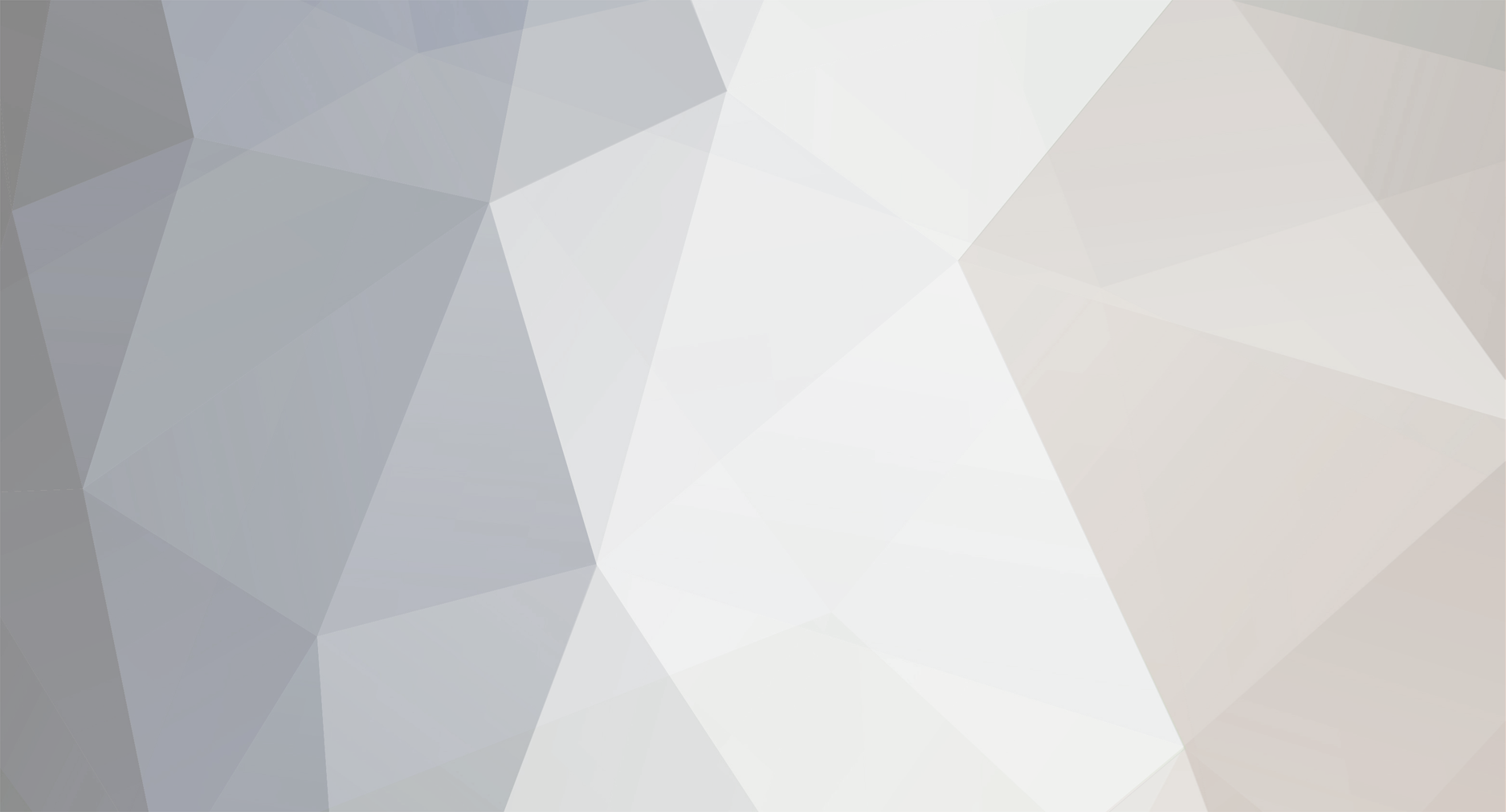
Floydian
Members-
Posts
116 -
Joined
-
Last visited
Never
Everything posted by Floydian
-
[SOLVED] Javascript is driving me nuts. This is like the 20th version
Floydian replied to simpli's topic in Javascript Help
Safari also comes with a built in javascript console. I couldn't tell ya how to get to it, but it shouldn't be hard to do. http://www.jslint.com/ That web site can check your js code. It's owned/run by a Yahoo JavaScript Architect and inventor of JSON. This is a very recommended site. -
$sql = "SELECT * FROM teams LEFT JOIN import_stats ON teams.EmpID = import_stats.EmpID WHERE import_stats.EmpID = '$agentID' and import_stats.date between '$start' and '$end' ORDER BY date ASC"; lol there ya go
-
SELECT id from previous INSERT and INSERT into new table
Floydian replied to cs.punk's topic in PHP Coding Help
$insert_id = mysql_insert_id(); Put that after the insert query in question. Hope that helps. -
lol it's not hefty at all, but it's a bit tricky <?php $count = 0; $a = array(); while (isset($_POST['a' . $count])) { $a[] = $_POST['a' . $count]; $count++; } Hope that helps...
-
<?php $conn = mysql_connect("xxxxxxx", "xxxxxxx", "xxxxxxx"); if (!$conn ) { echo "Could not connect to the database."; } $check = mysql_select_db("xxxxxxx", $conn); if (!$check) { echo "Invalid database name."; } // You've already created the table. Most of the time, you'll create tables outside of // your php scripts. (most of the time...) // $data = mysql_query("CREATE TABLE people (name VARCHAR(30),level VARCHAR(30))"); // You don't have a primary key, so inserting the same exact values over and over again is fine here mysql_query("INSERT INTO people VALUES ('Peter','40')", $conn); // the mysql_affected_rows function will tell you if the insert was succesful if (mysql_affected_rows($conn) < 1) { echo "Insert query failed."; } $data = mysql_query("SELECT name, level FROM people", $conn); // Your SELECT query will likely return more than one ROW from the db. // I'm using LIST() which takes an array (which is what you get when using mysql_fetch_array()) // and stores the array values in variables. I set the result type to MYSQL_NUM // because LIST() works with numeric arrays. while(list($name, $level) = mysql_fetch_array($data, MYSQL_NUM)) { echo "Name: $name and Level: $level<br>\n"; } Hope that helps...
-
[SOLVED] Making advanced search, need help matching criteria
Floydian replied to CrazeD's topic in PHP Coding Help
You're welcome -
How about $sql = "SELECT * FROM teams LEFT JOIN import_stats ON teams.EmpID = import_stats.EmpID WHERE import_stats.EmpID = '$agentID' and import_stats.date between '$start' and '$end' ORDER BY date ASC";
-
The first thing to do is use an alert() function in each of those methods to confirm they are being called. Secondly, style.background should probably be changed to style.backgroundColor since you're just setting the background color and not all of the background properties. Third, I've found far better cross browser functionality by using the hex numbers instead of color names. Forth, the code if (input.focus = true) has a = instead of a == or ===. Fifth, I suspect that using element.focus might not have good cross browser support. I could be wrong, but why not just eliminate the if block since your blur function will cancel anything the focus function does, since it will be called after the focus. I presume you are concerned about the focus function being called and the user quickly clicking over to something else before the focus function finishes. Which, while that can happen, the blur function will correct that for you.
-
You're welcome
-
[SOLVED] Making advanced search, need help matching criteria
Floydian replied to CrazeD's topic in PHP Coding Help
hm. that's a hard road to travel there I personally construct a query using the different search options and then just do one database query at the end. So, how about something like this: <?php // initialize a query string $query = ''; $options = array('name', 'zipcode', 'city', 'music', 'music_genre', 'bar_type'); // The loop lets us check all the posted data foreach ($options as $option) { // if a search option is used, a request var would be available if (isset($_REQUEST[$option])) { $value = $_REQUEST[$option]; // we may want to handle name different from zip code or city switch ($option) { case 'name': $query .= ' and name = ' . mysql_real_escape_string($value); break; case 'zipcode': $query .= sprintf(' and zipcode = %d', $value); break; } } } // since our where clause ($query) starts with "and", // we'll cut that out of the string // we'll also add in the "where" part if (strlen($query) > 0) { $query = 'where' . substr($query, 4); } mysql_query('select blah from foo ' . $query); I should note that the $options array values would have to correspond to the html form element names. so your text inputs or whatever it is you're using would need names like "name" or "zipcode", for this script to work. -
I see two things, number one, you can only pass one query at a time in a mysql_query() function call. Split the create and insert queries into two function calls. Number two, the insert query has double quotes in it which is breaking the string, since your query string is quoted using double quotes. Try replacing the quotes in the query with single quotes, and leaving the quotes surrounding the string with double quotes. A better test for the connection would be: $conn = mysql_connect("*********", "*******", "***"); if (!$conn ) { echo "Could not connect to the database."; } $did_we_find_a_db_to_use = mysql_select_db("**********"); if (!$did_we_find_a_db_to_use) { echo "Invalid database name."; }
-
The reason is due to floating point math and it's documented inaccuracies. Multiply --- 0.2+0.7 --- each of those numbers by 10, add together, then divide by ten, and it'll work as expected. The idea is convert the 0.2 and 0.7 to an integer first (2 and 7) add them, then convert back to floating point.
-
Did you try using single quotes? If that was a response to -- I think you missed my point there. What I said is about where you get the string from, you're talking about where it goes when you're done.
-
You're welcome, and apologies on the explode problem. I would have bet $5 on it working, as I could have sworn I've done that before lol. The foreach loop allows the value to set by reference. Therefore, changing the value of the value changes the value of the value in the array. In this case, &$char in the foreach loop allows me to set the value of $char and that change is reflected in the $exp array. The alternative is using the $exp as $key => $char deal and setting $exp[$key]. The big thing is unset()ing the $char variable since any modification of that var after the foreach loop would still edit the wichever array value it points to.
-
I have no idea on questions >= 2, but on #1 the answer is: The __construct() method is called when you use the "new" keyword. So, when you make an instance of a class, the __construct() method is called allowing you to initialize your objects properties, or whatever else you might want initialized every time you use that class. The topic is labeled global vars (partially), but perhaps you might not know that global vars in PHP are known as superglobals. And there are a few of these available to you. $_SESSION, $_GET, $_POST, $_REQUEST To name a few of them. PHP is designed, IMHO, to be "anti-global". All functions and classes are isolated from all variables out of their scope except for the superglobals. So, if you don't want to use the superglobals as a means of freeing up a variable for use anywhere, I'd suggest having a class that you can carry around with you that stores your variables as public properties. Then you'd only need to use the "global" keyword to pull in that class, and all your variables with it. Personally. since PHP only has single inheritance, I use the __constructor of many of my classes to pull in other classes that I might need in the class. I'd then store those other classes as properties of the class I just instantiated.
-
You make 54 grand a year programming, and yet you're wanting to know how to make more money? Methinks you need to be thankful that in this terrible economy that you are making decent money. And don't forget to give to charity!
-
The easiest way to do something like this, is to import the text into the script. Otherwise, you'll just have to manually escape anything that needs escaping. Luckily for you though, in this case, you can simply use single quotes and the string you have there will be saved to the variable just fine. Just in case you're planning on using that string in an eval(), remember the wise words of the creator of PHP Rasmus Leardorf: "If the answer to your question is using eval(), then you almost certainly did not ask the right question.";
-
Why not use an explode function? Say something like: $blah = 'foooobar'; $exp = explode('', $blah); foreach ($exp as &$char) { if (is_numeric($char)) { $char = ''; } } unset($char); $blah = implode('', $exp); Oh almost forgot, if you put an else on that loop with a break; in it, then you'll get the recursive behavior you were looking for, except that it's not really recursive in this case lol (a plus in my mind )
-
I am not seeing where any variables are being named using the value from the input. I do hope that your php script isn't taking the $_GET['fav'] and storing that using a variable variable name... Since you didn't say where your code is breaking, and I don't see where that would be, it's impossible to give an exact fix, but I can tell you that allowing user input to determine the name of a variable is a horrible idea. And if the variable is a php variable, and not just a javascript variable, you're in for some serious security problems down the road.
-
It sounds like you can get away with just opening that page in an iframe. Since you aren't doing anything more complicated than just showing a media player, it makes sense to load the tv player into an iframe.
-
I highly recommend that you use a javascript library for this. It would only take two or three lines of code to do an ajax request, compared to the hundred or so lines of code you have there. And the big benefit is, the libraries code works from the git allowing you to move on to the good stuff I use and recommend Yahoo YUI. jQuery is another great library as well.
-
How do I make an AJAX rollover button for my current contact form?
Floydian replied to iasiis's topic in Javascript Help
I see you're using jQuery, and since I'm not a jQuery guy, I can't give you the exact code to use here, but I can tell you a method that will do what you want. You will want to attach two event listeners to your button. You need a mouseover and a mouseout event listener. Then, in the event handler functions, you would set the style of button's "background-position:" to the pixel position you want. It follows an x/y deal. Since you would be setting the bg position dynamically, you would use something like element.style.backgroundPosition = '10px, 0'; or something like that. If you aren't sure how to attach event listeners with jQuery, I'm sure you can find some sample code on their site for that. -
$("#username").blur(function() I'm not a jQuery guy, but you should be able to change blur to "change" or perhaps "onchange". Doing that would give the user feedback on the name availability as they type. I know it's more page requests, but is more user friendly than requiring a blur. $.post("include/user_availability.php",{ username:$(this).val() } ,function(data) Again, I'm not a jQuery guy, but what seems odd to me, is the data variable that is an argument in your function there may be an xmlhttl response object. if(data=='no') //if username not avaiable Simply comparing it to "no" may not be enough. Perhaps it's data.responseText == 'no' responseText would be where the text response is traditionally stored in an xmlhttp response object. Since an object wouldn't == 'no', that is a likely cause of the script always saying the name is available. In the php script, you have a security voulnerability. $username=$_POST['username']; You need to wrap that post var in a mysql_real_escape_string(); If you are using magic quotes, wrap that post in a stripslashes and then the escaping function. And the way you do the query isn't exactly the best. $query="SELECT count(*) from `members` where `username`=`$username`"; //Here I check the username $result=mysql_query($query); // I'm going to grab the first array result and store // it in $num_matches which will always be a number in this case list($num_matches)=mysql_fetch_array($result); // If the number is 1 or more, the username is not available if ($num_matches) { //user name is not availble echo "no"; } else { //user name is available echo "yes"; } ?> Good luck!
-
it's because everything you want to do with a single xmlhttp request object is anchored to that one object. if you send out a request using a variable named "ajax" as your xmlhttp request object, and then overwrite that variable with something else, you lose your anchor. If you have a function that is creating an ajax object for you, make it return a new object. return new ajax; like so (it might take a bit more than that depending on the way you have your function setup, but if you do have a function that creates the object for you, make sure to return a new one of what you make )
-
The reason I suggested the click deal was because the user would have to click the image map to move there. So the region they clicked is the one that's of interest. You could alternatively mark that <area> tag with a unique id which your javascript could pick out, and then get the position of that <area> tag. Using that position information, you would then be able to calculate where to place your overlay. From what you've told me, there is no way to predict where the "unit, base, or item" of interest is on any given user's browser page. So, you have to render the page first, and then use javascript to find out where on the page that "unit, base, or item" is. Then place your overlay there. That's how I'd do it. I'm sure there's other ways, like rendering your map using a second unit/base/item image that has the cross hair you want. But if you are going to dynamically place one on top of the image map, javascript and css is the way, and getting the position info is how you do that. Using a region object and a javascript library would make it relatively easy to do.