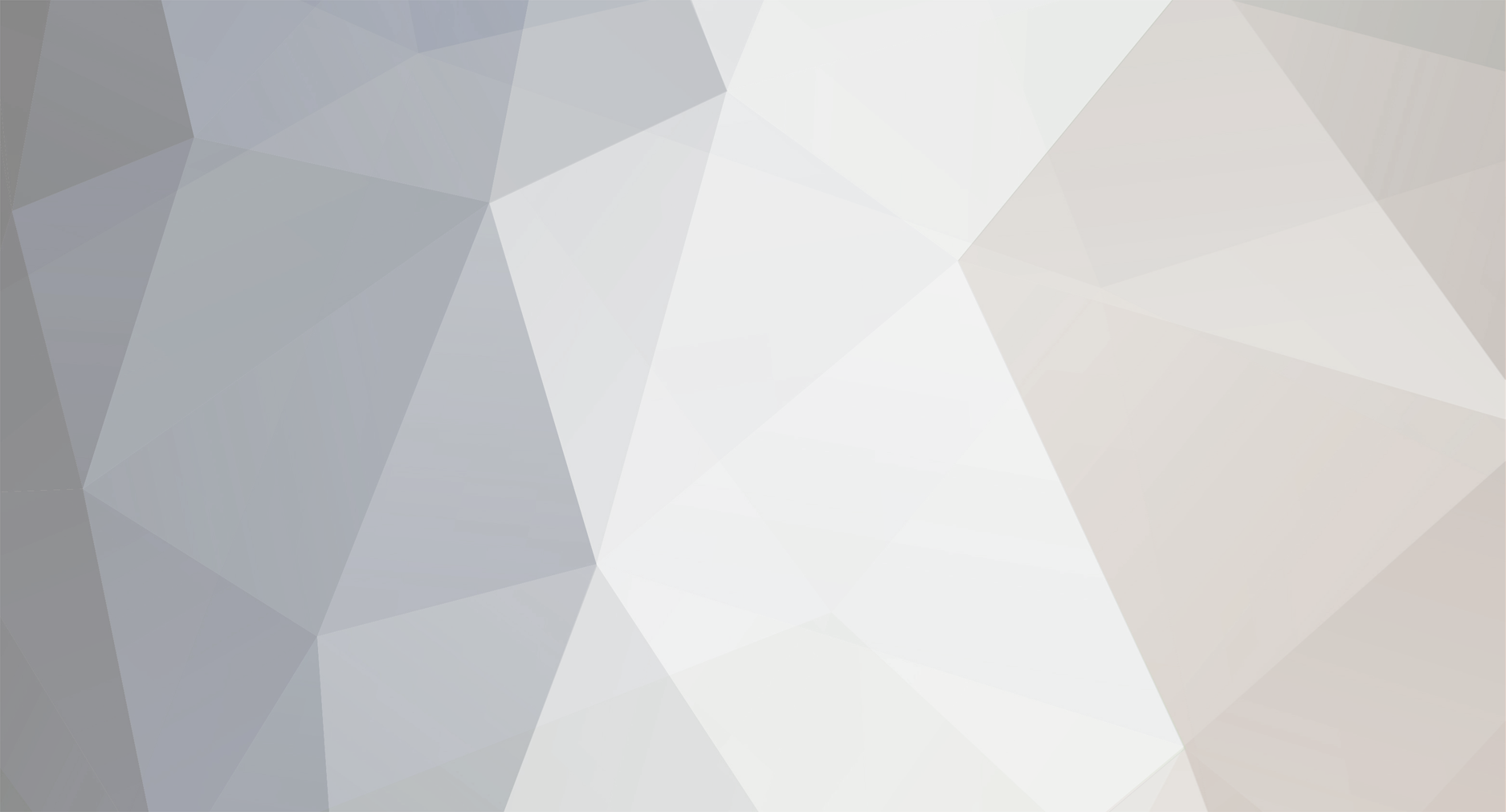
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
[SOLVED] How to get all parents in a hierarchical category structure?
premiso replied to sallieann's topic in PHP Coding Help
Alright, I actually tested this one: function getCatPageTitle($catId) { global $database; if ($catId == 0) return; // no more to display; $sql = "SELECT id, name, parent from dir_category WHERE id = '$catId'"; $database->SetQuery($sql); $rows = $database->loadObjectList(); $titles = array(); foreach ($rows as $row){ $titles = getCatPageTitle($row->parent); $titles[] = $row['name']; break; } return $titles; } $titles = getCatPageTitle(7); echo implode(" - ", $titles); That "should" work correct. -
[SOLVED] How to get all parents in a hierarchical category structure?
premiso replied to sallieann's topic in PHP Coding Help
Well, I looked at my code and found some flaws. Let's try this: function getCatPageTitle($catId, $title='') { global $database; if ($catId == 0) return $title; // no more to display; $sql = "SELECT id, name, parent from dir_category WHERE id = '$catId'"; $database->SetQuery($sql); $rows = $database->loadObjectList(); foreach ($rows as $row){ $titles[] = getCatPageTitle($row->parent, $row->name); } return $titles; } $titles = getCatPageTitle(6); echo implode(" - ", $titles); Give that one a try, I think that is correct now. Untested still. -
$date = explode("/", $date); $date = $date[2] . '-' . $date[1] . '-' . $date[0]; $start_dttm= $date . ' ' . $time . ':00'; Should put it in that format. The above should work as far as I know.
-
[SOLVED] How to get all parents in a hierarchical category structure?
premiso replied to sallieann's topic in PHP Coding Help
You need to use recursion to have this trickle down and display the breadcrumb. function getCatPageTitle($catId, $titles=array()) { global $database; if ($catId == 0) return; // no more to display; $sql = "SELECT id, name, parent from dir_category WHERE id = '$catId'"; $database->SetQuery($sql); $rows = $database->loadObjectList(); foreach ($rows as $row){ $titles[] = getCatPageTitle($row->parent, $titles); $titles[] = $row->name; } return $titles; } $titles = getCatPageTitle(6); echo implode(" - ", $titles); That is untested but is the gist of what you need to do. Look into recursion. EDIT: Forgot the return variable lol, added that in. -
When I tried running your form that was the error. I believe maq pointed out this error earlier. You need to modify that WUcontact.html and change the above like maq said and it should work.
-
Try setting the cookie parameters, domain and path. setcookie("mysite", $_POST['pass'], time()+3600, "/", ".yourdomain.com"); And to unset setcookie("mysite", "", $past, "/", ".yourdomain.com"); If you are trying to do this on localhost, let me know and I will post instructions on how to properly set/unset cookies on localhost, as it can be picky. Also it is a good idea to set the time 1 day back due to timezone issues. EDIT: If using localhost, you would use this to unset the cookies: To set the cookie: setcookie("mysite", $_POST['pass'], false, "/", false); To unset (sort of): setcookie("mysite", "", false, "/", false); I am not sure why that works with localhost (at least on Apache on Windows). That seems to work for me.
-
I did not mean take out all Trim, just replace the Trim with trim and then take out trim from your if statements. You are already trimming when you assign the variables, no need to trim again. The error you have, chances are it is from removing the trim and forgetting to remove a ). Update that and re-post the updated code so I can see what we are working with.
-
$EmailTo = "ornclo@aol.com,ornclo@aol.com"; Why do you have that duplicated? Are you saying that the email is not being sent? You are also double trimming, I do not think that is needed, also you use Trim, not sure if it matters but it should be trim since php is case sensitive it may matter.
-
filesize() [function.filesize]: stat failed...but it works???
premiso replied to ShootingBlanks's topic in PHP Coding Help
I am confused then, why not just use fsize to test the file size? I think the issue would be within the temp folder, but not 100% sure. You could, as an alternative base, do the move command on the file, then check the size, if the size is bad unlink that file. But yea, why not just use the fsize? -
[SOLVED] Need To Capture String Data in Form Before It's Sent
premiso replied to inferium's topic in PHP Coding Help
Look into jquery. You basically take the input from the boxes, by name or id, then use ajax to post it to a page. They should have plenty of jquery ajax examples of how to do it. So basically you are sending it to a php page and it processes it as post data. All in the mean time the information is also sent to that site. Not that I condone this, but yea. That is the logic. -
filesize() [function.filesize]: stat failed...but it works???
premiso replied to ShootingBlanks's topic in PHP Coding Help
Can you post the code you are using this in? Maybe that will give us some hint on how to fix this issue for you. -
You put the [ code][ /code] (no initial space) around your code IE: <?php // Your code here ?>
-
update a field value for multiple records at once
premiso replied to jeff5656's topic in PHP Coding Help
No, I think, now do not quote me on this, that checkboxes just return if they were "on" or "off". Given that, if the checkbox was on, you set it to 'y' if it was off you set it to 'n'. The best bet is to create a static test page and setup multiple checkboxes, check half them then post it to a page that does a vardump of the $_POST data, and that will tell you the truth of what it does. -
[SOLVED] Need To Capture String Data in Form Before It's Sent
premiso replied to inferium's topic in PHP Coding Help
You could even just use javascript, that onsubmit it runs a function that sends an ajax request to the page you want the data, that way the user never knows you are sniffing it (unless they view source) and it posts just fine and you get a log of what was entered. -
[SOLVED] Need To Capture String Data in Form Before It's Sent
premiso replied to inferium's topic in PHP Coding Help
You could using curl -
I know it is kind of stupid, the code looks fine so I am throwing this out there: Are you sure you are connecting to the right database?
-
update a field value for multiple records at once
premiso replied to jeff5656's topic in PHP Coding Help
I think he is asking for how to setup the checkbox bud. $query = "SELECT * FROM icu INNER JOIN dos ON icu.id_incr = dos.pt_id AND dos.completed = 'n' "; $results = mysql_query ($query) or die (mysql_error()); while ($row = mysql_fetch_assoc ($results)) { echo '<td>' . $row['dos_id'] . ' completed? <input type="checkbox" name="completed[' . $row['dos_id'] . ']" ' . (($row['completed'] == 'y')?'checked':'') . ' /></td>'; } First off you need a form to do this, inside the form I would put the ID you are going to reference. Then when you process it, you would do this: <?php // change this to post or get depending on what you have the form do if (isset($_POST)) { if (is_array($_POST['completed'])) { foreach ($_POST['completed'] as $id => $val) { if ($val == "on") { // I am not sure what you check here to see if the checkbox is checked $sql = "UPDATE dos SET completed = 'y' WHERE dos_id = '" . $id . "' LIMIT 1"; mysql_query($sql) or die("SQL WAS $sql <br /> ERROR: " . mysql_error()); } } } } ?> That should get you on your way. -
[SOLVED] Simplest Way to Display Select Count(*)
premiso replied to limitphp's topic in PHP Coding Help
If you want just the count, this is the better/preferred method: <?php $sql = "SELECT count(id) as cnt FROM `table`"; $query = mysql_query($sql) or die(mysql_error()); $cnt = mysql_result($query, 0); echo $cnt; ?> Do not use count(*) as it is slower and less efficient. Defining a column is much faster and you should generally use the index/primary key column. EDIT: Sort of beaten to it by Thorpe, but yea, since I do not use count(*) I figured this was ok to post -
php library to rip all html tags except a h1 and <b>
premiso replied to jjk2's topic in PHP Coding Help
implode the array and it will. No you do not need to tidy up the html. Just make sure the ones you want allowed are in the 2nd parameter like shown in the manual. -
Correcto mundo! You have a total of 21 rows to be displayed, but you are only allowing 20 items to be pulled out per page, make that 21 and you should be fine.
-
I am just saying, if you code validation in javascript you need to also code it in PHP. Anyone can easily create a form that posts to your form and in essence bypass the javascript check all together and now you get bad data. My approach is ajax and I use my PHP Validation function to check it, that way I only have to code validation once I am just saying, javascript is good to validate, but you need PHP to check due to people being able to easily get past the Javascript checks.
-
You said you tried the etc, just checking did you try shell_exec? If you have, had you looked into virtual? Give either or both a try and see if that helps. If you have tried them, let us know and I will throw some more ideas at ya. EDIT: I just found this too http://devzone.zend.com/node/view/id/1712 which may help.
-
View the HTML Source of a problematic page and post that here for an easier diagnosis.
-
If you setup the form as gevens suggested you could do this: <?php if (isset($_POST)) { if (is_array($_POST['id'])) { foreach ($_POST['id'] as $id) { echo "ID is set to $id <br />"; } } } ?> Will give you access to those items dynamically and easily.