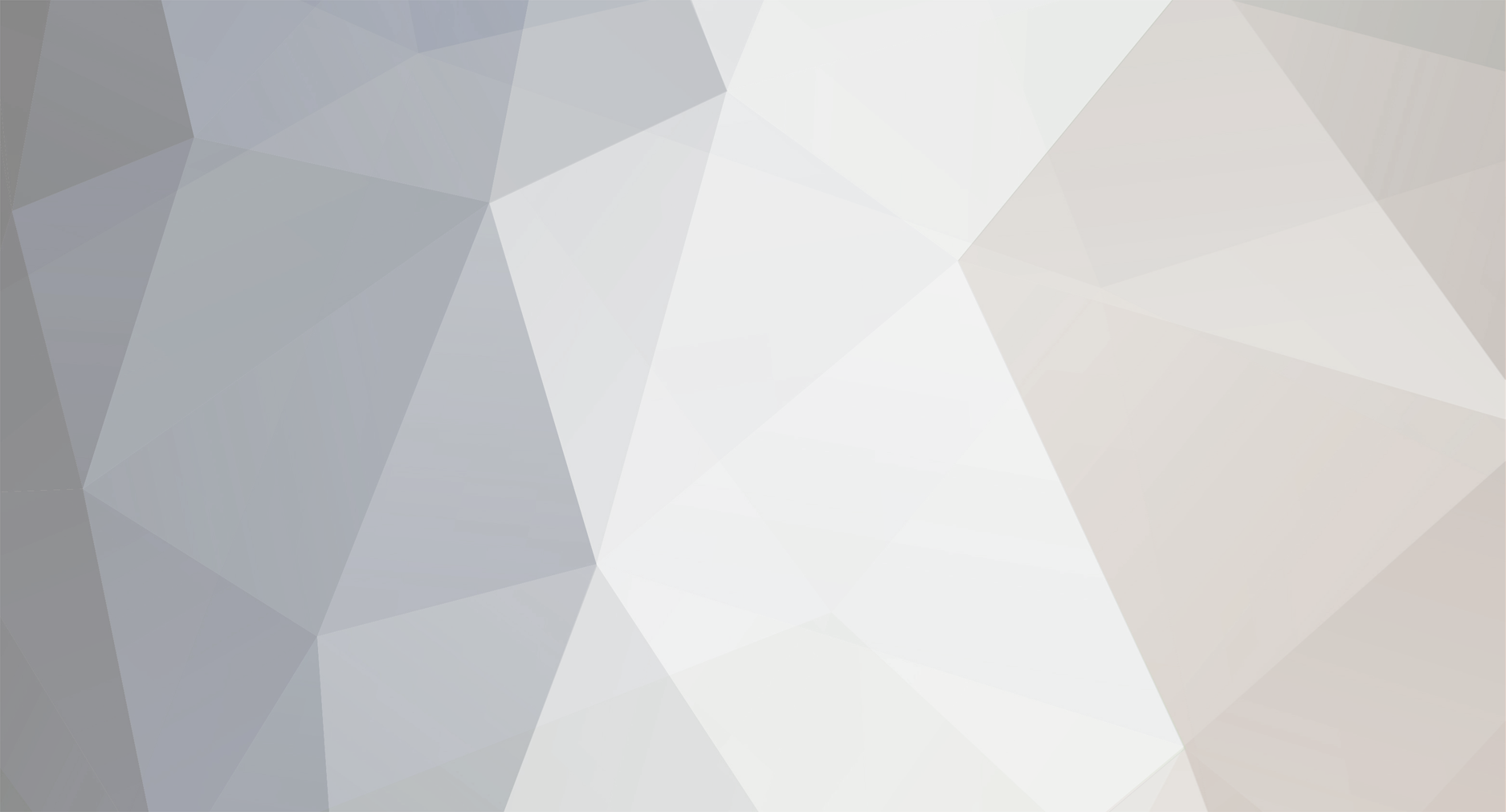
mpharo
Members-
Posts
221 -
Joined
-
Last visited
Never
Everything posted by mpharo
-
[SOLVED] Use php to read and display text from html page
mpharo replied to WEvans's topic in PHP Coding Help
So your information is stored in a database....it is much easier than what the previous post said... You just need to do something like this... //ASC will sort 1 to 100 DESC will sort 100 to 1 $select = mysql_query("SELECT * FROM table ORDER BY rank LIMIT 0, 100 ASC") or die(mysql_error()); while ($sql=mysql_fetch_array($select)) { echo "Rank: " . $sql[rank]; echo "User: " . $sql[user]; } You will obviously need to adapt it to your table structure, but you should get the idea. -
[SOLVED] Displaying multiple items from database
mpharo replied to wrathican's topic in PHP Coding Help
try this... $result = mysql_query($query) or die(mysql_error()); echo $result; you should get an error from your mysql server giving a reason as to why your query isnt working. -
[SOLVED] Displaying multiple items from database
mpharo replied to wrathican's topic in PHP Coding Help
fails with what error? -
Resource overhead of MySQL? it takes miliseconds to return a result and has less than 1% utilization on a low end server...there is no great overhead for a single query to grab one result??
-
what you want are $_SERVER variables... http://us.php.net/reserved.variables
-
[SOLVED] Displaying multiple items from database
mpharo replied to wrathican's topic in PHP Coding Help
mysql_fetch_row(); is used to return a single result, what you have is an array of results, so you need to use the mysql_fetch_array(); function... -
[SOLVED] Displaying multiple items from database
mpharo replied to wrathican's topic in PHP Coding Help
That error means that the variable that it is linked to is not a valid source...so either your query failed or did not return any results...or your have the wrong variable name in the function... -
your syntax looks fine, are you sure you have the correct column names and data types?
-
You need to set up an alias for that folder on the webserver...which if the folders are dynamic this will not work.... If they are dynamic you could use the scandir() function to get a directory listing... then you would put the folder that the user wants to goto into a variable and when they go there you just send them there via header() function.... example: directory: test sub-dir: stest1 sub-dir: stest2 $dir = scandir('/usr/local/www/apache22/data/test'); $i_total=count($dir); for ($i=0; $i<$i_total; $i++) { echo "<a href=\"http://192.169.0.1/$dir[$i]/\">" . $dir[$i] . "</a><br>"; } will give you an output of: stest1 stest2 as links and then they will take you to that directory... im not sure if this is what your looking for but you can also use the header() function to do something similar by populating the directory into a variable then use the header() function to send them to that folder.
-
[SOLVED] Displaying multiple items from database
mpharo replied to wrathican's topic in PHP Coding Help
-
[SOLVED] Displaying multiple items from database
mpharo replied to wrathican's topic in PHP Coding Help
That is a nasty query and I would not reccomend doing it that way...using my example above it is simple and straight forward, so if you need to hand this off to someone else or ask someone for help on this in the future also for you own benefit, it is very easy to interpret and go with... -
[SOLVED] Displaying multiple items from database
mpharo replied to wrathican's topic in PHP Coding Help
You can do it with one query... $query = mysql_query("SELECT proj_title, proj_decrip, proj_imagepath FROM project GROUP BY proj_title ASC"); echo "<table><tr><td>"; while($m = mysql_fetch_row($query)){ If ($title != $m[proj_title]){ echo "<table><tr><td colspan=\"3\">$m[proj_title]</td></tr><tr><td>$m[proj_decrip]</td><td>$m[proj_imagepath]</td></tr>"; }Else{ echo "<tr><td>$m[proj_decrip]</td><td>$m[proj_imagepath]</td></tr>"; } $title=$m[proj_title]; } echo "</table></td></tr></table>"; This is just to give you an idea of how you can do it, this code should work in your schema but it may not produce the results you want, give it a try and let me know if/how it works and what you need changed...or if you have any questions...Also this will loop through everything, I am not sure what the content of the fields are in your table, if you can post the result from one row in your table I can edit the code to return only 2 results for each heading... -
[SOLVED] Displaying multiple items from database
mpharo replied to wrathican's topic in PHP Coding Help
Can you post your table structure as well? also it would be much easier to see the whole page instead of just a section... -
[SOLVED] Displaying multiple items from database
mpharo replied to wrathican's topic in PHP Coding Help
You can do it from one table or from a collection of tables very easily....if you post some code and how you want it to work I would be happy to assist you... -
What you need to do if you resetting a session variable to another value is use the unset(); function to clear the value of it...then reset it to the value... <?php session_start(); if(isset($_SESSION['langues'])){ unset($_SESSION['langues']); $_SESSION['langues'] = $_GET['langues']; header("location:login.php"); exit(); }else{ echo "ERROR!"; } ?>
-
actully on any page where you using sessions (unless it is an embedded page) you need the session_start().... try using the unset($_SESSION['langues']); function instead of killing the session with session_destroy();
-
out of curiosity why wouldnt this work?
-
if you want straight 30 days or 7 days then just use the strtotime() function... date("Y-m-d", strtotime("last week")); will get you 7 days ago from today..... date("Y-m-d", strtotime("last month")); will get you this day last month...
-
yes you can, you just do it like you would any other variable $insert=mysql_query(INSERT INTO table (field) VALUES ('$_SESSION[var]' ) );
-
[SOLVED] Figure out which posts has been read and not read
mpharo replied to SkyRanger's topic in PHP Coding Help
if ($count==0 AND $postread==0) of if your trying to take it from your query if ($count==0 AND $sql[postread]==0) you can also use OR instead of AND... but why? -
[SOLVED] Figure out which posts has been read and not read
mpharo replied to SkyRanger's topic in PHP Coding Help
no problem, if you need anymore help you know where to get it, if this topic is solved please click the solved button at the bottom of the page...Glad I could help... -
[SOLVED] Figure out which posts has been read and not read
mpharo replied to SkyRanger's topic in PHP Coding Help
Another reason not to use cookies for something like this, is because most browsers have a max cookie size, once you reach a point it wont allow you to write to it anymore, this is because anyone could easily write a script that continues to loop and write values in acookie until you run out of disk space....not really harmful but a very big annoyance...the entires your going to have in a database like this are going to be relitavly small, I have a MySQL database with 2 million entries, the size is less than 5MB, it is all about how the data is stored, and these values are just simple text that take up hardly any space at all for a database....MySQL dosent have a limit for a DB size, but you can notice significant performance hits when your over the 10GB range....I havent done the tests, but there are a lot of good articles out there that do great testing on this stuff... -
[SOLVED] Figure out which posts has been read and not read
mpharo replied to SkyRanger's topic in PHP Coding Help
with one or 2 posts a cookie or session would work, but if you had a cookie with every one that you clicked on to mark as read, your cookie would become enormous very quickly, in your link every time you click on it your passing the same thing over and over....you need to setup 2 links one for if it is read then another if it isnt.... -
[SOLVED] Figure out which posts has been read and not read
mpharo replied to SkyRanger's topic in PHP Coding Help
I assume that you have register global variables turned off? otherwise this code wont work....but in your link you would just add something like this.... <a class=\"forummain\" href=\"officeview.php?oid=$row['oid']&post_read=true\">$outtitle</a> "; then in your officeview.php page you would do: If ($post_read){ $insert=blah blah blah } But if you dont have register globals turned off or your running php4.1 or greater you need to use the $_GET[] function to reference any of the variables passed in the url... -
[SOLVED] Figure out which posts has been read and not read
mpharo replied to SkyRanger's topic in PHP Coding Help
is : <a class=\"forummain\" href=\"officeview.php?oid=".$row['oid']."\">$outtitle</a> "; what a person will click on to take them to that post? if so what does officeview.php look like?