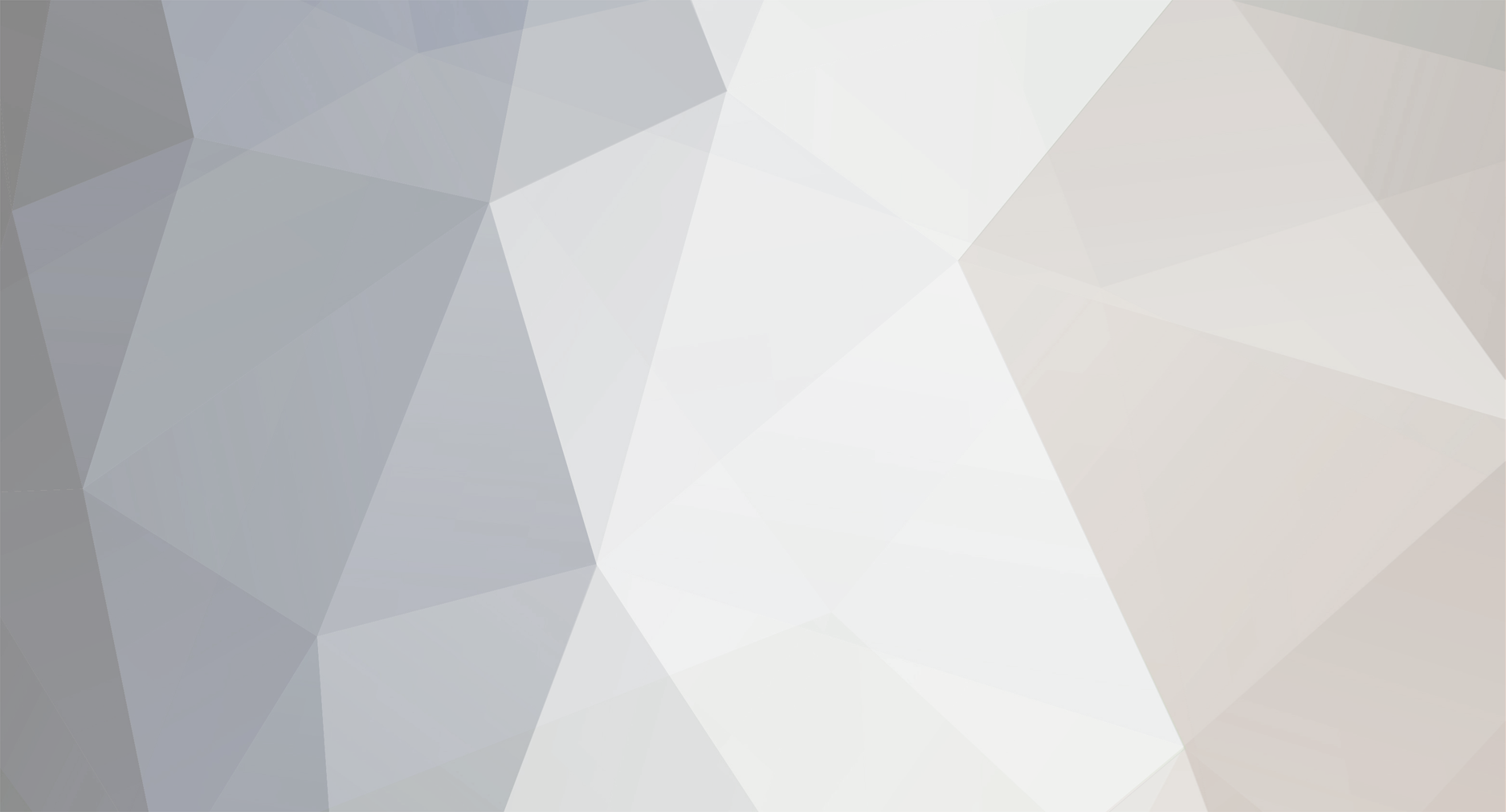
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
$xscale is not being populated right. Since you cannot divide by zero, it is an error. Where is $xscale suppose to come from? I do not see that anywhere in the code... EDIT: And the function I gave you is bad, try this instead: function getImagesList($path) { $images = glob($path . "{*.gif,*.jpg}", GLOB_BRACE); // given that $path does not have the trailing slash, if it does remove that part. return (count($images) > 0)?$images:false; } I always forget about the GLOB_BRACE part.
-
This seems like a CSS/HTML issue, not PHP.
-
Sorry, this was just bugging me: $return = insert($uid,$dist,$pb); if ($return === true) echo "The database should have been updated."; else echo "The database was not updated."; //insert new or update existing record function insert($uid,$dist,$pb) { if (!is_numeric($uid) || empty($dist) || empty($pb)) return false; //see if already exists $sql = "SELECT * FROM pb WHERE uid=$uid AND distance='$dist'"; $result = mysql_query($sql) or die(mysql_error()); if (mysql_num_rows($result) > 0) { //already exists $sql = "UPDATE pb Set `pb`.`pb` = '$pb' WHERE uid=$uid AND distance='$dist'"; //try updating in case already exists }else { //need a new record $sql = "INSERT INTO pb (uid, distance, pb) values ($uid,'$dist','$pb')"; } echo 'sql is ' . $sql . '<br>'; $result = mysql_query($sql) or die(mysql_error()); return (mysql_affected_rows($result) > 0)?true:false; } That would be a better way to structure that function, reduces reptitive code meaning less prone to errors. As far as what the issue is, why do you have a column name the same as your table? I do not know if this would cause issues, but that is just weird and can make some confusion. See if the above function works or not and let us know. And to answer manny's question, he only calls insert right before the function definition as far as I can tell.
-
$minutes = intval(round((time() - $urtime)/60)); $seconds = round($minutes%60); I would order it that way, since we use the minutes to calculate the seconds. See if that does it right.
-
I would use glob instead of the way you are doing it: function getImagesList($path) { $images = glob($path . "/{*.gif,*.jpg}"); // given that $path does not have the trailing slash, if it does remove that part. return (count($images) > 0)?$images:false; } The images array will return all the images in the folder, with the path in the name. See if that helps at all, Not sure if it will, but either way that should be a bit nicer/friendly than your old way of doing it.
-
Your database is not setup to receive remote connections. Google how to setup your SQL server to receive remote connections. You will also have to forward ports. If you intend to go this route, I would highly suggest changing the root password and creating a new account that only has access to that one table. You have some reading/googling to do now.
-
[SOLVED] Want to confirm userinput only between a-z
premiso replied to ameyjah's topic in PHP Coding Help
<?php $input = $_GET['input']; if (strlen($input) == 1 && preg_match('~[a-z]~', $input)) { echo "Yep {$input} is a single letter and small."; }else { echo "Nope {$input} is not a single letter or small."; } ?> -
mysql_connect $database = "test"; $hostname = "69.113.255.195"; $port = "3306"; $username = "root"; $password = '147171'; $con = mysql_connect($hostname . ":" . $port, $username, $password); if (is_resource($con)) { mysql_select_db($database, $con) or die("Unable to connect to database"); }else { echo "Unable to connect to DB"; } echo "Mysql connected just fine!";
-
Post the portion of the script where you call the resize image. It seems like somewhere you have a memory leak (or infinite loop).
-
$sql = mysql_query("UPDATE users SET `gold` = `gold`-100, `potions` = `potions`+ 1 WHERE `username` = '$username'") or die(mysql_error()); Will get it to update the database.
-
I think this would work.... $seconds = (((time() - $urtime)/60)%60); You want the remainder of the difference divided by 60.
-
<?php mysql_connect("database", "username", "password") or die(mysql_error()); mysql_select_db("members") or die(mysql_error()); $sql = mysql_query("SELECT * FROM `users` WHERE `username` = '$username'"); if (mysql_num_rows($sql) > 0) { $row = mysql_fetch_array($sql); if ($row['gold'] >= 100) { ////Had error in this line //// $sql = ("UPDATE users SET gold = gold-100, potions = potions+1 WHERE username = '$username'") or die(mysql_error()); $sql = ("UPDATE users SET `gold` = `gold`-100, `potions` = `potions`+1 WHERE `username` = '$username'") or die(mysql_error()); echo "<center>You have bought one health potion.</center>"; }else{ echo "<center>You don't have enough gold. Come back when you getmore.</center>"; } }else { echo "No rows were returned by the SQL. Username entered was " . $username; } ?> Where does $username come from? Try the above, my bet is that $username will not display. You need to assign $username a value some how whether from $_POST/$_GET/$_SESSION, it seems like you are assuming register_globals is turned on, which it is not and this is causing the problem.
-
Well if it re-renders the image 3 or 4 times, that bumps up the memory to around 12 MB. Try changing the Memory limit to 32M if it is at 8M or 16M and see if that solves the issue.
-
You have the foreach in the while. That is where you are getting the issue at. If you call $_POST['checkbox']['itemnamehere'] that will solve the problem. I am not sure how you get the checkbox name value but you can use that to pull out the item you want to update. Hope that makes sense, as I would not even know where to start on your code to show you how to implement it.
-
Hmm. Not sure why it would have returned that...maybe it is an older version of PHP I do not know. This this instead: $currentGold = $row['gold'] - 100; echo "<center>You have bought one health potion. You now have " . $currentGold . ".</center>"; }else{ echo "<center>You don't have enough gold, you currently have " . $row['gold'] . ". Come back when you getmore.</center>"; } See if that produces a number. I have a hunch that it showed the $row['gold'] portion maybe because it is not defined...no clue....it seems there maybe an issue on your server ???
-
Do they have enough gold? I would print out the $row['gold'] in the else: $currentGold = $row['gold'] - 100; echo "<center>You have bought one health potion. You now have {$currentGold}.</center>"; }else{ echo "<center>You don't have enough gold, you currently have {$row['gold']}. Come back when you getmore.</center>"; } And see what is displayed.
-
Out of curiosity, what is the size of the image? If it is close to 8 or 16m you may need to use ini_set and set the memory_limit for this script to be a bit more, as it does re-render the image at least twice, which would double the memory usage. Let us know. I am not too good with the image functions, so yea.
-
It looks like it is just looping through the same checkboxes. Post your fully generated form code so we can look at it.
-
[SOLVED] How do I code an if statement in this way?...
premiso replied to jandrews's topic in PHP Coding Help
Oh well why did you not just say that if (!empty(trim($row_Tab['Tab']))) { echo $row_Tab['Tab']; }else{ echo 'no tabs!'; } I doubt the trim is needed, but yea. See if that works for you (and ignore the query up above revert it back to your original one). -
[SOLVED] Second variable in combine string is ignored...
premiso replied to bubbasheeko's topic in PHP Coding Help
If you are echoing it to the screen, it is because of the < and > they are taken by html as a tag. echo "<pre>" . $to . "</pre>"; will show it, alternative you could view the source and see it as well. I take it this is for debugging, but if you want it to display in HTML, you can use htmlentities to convert the < and > to it's html code to display properly. -
Special Characters: _GET option not working
premiso replied to EternalSorrow's topic in PHP Coding Help
Well the next issue is your charset, your DB charset is probably latin, this should UTF-8 for special characters. Your page that you submit the data to the DB to should also be in UTF-8 format for proper usage. Try changing the DB charset to UTF-8 and defining your pages charset to also be UTF-8 using either header or the meta tag in HTML. -
Ok, well for one, the name has a space in it. That usually is not kosher. Second you could make it an array of checkboxes by doing this: foreach ($qwert as $v) { if (in_array("$v", $qwert2)) { echo "<input name='checkboxname[$v]' type='checkbox' value='$v' checked/> $v"; } else { echo "<input name='checkboxname[$v]' type='checkbox' value='$v' /> $v"; } } Then using this: foreach ($_POST['checkboxname'] as $key => $val) { echo "Checkbox: {$key} contains a value of {$val}<br />"; } Should give you an idea how to use that to do your tests.
-
Post a few checkboxes (the actual html) of your form, so we can see what you are working with. As for the pretty colors you can use without the <?php or with the <?php should work for coloring.
-
Nope, you should not need to. You can close it, but after the script runs the memory is generally dumped anyways. Doing it would just be an "ensuring" step in my opinion that it is indeed dumped.
-
[SOLVED] How do I code an if statement in this way?...
premiso replied to jandrews's topic in PHP Coding Help
if (stristr($row_Tab, "\t") !== false) { echo $row_Tab['Tab']; } else { echo 'no tabs!'; } That should work I would think. See if that is what you want...I take it you are trying to see if the result has a tabbed character in it. Alternatively, this may work as well (although I am not sure): $query_Tab = sprintf("SELECT Tab FROM flamplayer_musics WHERE (title_music LIKE '%%%s%%' AND title_music LIKE '%%%s%%')", $colname_Tab, "\t"); Unsure of my sprintf usage, but yea. The query I would think would be the preferred method, but however you want it should work, as long as it does in deed work