'$heading' Updated with the current information"; } } ?> I am not sure what you key is on that table so you WILL need to modify this code.
-
All you will need to do is setup a query to get all of category. Then you will setup another query inside of your loop to query for all the results for that single category for each subcategory. After this query you will then echo out the javascript to add each result to the array. The array key is the category and the array value is the subcategory.
-
Thorpe is right, thats the best way to do it with what your needs are if you cant refresh the page.
-
What you have is much more complicated than what you need...try something like this... <?php //testpage.php $select=mysql_query("SELECT * FROM table") or die(mysql_error()); echo "<form name=\"category\" method=\"post\" action=\"testpage.php\">"; echo "Category 1: <select name=\"category1\" onChange=\"javascript: document.category.submit()\">" . "<option value=\"\" selected>--Category 1--<\option>"; while($cat1=mysql_fetch_array($select)){ echo "<option value=\"$cat1[category]\""; If($_POST[category1]==$cat1[category]) { echo " selected"; } echo ">$cat1[category]</option>"; } echo "</select></form>"; If ($_POST[category1]) { $select2=mysql_query("SELECT * FROM table WHERE category='$_POST[category1]' ") or die(mysql_error()); echo "SubCategory: <select name=\"category2\">" ."<option value=\"\" selected>--SubCategory--</option>"; while ($cat2=mysql_fetch_array($select2)) { echo "<option value=\"$cat2[category2]\">$cat2[category2]</option>"; } echo "</select>"; }Else{ echo "<select name=\"category2\"><option value=\"\">--SubCategory--</option></select>"; } ?> You will need to tailor this to your environement, it is a little sloppy im sure you can clean this up, I just did it quick on the fly, but you should get the jist of it.
-
a snippet of your current code would be most helpful in generating code that you can use.
-
Thats very easy to do with PHP and Javascript, you dont need to use AJAX. All you do is create a form then onchange submit the form, then in the second select box you check for a non default value and if so then query for the possible subcategories and display the results. If you post an example I can show you.
-
Looks good to me, what seems to be the actual problem?
-
If you are getting the file to show up but has a 0 size, I have found that to be a permissions error, where the person your grabbing the file as dosent have access to the file or only has read only access. I havent looked through your code yet, but be sure you have complete access to that file to grab it, and then to write it.
-
Im just shooting in the dark here.... <![endif]--> Do an echo of $strLastName and see if it is displayed on the page....if it is not on the page it is being added in the header or body of the e-mail message.
-
It will be a lot easier in your code if you just put all the data into an array instead of specifying each variable is a result. It will also speed up the page or script when it runs. It is also less code to use, try using something like this. <?php while($sql=mysql_fetch_array($select)) { echo "ID: $sql[id] Offspring: $sql[offspring] " . "Country: $sql[country] Race: $sql[race] Prize: $sql[prize]"; } ?>
-
$select = mysql_query("SELECT * FROM table") or die(mysql_error()); while($sql=mysql_fetch_array($select)) { If ($result==$sql[column1]){ echo ""; echo "$sql[column2]"; }else{ echo "$sql[column1]"; echo "$sql[column2]"; } $result=$sql[column1]; } Untested so im not sure if this will work or not.
-
Reload outside page onClick of submit button
mpharo replied to tcarpenter9's topic in PHP Coding Help
if the frame has a name you can refresh it with javascript using something like this... <a href="javascript:location.reload();">Refresh the page</a> -
[SOLVED] Use php to read and display text from html page
mpharo replied to WEvans's topic in PHP Coding Help
I stand corrected then, I didnt catch that part in the original post.