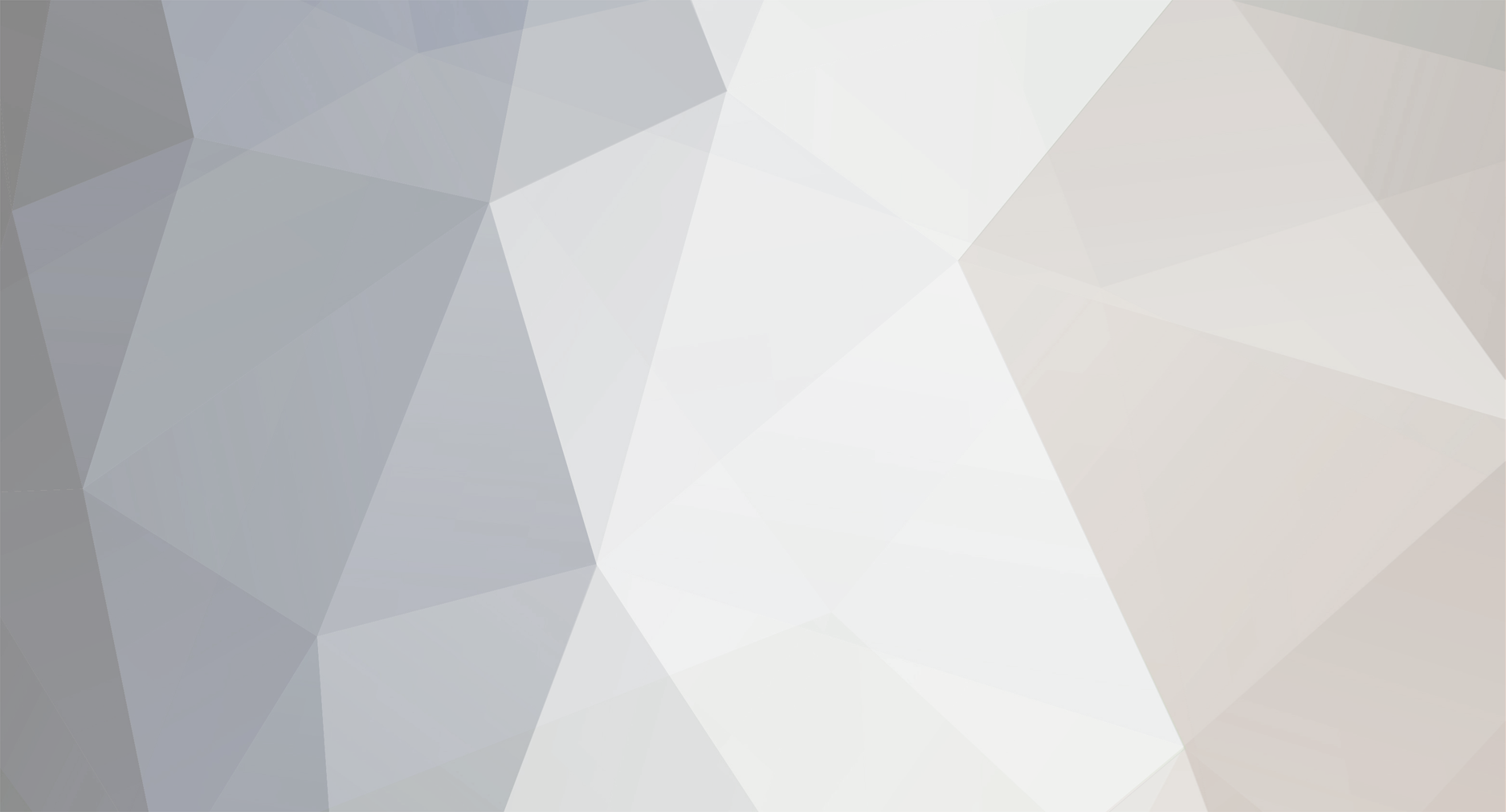
cluce
-
Posts
354 -
Joined
-
Last visited
Never
Posts posted by cluce
-
-
The same thing has been discussed here.
http://www.phpfreaks.com/forums/index.php/topic,148711.30.html
yes I saw that AND I couldnt find a solution there. thats where I got that code from and modified it.
-
I have the a small table that is username(varchar 50) and password(varchar 50). And a csv file with 2 colunms such as this.....
username|password
user1 pass1
user2 pass2
user3 pass3
user4 pass4
user5 pass5
I know how to read a csv in a loop and display it on a browser and use an UPDATE query to update one colunm but how can I pick out colunm 1 data(username) and put it in a string and pick out colunm 2 data(password) and put it in a string and use the INSERT INTO users(username, password) VALUES(userX, passX ) to insert the data into the database.
here is my code..
<?php $counter = 1; //initialize counter include'db.php'; $handle = fopen("pass.csv","r"); while(($data = fgetcsv($handle, 1000, ",")) !== FALSE) { $username = $data[0]; $password = $data[2]; $sql = 'INSERT INTO users (username , password) VALUES ("'.$username.'", "'.$password.'")'; mysqli_query($mysqli, $sql); $counter++; } ?>
what its doing is inserting only the passwords and putting it in the username colunm? can someone give me some insight on this?
-
you may also want to do something like this before sending data to your sql
//trims and strips tags and escapes fields
$checkuser = mysqli_real_escape_string($mysqli,trim(strip_tags($_POST['username'])));
$checkpassword = mysqli_real_escape_string($mysqli,trim(strip_tags($_POST['password'])));
-
Or you can create a field in your table called last_successful_login and record everytime the user logs on successfully with the NOW() function.
example from my web page
//record last login
$sql2 = "UPDATE employees SET last_login=NOW() WHERE username = '$checkuser' LIMIT 1";
mysqli_query($mysqli,$sql2);
-
it all depends. There really is no proper way. It is whatever is the most effecient way for that particular project
-
I usually start out with somehting simple than I build on that. try this. I have the exact same form as you on my website and I am using this code. Just add in the error checking. This will only send an email message to you not the person filling it out.
<?php $to = "you@youralternativeaddress.com"; $subject = "Contact form"; $body = " \n\n Name: $name \n\n E-Mail Address: $email \n\n Message: $message"; mail ($to, $subject, $body); header("Location: http://youralternativeaddress.com/thankyou.html"); ?>
-
also you may want to utilize this website too. I used all the others and I like this one the best.
-
something different.......Here is a simple php contact form code that I am using on my website right now. Our website has the exact same form as yours on your website. (2 textfields and 1 text area) Just make sure you name your fields on the contact form the same as the fields in the body section of the php code. This will email blank spaces as well as text.
<?php $to = "webmaster@iheartvacation.com"; $subject = "Contact form"; $body = " \n\n Name: $Name \n\n E-Mail Address: $Email \n\n Comments: $Comments"; mail ($to, $subject, $body); header("Location: http://www.iheartvacation.com/"); ?>
-
"Ok, another thing you could try is this function;
Basically using MD5 and SHA1. it will set passwords
as a unique ID. which means, Not only will bots who
match md5 strings fail to do so."
.......thankks for the SALT code but I dont think this will solve my problem. which the user can't logon when I use the loop code to import the passwords (in the csv file) through sha1 but when I import the passwords without the sha1 function it works?? Im lost here??? And I am matching the passwords [password input]sha1()=[database password]sha1()
-
well this what ive used in the past for login and it worked. but this was done with a registration page and the sha1 was in an insert statement through a POST method.
Now im using an UPDATE statement in a loop to read a csv file to update their passwords to sha1..if this makes a difference???
//connect to database include'db.php'; //trims and strips tags and escapes fields $checkuser = trim(strip_tags($_POST['username'])); $checkpassword = trim(strip_tags($_POST['password'])); mysqli_real_escape_string($mysqli,$checkuser); $_SESSION['password'] = mysqli_real_escape_string($mysqli,$checkpassword); //create and issue the query $sql = "SELECT username, f_name, l_name FROM employees WHERE username = '$checkuser' AND password = sha1('$checkpassword') LIMIT 1"; $result = mysqli_query($mysqli, $sql);
I am trying some alternatives right now.
-
well in another project....... I have a user registration page that registers users passwords in sha1 hash in the table and it works fine. users can login through an sql query that matches the sha1 password just fine.
I think it may be something to do with the update query and csv file in the loop code. It might be hashing a blank space or something I dont know about??? But I dont know whats going on because the loop code works fine without the sha1 function but the whole purpose of this was for me to store the users(about 300) passwords in a table in hash form. I did replace my password string with the one in your example and it doesnt work either.
It may not be able to be decrypted by that function but it will definitely increase your MySQL performance not making MySQL hash up the password. That was the goal.
As for decrypting it, I am sure that PHP or MySQL has something setup (or maybe apache?) that adds their own seeds to the hash. If the md5 function or sha1 function have been seeded in a way than that md5encryption site is useless as it can only decrypt hashes that have not been seeded.
OK I understand all of this. But can you give me any suggestions why a user can't logon when I use the loop code to import the passwords (in the csv file) through sha1 but they can login when I import the passwords without the sha1 function?? Im lost here???
-
well thanks for the performance tip. as far as the decryption part. I dont know whats going on. I guess I will have to troubleshoot some more later. im punching out for today.
-
well in another project....... I have a user registration page that registers users passwords in sha1 hash in the table and it works fine. users can login through an sql query that matches the sha1 password just fine.
I think it may be something to do with the update query and csv file in the loop code. It might be hashing a blank space or something I dont know about??? But I dont know whats going on because the loop code works fine without the sha1 function but the whole purpose of this was for me to store the users(about 300) passwords in a table in hash form. I did replace my password string with the one in your example and it doesnt work either.
-
can you please elaborate on this? im not sure what yuo mean? I though I was using php functions but I was stoiring it in mySQL in a table in a hash form. Unless you mean dont store it in the table in a hash form but only when using the passwords in the php script. Is this what you mean?? If so wouldn't that be insecure??The issue could lie within the fact that your mysql server may be setup to seed the hashes a certain way. I would use php functions to hash up the string instead of mysql, as it will be more efficient and less of a toll on your server and see if that helps you out.
-
yes, I use a website that does decryption for you. just to see what was going on with my code.I did not think MD5 or SHA1 was decryptable unless you are using a specialized function. They are generally 1 way hashes. To check if passwords match you usually take the user entered password from the form and hash it up and check it against the md5/sha1 hash.
http://www.md5encryption.com/?mod=decrypt
I thought I have this done"To check if passwords match you usually take the user entered password from the form and hash it up and check it against the md5/sha1 hash." its just not working it says invalid password/username combo
-
hello ,
I have some code that loops through a csv file of passwords and stores them in the database. This works fine if I input the passwords as is but if I try to update the passwords in a md5 or sha1 function I cant logon. its like its not importing the same password. And when I use a sha1 or md5 decrpyter that usually works it says ," could not find a matching decryption." can someone look at my code and tell me if anything is wrong with the login and update script. In the loop, I dont think it is UPDATING correctly with one of those security functions??
loop to import passwords in table
<?php $counter = 1; //initialize counter include'db.php'; $filename = "pass.csv"; $fp = fopen($filename, "r") or die("Couldn't open $filename"); while (!feof($fp)) { $line = trim(fgets($fp, 1024)); $sql3 = "UPDATE employees SET password = sha1('$line') WHERE EmployeeID = '$counter' LIMIT 1"; mysqli_query($mysqli, $sql3); echo "$counter<br>$line<br>"; //echo output $counter++; //adds 1 to counter } ?>
login page code
//initialize the session session_start(); //connect to database include'db.php'; //trims and strips tags and escapes fields $checkuser = trim(strip_tags($_POST['username'])); $checkpassword = trim(strip_tags($_POST['password'])); mysqli_real_escape_string($mysqli,$checkuser); $_SESSION['password'] = mysqli_real_escape_string($mysqli,$checkpassword); //create and issue the query $sql = "SELECT username, f_name, l_name FROM employees WHERE username = '$checkuser' AND password = sha1('$checkpassword') LIMIT 1"; $result = mysqli_query($mysqli, $sql); //get the number of rows in the result set; should be 1 if a match if (mysqli_num_rows($result) == 1) { //if authorized, get the values of f_name l_name while ($info = mysqli_fetch_array($result)) { $f_name = stripslashes($info['f_name']); $l_name = stripslashes($info['l_name']); $username = stripslashes($info['username']); } //set authorization cookie setcookie("auth", "1", 0, "/", "rwwww.com", 0); $_SESSION['usersname'] = $f_name . " " . $l_name; $_SESSION['validate'] = $username; //get last successful login $last_login = ("SELECT DATE_FORMAT(last_login, '%b %e %Y at %r') aS last_login FROM employees WHERE username = '$checkuser' LIMIT 1"); $result = mysqli_query($mysqli, $last_login); $result_login = mysqli_fetch_assoc($result); $_SESSION['login'] = $result_login["last_login"]; //record last login $sql2 = "UPDATE employees SET last_login=NOW() WHERE username = '$checkuser' LIMIT 1"; mysqli_query($mysqli,$sql2); //clears failed logins $sql3 = "UPDATE employees SET failed_logins = 0 WHERE username = '$checkuser' LIMIT 1"; mysqli_query($mysqli, $sql3); //sets session to identify $_SESSION['identity'] = $checkuser; //close connection to MySQL mysqli_close($mysqli); //sets login timer $current_time = time(); // get the current time $_SESSION['loginTime']=$current_time; // login time $_SESSION['lastActivity']=$current_time; // last activity //directs authorized user header("Location: resource.php"); exit();
-
thanks..the trim function worked.
FYI.....about the other code I was able to delete one square by doing this...
file($filename,FILE_IGNORE_NEW_LINES);
-
thanks for clearing that up. I tried the file() to read it but it does the same.
-
I wrote a loop that reads a csv file which contains passwords for users in a table. and when I run my script, it reads the file fine and updates the passwords next to the appropriate user but it adds two little squares behind the passord. for example, password[][]. it looks something like that. does anybody know why this is?
my code does work but I post it here just to give a bigger picture of what im doing.
<?php $counter = 1; //initialize counter include'db.php'; $filename = "pass.csv"; $fp = fopen($filename, "r") or die("Couldn't open $filename"); while (!feof($fp)) { $line = fgets($fp, 1024); $sql3 = "UPDATE employees SET password = '$line' WHERE EmployeeID = '$counter' LIMIT 1"; mysqli_query($mysqli, $sql3); echo "$counter<br>$line<br>"; //echo output of each count*2 $counter++; //adds 1 to counter } //} ?>
-
Is there anyway to way to use mysql_real_escape_string when updating a record?
you can do something like this...
$failed = mysqli_real_escape_string($mysqli,trim($_POST['failed_logins']));
//update failed logins
$sql = "UPDATE employees SET failed_logins = '".$failed."' WHERE username = '".$_SESSION['user']."' LIMIT 1";
mysqli_query($mysqli, $sql);
-
I use this for my login form.
//trims and strips tags and escapes fields
$checkuser = trim(strip_tags($_POST['username']));
$checkpassword = trim(strip_tags($_POST['password']));
mysqli_real_escape_string($mysqli,$checkuser);
$_SESSION['password'] = mysqli_real_escape_string($mysqli,$checkpassword);
-
What file types are showing up bad, .xls? It seems like you are reading a binary file, which would be the reason for the garbled text. Remember you cannot read a binary file like that you have to have some type of a program/intrepretor. Such as .xls (excel) file provides a way to export the data as cvs so it can be easily read.
yes. thats what haappened.
But I did try reading doc, txt, and xls just to see what would happened and none of those worked. the only files I can read with php are the csv files and I have to actually save it as csv not just rename the file extension for it to work. I think I got it for now. thanks
-
actually csv file are the only type of files I can get to display right. well some csv files does and some dont. I am thinking it might be the data in the file that screwed up????
-
when reading from file it displays the data all crazy looking and I am not sure why this is. Some files work and some don't. Can anybody shed some light on this?? I am pretty sure my code is right.
<?php $filename = "AD.csv"; $fp = fopen($filename, "r") or die("Couldn't open $filename"); while (!feof($fp)) { $line = fgets($fp, 1024); echo $line."<br/>"; } ?>
I just would like to know whats going on. here is an example of the output...);_(* "-"??_);_(@_)àõÿ À àõÿ ôÀ àõÿ ôÀ àõÿ ôÀ àõÿ ôÀ àõÿ ôÀ àõÿ ôÀ àõÿ ôÀ àõÿ ôÀ à
Also, does this code only work on certain file types?
[SOLVED] trying to read a csv file and insert individual fields into correct colunms
in PHP Coding Help
Posted
OK here is what I have which I was expecting. I just dont know how to fix it
SQL=INSERT INTO users (username , password) VALUES ("pass1", "")
SQL=INSERT INTO users (username , password) VALUES ("pass2", "")
SQL=INSERT INTO users (username , password) VALUES ("pass3", "")
SQL=INSERT INTO users (username , password) VALUES ("pass4", "")
SQL=INSERT INTO users (username , password) VALUES ("pass5", "")