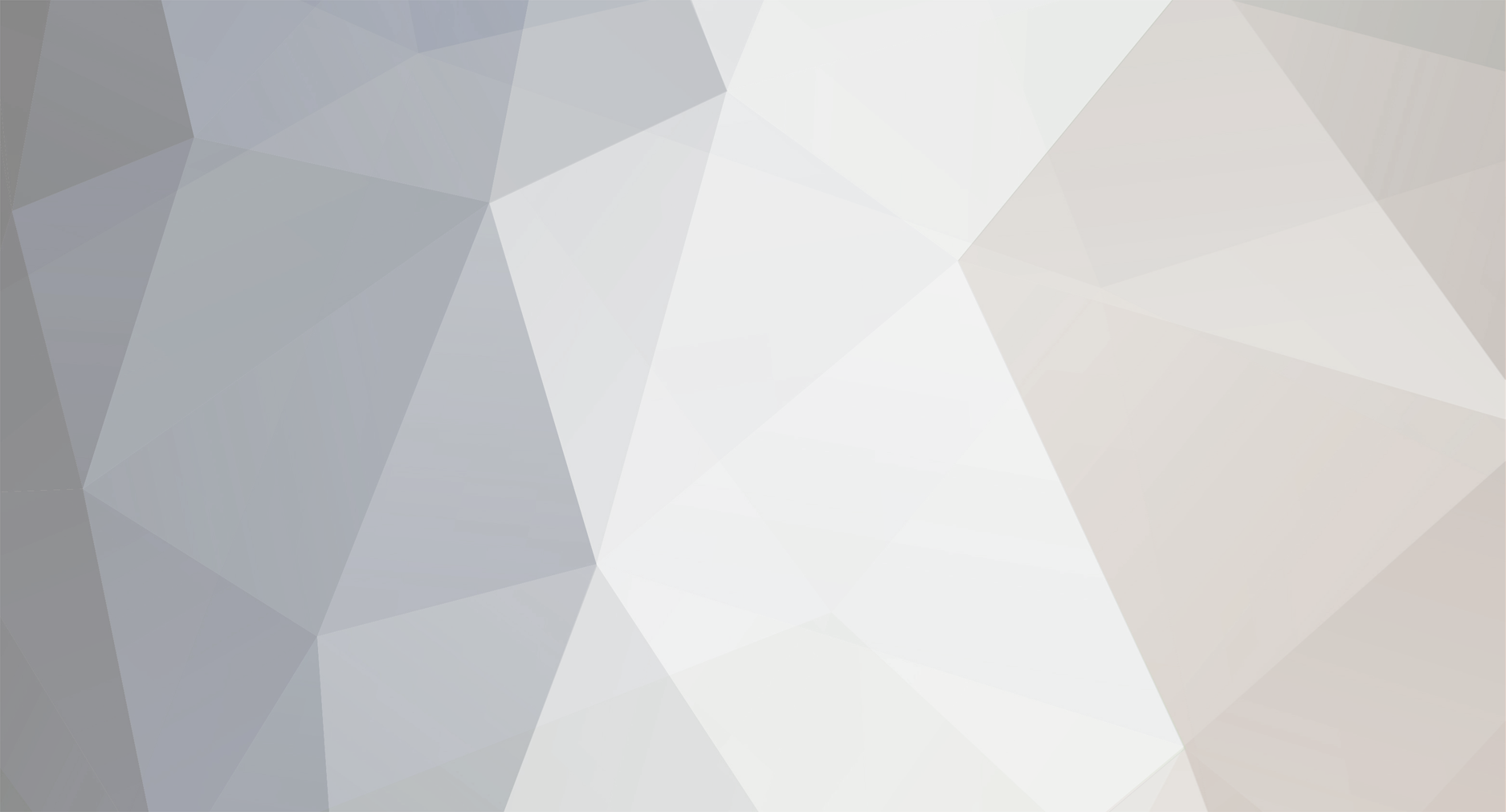
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
The header error is from the debug echos, remove them and it will fix that. As far as the sprintf, it is not a bad habit, you were just using it wrong. If you want to use it read up on the correct usage.
-
You are including a URL, you have to include the file from the server. include ("header.php");
-
[SOLVED] Displaying Text File. - Entries Missing. !
premiso replied to TomT's topic in PHP Coding Help
htmlentities <?php $file = "c:\Logs\LogFile.txt"; $users = file($file); echo "<table border='0' width='95%'>"; echo "<font face='Verdana' size='2'><b>" . $file . "</b><br><br>"; foreach ($users as $user) { echo "<tr>"; echo "<td align='left'><font face='Verdana' size='2'>" . htmlentities($user) . "</td></tr>"; } $result = count($users); echo "</table><br><font face='Verdana' size='2'>Number of Entries : <b>" .$result ."</b><br>"; echo "Last Updated: <b>" . date('D jS M \'y H:i:s') . "</b>"; ?> -
Here is a corrected version of your code, with comments on what/why I changed it. <?php session_start(); if(isset($_POST['username'])) { require_once 'config.php'; /*DATABASE SETTINGS */ $username = mysql_real_escape_string($_POST['username']); // do this here echo "DEBUG: Username = " . $username . "<br />"; // check that it contains the right value. $password = md5($_POST['password']); echo "DEBUG: Password = " . $_POST['password'] . " Encrypted = " . $password . "<br />"; // check that it contains the right value. $query = "SELECT COUNT(user_id) FROM users WHERE user_name = '$username' AND user_pass='$password'"; // not sure why you were using sprintf Since you manually define the values this should work. $result = mysql_query($query) or die("SQL Error: SQL: {$query}<br /> mySql error: " . mysql_error()); list($count) = mysql_fetch_row($result); if($count == 1) { $_SESSION['authenticated'] = true; $_SESSION['username'] = $_POST['username']; // just so the escaped one is not used. $query = "UPDATE users SET last_login = NOW() WHERE user_name = '$username' AND user_pass = '$user_pass'"; // since you put the values in, I doubt sprtinf is needed also note the added quotes to $username mysql_query($query) or die("SQL Error: SQL: {$query}<br /> mySql error: " . mysql_error()); header('location:gedichten/gedichten.php'); } else { $color = "red"; $echo = 'There is no username/password combination like that in the database.'; } } ?>
-
So it runs every 6 minutes fine, but if you change it to hours, it runs for every video...correct? It seems like it may be a problem with the cron in the Direct Admin. For whatever reason it is running it at quicker intervals than you want it to. I take it that is your control panel and I would talk to your host about it.
-
[SOLVED] Using classes within if statement
premiso replied to DanielHardy's topic in PHP Coding Help
Honestly, about 80% are thrown off from this as you did not use the tags to surround your code. It makes it tedious and hard to read code without them. As far as why that ereg is not working, A ereg should not be used it is being depreciated. B you are just checking if the string is empty? Why not use empty. C, that ereg always returns true cause it has nothing to check for, thus it is true. Hope that makes sense. -
I cannot remember but I think the hours should be 0/6 not */6. You may want to read up more on Cron Syntax as this is a result of the cron running too often, I believe. As for the script, I would change $count_rows != 0 to == 1 that way you know if more rows are being returned (for whatever reason) that will not run. I would also have it log to an "Error" file if that is the case so you can see what is happening on an error.
-
if($_POST) { Is always true, instead use isset of a form element that is required. if(isset($_POST['username']) { You should also check that the query returned a result by either using is_resource. Also the UPPER in the SQL is not required for username, as MySQL is already case insensitive. And you do not need to escape md5 hashes (they will not contain bad characters). Why are you running the query twice? You should add an or die to your mysql_query (at least while debugging). $result = mysql_query($query) or die("SQL Error: SQL: {$query}<br /> mySql error: " . mysql_error()); To give you better feedback.
-
[SOLVED] How can I do this...not sure where to start
premiso replied to kevinritt's topic in PHP Coding Help
No worries. I am just a little moody today, and yea. The reasoning for just posting "AJAX" was that I figured he was a beginner and if he wanted to learn it, it is better to search for it. At least he now knows the terminology. Anyhow, it was my bad in that I completely mis-read the post (and probably did not supply sufficient data with it). He was just asking for data to post/populate data and never specified a page-refresh, which ajax would not be affiliated with. -
[SOLVED] How can I do this...not sure where to start
premiso replied to kevinritt's topic in PHP Coding Help
So what, I cannot inform them that it is an option? @cooldude, maybe. However it is still a way to do a Dropdown list when you click on someone's name have their data display. @both, granted I just mis-read the post, I thought he was looking at dual drop down lists (IE I select this name a new one is populated). But still, lay off. I did nothing wrong with informing him of another item he can later look into for populating data on his webpage. -
If they are uploaded, they are already stored in a temp folder. If they are on the server, no. You can just read them straight from where they are.
-
[SOLVED] How can I do this...not sure where to start
premiso replied to kevinritt's topic in PHP Coding Help
Another option is to look into AJAX. (AJAX does not require a page re-load). -
I do not think you can....that is just a bad designed DB. MySQL Date Functions About all I could think is you can try the MakeTime function, or the STR_TO_DATE function and see if they allow you to convert it to a good date format to sort by. To fix this issue at it's source, you should be able to create a new date column, which will store a new date and be of DATETIME and use that str_to_date function to convert the current date to a good date and update it. If that does not work, you can pull it out in php explode the date at the "." then re-create it in a good date format and input it back into the DB so you have the correct format and not have to do this "hack" to get your sql to sort by date.
-
Yep, you are right. Sorry, my brain is dead tired today lol! Thanks for correcting me, however.
-
You will need to add ceil to round it up I believe. But have you tested that code? Generally you should try it before posting and if you have tried it make a note of that.
-
The above is bad. My internet connection died out so I could not fix it. <?php $ipSearch = array('192.168.0.1', '192.168.0.2', '192.168.9.1'); $ipaddresses = array('192.168.0.3', '192.168.0.2', '192.168.1.1', '192.168.9.1'); $found = array_intersect($ipSearch, $ipaddresses); if ($found !== false) { foreach ($found as $val) { echo "{$val} was in both arrays.<br />"; } } die(); ?> array_intersect_keys should give you the indexes if that is what you are after.
-
array_search may help you, you can pass in an array and check if the values are in the array, if they are it returns you the indexes. <?php $ipSearch = array('192.168.0.1', '192.168.0.2', '192.168.9.1'); $ipaddresses = array('192.168.0.3', '192.168.0.2', '192.168.1.1', '192.168.9.1'); $found = array_search($ipSearch, $ipaddresses); if (count($found) > 0) { foreach ($found as $key) { echo "{$ipaddresses[$key]} was found at index {$key}.<br />"; } } ?>
-
[SOLVED] Create Thumbnails working on FF doesn't work on IE
premiso replied to balkan7's topic in PHP Coding Help
Did you write this code? if ($_FILES['slika']['type'] == "image/jpg" or $_FILES['slika']['type'] == "image/jpeg" or $_FILES['slika']['type'] == "image/pjpeg"){ Well either way, this is how you fix it. -
foreach ($allowed_url as $allowed) { if(stristr($allowed, $passed_url) !== false) { $string = file_get_contents('http://www.domain.com/content.php?a=uhh&?b='.$allowed); preg_match("~thepage=\"(.+?)\"~si", $string, $matches); header('Location: '.urldecode($matches[1])); exit; } }
-
[SOLVED] accessing the array value witout putting it in another variable
premiso replied to asmith's topic in PHP Coding Help
Honestly clean code is nice, but easy to read code is better. I tend to keep my code where people can know what is going on if someone is coming in behind me or to help me. Memory is abundant, so you might as well use it, especially with a simple thing as a variable or an array of variables. The list is good for what you want, but say it explodes 50 characters and you never know which one you want (IE it is not always the second one). The array would be much easier/better imo for that situation. It all depends on your ultimate goal. -
How do i use php code to do $date - $singup_date
premiso replied to lkh11lkh's topic in PHP Coding Help
If you are pulling this from mysql, use that to do it given the function DATE_SUB. -
MySQL round SELECT u.downloaded,u.uploaded, round((u.uploaded/u.downloaded), 2) as ratio ,u.uid
-
Well you would need to look more into the SimpleXML setup, I do not htink you are using it wrong, hence the error. For a preg_match function to do this, this would work. <?php $string = '<user type="cool" thepage="http%3A%2F%2Fgoogle.com" />'; preg_match("~thepage=\"(.+?)\"~si", $string, $matches); echo urldecode($matches[1]); ?> As far as which is better, I do not know. I have a hunch that the preg_match is quicker due to it does not have to create an object of all the xml elements since you simple want a static element etc.
-
When all else fails use absolute paths. <?php include ($_SERVER['DOCUMENT_ROOT'] . '/folder/where/nav/is/in/navigation.php') ;?> Simply replace the /folder/where/nav/is/in with the right folder and that should work.
-
Take the bytes uploaded and divide it by the bytes downloaded. This should give you the ratio you want. SELECT u.downloaded,u.uploaded, (u.uploaded/u.downloaded) as ratio ,u.uid FROM users u ORDER BY ratio LIMIT 3 If that does not work for the order by, change it to ORDER BY (u.uploaded/u.downloaded)