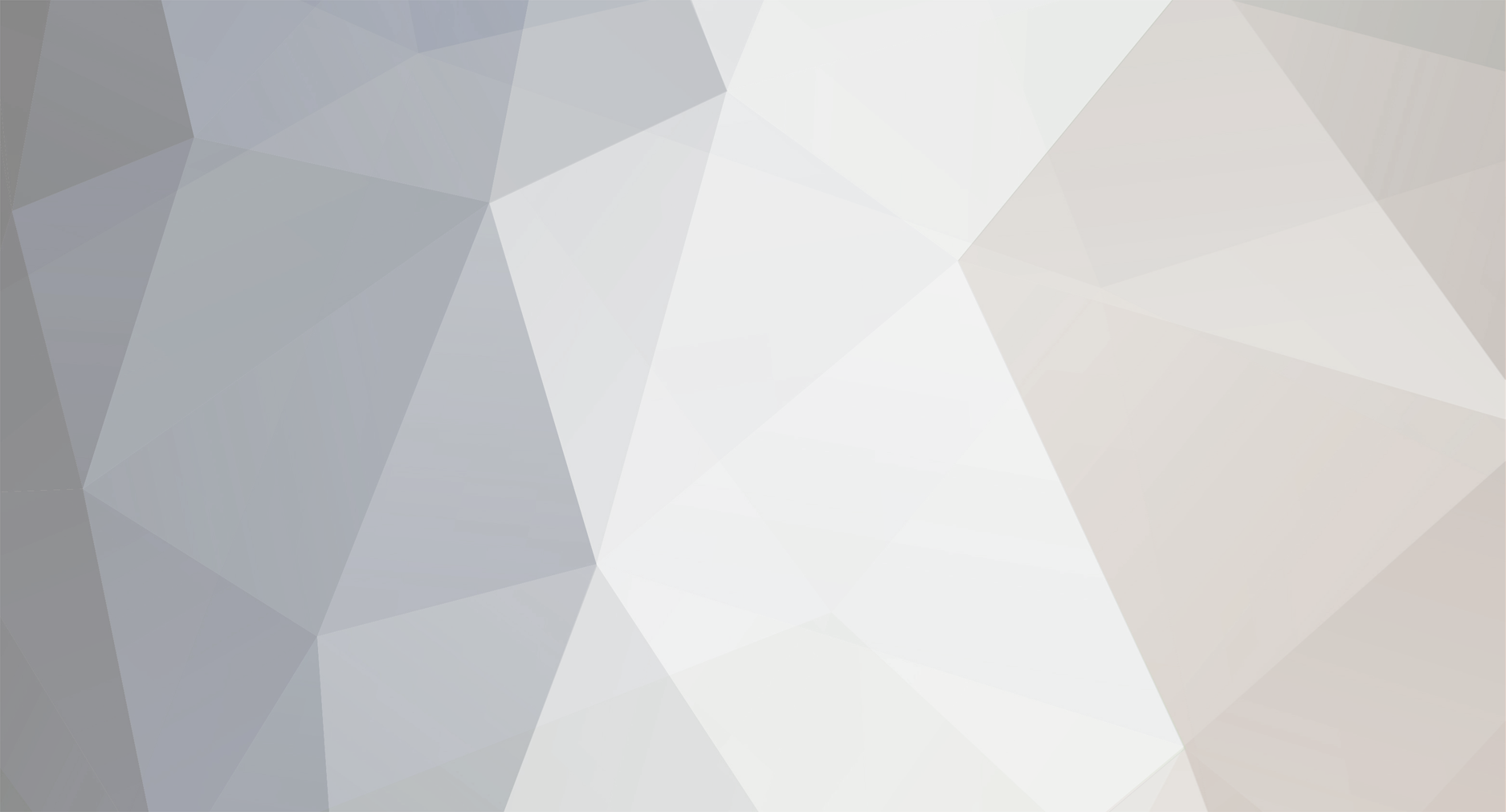
Wolphie
-
Posts
682 -
Joined
-
Last visited
Never
Posts posted by Wolphie
-
-
Not only does it make long queries shorter, most people (including myself) prefer not to inject variables directly into the query so therefore we use sprintf().
-
-
Why are you storing the data in an array? Retrieving data from the database is already in the form of an array, with keys. Therefore you could make the request, loop through the results and return the output directly. Unless you're trying to manipulate hierarchical data in which case multi-dimensional arrays would make sense.
To elaborate, if I had 2 database fields with 2 records in each:
ServerName ServerDescription myserver1 server1 description myserver2 server2 descrption
The results would already be in the form of an array i.e.
$row = array("myserver1" => "server1 description", "myserver2" => "server2 description");
-
You shouldn't really post your database connect details on forums
perhaps a moderator will remove it.
Anyway, back to the point:
<?php if($con = mysql_connect('servername', 'username', 'password')) mysql_select_db('db_name') or die('MySQL Error: ' . mysql_error()); if(isset($_SESSION['id'])) { // make sure the session is set if(is_int($_SESSION['id'])) $uid = mysql_real_escape_string(htmlentities(htmlspecialchars($_SESSION['id']))); // secure the data for inserting into the database! $query = sprintf("SELECT * FROM `db_name` . `table_name` WHERE `id` = '%d' LIMIT 1", $uid); // Make sure it only calls one user $result = mysql_query($query) or die('MySQL Error: ' . mysql_error()); while($row = mysql_fetch_array($result)) { // while loop if(mysql_num_rows($result) > 0) { print $row['username']; // etc... print $row['email']; // etc... } else print 'User does not exist!'; } } else die 'You are not logged in!'; mysql_close($con); ?>
That's how I would do it personally, but your query should be:
$result = mysql_query("SELECT * from members WHERE member_id=' . $_SESSION['SESS_MEMBER_ID'] . '");
EDIT: whizard got there before me =[
-
In order to make them into an easy to use string you would have to make the checkboxes an array.
<input type="checkbox" name="procedures[]" value="Value 1" /> <input type="checkbox" name="procedures[]" value="Value 2" />
Then for the PHP
<?php if(isset($_POST['procedures'])) { $procedures = implode(', ', $_POST['procedures']); echo $procedures; // Output if both were checked: Value 1, Value 2 } ?>
This can then be stored in one database field. And when calling them you could display them however you liked by exploding them again into an array.
<?php $procedure = explode(', ', $row['procedure']); echo '<p>' . $procedure[0] . '</p>'; // Value 1 echo '<p>' . $procedure[1] . '</p>'; // Value 2 ?>
-
DarkWater: Could you possibly provide an example of it in use?
Also, from what I can make out, it's still returned as an array, is it not possible to have unlimited arguments like sprintf() which isn't an array (i don't think) or have I misunderstood?
-
How would I pass unlimited arguments in a function? sprintf() is a clear example of what i'm trying to achieve.
-
Why not put the echo statement inside the same loop? Then you have no need for the array.
e.g.
<?php if ($sql = mysql_query("SELECT name FROM type")) { if (mysql_num_rows($sql) > 0) { while ($array = mysql_fetch_array($sql)) { echo "var " . $array['name'] . " = document.getElementById('" . $array['name'] . "').value;"; } } } ?>
-
What do you mean by combine the same values, do you mean added together? Or just all occurrences removed but 1?
-
No, i'm referring to your use of exit();
-
tapos:
(==) is not a logical operator. It's a comparison operator.
Logical operators are (&&), (||), (!)
-
if($info['url'] = "")
You are using an assignment operator "=" not a comparison operator "==".
if($info['url'] == "")
However, you should be using:
if(empty($info['url'])) { // do something }
or
if(strlen($info['url']) < 0) { // do something }
-
As mjdamato said, always assume that data coming from the user is unsafe and invalid. Also, never give too much information as to why something went wrong. For example, if I had a log in form, and entered a username and password, and the username was correct but it was telling me the password was in-correct, then i'd know I would have the correct username. This is an advantage for people trying to exploit it, as such they would know the username. Therefore, if the username and/or password is in-correct display a notice saying "username or password is in-correct" or simply "log in failed".
<?php if(isset($_GET['id']) && is_int($_GET['id'])) { $id = (int) mysql_real_escape_string(htmlspecialchars(htmlenities($_GET['id']))); $sql = sprintf("SELECT * FROM `db_name` . `table_name` WHERE `id` = '%d'", $id); $result = mysql_query($sql) or die('MySQL Error: ' . mysql_error()); if(mysql_num_rows($result) > 0) { // do something } else { // print 'Invalid ID.'; } } else { // print 'Invalid ID'; } ?>
fook3d:
exit is a language construct, not a function, and therefore parenthesis aren't necessary unless a parameter is required. Such as an error code, or exit('Script Terminated!');
-
Please mark the topic solved.
-
<?php error_reporting(E_ALL); $sql = mysql_query("SELECT `date` FROM `db_name` . `table_name`") or die('MySQL Error: ' . mysql_error()); while($row = mysql_fetch_array($sql)) { // $exp = explode(' ', $row['date']); // Return the date only without the time. $date = date('l jS F Y', $row['date']); print $date; // OR mysql_query("UPDATE `db_name` . `table_name` SET `date` = '$date'"); } ?>
Try that, sorry if i'm not being much help.. I'm at work so it's difficult for me to go in-depth.
-
Yes, this is a JavaScript issue. And just to point out, Java is NOT JavaScript.
If you're going to be validating the form with PHP, then Ajax is required in order to make the requests to the server. However, you can also validate it with JavaScript too.
<form> <input type="text" name="username" id="username" onkeyup="return validate(this.value)"; </form>
function validate(element) { switch(element) { case 'wolphie': window.location = 'users/wolphie.php'; break; case 'jimmy': window.location = 'users/jimmy.php'; break; } // Or.. if(element == 'wolphie') alert('Hello ' + element); }
-
<?php error_reporting(E_ALL); $sql = mysql_query("SELECT `date` FROM `db_name` . `table_name`") or die('MySQL Error: ' . mysql_error()); while($row = mysql_fetch_array($sql)) { $exp = explode(' ', $row['date']); // Return the date only without the time. $date = date('l jS F Y', $exp[0]); print $date; // OR mysql_query("UPDATE `db_name` . `table_name` SET `date` = '$date'"); } ?>
That should work, however don't hold me to it.
-
I take it you're using a unix timestamp to get that date format, i.e. using the timestamp data type in SQL.
You can just use
<?php $date = date('l jS F Y'); // Wednesday 12th August 2008 mysql_query("INSERT INTO `db_name` . `table_name` ( `field_name` ) VALUES ( '$date' )") or die('MySQL Error: ' . mysql_error()); ?>
-
You shouldn't die a custom error, but you should die a mysql error.
This is how a connection should be made
<?php error_reporting(E_ALL); $con = mysql_connect('server_name', 'db_username', 'db_password') or die('MySQL Error: ' . mysql_error()); if($con) mysql_select_db('db_name') or die('MySQL Error: ' . mysql_error()); ?>
Using mysql_error() will explicity give you the specific error as to why PHP can't make a connection to the database.
-
Could someone explain how to get output variables from a regular expression?
For example, if I wanted to retrieve the text from inside BBCode tags. I know it's called from something like $1.
-
Precisely.
-
Unless you're getting all of your content from a database, just do a preg_replace() on that.
-
If you store timestamp in seconds you can do it easily.
e.g.
"INSERT INTO `db_name` . `table_name` ( `user_id`, `timestamp` ) VALUES ( '23', '" . time() . "' )";
Then to check the timeout:
<?php // $uid = $_SESSION['user_id']; // Your user ID variable $sql = "SELECT `timestamp` FROM `db_name` . `table_name` WHERE `user_id` = '" . $uid . "' LIMIT 1"; // timestamp should be an integer (INT) $res = mysql_query($sql) or die('MySQL Error: ' . mysql_error()); while($row = mysql_fetch_array($res)) { $timeout = 5 * 60; // 5 minutes if((time() - $row['timestamp']) < $timeout) { // Remember, if big time minus little time is less than timeout // do something } else { // do something else } } ?>
-
Without the use of Ajax, the page would need to be refreshed every time the button is clicked in order for PHP to update it. However, I would always recommend a JavaScript library to do most of the work for you.
To get an idea of how to use Ajax and PHP take a look at http://www.w3schools.com/php/php_ajax_suggest.asp
[SOLVED] get value of Input type image in IE
in PHP Coding Help
Posted
Try giving it an ID also, I've heard that PHP also uses ID's as keys. However I may be mistaken.