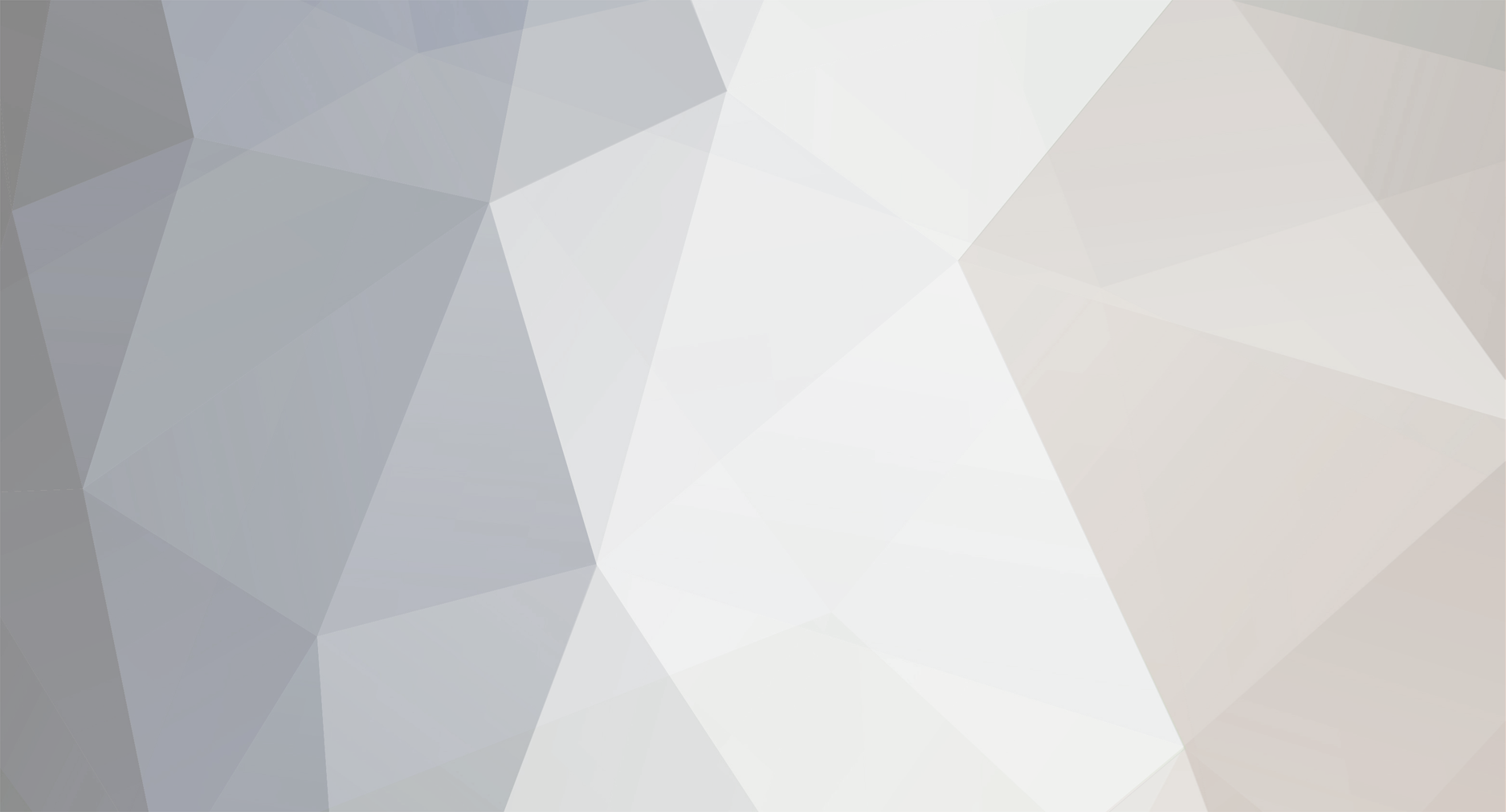
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
Adding database name within the initial parameters
premiso replied to 9three's topic in PHP Coding Help
Why are you putting the select in a different function if you are not even providing the option to add a different database as a parameter? <?php class mysql { private $host, $username, $password, $dbname; public function __construct($host = '', $username = '', $password = '', $dbname = '') { $this->host = $host; $this->username = $username; $this->password = $password; $this->dbname = $dbname; $this->connect(); $this->select(); } public function connect() { $this->link = mysql_connect($this->host, $this->username, $this->password); if (!is_resource($this->link)) Throw New Exception(mysql_error()); return $this->link; } public function select($dbname='') { if (isset($dbname) && !empty($dbname)) { $this->dbname = $dbname; } $this->select = mysql_select_db($this->dbname, $this->link); if (!is_resource($this->select)) Throw New Exception(mysql_error()); else return $this->select; } ?> Now this code: $connection = new mysql('localhost', 'root', ''); $connection->select('cms'); // note quotes $query = "SELECT ID, Username, Password, Email FROM admins"; $connection->query($query); Should work. It should also work with the db being passed in as a parameter to the constructor. -
Ok, using a do while you would have to pull the first result. Instead just use a while. No reason to do the do while in this scenario: <?php $RsCities_endRow = 0; $RsCities_columns = 5; // number of columns $RsCities_hloopRow1 = 0; // first row flag while ($row_RsCities = mysql_fetch_assoc($RsCities)) { if($RsCities_endRow == 0 && $RsCities_hloopRow1++ != 0) echo "<tr>"; ?> <td><a href="http://mysite.com/city_hotels.php?CityID=<?php echo $row_RsCities['CityID']; ?>" class="style1">HOTELS IN<?php echo $row_RsCities['CityName']; ?></a></td> <?php $RsCities_endRow++; if($RsCities_endRow >= $RsCities_columns) { ?> </tr> <?php $RsCities_endRow = 0; } } if($RsCities_endRow != 0) { while ($RsCities_endRow < $RsCities_columns) { echo("<td> </td>"); $RsCities_endRow++; } echo("</tr>"); }?> Try that and see if it works. Chances are it was throwing an error. Part 2, I do not see the query statement in that code. I assume you omitted it. That is fine, if you did not omit it, you need it . Similar to what steve wrote, except remove the last 2 lines and then put your original code.
-
Read up on CSS. This is really not a PHP issue, the first question was, but if that is solved this is a CSS/HTML issue. http://www.echoecho.com/css.htm a good tutorial on CSS.
-
If it works for you, great. One word of advice: <?php include ("header.html"); ?> <?php include ("menu.html"); ?> <?php Change that to: <?php include ("header.html"); include ("menu.html"); No need to go in and out when doing multiple includes.
-
Sounds like you have been hacked to me. That will allow any user who knows the hash for rnk to execute any code on your server. Scary stuff man. Check logs, contact host and remove that from every page is it is on. If your site has an uploader, I would look into that to increase security on it, as that could be the source, or if you allow dynamic including of files, "include($_GET['file'])" I would also look into securing that too.
-
Using the PHP CLI and extending the max execution time to 30 minutes, this should be possible. As for running it in the browser, it is not probable, due to memory on the side with the browser etc.
-
How many dimensions are you expecting to have? If a bunch you want to look into recursion, if not this would work: <?php foreach( $_POST as $key => $value){ if (is_array($value)) { foreach ($value as $k => $v) { echo " <strong class='textb'>$k : $v <br /></strong>"; } }else { echo "<strong class='textb'>$key : $value <br /></strong>"; } } ?>
-
NetBeans IDE is the best for PHP development? Maybe I should try it...I have used Eclipse, which works great for PHP. For small projects I just simply use Notepad++ As far as them needing NetBeans or a fancy IDE, it is not required. It can actually complicate things at first and distract them from actually learning. At least that is my opinion. When I started out php I just used notepad until I found Notepad++.
-
Store the post variables in SESSSION on the register page check the session variables which may be populated, if isset print them out. So they do not lose the form data. I would suggest making them re-enter their password no matter what error.
-
Quick Simple Question: How do I test pages?
premiso replied to johnsmith153's topic in Miscellaneous
Another option is, let them know of downtime at like midnight, then "turn off" the site while you upload the files and do a test for the production site. This way users do not get weird errors, it is expected, and you can upload/test your site first. Always keep a backup of the old site just in case something went horribly wrong and you have to revert and do more testing locally. But yes, developing locally is the way to go. If you have many people developing I would look into Subversion. Great software. -
The browser is probably timing out. You can use ob_flush and flush to output items to the browser in iterations, say every 10 loops you echo a "." to the browser. Here is an example of that: <?php set_time_limit(0); // unlimited for ($i=0;$i<10000;$i++) { if (($i%100) == 0) { echo '.'; ob_flush(); flush(); } } ?> This will only work in certain browsers. I know it works on FF 3+. See if that helps you out at all.
-
<?php $content = file_get_contents('http://doerfler.gtamp.de/monitor/index2.php?ip=85.17.200.135&port=4800&ss=s&c=grey'); preg_match('~<td valign="top">P(.+?)</td>~s', $content, $matches); print $matches[0]; die(); ?> Should print: <td valign="top">Players</td><td valign="top">0/20</td></tr> preg_match
-
Selling it on site means you get a huge discount and jack the rate of liquor up. The average uprate of liquor is like 90% or something insane like that. If you want to make more money, it is worth it to get the license and have people pay to buy drinks.
-
Sometimes just writing out exactly what you want to get it can solve an issue. Especially with MySQL. If you only want data from one table, 99% of the time your query only needs to run through that one table.
-
Can you describe to me how it is setup? Does not need to be hugely indepth. Here is what I am getting: You have two tables, the houses you own and the houses you rent. You want to display all the houses that you own and rent, but you do not want to display the houses that you are renting to which you are the owner? Now, I see the inner join, you are trying to join rented rHouse to oID, are these to values coinciding with each other? I take it they are, just had to verify. Essentially that join, is limiting your query. It will show only houses that you are renting because of that portion: $my_houses = mysql_query (" SELECT o.oHOUSE,o.oWILL,o.oRENTED,o.oIMAGE,o.oUPGRADES,o.oID,o.oOWNER FROM house_owned o WHERE ( o.oOWNER = ".$ir['userid']." OR o.oRENTED = ".$ir['userid'].") ") or die(mysql_error()); Now where I am getting confused, is why are you trying to access the house rented table, if you are not even using that in your select statement? I think the query above is what you want. Give it a try and see.
-
[SOLVED] how to add parameters into a header location
premiso replied to otuatail's topic in PHP Coding Help
header('Location: details.php?id=' . $ID); -
It very well could be they were writing it designed for Mysql 3.x, and in that version InnoDB was not enabled by default. That and really a lot of people disregard that cause it makes it a "headache" for some stuff to do. Doing it without the relationship makes stuff a bit simpler when it comes to some tasks, when it comes to others, such as removing a whole section and having it cascade down it makes it a ton easier.
-
WHERE (o.oOWNER = ".$ir['userid']." OR o.oRENTED = ".$ir['userid'].") AND r.rOWNER <> o.oOWNER Try that and see if you get the correct results.
-
You would have to do this via javascript using the onsubmit or do it on the page reload: <?php if (isset($_GET['trackTxtArea'])) { header('Location: http://fedex.com/Tracking?tracknumbers=' . $_GET['trackTxtArea']); exit; } ?> <form id="form1" name="form1" method="post" action="<?php echo $_SERVER['PHP_SELF']?>"> <span>Please enter your tracking number:</span> <div id="trackTxtArea" class="textarea"> <textarea name="trackTxtArea" id="trackTxtArea" cols="30" rows="2"></textarea> <span class="textareaRequiredMsg">Please enter a tracking number</span> </div> <input type="submit" name="trackSubmit" id="trackSubmit" value="" class="trackSubmit"/> </form> Should work for a php example.
-
No clue. My bet is that file_get_contents is not working on your end, for whatever reason. Maybe you are blocked from viewing that site? There are a lot of scenarios that would make that not work. Try using curl to retrieve the web page data and see if that makes it work. If you are trying this on a shared host, chances are they disallow fopen_url which would make the file_get_contents function not work for remote urls.
-
You only use mysql_real_escape_string on data going into the database, not when outputting it to the screen. The whole point of that is to escape characters that will throw errors to MySQL. Check of Magic Quotes is on, if it is I would disable it as it is being depreciated. So the first code you posted, that updates the MySQL DB, on any "string" $_POST data you want to use that mysql_real... function to escape that data.
-
Why are you doing ` around every little thing? It just makes it weird and odd. $my_houses = mysql_query (" SELECT o.oHOUSE,o.oWILL,o.oRENTED,o.oIMAGE,o.oUPGRADES,o.oID,o.oOWNER FROM house_owned o INNER JOIN house_rentals r ON r.rOWNER <> o.oOWNER WHERE o.oOWNER = ".$ir['userid']." OR o.oRENTED = ".$ir['userid']." AND r.rHOUSE = o.oID ") or die(mysql_error()); If you want it to be != you need to use <> in MySQL. See what that gets you.
-
Come on now, no dissing on me. It is not wrong, here it is so you can see for yourself: http://www.emocium.com/test/test.php Tested and working on PHP 4 and PHP 5.