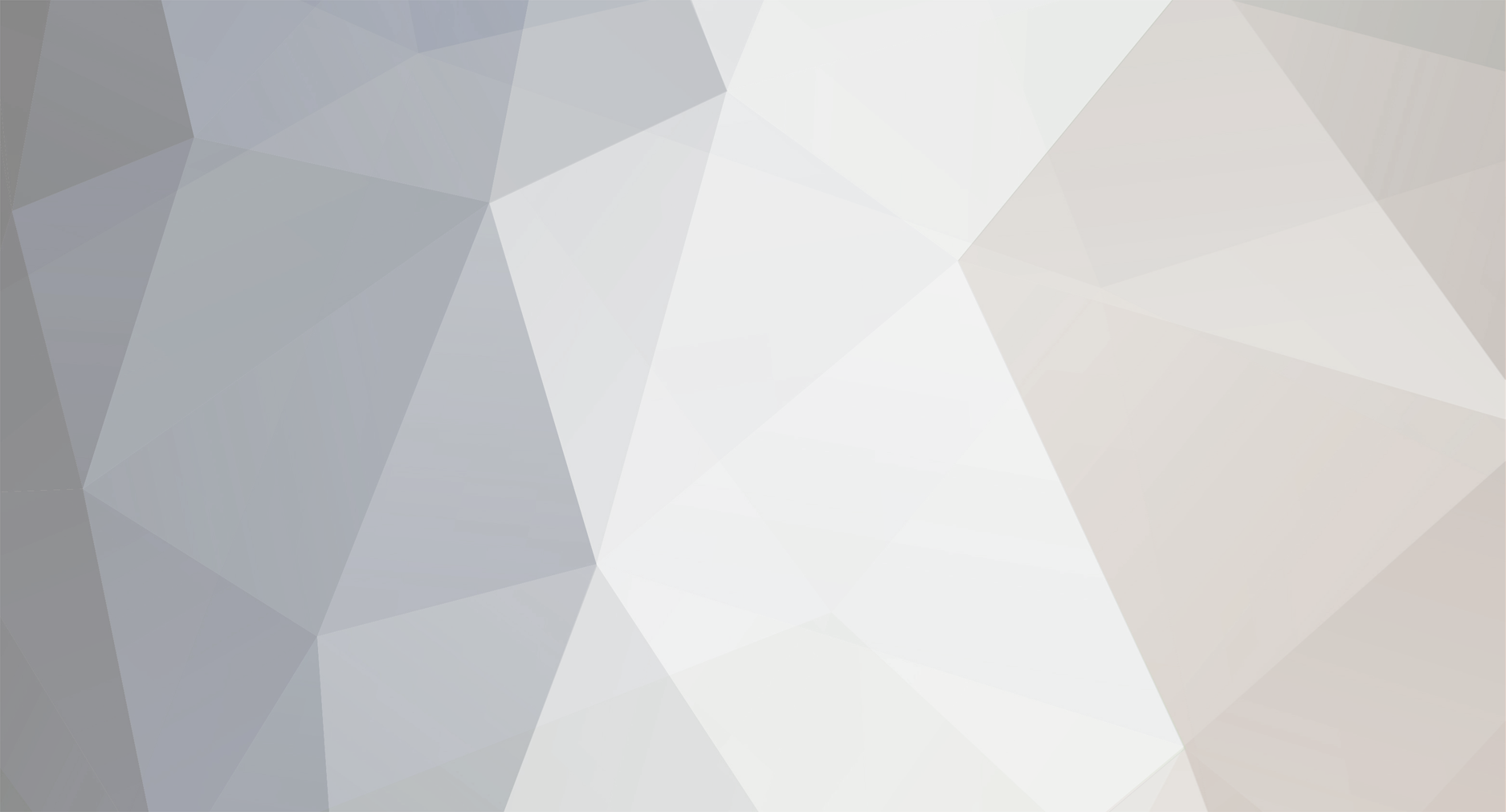
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
To open a window you will need to use Javascript. As far as generating an XML file dynamically from a MySQL database you bet that is possible. If you create a loop of your own markup it would work or even try using simple_xml.
-
file_get_contents or curl would be the most likely utilities to use along with fopen, fwrite, fclose.
-
Well let me throw this out there, in your SQL you are checking firstname against email...should you be checking email against email?
-
You can use glob to generate a dynamic list via an array and loop through it and test/include that if you would like. $files = glob('*.php'); if (isset($_GET['page'])) { foreach ($files as $file) { if (strtolower($_GET['page']) . ".php" == $file) { include($file); } } } Un-tested but it is a rough example of what you can do.
-
The new forum upgrade removed that addon for now as it has to be re-coded etc.
-
Look into the switch statement: switch ($_GET['page']) { default: case 'home': include('home.php'); break; case 'about': include('about.php'); break; } Hope that helps ya.
-
You hit the nail on the head "get" data. $username = isset($_GET['username'])?$_GET['username']:''; echo $username; isset tests if the data has been set, if not the ? and : (ternary operator) is like a short if else, if it was set set $username to the get data, else set it to blank to avoid errors further down the line. Hope that helps ya.
-
<?php mysql_connect('localhost','root',''); mysql_select_db("clients"); if (!isset($_POST['email'])) { $q = mysql_query("SELECT * FROM members"); echo "<select name='email'>"; while ($row = mysql_fetch_assoc($q)) { echo "<option value='{$row['email']}'>{$row['firstname']}</option>"; } echo "</select>"; }else { $row = mysql_fetch_assoc(mysql_query("SELECT * FROM members WHERE firstname = '{$_POST['email']}'")); print_r($row); } ?> Should do it.
-
Post the code to show us where it attempts to create the file. As if it does create the DIR, the file should be created as well...or are you saying it is executing but the DIR is not being put on the server.
-
You would need to use output buffering... ob_start and ob_flush. That should get you what you want.
-
If the spaces are being removed when uploaded then ya, commenting out that line will not work. Instead add this in there: <?php // force to download a file $file = str_replace("\'","'",stripslashes($_GET['link']));//"http://www.crazyjust.com/files/2/20 Fingers - Choke My Chicken.mp3"; $filename = str_replace("-"," - ",$file); $filename = substr($filename,strrpos($filename,"/")+1); header("Pragma: public"); header("Expires: 0"); header("Cache-Control: must-revalidate, post-check=0, pre-check=0"); header("Content-Type: application/force-download"); header( "Content-Disposition: attachment; filename=".$filename); header( "Content-Description: File Transfer"); @readfile($file); ?> And see if that works for you, unsure if it will but it should.
-
No, you cannot open MySQL with pure HTML, I am not sure how ssi works, if they do any back end servers side stuff, so you would have to look more into that part of it. But with just a .html, no it cannot as it is client based coding when you need server side coding to do that.
-
The ! signifies a "not" So if $_POST['submit'] is not set, then execute the code in the brackets. Or if something like this: if ($x != 1) { Will only execute if $x does not equal 1, hope that helps.
-
Flaming you huh? For not posting the error? I see no where that you stated the error at all, I saw someone guess the error...but if you want help try providing decent information and you will actually get decent help. Posting: I have an error, Fix it. Is being a douche way more, as you are basically telling us to look for your issue and solve it instead of providing us with everything you can to help us help you. But it is all good man, I will just stay away from your posts. Have a good one.
-
echo '".($_POST['email'])."'; That is not proper syntax. Fix that to this: echo $_POST['email']; As stated earlier, or if you want the quotes to also be echo'ed out: echo '"{$_POST['email']}"'; But yea, that was the error, you just had improper syntax so of course it would throw a syntax error. You may want to read more up on PHP Syntax to help you avoid this in the future.
-
header You are echoing output before making the header call, you cannot have any type of output before a header call. http://www.phpfreaks.com/forums/index.php/topic,37442.0.html will also be helpful.
-
Just tested the code as is, it worked just fine. Try adding the length to the fwrite and set it to 1048 and maybe that will help. If it does, there is probably a setting on your server somewhere that was causing the issue.
-
Wow that is really helpful. So in order to solve your issue we have to convey our telepathic skills? What was the error given, and maybe you can get some help.
-
It depends on what your database stores TIME as, if it is just the H then yea, if not and it is a timestamp you need to use strtotime to convert it in order to test it. With the poor indentation it is hard to see if you do display anything if the upload is successful, so that could be the reason for the blank page.
-
You are way off on your syntax: <?php $cols = "" foreach( $Array1 as $key => $Value){ $cols .= $Value; if ($key != $SizeOfArray1){ $cols .= echo ","; } } $vals = "" foreach( $Array2 as $key => $Value){ $vals .= "'".{'$Value'}."'"; if ($key != $SizeOfArray2){ $vals .= ","; } } $sql = mysql_query(" INSERT INTO TestTable ($cols) VALUES ($vals)"); ?> Notice in the mysql_query statement you had single quotes ( ' ) around TestTable, in MySQL it should be backticks ( ` ) around column names and tables which are really only needed if you are using a reserved word. Questions let me know.[/code]
-
$last = "SELECT * FROM users WHERE username='{$_POST['username']}' AND last<$time"; And: $sql="INSERT INTO publicgallery (path, name, username, description, thumbpath, last) VALUES ('$filePath','{$_POST['rendername']}','{$_POST['username']}','{$_POST['description']}', '$thumbpath', '$time')"; $result = mysql_query($sql); And: $sql="UPDATE users SET points=points + 1 WHERE username='{$_POST['username']}'"; Those are a few errors you can fix, as will this fix the 500 internal server error, I am not sure.
-
http://dev.mysql.com/doc/refman/5.1/en/reserved-words.html Has a list of reserved words in MySQL, if you want to use them encase the reserved ones in backticks, however it is preferred to change them. $sql = "UPDATE books SET id = '$id', (etc...) `READ`= '$READ, `CONDITION` = '$CONDITION', (etc...)
-
To have a preview you need to store the information in a session variable and use that.
-
You have an error in your SQL, you are using single quotes around tutorials it needs to be back ticks ( ` ) $query= "SELECT name, description, link, username, fullname FROM `tutorials` WHERE id=1"; See if that fixes your issue. The most likely reason for your issue is that you used $row prior in the script and it was pulling that data and since the query error'ed out it did not set $row.
-
Look into wordwrap as that should do what you want.