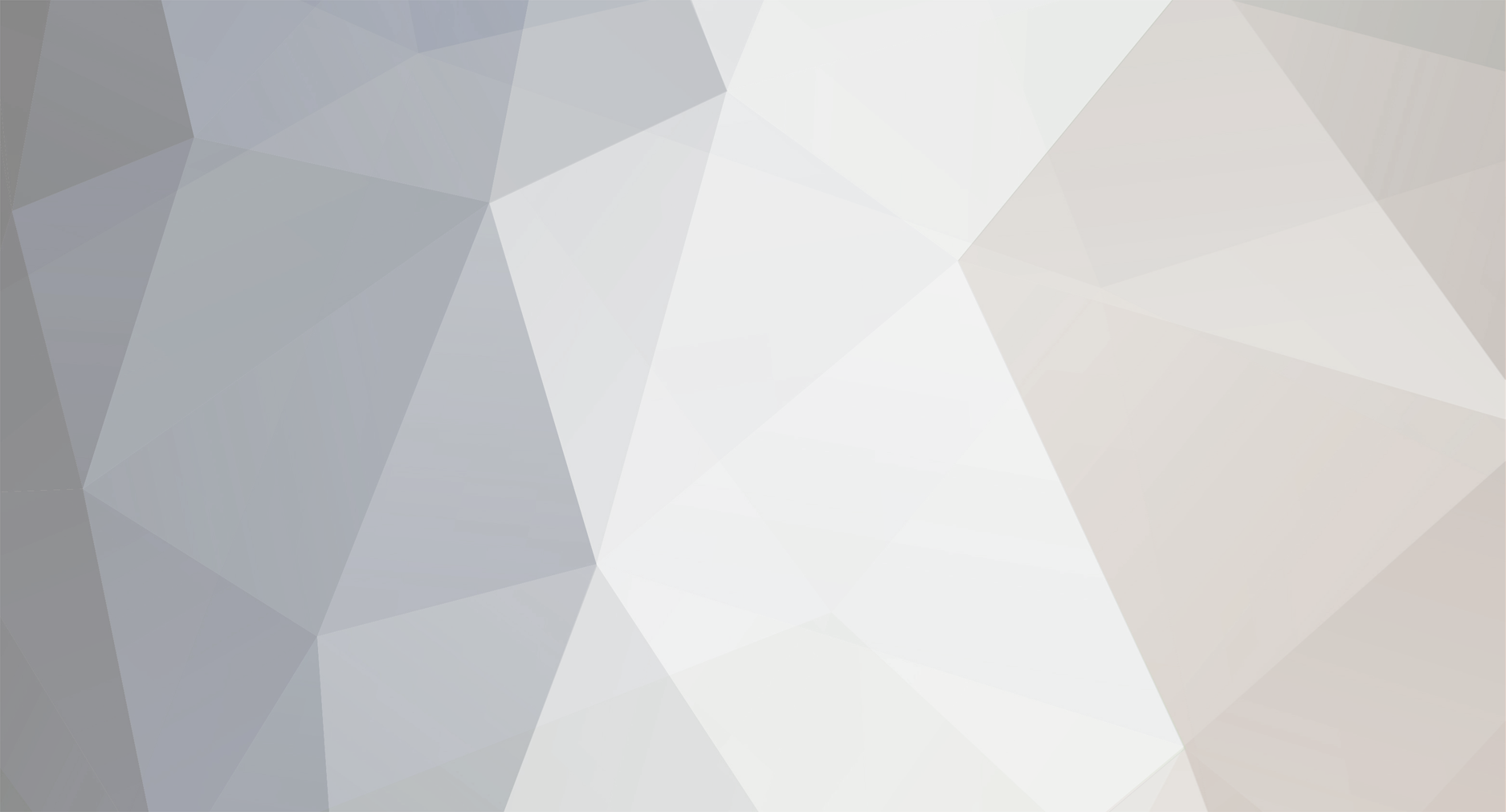
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
You can try it out yourself and see if you can.
-
Help turning domain.com/file.php?id=xxx into an image
premiso replied to seriosbrad's topic in PHP Coding Help
<?php $ip = $_GET["ip"]; $port = $_GET["port"]; $timeout = 1; header('Content-Type: image/png'); function ip($address){ list($ip,$port)=explode(":",$address); if($fp=@fsockopen($ip,$port,$ERROR_NO,$ERROR_STR,1)) { //print "<img src='images/status/up.png'>"; fclose($fp); readfile($_SERVER['DOCUMENT_ROOT'] . "/images/status/up.png"); }else{ readfile($_SERVER['DOCUMENT_ROOT'] . "/images/status/down.png"); } } ip("$ip:$port"); ?> Give that a shot. This is assuming images is located in your webserver root (IE http://www.yoursite.com/images). -
Never used GETDATE, try NOW() instead.
-
SELECT * FROM table1, table2, table3, table4 WHERE condtion=something You may want to look into joins, but yea. There you go.
-
Here is the code, if you want the tutorial go here: Create Directory with PHP and User Input (click to view the tutorial). <?php /********************** File: createDir.php Author: Frost Website: http://www.slunked.com ***********************/ // set our absolute path to the directories will be created in: $path = $_SERVER['DOCUMENT_ROOT'] . '/uploads/'; if (isset($_POST['create'])) { // Grab our form Data $dirName = isset($_POST['dirName'])?$_POST['dirName']:false; // first validate the value: if ($dirName !== false && preg_match('~([^A-Z0-9]+)~i', $dirName, $matches) === 0) { // We have a valid directory: if (!is_dir($path . $dirName)) { // We are good to create this directory: if (mkdir($path . $dirName, 0775)) { $success = "Your directory has been created succesfully!<br /><br />"; }else { $error = "Unable to create dir {$dirName}."; } }else { $error = "Directory {$dirName} already exists."; } }else { // Invalid data, htmlenttie them incase < > were used. $dirName = htmlentities($dirName); $error = "You have invalid values in {$dirName}."; } } ?> <html> <head><title>Make Directory</title></head> <body> <?php echo (isset($success)?"<h3>$success</h3>":""); ?> <h2>Make Directory on Server</h2> <?php echo (isset($error)?'<span style="color:red;">' . $error . '</span>':''); ?> <form name="phpMkDIRForm" method="POST" action="<?php echo $_SERVER['PHP_SELF']; ?>"> Enter a Directory Name (Alpha-Numeric only): <input type="text" value="" name="dirName" /><br /> <input type="submit" name="create" value="Create Directory" /> </form> </body> </html>
-
It is the difference between the date in the DB and NOW. http://dev.mysql.com/doc/refman/5.1/en/date-and-time-functions.html#function_datediff
-
Problem using strtotime to convert two variables into a date
premiso replied to ghurty's topic in PHP Coding Help
Do you mean: {ODAY} to be {$ODAY} ? -
Transferring records from Access to MySQL via PHP
premiso replied to Eiolon's topic in PHP Coding Help
You need to loop the data: http://php.net/manual/en/function.odbc-fetch-array.php while( $myRow = odbc_fetch_array( $rs ) ) { $accessRows[] = $myRow; } Then you will have an array of the results and can use foreach to loop through them and do what you will with them (or you can add that logic inside the while.) -
Yep, using POST / GET data from the form. But you will want to verify that the POST / GET data does not contain bad characters for DIRs (such as / or ' \) as they can make a folder not deletable.
-
Try it out and see. The best way to see if something works is to try it: $hour = 1; $minute = 30; $ampm = "pm"; $time_in_24_hour_format = DATE("H:i", strtotime("{$hour}:{$minute} {$ampm}")); echo $time_in_24_hour_format . " 24 hour time"; If it displays the 24 hour time, it worked. If not, you can use the mktime function instead of strtotime.
-
Cool, I will test out different colored themes, thanks the File God
-
Hello all, I recently setup a new Blog of mine, please let me know what you think. I am currently working on a logo of sorts etc, but any feedback on colors etc is well appreciated! The goal of this blog is to provide different tutorials for items I find in the computing / technology world that will help people achieve certain items and figure out others. I will also be providing reviews of some technologies and the tutorials are not limited to strictly code, but more or less anything computer related. I know it is new and "fresh" which is why I want the critique to improve it to become much more than it is! Thanks. Slunked.com - Help Source for Coders
-
http://dev.mysql.com/doc/refman/5.1/en/reserved-words.html Key is a reserved word. Either enclose the column title in backticks ( ` ) or rename the column name.
-
Sometimes googling yields the results you want: http://www.phpeasystep.com/mysql/8.html
-
You can try adding this to see if the SQL is causing any errors: $result=mysql_query($sql) or trigger_error("Query Failed: " . mysql_error());
-
There is not enough information here to provide you with a reason, how are you getting the values? Are you assuming that register_globals is on and it could be off? Was this working before... But either way, that is not enough information to tell you why you are not getting any results from the query with the PHP code.
-
You will need more than PHP for this. I would look into HTML / CSS and possibly AJAX. As most of this will need to be done on the client side for the visual portion.
-
How can I have some code executed every, say, 30 seconds or so?
premiso replied to DWilliams's topic in PHP Coding Help
If on UNIX / LINUX look into a CRON job http://en.wikipedia.org/wiki/Cron -
The function name of "Date" is most likely causing the problem as date is already a function. Rename that to something like "dateDisplay" and it should fix the issue.
-
syntax for paths in different directorys? (very basic question)
premiso replied to galvin's topic in PHP Coding Help
It is not always the best, as it all depends on how it is coded. An alternative is using $_SERVER['DOCUMENT_ROOT'] to specify the absolute path to the file. But as long as you code it properly either way is fine. -
You would be better off posting a section (10-15 lines max) of the link and the H1 tag you are trying to fetch. It is hard to tell not knowing what is being traversed.
-
$_SESSION['arrays'] = array(); while ($row = mysql_fetch_assoc($result)) { $_SESSION['arrays'][] = $row; } echo "<pre>", print_r($_SESSION['arrays'], true), "</pre>";
-
You need to also incorperate the isset on the ids: $ids= isset($_GET['ids'])?(int) $_GET['ids']:null; $id= isset($_GET['id'])?(int) $_GET['id']:null; Will remove that error.
-
To put that statement in more correct terms, it escapes the double quote since you are in double quotes as if you do not there will most likely be a syntax error. Alternatively you could do this: echo '<a href="' . $url . $post[1] . '" target="_blank">' . $post[2] . '<font size="3">' . $post[3] . '</font></a><br />'; And not have to escape any quotes. But either way works fine.
-
echo "<a href=\"$url{$post[1]}\" target=\"_blank\">{$post[2]}<font size=\"3\">{$post[3]}</font></a><br />"; What was most likely happening is that the target you posted is using Smart Quotes and not straight, those do not get parsed correctly and tend to cause errors.