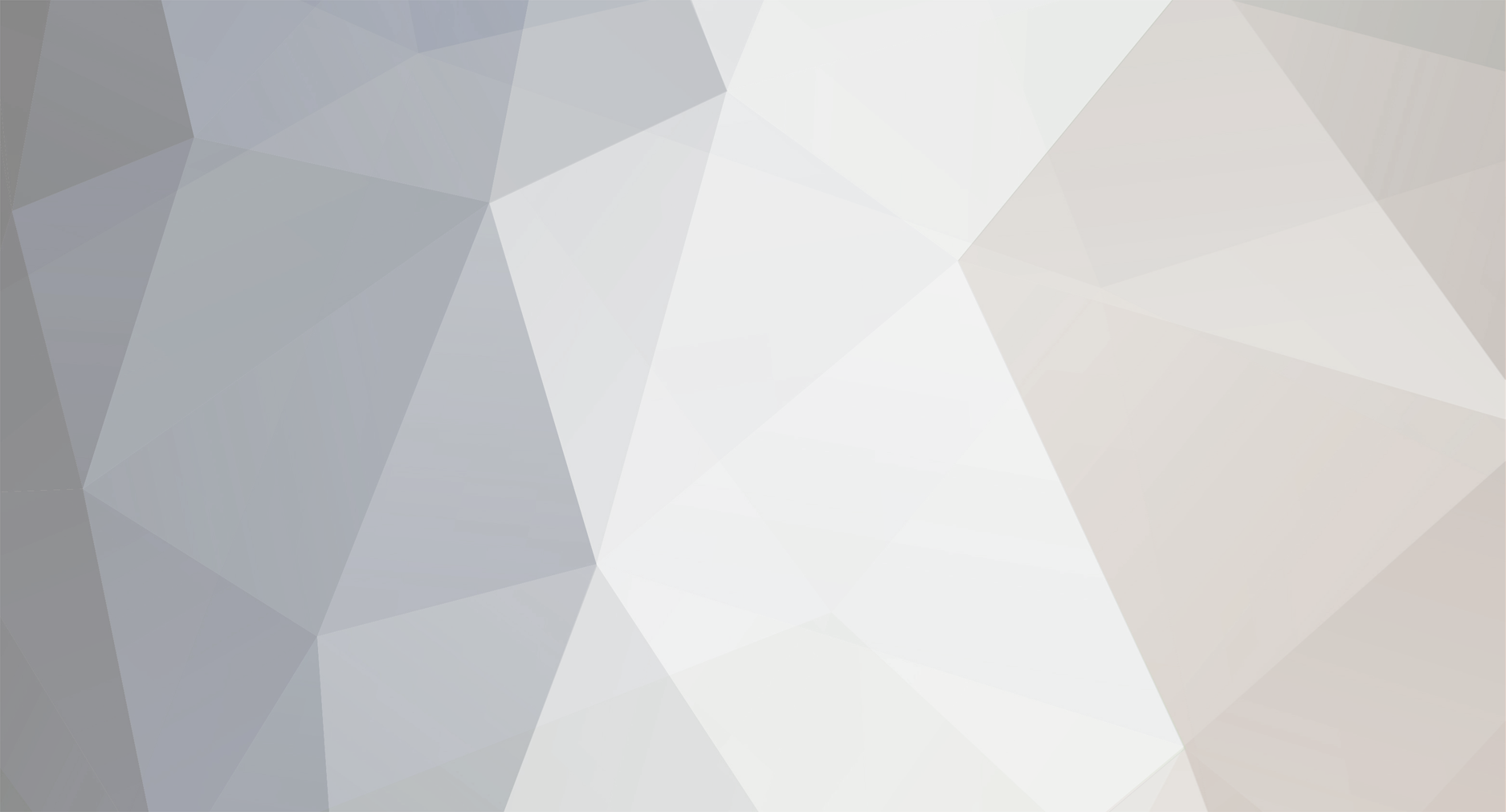
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
Math in PHP is pretty straight forward. Do you know how to write SQL? Do you know how to write PHP? Do you know how to use MySQL? If not I would suggest learning that first. As it is essential to do a database driven PHP script. Do you have current code to show us? (I kind of doubt you do). Math in PHP is not much different than math done on paper, except it is typed on the computer and the computer can do all the computing a ton faster than you can. So yea. Provide us with some code or learn the basics of PHP and MySQL then come back when you have attempted this (if you have not already attempted it) and run into a road block.
-
if (!is_resource($res1)) { // should be $res1 added the is_resource check $errors[] = "Could not run query."; //break; // not sure why break is in here, as the if statement will exit either way. Maybe you wanted die() or exit ??? } // Not sure where $res comes from //mysql_free_result($res); mysql_free_result($res1); // not needed // mysql_close($link); Not sure where $res is coming from. Also used the is_resource test. The free result is not really needed, but ok.
-
PHP MySQL Search Engine Tutorial Plenty of links to tutorials there.
-
[SOLVED] From numerical to alphabetical order - Help
premiso replied to tqla's topic in PHP Coding Help
To do it, I doubt it is possible with MySQL, unless you converted the numbers to the actual numbers. It may be possible in the array form, but I think it would be way too much trouble. If you want it done I would suggest whenever you enter a number into the DB that you extract the first character with substr then match that to an item in your array (You would have to set an array of indexes up where 0 would contain Z etc.) And append that character to the first part of the title, then when displaying, you would just remove that part of it. The test if it is a numerical index would be tricky. But that would be the only "easy" way to do it that I could think of. -
Do not use register_globals. It is depreciated as of PHP 6 as it should have been from the get go. extract is better, but I would not use it on a SUPER GLOBAL. Just call the array index instead. It is faster than using a variable, and ensure's more security. But yes, extract would be more secure than leaving register_globals on. But extracting still poses the similar threat if your code is not done properly.
-
I think it would be set. As long as they had session_start() at the top of each page. There is no reason for it not to as PHP is done on the server not the client. The guarantee would actually be 100% certain due to that fact.
-
For a URL? Wow dude, that has to be some of the worst advice. The only reason to use Full Text is for searching long entries, like in a forum or blog. No other reason to use it. For a URL Varchar(255) will be more than enough. If their URL is longer than 255 characters, then something is wrong with that URL. Even a proxy url is not that long. Stick with the Varchar for the URL, not the full_text. And honestly, even 255 is a bit extensive, but you would be safe with that.
-
Fixing some minor issues. if (isset($_POST['submit'])) { $message = "FName: {$_POST['first_name']} \n LName: {$_POST['last_name'] \n Message: {$_POST['message']} \n\n"; // the \n indicates a line break. mail("info@mysite.co.uk", "Feedback Form results", $message, "From: {$_POST['email']}", "-f".$_POST['email']); echo "Thank you for submitting a request"; // although a header redirect would be better to prevent double submittals by refresh. }else { echo "No data was submitted."; } It is also better to use the method you expect, so POST or GET as appose to REQUEST. This adds an extra precaution to the data and how it is received. You should know what type of data you expect and where you expect it to come from. As a note, your host has to have a mail server installed for mail to work. Also a minor fix to the form posted: <form method="POST" action="contact.php"> Forname: <br /><input name="first_name" type="text" /><br /> Surname: <br /><input name="last_name" type="text" /><br /> Email: <br /><input name="email" type="text" /><br /> Message:<br /> <textarea name="message" style="width:500px; height:150px" > </textarea><br /> <input name="submit" type="submit" value="Submit" /> </form> Hope that helps out a bit. It is always good to name your input boxes, especially the submit.
-
Just fixing outdated code. echo "<script type='text/javascript'>window.onload=alert('message here');</script>"; The language attribute has been depreciated for a while. It is better to use the type="text/javascript" for correct markup etc. Why even add the window.onload? Just simply calling alert would work.
-
setcookie As far as which is better, PHP or JS Cookie setting. They do the same thing, but Javascript can be disabled, so PHP would be more "Reliable" in a sense.
-
You cannot do that with PHP. The variable would have to be passed to the frame.php via Javascript or GET. This would allow you to use $_GET['hi'] to get that variable: <?php $hi = url_encode("blah"); $another = url_encode("hello bob"); ?> <iframe src='frame.php?hi=<?php echo $hi; ?>&another=<?php echo $another; ?>' width='100' height='350'> url_encode ensures that the string is passed correctly. Then in the frame.php script: <?php $hi = isset($_GET['hi'])?$_GET['hi']:null; $another = isset($_GET['another'])?$_GET['another']:null; if (!is_null($hi)) echo "You passed in {$hi} for the hi."; if (!is_null($another)) echo "You passed in {$another} for the another."; ?> A very simple example, but should get the point across.
-
echo $line . "<br />";
-
<table border='0' align="center" cellpadding='0' cellspacing='0'> <?php while ($row_Recordset1 = mysql_fetch_assoc($Recordset1)) { echo "<tr><td>".$row_Recordset1['element_1_1']."</td><td>".$row_Recordset1['element_1_2']."</td></tr>\n"; } ?> </table> Small edit in the while loop. Instead of $row switched to $row_Recordset1
-
It would be better for a singleton in this case vs extending. You really should only extend when you have a base class and the extended class does just that. Such as for an example you have an Address class. Then for the collection you have an Addresses class, which extends the address. As every address has the base essentials, street, city, zip etc. But then the addresses can add information, such as company name, contact name etc incase a person has more than one address (billing/shipping) but you do not want to have to re-create all the fields for the different class. Hopefully that is clear. I got interrupted halfway through and distracted.
-
[SOLVED] Store preg match all results in one variable??
premiso replied to john_bboy7's topic in Regex Help
implode would probably be a lot easier to do -
Singleton is a good way to go with a DB class. This would ensure that only one copy of the class is ever instantiated. Then where you use it, you just "re"-instaitate the object. Although it does not actually re-instantiate it. Instead it gives you the already instantiated object. This way you do not have to use global for it. An example: <?php class testSingleton { private static $instance; public function __construct() { echo "Test Construct<br />"; } public function test() { echo "This is the test function<br />"; } // delivers the current instance of this class "singleton" public static function getSingleton() { if (!isset(self::$instance)) { $c = __CLASS__; self::$instance = new $c(); } return self::$instance; } } class testSingletonTwo { private $testOne; public function __construct() { $testOne = testSingleton::getSingleton(); $testOne->test(); } } function testThree() { $testOne = testSingleton::getSingleton(); $testOne->test(); } testThree(); $singletonTwo = new testSingletonTwo(); ?> There is a rough break down. Be cautious when using them and only use them for classes (such as a database class) that actually need it.
-
[SOLVED] Store preg match all results in one variable??
premiso replied to john_bboy7's topic in Regex Help
Sorry, I meant to refer you to preg_match_all See the examples for ways the matches array is output and can be utilized. -
[SOLVED] Store preg match all results in one variable??
premiso replied to john_bboy7's topic in Regex Help
preg_match preg_match_all The third parameter is the array to store the matches. That puts them all in "one" variable already. -
Yep you are right. He must not be calling the database class first or unsets it.
-
I think we still get to the point of the variable scope. global $database; $database = new PDOMySQL(); ?> If you add that it should work for the session. As far as this being a good way to do it, it is not. I would look more into OOP Programming in PHP.
-
I do not think you can accomplish all that you want with 1 regex, and not that you would want to. Doing multiple would give you input to tell the user what was wrong. <?php function checkUsername($username) { // first character check $first = substr($username, 0, 1); if (preg_match('~[a-z]~i', $first) < 1) return "{$username} must contain a letter for the first character."; // last character check $last = substr($username, -1, 1); if (preg_match('~[a-z]~i', $last) < 1) return "{$username} must contain a letter for the last character."; // underscore check (test for 1 as you only want 1 occurance) $underscore = false; $numUnderscore = count(explode("_", $username)); if ($numUnderscore > 2) return "{$username} cannot contain more than 1 underscore."; elseif ($numUnderscore == 2) $underscore = true; $hyphen = false; $numHyphens = count(explode("-", $username)); if ($numHyphens > 2) return "{$username} cannot contain more than 1 hyphen."; if ($numHyphens == 2 && $underscore) return "{$username} can only contain a hyphen (-) or an underscore (_) not both."; elseif ($numHyphens == 2) $hyphen = true; if (!$hyphen && !$underscore) return "{$username} must contain a hyphen (-) or an underscore (_)."; return true; } $usernames = array("test_valid", "1notvalid", "notvalid", "not-valid-d", "noted1", "not__valid", "vali-d"); foreach ($usernames as $username) { $message = checkUsername($username); if (!is_bool($message)) echo $message . "<br />"; elseif ($message) echo $username . " was valid.<br />"; } ?> This way your user knows what they did wrong and can fix it properly.
-
No truer words said. I can type it 100 times quicker than that damn popup screwing me up. I also generally know the parameters required. Auto-completion is just a big joke for kids who are just taking the school class who do not want to program. Or horrible typers.
-
I do not know if this is solved...??? If not try ORDER BY player_rank DESC, player_roll DESC that should order by rank first and roll second both in descending order.
-
echo $row3['pud'];
-
Where is the code where you actually call add_active_guest. $database has not been defined as a class. Are you calling it in a function? If so I would have to referrer you to variables scope. As far as that being the way it "was" (the global comment), I am not a mind reader. I just see what you post.