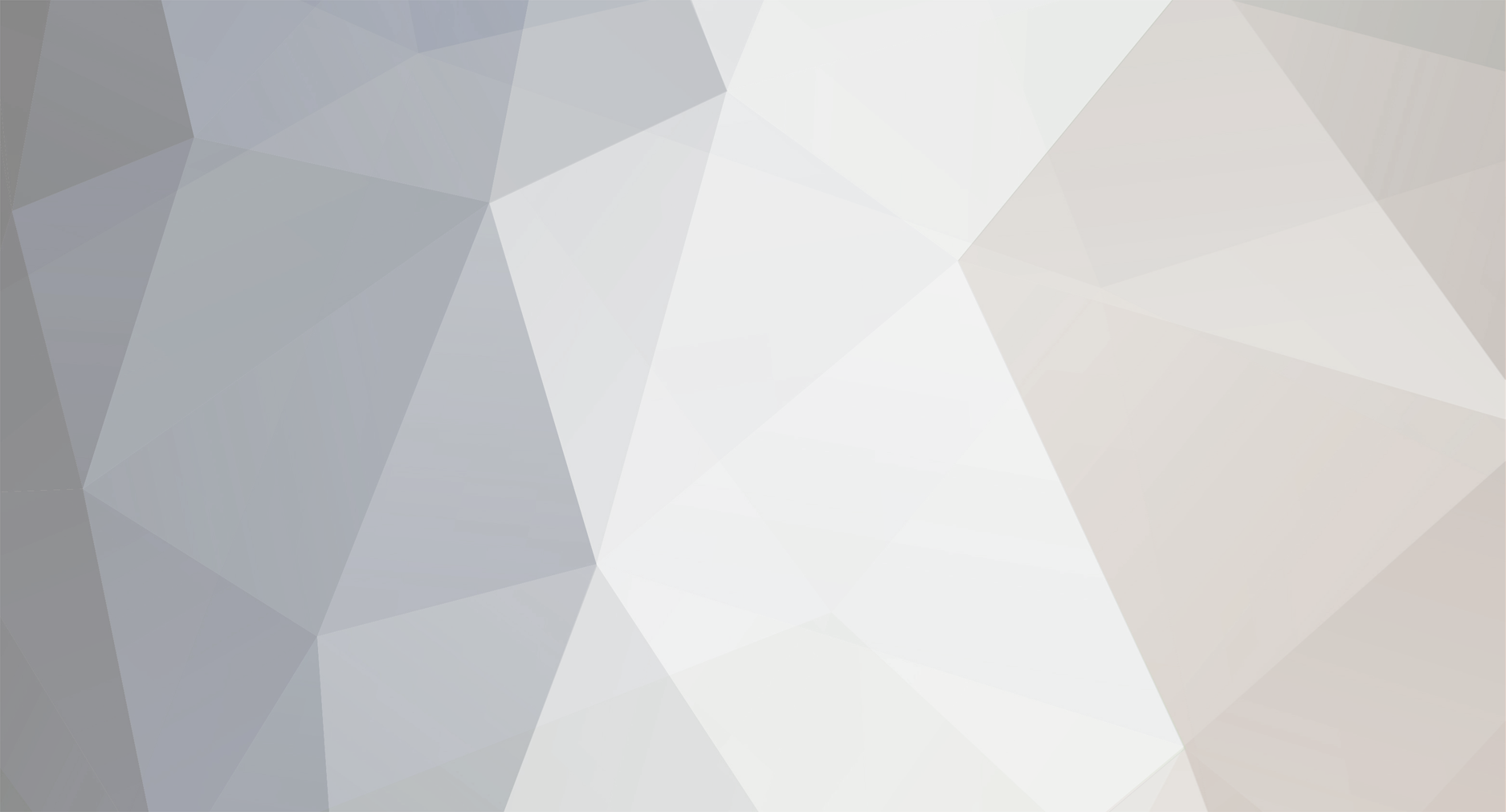
cluce
-
Posts
354 -
Joined
-
Last visited
Never
Posts posted by cluce
-
-
thanks for the quick reply but I solved it. I just took out the table border tags from the loop. and added like this.........
$display_block .= "</table>";
-
can someone tell me how to fix this table thats in the attachment. before I pull all my hair.
I know I am looping the table border I just dont know how to get it to work outside the loop.
here is my code..
<?php //connect to database include'db.php'; //get all data from table $sql = "SELECT * from products"; $result = mysqli_query($mysqli, $sql) or die(mysqli_error($mysqli)); //if authorized, get the values while ($info = mysqli_fetch_array($result)) { $ItemNo = stripslashes($info['Item_No']); $Man = stripslashes($info['Manufacturer']); $Cat = stripslashes($info['Category']); $Des = stripslashes($info['Description']); $Model = stripslashes($info['Model']); $Qty = stripslashes($info['Qty']); $Kw = stripslashes($info['Kw']); $Hours = stripslashes($info['Hours']); $Price = stripslashes($info['Price']); //create display string $display_block = " <table border = '1'> <td>".$ItemNo."</td> <td>".$Man."</td> <td>".$Cat."</td> <td>".$Des."</td> <td>".$Model."</td> <td>".$Qty."</td> <td>".$Kw."</td> <td>".$Hours."</td> <td>".$Price."</td> </tr> </table>"; //displays string with data echo "$display_block"; } ?>
thanks in advacne
[attachment deleted by admin]
-
CAn someone give me some guidance on how to code a customizable table to display on a web page. I am trying to create a web page just like this.
http://www.kraftpower.com/equipment/index.php
I am have an intermmediate skill level and my coding style is procedural. I cant think abstract enough for object oriented. I know most of it is SQL queries and HTML and php. But I am unclear on how to get it to display the parts like they do only one web page. The way I am thinking of invloves multiple pages and probably redundant code. I dont need anyone two write the code. I just need some ideas from anybody on how to write a page like this. I dont think it will be to difficult. And if someone has an example of something similar to what I am trying to accomplish. I can probably fiigure it out.
-
Just a bit of info for you, you don't need to assign all those variable you could just say something like
<?php echo $row['Var1']."<br/>".$row['Var2']; ?>
In the while loop because you are rewriting the variables in each iteration of the while loop it is pointless to store them there unless you have some goal to use them before they are rewritten.
Actually, I do have a goal which I am not sure how I am going to do it yet but I have some ideas.
My goal is to have the table more user friendly. At the top of the table I will have my heading row such as manufacture, category, price, etc. and I want to allow the user to click on each heading and this will execute a query to sort the rows in that table by that heading in that colunm. I am just not sure how to do this on one page without having multiple webpages???
-
I have a table with a number of records and I am trying to display them in a table on my web page. Most of my code works except it only displays the first record in the database. Can someone tell me how to get me loop to work properly? I know it has to do something with a counter to loop through the right amount of times but loops are my weakness in php prgramming.
Here is my code...
<?php //connect to database include'db.php'; //get all data from table $sql = "SELECT * from products"; $result = mysqli_query($mysqli, $sql) or die(mysqli_error($mysqli)); //if authorized, get the values while ($info = mysqli_fetch_array($result)) { $ItemNo = stripslashes($info['Item_No']); $Man = stripslashes($info['Manufacturer']); $Cat = stripslashes($info['Category']); $Des = stripslashes($info['Description']); $Model = stripslashes($info['Model']); $Qty = stripslashes($info['Qty']); $Kw = stripslashes($info['Kw']); $Hours = stripslashes($info['Hours']); $Price = stripslashes($info['Price']); //create display string $display_block = " <table border='1' cellspacing='0' cellpadding='0'> <tr> <td>".$ItemNo."</td> <td>".$Man."</td> <td>".$Cat."</td> <td>".$Des."</td> <td>".$Model."</td> <td>".$Qty."</td> <td>".$Kw."</td> <td>".$Hours."</td> <td>".$Price."</td> </tr> </table>"; } //displays string with data echo "<p align=\"center\">"; echo "$display_block"; echo "</p>"; ?>
thanks in advance
-
-
I am using dreamweaver 8 and I am trying to wrap text around an image on a page but it is not working. Can someone guide me on how to do this? My images are either below my paragraph or on top. But I would like my images to be within the paragraph.
-
NewImg = new Array (
"images/home/links_rotating_image_01.jpg",
"images/home/links_rotating_image_02.jpg",
"images/home/links_rotating_image_03.jpg",
"images/home/links_rotating_image_04.jpg",
"images/home/links_rotating_image_05.jpg",
"images/home/links_rotating_image_06.jpg",
"images/home/links_rotating_image_07.jpg",
"images/home/links_rotating_image_08.jpg",------I needed to delet this comma
problem solved
-
thanks , i look into it.
-
I have an image slideshow using javascript but I cant see why it does not work on one page but it works on all the others can someone help me with this??
here is the page with the slide show on the left hand side..
http://www.kilopak.com/home.php
here is my code behind the page..
<link href="kilopak.css" type="text/css" rel="stylesheet" /><SCRIPT LANGUAGE="JavaScript"> NewImg = new Array ( "images/home/links_rotating_image_01.jpg", "images/home/links_rotating_image_02.jpg", "images/home/links_rotating_image_03.jpg", "images/home/links_rotating_image_04.jpg", "images/home/links_rotating_image_05.jpg", "images/home/links_rotating_image_06.jpg", "images/home/links_rotating_image_07.jpg", "images/home/links_rotating_image_08.jpg", ); var ImgNum = 0; var ImgLength = NewImg.length - 1; //Time delay between Slides in milliseconds var delay = 3000; var lock = false; var run; function chgImg(direction) { if (document.images) { ImgNum = ImgNum + direction; if (ImgNum > ImgLength) { ImgNum = 0; } if (ImgNum < 0) { ImgNum = ImgLength; } document.slideshow.src = NewImg[imgNum]; } } function auto() { if (lock == true) { lock = false; window.clearInterval(run); } else if (lock == false) { lock = true; run = setInterval("chgImg(1)", delay); } } </script><script language="JavaScript" type="text/JavaScript"> <!-- function MM_swapImgRestore() { //v3.0 var i,x,a=document.MM_sr; for(i=0;a&&i<a.length&&(x=a[i])&&x.oSrc;i++) x.src=x.oSrc; } function MM_preloadImages() { //v3.0 var d=document; if(d.images){ if(!d.MM_p) d.MM_p=new Array(); var i,j=d.MM_p.length,a=MM_preloadImages.arguments; for(i=0; i<a.length; i++) if (a[i].indexOf("#")!=0){ d.MM_p[j]=new Image; d.MM_p[j++].src=a[i];}} } function MM_findObj(n, d) { //v4.01 var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) { d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);} if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n]; for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document); if(!x && d.getElementById) x=d.getElementById(n); return x; } function MM_swapImage() { //v3.0 var i,j=0,x,a=MM_swapImage.arguments; document.MM_sr=new Array; for(i=0;i<(a.length-2);i+=3) if ((x=MM_findObj(a[i]))!=null){document.MM_sr[j++]=x; if(!x.oSrc) x.oSrc=x.src; x.src=a[i+2];} } //--> </script><script language="JavaScript" type="text/JavaScript"> <!-- function MM_reloadPage(init) { //reloads the window if Nav4 resized if (init==true) with (navigator) {if ((appName=="Netscape")&&(parseInt(appVersion)==4)) { document.MM_pgW=innerWidth; document.MM_pgH=innerHeight; onresize=MM_reloadPage; }} else if (innerWidth!=document.MM_pgW || innerHeight!=document.MM_pgH) location.reload(); } MM_reloadPage(true); //--> </script><style type="text/css"> <!-- .style1 {font-size: 9px} .style4 { font-size: 12px; font-weight: bold; color: #010159; } .style10 { font-size: 18px; font-weight: bold; color: #000099; } .style11 {color: #000000} a:link { text-decoration: none; } a:visited { text-decoration: none; } a:hover { text-decoration: underline; color: #3366FF; } a:active { text-decoration: none; } body { background-image: url(images/body_bottom_blue.gif); } --> </style> <table height="560" cellspacing="0" cellpadding="0" width="100%" align="center" border="0"> <tbody> <tr> <td valign="top" colspan="2" height="109"><a href="home.php"><img height="109" alt="" width="686" border="0" src="images/topbar_01.jpg" /></a></td> <td class="expandsidebackground" valign="top" rowspan="2"><img height="560" alt="" width="42" src="images/side_expand_plan.gif" /></td> <td valign="top" width="43" rowspan="2"> <div id="Layer2" style="Z-INDEX: 2; WIDTH: 26px; POSITION: absolute; HEIGHT: 564px"><img height="560" alt="" width="43" src="images/expand_side_04.jpg" /></div> </td> </tr> <tr> <td valign="top" align="left" width="195"> <div align="right"><!-- #BeginLibraryItem "/Library/sidnav.lbi" --> <link href="kilopak.css" rel="stylesheet" type="text/css" /> <script language="JavaScript" type="text/javascript"> <!-- function MM_reloadPage(init) { //reloads the window if Nav4 resized if (init==true) with (navigator) {if ((appName=="Netscape")&&(parseInt(appVersion)==4)) { document.MM_pgW=innerWidth; document.MM_pgH=innerHeight; onresize=MM_reloadPage; }} else if (innerWidth!=document.MM_pgW || innerHeight!=document.MM_pgH) location.reload(); } MM_reloadPage(true); function MM_findObj(n, d) { //v4.01 var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) { d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);} if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n]; for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document); if(!x && d.getElementById) x=d.getElementById(n); return x; } function MM_showHideLayers() { //v6.0 var i,p,v,obj,args=MM_showHideLayers.arguments; for (i=0; i<(args.length-2); i+=3) if ((obj=MM_findObj(args[i]))!=null) { v=args[i+2]; if (obj.style) { obj=obj.style; v=(v=='show')?'visible':(v=='hide')?'hidden':v; } obj.visibility=v; } } //--> </script> <table width="195" border="0" cellspacing="0" cellpadding="0"> <tr> <td height="253" valign="top" background="images/side_nav_background_02.jpg"><img src="images/spacer.gif" width="36" height="260" align="left" /> <table width="145" border="0" cellspacing="0" cellpadding="0" class="nav"> <tr> <td width="145" valign="middle" class="nav"><img src="images/spacer.gif" width="2" height="40" /><a href="home.php" class="nav" onmouseover="MM_showHideLayers('kilopakdifference','','hide')">Home</a> <div id="hideall" style="position:absolute; width:220px; height:95px; z-index:1; visibility: hidden; top: 130px;"><a href="javascript:;" onmouseover="MM_showHideLayers('hideall','','hide')"><img src="images/spacer.gif" width="200" height="299" border="0" align="right" /></a> </div></td> </tr> <tr> <td valign="middle" class="nav"><img src="images/spacer.gif" width="2" height="20" /><a href="company_profile.htm" class="nav" onmouseover="MM_showHideLayers('hideall','','hide')">Company Profile</a></td> </tr> <tr> <td><a href="kilopak.htm" class="nav" onmouseover="MM_showHideLayers('hideall','','show')"><img src="images/spacer.gif" width="2" height="20" border="0" />Kilo-Pak</a> </td> </tr> <tr> <td><img src="images/spacer.gif" width="2" height="20" /><a href="kilopak_sound_enclosures.htm" class="nav" onmouseover="MM_showHideLayers('hideall','','show')">Sound Enclosures</a></td> </tr> <tr> <td><img src="images/spacer.gif" width="2" height="20" /><a href="kilopak_custom_controls.htm" class="nav" onmouseover="MM_showHideLayers('hideall','','show')">Kilo-Pak Custom Controls </a> </td> </tr> <tr> <td> <img src="images/spacer.gif" width="2" height="20" /><a href="kilopak_spartan.htm" class="nav" onmouseover="MM_showHideLayers('hideall','','hide')">Kilo-Pak Spartan</a> <!--<div id="soundenclosures" style="position:absolute; width:126px; height:24px; z-index:1; visibility: hidden; top: 248;"> <table width="136" border="0" cellpadding="4" cellspacing="0" bgcolor="#666666" class="border"> <tr> <td height="35" valign="top"> <div align="left" class="subnav">--------------------------------------<br> <a href="../quiet_pak.htm" class="nav">- Quiet Pak</a><br> <a href="../whisper_pak.htm" class="nav">- Whisper Pak</a></div></td> </tr> </table> </div>--></td> </tr> <tr> <td><img src="images/spacer.gif" width="2" height="20" /><a href="request_information.htm" class="nav" onmouseover="MM_showHideLayers('hideall','','hide')">Request Information</a></td> </tr> <tr> <td><img src="images/spacer.gif" width="2" height="20" /><a href="employment.htm" class="nav" onmouseover="MM_showHideLayers('hideall','','hide')">Employment</a></td> </tr> <tr> <td> </td> </tr> <tr> <td><img src="images/spacer.gif" width="2" height="20" /></td> </tr> </table> </td> </tr> </table> <!-- #EndLibraryItem --><table cellspacing="0" cellpadding="0" width="100" border="0"> <tbody> <tr> <td valign="top"> <div align="center"><img alt="" name="slideshow" src="images/home/links_rotating_image_01.jpg" /> </div> <table align="center"> <tbody> <tr> <td align="right"><a href="javascript:chgImg(-1)"><img height="18" alt="" width="19" border="0" src="images/image_controls_01.jpg" /></a></td> <td align="center"><a href="javascript:auto()"><img height="18" alt="" width="34" border="0" src="images/image_controls_02.jpg" /></a></td> <td align="left"><a href="javascript:chgImg(1)"><img height="18" alt="" width="20" border="0" src="images/image_controls_03.jpg" /></a></td> </tr> </table> <div align="center"><br /></div></td> </tr> </tbody> </table> </div> </td> <td class="bodyback" valign="top" width="491" background="images/body_expand.gif"> <table height="814" cellspacing="25" cellpadding="0" width="100%" border="0"> <tbody> <tr> <td class="body" valign="top" height="764"> <div align="center"></div> <table height="666" cellspacing="0" cellpadding="0" width="100" align="right" border="0"> <tbody> <tr> <td class="body" valign="top" align="center" height="333"> <div align="left">Over the past decade, our Kilo-Pak line of quality marine generator sets have become the most trusted name in marine power generation because Kilo-Paks are specially designed for unmatched reliability in a humid, salty marine environment. Our electric ends are sized for the power factors encountered aboard yachts. Diesel horsepower is de-rated to insure that the required power is available continuously, even in 130 degree engine rooms. <br /><br />Simply put, our generator sets will produce their full rated kilowatts and hertz continuously, even at peak demand conditions, without flinching. When it comes to building heavy duty, reliable generator sets for marine applications, no one does it better. <br /><br />Kilo-Paks range in size from 8kW to 300kW. <br /> <div align="center"> </div> <div align="center"> <table width="177" border="0" cellspacing="0" cellpadding="0"> <tr> <td bgcolor="#FF3300"><div align="center"><span class="style10">SALE</span></div></td> </tr> <tr> <td bgcolor="#FF3300"><div align="center" class="style11"><a href="kilopak.htm">Kilo-Pak Inventory </a></div></td> </tr> <tr> <td width="177" bgcolor="#FF3300"><div align="center" class="style11"><a href="hurricane.htm">Hurricane Packages </a></div></td> </tr> </table> <br /> <br /></div></div> <table cellspacing="0" cellpadding="0" width="100%" border="0"> <tbody> <tr> <td align="center"><img alt="" src="images/news_01.jpg" /></td> </tr> <tr> <td class="newsback" valign="top" height="100%"> <p><strong><font color="#000066" size="2"> <font face="Arial, Helvetica, sans-serif" size="3"> News</font></font><font color="#000066"><font face="Arial, Helvetica, sans-serif"><span class="style1"><strong><font color="#000066"></font></strong></span></font></font></strong> </p> <a target="_blank" href="http://www.projectusa2007.com"><img style="WIDTH: 193px; HEIGHT: 115px" height="73" alt="" width="250" align="bottom" src="images/project_USa_banner[1].gif" /></a> <p align="center"> </p> </td> </tr> <tr> <td align="center"><img alt="" src="images/news_03.jpg" /></td> </tr> </tbody> </table> </td> </tr> <tr> <td class="body" valign="top" align="center" height="333"> </td> </tr> </tbody> </table> <div class="body"><a href="kilopak.htm"><img height="166" alt="" width="221" border="0" src="images/home_kilopak.jpg" /></a><br /><br /><a href="kilopak_custom_controls.htm"><img height="166" alt="" width="221" border="0" src="images/home_kilopak_controls.jpg" /></a> <br /><br /><a href="kilopak_spartan.htm"><img height="166" alt="" width="221" border="0" src="images/home_kilopak_spartan.jpg" /></a><br /><br /></div> <table cellspacing="0" cellpadding="0" width="202" border="0"> <tbody> <tr> <td align="center"><img alt="" src="images/news_01.jpg" /></td> </tr> <tr> <td class="newsback" valign="top" height="100%"> <table cellspacing="8" cellpadding="0" width="100%" border="0"> <tbody> <tr> <td class="newstext" valign="top"><span class="style4">Distributor for Benelux:</span> <br /> <a target="_blank" href="http://www.kilopak.nl">Kilopak</a> Netherlands</td> </tr> </tbody> </table> </td> </tr> <tr> <td align="center" height="13"><img alt="" src="images/news_03.jpg" /></td> </tr> </tbody> </table> </td> </tr> </tbody> </table> </td> </tr> </tbody> </table> <table cellspacing="0" cellpadding="0" border="0"> <tbody> <tr> <td width="197"><br /></td> <td width="494"><style type="text/css"> <!-- .style1 {color: #999999} --> </style> <div id="Layer3" style="Z-INDEX: 3; LEFT: 205px; WIDTH: 525px; POSITION: absolute; HEIGHT: 168px; top: 973px;"> <table cellspacing="0" cellpadding="2" width="100%" border="0"> <tbody> <tr> <td class="bottomnav"><div align="center"> <p><a href="home.php">Home</a> - <a href="company_profile.htm">Company Profile</a> - <a href="kilopak.htm">Kilo-Pak</a> -<a href="kilopak_custom_controls.htm"> </a><a href="kilopak_sound_enclosures.htm">Sound Enclosures</a><a href="kilopak_custom_controls.htm"><br /> Kilo-Pak Custom Controls </a>- <a href="kilopak_spartan.htm">Kilo-Pak Spartan </a><a href="kilopak_custom_controls.htm"></a>- <a href="request_information.htm">Request Information</a> - <a onmouseover="MM_showHideLayers('hideall','','hide')" href="employment.htm">Employment</a><br /> Kilo-Pak• 2230 Peters Road Harvey • LA 70058 • p 1.888.KILOPAK(545.6725)</p> <p class="style1">© 2006 Kilo-Pak Some items shown in these photos may be optional equipment. <br /> hosted by <a style="COLOR: white" target="_blank" href="http://www.point2point.org/">point2point innovations</a>. </p> </div></td> </tr> </tbody> </table> <img height="60" alt="" width="491" align="bottom" src="images/body_bottom_17.jpg" /><br /> </div> </td> </tr> </tbody> </table> <p></p>
-
I am using dreamweaver 8 and fireworks. Can someone tell me how I can use create a top and bottom border only on a table? Or How I can use HTML to do it? I am trying to create something like this.
__________
Sale
__________
with the background in the table a different color than the page
-
here is the mysql way to change the timezone
DATE_ADD(NOW(), INTERVAL 2 HOUR)
-
I have decided to use mysql to change my time zone and it was easy.......
DATE_ADD(NOW(), INTERVAL 2 HOUR)
-
thanks. I also found another way to...
I can use this
putenv("TZ=US/Central");
-
thanks. for the feedback.
I found 2 WAYS IN PHP HOW TO DO IT.
one way is
putenv("TZ=US/Eastern");
and the other is to add 2 hours to the system time before I display it.
-
Can someone please tell me how to alter this to display in CST time. I looked in the manual but it is not clear on how to do this. I know its not hard. I just never did it before.
this is what I have....
echo date("F j, Y, g:i a"); //(ex.)March 10, 2001, 5:16 pm
-
yes, I did check the ref and I did find the instructions on how to do it but I cant do it on my end. the web host co. has to do it. He saying it may not be possible but Idont beleieve. b/c I knnow it can be done according to
http://dev.mysql.com/doc/refman/5.0/en/time-zone-support.html
if his giving me trouble I probably need to find out how to do it in php
-
ok, I figured it is pulling the system time from the server into the mySQL? Can someone tell me how I can change this from the client side?
I triied to set GLOBAL time_zone = timezone; buut I dont have super privileges.
and I tried this ..
SET time_zone = 'US/Central';
and it says incorrect time zone......can some one tell me if Idid this right?
-
In the following string I have a system date and time that displays on a web page from the database. The problem I have is the web server is in a different time zone as I am. Can someone tell me how to format this query to central time? Can this be done in MySQL? OR it has to be done in PHP?
//gather the topics
$get_topics_sql = "SELECT topic_id, topic_title, DATE_FORMAT(topic_create_time, '%b %e %Y at %r') aS fmt_topic_create_time, topic_owner FROM forum_topics_reagan WHERE topic_id = '".$_GET["topic_id"]."' LIMIT 1";
I know all of though operators but I don’t see anything regarding time zones.
if I have the server in a pacific time zone and I need the web to display central time, can someone tell me how to get this configured to display central time. I thought it would be pulling the time from the system time but I tried that and it didn’t work.
Is there something in myssql config/settings to change this to central?
-
yes, I got all of those but if I have the server in a pacific time zone and I need the web to display central time, can someone tell me how to get this configured to display central time. I thought it would be pulling the time from the system time but I tried that and it didnt work. Is there something in myssql config/settings to change this to central?
-
In the following string I have a system date and time that displays on a web page from the database. The problem I have is the web server is in a different time zone as I am. Can someone tell me how to format this query to central time? Can this be done in MySQL? OR it has to be done in PHP?
//gather the topics
$get_topics_sql = "SELECT topic_id, topic_title, DATE_FORMAT(topic_create_time, '%b %e %Y at %r') aS fmt_topic_create_time, topic_owner FROM forum_topics_reagan WHERE topic_id = '".$_GET["topic_id"]."' LIMIT 1";
-
thanks for clearing this up.
I think It is the wrong extension for the wrong version installed. I need 5 and he has 4 on his web server. ANd the pages are .php5.
-
another straightforward question"why the php code displays instead of the page design."
Is this because php is not installed? or configured properly? What could be the possible solutions to fix this? Or the wrong version of php is installed?
-
there's an apache setting in the httpd.conf file that tells the server what extensions to look for and what to parse them with. if you add .php5 to the mix in your local server, you can keep all files named .php5 and have them test the same (assuming the same PHP5 versions are running), be they local or host-run.
unfortunately you're stuck with that setting on your host, unless you buy a VPS.
yeah, I figured I would have to make some changes to my server
is this where I would add the .php5 .....
AddType text/html .php .phps .php5
AddHandler application/x-httpd-php .php .php5
[SOLVED] need some guidance on how to code a customizable table
in PHP Coding Help
Posted
well I have my table straight but can someone please give me some guidance on how to set it up like this company's web page. I know I need some hyperlinks to execute queries but how do they display the results all on one page???
http://www.kraftpower.com/equipment/index.php
I would like to have all the functionality their table has. instead of just having a simple display like I currently have I think I am on the right track.
here is my current code..