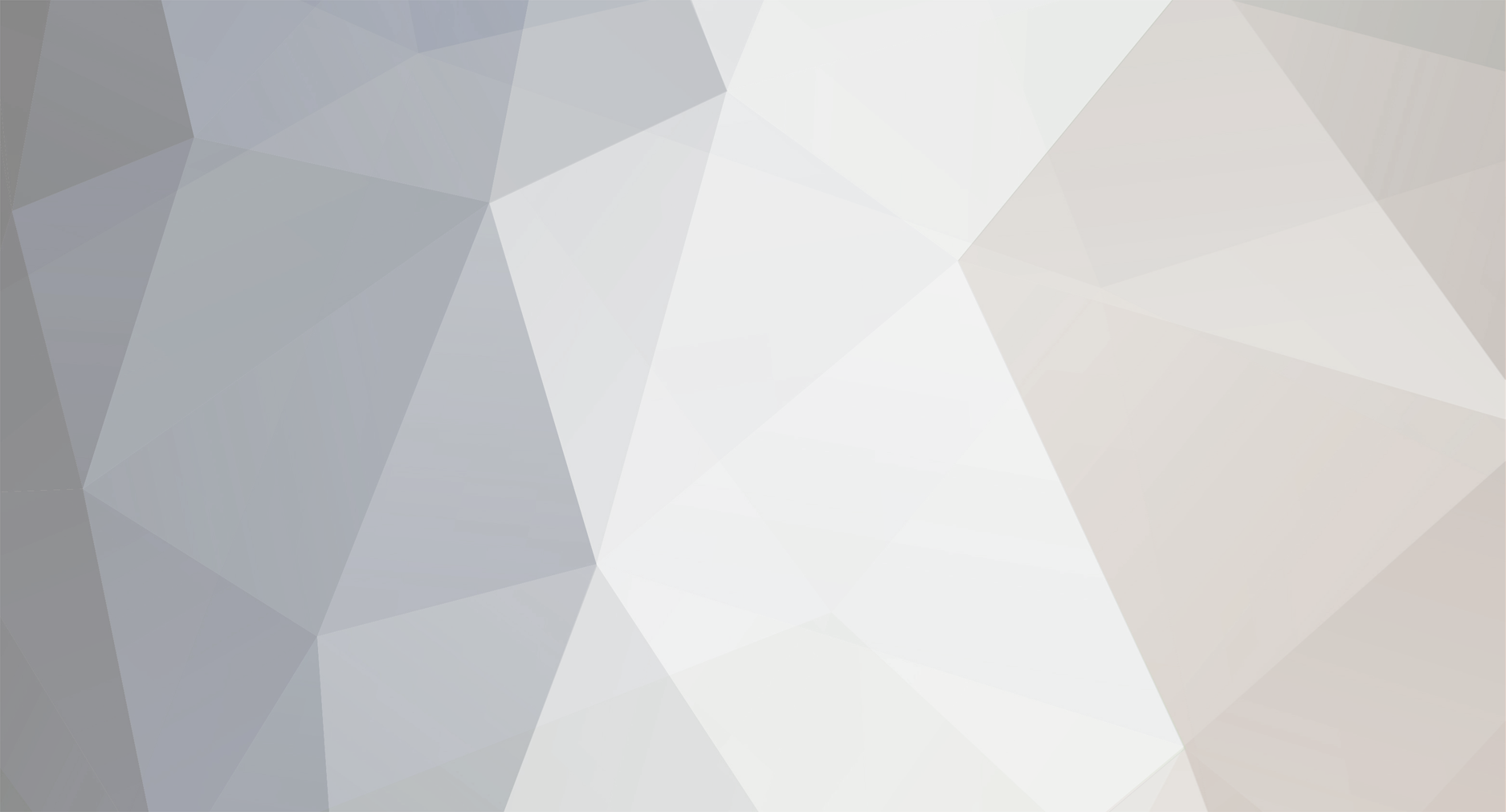
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
<?php class msSql { private $con; public function __construct($host, $user, $pass, $db) { $con = mssql_connect($host, $user, $pass); mssql_select_db($db, $con); } public function query($sql) { return mssql_query($sql); } } ?> Something like that is a rough example, I am not sure how MSSQL works, so the functions may not be correct. But yea.
-
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_exec and curl_setopt
-
Since no one pointed it out really, date the PHP Manual for date tells you all the variables you can use and what they will return.
-
Nope. Your host must not like that/had issues with it in the past. imo, switch hosts. There are plenty that at least allow Curl that are decently priced. You can try using Sockets but I doubt they will work given that the latter two did not.
-
<?php // create a new cURL resource $ch = curl_init(); // set URL and other appropriate options curl_setopt($ch, CURLOPT_URL, "http://www.example.com/"); curl_setopt($ch, CURLOPT_HEADER, 0); // grab URL and pass it to the browser curl_exec($ch); // close cURL resource, and free up system resources curl_close($ch); ?> curl_exec
-
Are you on shared hosting? If so then chances are they do now allow fopen_url, they may allow CURL but for file_get_contents to work the server has to have that option set (you can do a phpinfo to find this out). My bet is that is what is going on.
-
EDIT: Post the actual url not some http:// this is a weird site url that i cannot just write http://www.site.com/file.mp3 That will get you more help than anything. My bet is your are urlencoding the whole url, you should only do this for the name of that file without the extension. http://www.google.com/(your file name needs to be encoded).mp3
-
javascript items into php to store in database.
premiso replied to brown2005's topic in PHP Coding Help
You would have to pass those values from javascript to php via GET/POST or a Cookie. Javascript is Client PHP is server thus that is the only way to get them to talk. -
$query="UPDATE tblname SET Headline='$headline', Date='$date', Preview='$preview', Body='$body' WHERE AID='$aid'"; Notice it is not $AID it is $aid, php Is CaSe SenSiTive.
-
setcookie PHP Has a function to do this, look into that.
-
Turn them off and fix your code to work properly. It is a security risk having them on and they will soon be left out (I think PHP6 you can no longer turn them on/off). Read up on them at http://www.php.net/register_globals Basically instead of accessing the variable with just $varname it would be $_GET['varname']. It is a very basic and easy change/fix to any code, just some people are to lazy to take the hour or so it may take to fix their variable declarations. EDIT: Security risk, potentially anyone can gain authenticated access to a site if the site is not coded properly by declaring variables. For example $_SESSION['loggedin'] is what a bunch of people use. If they just access this by $loggedin I just pass page.php?loggedin=true and wham I am authenticated. (Given that they did not code for this which if register_globals is on the chances are high.)
-
How can I achieve this? That has to be the worst question in the word. Please post EXACTLY what you are trying to achieve in words, what you need help with, what you have tried and not tried and how is it "not working".
-
That code is missing some brackets, leave the mall off or keep them all on. if ($user == 'admin') { header('Location: http://localhost/staff.php'); }elseif ($user == 'client') { header('Location: http://localhost/client/client.php'); }elseif ($user == 'guest') { See if that gets you your redirect.
-
basic php/mysql syntax question on a single line of code
premiso replied to cunoodle2's topic in PHP Coding Help
It works for any array if you do not have a constant set with that name. Because if PHP does not find it as a constant it throws an error and interprets the "value" literally. It is bad practice not to surround non-numeric indexes of arrays in quotes (single or double). Due to the Undefined constant error. It is not a huge error, but if you code it right it is more efficient, since it does not have to throw that error and search if a constant is defined with that etc. With the single/double quotes it does not take that extra time searching and just does. The only options you are are "As", the numerical index, or re-designing your table column names as I suggested above. Simple as that. -
Where is $project being set? I fail to see that part. It echos out array because to print an array in a message you need to use print_r (with 1 for the 2nd parameter). You are "printing" an array like a string, which just prints array. Also note the foreach you use the same variable (I am surprised this works due to that).
-
basic php/mysql syntax question on a single line of code
premiso replied to cunoodle2's topic in PHP Coding Help
That is interesting. Basically it overwrites the id. This is why I use unique ids for tables, like server table it would serid and detail would be detid as the column name. Avoids this and other issues. To avoid this use as, but since you are using the mysql_fetch_array you can call it by the index listed at. So for the serverid it would be $row[7] or $row['id'] since that is last in the list it overwrites the first and the detail id would be accessed by $row[0]. EDIT: I generally name my columns in table that way (sername detname) because of such issues with joins and that way I know which table I am pulling from. Because of this and since you actually list the columns I would just use mysql_fetch_row and access the data by their indexes, -
Technically he does not need to escape it as an MD5 hash will have all allowed values. That is just doing an unnecessary process. However if on the $password he escaped it going into the DB then MD5'd it, that should be added back in there as the password may depend on variables be escaped then md5'd.
-
SELECT attname, count(id) as num FROM war GROUP BY attname Should do it, given that the primary key field is "id"
-
array_shift would "remove" the headers if that works. If not you can mvoe the internal array pointer with next to skip to the 2nd result.
-
[SOLVED] Drop Down Menu question, I think
premiso replied to jim.davidson's topic in PHP Coding Help
Yep, and using google you can find your answer too....ironicly. http://www.dynamicajax.com/fr/Suggest_Frontend-.html They use AJAX. -
You should readfile the file instead of printing it.
-
A few issues I spot off the back, you are making it so only admin's can login? Is that correct? // username and password sent from form $username=stripslashes($_POST['username']); $password=stripslashes($_POST['password']); // as this would make a different if magic_quotes are on, so it is good to have it the same. $enc=md5($_POST['password']); // $username = stripslashes($username); // also might as well move this up // $password = stripslashes($password); // no point in doing this here as the password is md5, move it up $username = mysql_real_escape_string($username); $password = mysql_real_escape_string($password); Second, you should only be expecting 1 item from the DB so the while loop is unnecessary: // while($row = mysql_fetch_array($sql)){ // not needed $row = mysql_fetch_assoc($sql); // use assoc over array. $_SESSION['username'] = $row['username']; $_SESSION['password'] = $row['password']; // $row is how you should access it. Try those changes and see what happens.
-
How to trigger mail & get to next program line in PHP
premiso replied to freephoneid's topic in PHP Coding Help
The command just looks weird to me either way, try invoking it with the PHP CLI and see what happens: $command = "/usr/bin/php -f /var/www/html/ers/emailsender.php"; The only other way I would recommend is setting up a Cron Job to run this script, this way it is ran in the background on a routine schedule without you having to put this in a script etc. Go Here for an explanation/tutorial on how to set that up. -
$querySongs = "SELECT COUNT(songID) as SongCount FROM vote WHERE songID = '$songID'"; $resultSongs = mysql_query($querySongs) or die (mysql_error()); $rowSongs = mysql_fetch_assoc($resultSongs); echo $rowSongs['SongCount']; Should do it. Using the "AS" keyword allows you to create an alias of the actual field (essentially renames it when it is fetched). EDIT: To not use the "as" this would also work. And actually I prefer this way for these type of queries: $querySongs = "SELECT COUNT(songID) FROM vote WHERE songID = '$songID'"; $resultSongs = mysql_query($querySongs) or die (mysql_error()); $rowSongs = mysql_result($resultSongs, 0); echo $rowSongs; As you just pull that first column out
-
That would not work, due to the max being in the wrong place and that his table structure is most likely varchar for "td". The ` are used around table, db and column names to signify them as such. They are not required and do not hurt to be there. To correct your SQL (just to show you the proper way) here it is: SELECT * FROM `war` ORDER BY MAX(`war`. `td`) DESC LIMIT 1 But I doubt that would work, cause MAX is for the select portion and needs/requires a group by. Group By Aggregate Functions For a tutorial on MAX go here.