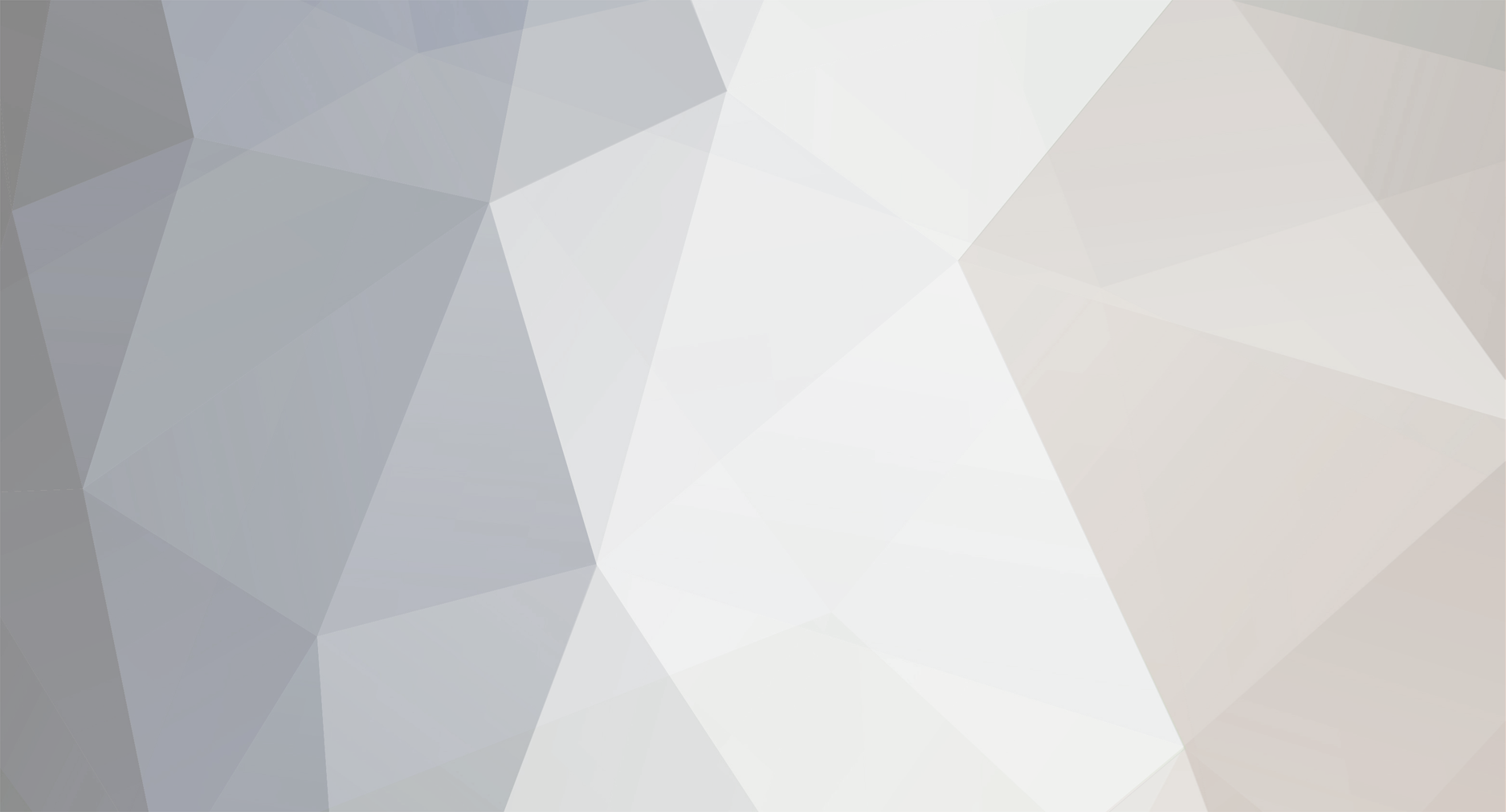
mpharo
Members-
Posts
221 -
Joined
-
Last visited
Never
Everything posted by mpharo
-
I do not see the $connection variable anywhere in the scripts... you will need to use the mysql_connect() function to variable $connection...
-
$select = mysql_query("SELECT * FROM table1"); while ($sql=mysql_fetch_array($select2)) { $select2 = mysql_query("SELECT * FROM table2 WHERE NUMBER=$sql[NUMBER]"); while($sql2=mysql_fetch_array($select2)) { echo $sql[HEADLINE] . " " . $sql2[CONTENT]; } }
-
You will need to use stdin variables instead of post, it will not work correctly unless you use stdin...
-
I am not sure if this was what you were looking for but... Post data is stored in an array called $_POST... You can do something like this... <?php foreach($_POST as $key=>$val) { echo "Key: $key Value: $val<br>"; } ?> This will output each post element. You can specify single post elements by a typicall array echo...ie... <?php echo $_POST['fieldname']; ?>
-
[SOLVED] Is there a more efficient way to code this than what I have?
mpharo replied to carlos1234's topic in PHP Coding Help
is using a database out of the question? a query would definatly simplify this... -
there is a s after the closing loop bt that is out of place...
-
Microsoft Internet Explorer (Browser) Microsoft states that the maximum length of a URL in Internet Explorer is 2,083 characters, with no more than 2,048 characters in the path portion of the URL. In my tests, attempts to use URLs longer than this produced a clear error message in Internet Explorer. Firefox (Browser) After 65,536 characters, the location bar no longer displays the URL in Windows Firefox 1.5.x. However, longer URLs will work. I stopped testing after 100,000 characters. Safari (Browser) At least 80,000 characters will work. I stopped testing after 80,000 characters. Opera (Browser) At least 190,000 characters will work. I stopped testing after 190,000 characters. Opera 9 for Windows continued to display a fully editable, copyable and pasteable URL in the location bar even at 190,000 characters. Apache (Server) My early attempts to measure the maximum URL length in web browsers bumped into a server URL length limit of approximately 4,000 characters, after which Apache produces a "413 Entity Too Large" error. I used the current up to date Apache build found in Red Hat Enterprise Linux 4. The official Apache documentation only mentions an 8,192-byte limit on an individual field in a request.
-
and also remember average grade is calculated differently than median grade... Average sum of elements / number of elements Median Step 1: Count the total numbers given. There are 5 elements or numbers in the distribution. Step 2: Arrange the numbers in ascending order. 1,2,4,5,7 Step 3: The total elements in the distribution (5) is odd. The middle position can be calculated using the formula. (n+1)/2 So the middle position is (5+1)/2 = 6/2 = 3 The number at 3rd position is = Median = 4
-
try this... //display all roof height vehicles when submit button isnt pressed if (!$_POST['N/ARoofHeight'] && !$_POST['Standard Roof'] && !$_POST['Medium Roof'] && !$_POST['High Roof']) { $RoofHeight = "N/A', 'Standard Roof', 'Medium Roof', 'High Roof"; } You also might want to avoid using spaces and slashes in names...
-
Your $plusOne variable is not increasing, therefore returning the same result each time. You will need to change the +1 year to include the $l variable... <?php $y = date('Y'); $l = 1; while ($l <= 10){ ?> <option value="<?php echo $y; ?>"><?php echo $y; ?></option> <?php $plusOne = strtotime(date("Y", strtotime($y)) . " +$l years"); $y = date('Y', $plusOne); $l++; } ?>
-
I forgot to copy for beginning form element, copy and paste the above again and it will work... also to make sure you are using <?php ?> and not just <? ?>...
-
<form method='post' action=''>Grade 1:<input name="grades[]" type="text" /> Grade 2:<input name="grades[]" type="text" /> <input type='Submit' name='Submit' value="Submit" /> </form> <?php //initalize the total $total = 0; //loop through the grades entered if ($_POST['grades']) { foreach($_POST['grades'] as $grade){ $total += $grade; } } //show the total echo $total; ?>
-
Typically you wouldnt use (!mysql_query($sql) you would just do (!$result1)....
-
Your array isnt initialized until you perform the post, you need to add an if statment to see if it is posted yet, then do it else do nothing...
-
probably going to see more of the code on the page...
-
You would need to store the username and passwords in the database, encrypt the passwords using a MD5 hash before you insert it. <?php session_start(); if (!$_SESSION['username']) { $username = strip_tags(addslashes($_POST[username])); $password = strip_tags(addslashes($_POST[password])); $passwordhash = md5($password); $select = mysql_query("select * from tlogin where username=$username and password=$passwordhash") or die(mysql_error()); $rows = mysql_num_rows($select); } if ($rows>0) { $_SESSION['username'] = $username; } else { echo "Login Failed!"; } ?> This is rough code, but it should get you on the right track...
-
in your form action you have $_SERVER['PHP_SELF'], which will return the page that it is being called from, so if you are on index.php it will return index.php. Which in looking at your login section at the top of the page is how you want it setup. Cookies are not a secure way to store login info, espically passwords, and from the looks of it your not even encrypting the password in the cookie. You should look at changing this to session based and not cookie based.
-
The way taquitosensei reccommended is a preferred method, as it only requires 1 SQL query and 1 loop.
-
you dont need to echo 2 times. <?php echo "<meta http-equiv=Refresh content=4;url=/marlon/photo/thumbs/delete_pic_tn.php?bin_data=$info[bin_data]>"; ?> or.... <?php echo "<meta http-equiv=Refresh content=4;url=/marlon/photo/thumbs/delete_pic_tn.php?bin_data=" . $info['bin_data'] . ">"; ?>
-
Hello, I am witing a new application that generates information daily. Right now I have it setup that the initial information is set for a start date and end date with an active and deactive flag. Basically what I need to do is query for all of the information that is greater than the start date and less than the end date where they are active. But the catch is that there is also a sunday-saturday flag to be set for each day. So each day where Monday is set to active and it is within the start date and end date I need to generate information, the Sunday thru Saturday flags can be changed in the middle of the duration. What I was thinking if doing was havin a daily script that runs that filters out the information for each day and then generates the report based on that information, that way if a monday is now deactive that was previously active I still have a record of all of the previous mondays that were active. In total this will generate about 1000 records per day and much less on the weekends. Is this the correct way to go about this? is there another way? I am open to ideas. Thanks in advance,
-
I am working on a new login scheme and am having a debate with a co-worker on how to properly secure a password in the database. My theory is to use a SSL cert to encrypt the information over the wire and then use the md5 encryption to store it in the database. He claims storing it in this method allows for decryption of the password if there is a SQL Injection hack. I say yes that it is possible to do so but as long as you protect the best you can aginst SQL Injects this is the best method to store the password. Any ideas??
-
your gonna hafta post the entire code and not just sections of it....
-
The way you have it setup it will only loop through 1 result and not them all...try this...it is not tested but you should be able to get it to work from here....