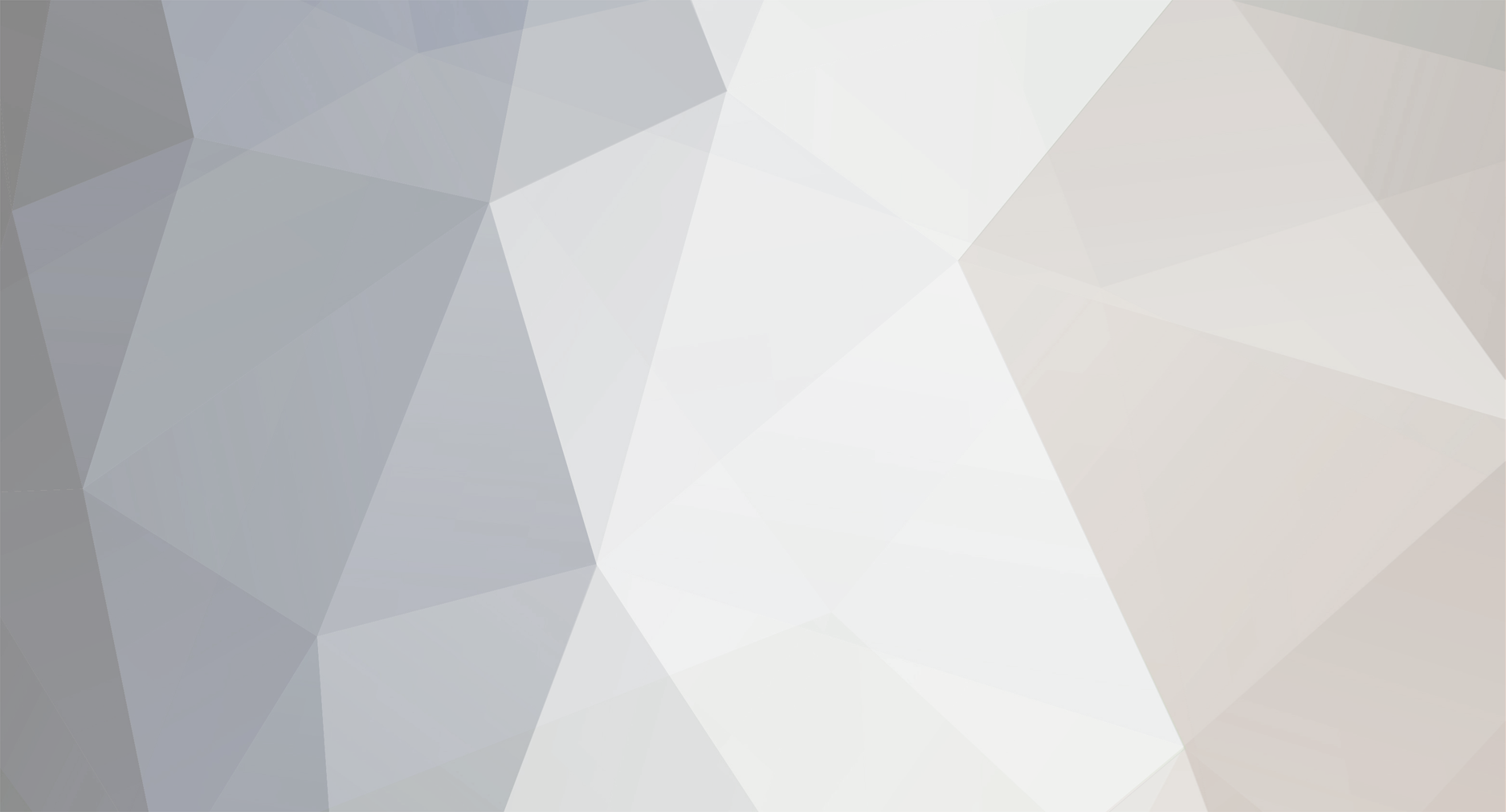
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
In what sense? Are you referring to the $$ command? Really this should not be used, but here is an example: <?php $varname = "var1"; $var1 = "bob"; echo $$varname; ?> The above should echo "bob", however this is why we have arrays... <?php $varname = array("var1" => "bob"); echo $varname["var1"]; ?> Same logic.
-
Weaving in and out makes code a huge mess. I would use echo '' with single quotes and not escape the double quotes and remove the \n's from the code, but yea. I actually tend to store my output in a string then display it after processing. But yea, I shutter at the weaving php tags....yuck. And as far as performance, they are about the same. <?php if(isset($_POST['Submit'])){ $open = fopen("hometext.txt","w+"); $text = stripslashes($_POST['update']); fwrite($open, $text); fclose($open); echo 'File updated.<br />'; echo 'File:<br />'; $file = file("hometext.txt"); foreach($file as $text) { echo $text."<br />"; } echo '<p><a href="../indexDynamic.php">click here to view your changes live</a></p>'; echo '<p><a href="../upload">click here to make more changes</a></p>'; }else{ $file = file("hometext.txt"); echo '<form action="'.$_SERVER{'PHP_SELF'].'" method="post">'; echo '<textarea Name="update" cols="50" rows="10">'; foreach($file as $text) { echo $text; } echo '</textarea>'; echo '<input name="Submit" type="submit" value="Update" /></form>'; } ?> Fixed some outdated issues, first added the stripslashes then changed $PHP_SELF to be $_SERVER['PHP_SELF'] then for the heck of it changed the echos to ' instead of " not to mention I properly indented your code. I would get in the habit of properly indenting code. It makes debugging much easier.
-
scandir, dir and or glob Those are the functions you want to look into.
-
You can always store the path in session then have a page called readImage.php which uses readfile and headers to display the image.
-
<?php // We'll be outputting a PDF header('Content-type: application/pdf'); // It will be called downloaded.pdf header('Content-Disposition: attachment; filename="downloaded.pdf"'); // The PDF source is in original.pdf readfile('original.pdf'); ?> Taken from header <?php // target $url = "http://whatever.com/"; // spoof $refer = "http://whatever.com/"; $ch = curl_init(); // set the target url curl_setopt($ch, CURLOPT_URL, $url); // referrer curl_setopt($ch, CURLOPT_REFERER, $referer); // set user agent curl_setopt($ch, CURLOPT_USERAGENT, $_SERVER['HTTP_USER_AGENT']); // follow curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); // return // curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); // how many parameter to post curl_setopt($ch, CURLOPT_POST, 1); // extra params curl_setopt($ch, CURLOPT_POSTFIELDS, "cc=ASD&key=xxx1234&by=1234134"); // log errors $error = curl_error($ch); // execute curl,fetch the result and close curl connection $result = curl_exec ($ch); // create new file $fp = fopen("data.csv", "x"); // THIS DOES NOT WORK... curl_setopt($ch, CURLOPT_FILE, $fp); // close connection curl_close ($ch); header('Content-type: application/csv'); // It will be called downloaded.pdf header('Content-Disposition: attachment; filename="download.csv"'); // display result print $error; ?> Not sure on the application/csv, but yea. That should give you an idea what to look for if it does not work.
-
Ah 1969, a very good year... From strtotime Although you are using 5.2, your system can be preventing it, if your system does not support greater than 2038 that function will not support it.
-
Just remember if you logged in once, you are valid until you close the browser since you do not have a logout function. You will need to kill the session data to be "logged out" again.
-
Awesome, thanks for that. Maybe I will set it up to just send the important emails, everything else can be sent and get trashed imo lol.
-
Hello All! Well let me layout my situation. I have a dedicated host and instead of using like Squirrel mail I let GMail Hosted handle the email accounts. The issue I am having is that the IP address of my site no longer shows an active MX record for it and the correct MX record is one of GMail server's records. Due to this Yahoo and Hotmail automatically trash the message/do not deliver them. If I use a script like phpMailer to send mail via the gmail smtp, is that kosher since the account is a hosted account? There are around 300-500 emails sent a day from my server and do not feel like getting locked out of GMail hosted. Anyhow, just thought I would ask if anyone had done this trick before. Any input is appreciated! Thanks!
-
mysql_connect, mysql_select_db, mysql_query, mysql_fetch_array You will need to use all of those. You first need a table in the database and the connection info for the database, then create the connection with mysql_connect, connect to the database with the select_db then query the db and put that result into mysql_fetch_array which you will loop through each row using the values in your mail function.
-
Function to make sure blog comments page is nofollow
premiso replied to eits's topic in PHP Coding Help
if(stristr($html,'nofollow') !== false) { return "follow"; } else { return "nofollow"; } Also check that it is nofollow and not no,follow or no-follow etc. But that should work. The !== operator checks that it is truly false, since 0 can be interpreted as a false, this makes sure it is just not at the start of a string. As for bots obeying the nofollow rule, that does not apply to spam bots. They choose to ignore it. That only applies to bots that index your site for search engines really. -
Add more lines or just replace that line with the new variables.
-
Either null or '' or actually defining the columns and omitting the id will work. It is just personal preference. And come on now, be more descriptive then "kept giving me an error". If you wonder why this is still unsolved, that is why, vagueness. What error did it give you? Where did it give the error? And how is it not working?
-
Nope it is not. It depends on preference really. I always define my form to goto which page I want it to, even if it is itself. But that is my preference.
-
Question about Query pulling from Several Tables
premiso replied to limitphp's topic in PHP Coding Help
songID does cause it is a foreign key which links the 2 tables together. As long as songname is not duplicated you are golden. -
mmmk, security issues aside, I would use a hash for the password if this is the case. Here is a revised version: login.php <?php session_start(); if (isset($_SESSION['loggedin']) && $_SESSION['loggedin']) { echo 'You are already logged in.'; exit; } if (!isset($_POST['username'])) { echo '<form name=login method=post action=""> user:<input type=text name=username><br> pass:<input type=text name=password><br> <input type=submit value=go> </form>'; }else { $data = file('passwords.txt'); foreach ($data as $line) { list($user, $pass) = split("|", $pass); if ($user == $_POST['username'] && $pass == md5($pass)) { $_SESSION['loggedin'] = true; $_SESSION['username'] = $_POST['username']; echo 'Logged in successfully.'; exit; } } echo 'Bad username or password provided'; } ?> The username/password file can be auto-generated using this: (remove it after the initial use.) generateInitial.php <?php $fh = fopen('password.txt', 'a'); $data = "admin|" . md5("test") . "\n"; fwrite($fh, $data); fclose($fh); ?> Use that to add any other users you want to the file.
-
This is securer than using a text file and it does not connect to a DB. Using this method you do not risk the chance of someone snooping your site and grabbing the username/password from a standard text file. And technically .php is a text file, just different extension that hides the code to anyone randomly browsing. And to make it even safer, I would put passwords.php in a directory that is not viewable to the outside world.
-
Easy explanation. You were using a web address to include php, the address should only be used on html side to display images style sheets etc. For the internal stuff, including php code, you have to use the actual file server path, not the virtual domain path.
-
session_unregister is not needed. Here is how I would setup the password.php file... <?php if (!$included) { die("You should not be here."); } $usernames = array("admin", "user1"); $passwords = array("admin" => "testpassword", "user1" => "testpassword2"); ?> Then in your login.php <?php $included=true; // set this so it can only be accessed by this script. require_once("password.php"); session_start(); if (isset($_SESSION['loggedin']) && $_SESSION['loggedin']) { echo 'You are already logged in.'; exit; } if (!isset($_POST['username'])) { echo '<form name=login method=post action=""> user:<input type=text name=username><br> pass:<input type=text name=password><br> <input type=submit value=go> </form>'; }else { if (in_array($_POST['username'], $usernames)) { if ($passwords[$_POST['username']] == $_POST['password']) { $_SESSION['loggedin'] = true; $_SESSION['username'] = $_POST['username']; echo 'Logged in successfully.'; }else { echo 'Invalid username or password given.'; } }else { echo "no"; } } ?> Then on your pages you want a user to be logged in add this: <?php session_start(); if (!isset($_SESSION['loggedin']) || !$_SESSION['loggedin']) { echo 'You must login to view this page <a href="login.php">Login Here</a>'; exit; } // if they get here they are a valid user. echo 'Welcome ' . $_SESSION['username'] . '. How are you today?'; ?> Remember username and password must match exactly CaSe wise with the above code.
-
You can access cookies with $_COOKIE...not sure exactly what you are asking, so yea. Decided to just throw that out there.
-
You can use mysql_fetch_assoc and call them by the column names, or simply pull them out of the DB in the order you want.
-
The list is not needed, that just broke out the array. $row is an array that contains all the data that list broke out inside of it's self to be accessed as shown above. I would suggest reading up on array's They are like a storage container for data to be easily access/iterated through.
-
Instead why not just access them via the array index??? while ($row = mysql_fetch_row($xyzQuery)) { for ($i=0;$i<5;$i++) { echo $row[$i]; } } Same principle, just easier and more efficient to do.... What is your logic behind assigning them to variables and not just using the array index?
-
while ($row = mysql_fetch_row($xyzQuery)) { foreach ($row as $val) { echo $val; } } That makes it easier =)
-
imagecopyresized Unsure of how to use it from an image coming out of the DB, but that would be the function/documentation on what you would want to use...