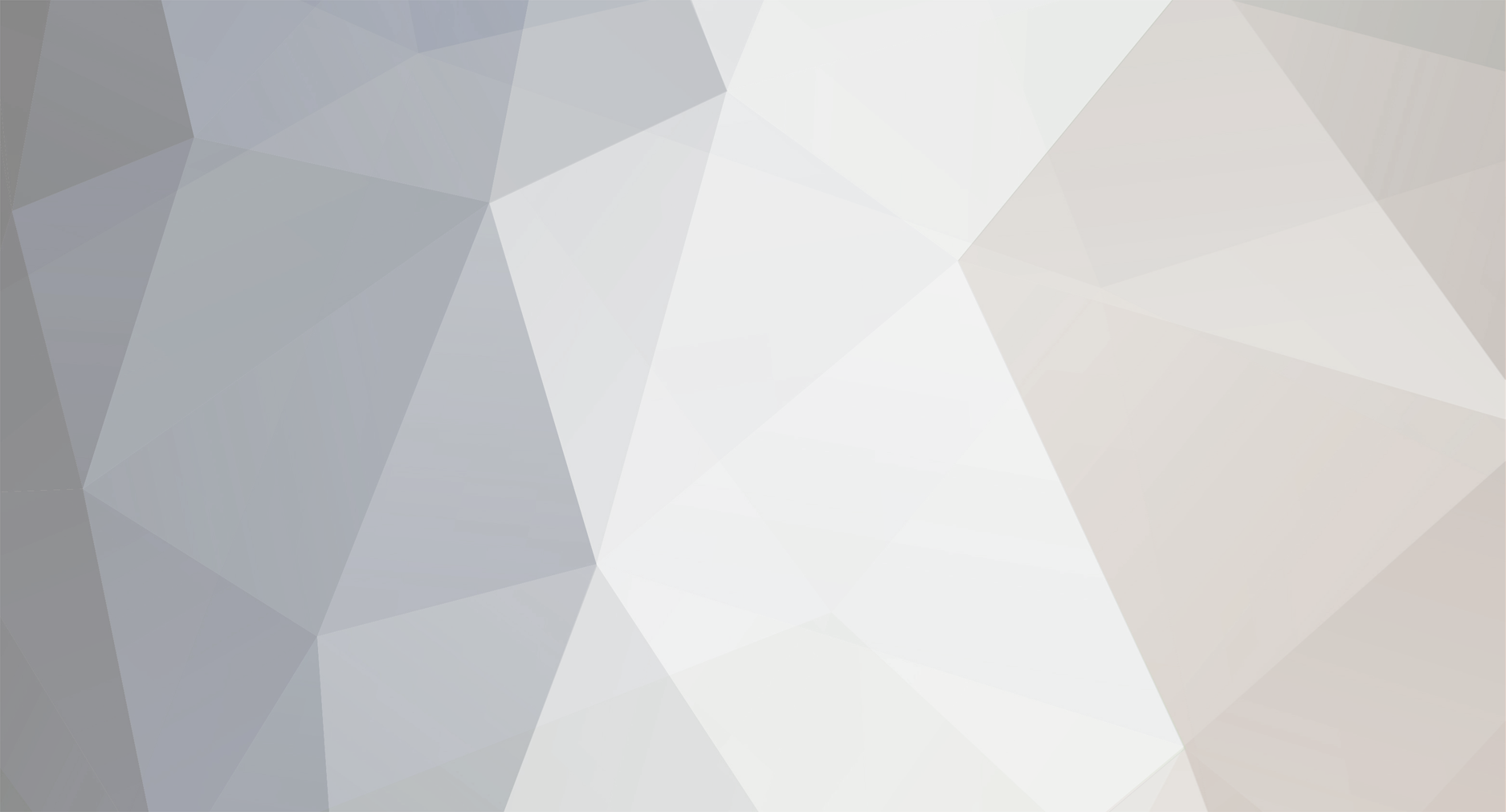
Wolphie
-
Posts
682 -
Joined
-
Last visited
Never
Posts posted by Wolphie
-
-
How does posting first justify it? And if you read, correctly. I wasn't suggesting his code. You didn't provide any evident help which could prove useful to the person asking the question, it's not exactly considered as a contribution. And by idiotic I meant your attitude, alright yes, you made a point which could have been put across better. But you didn't provide a solution. All in all, he didn't use the original method I proposed so therefore, discussion closed.
-
Well that's the fun and beauty of programming. The limitations are pretty much endless. There's usually always a way around something. Whether it be the most efficient method or not, it's still a method! Then when you learn more, you can just come back and refine it by perhaps using some of the new techniques you've learnt.
Programmers are never happy with their code, they will always come back and change parts of it, however small. Except when the boss is telling your a** to get it released.
-
It's called a "heredoc" string.
PHP reference: http://uk.php.net/types.string
However, I might have use it in-correctly. I don't usually use arrays with it.
<?php include 'opendb.php'; $currIndex = $_GET['index']; $query = "SELECT * FROM `reviews` WHERE `m_index` = '$currIndex'"; $result = mysql_query($query) or trigger_error(mysql_error()); // ALWAYS use error handling while($row = mysql_fetch_array($result)) { echo <<<DBRow <img src="{$row['m_Link']}"> {$row['m_Title']} <p> {$row['m_Review']} <br> DBRow; } // No need for mysql_close(). PHP automatically terminates all open connections when the script ends. ?>
Give that a try.
EDIT: Yep, my first post was correct. Arrays should be enclosed in curly braces.
-
I read all through that part of the manual and didn't pick up on that...
Haha, funny you say that. That's why I always have PHP.net and MySQL.com bookmarked! Plus I usually have a couple of tabs open for each. Just keep at it, once you use it once you'll usually get the hang of it and only use the documentation for reference. But hey! That's what it's there for! Right?!
-
Just to be sure, are you positive that the image path's are correct? Have you tried linking directly to one from the browser?
-
I always use heredoc strings for long multi-line block strings.
<?php include 'opendb.php'; $currIndex = $_GET['index']; $query = "SELECT * FROM `reviews` WHERE `m_index` = '$currIndex'"; $result = mysql_query($query) or trigger_error(mysql_error()); // ALWAYS use error handling while($row = mysql_fetch_array($result)) { echo <<<DBRow <img src={$row['m_Link']}> {$row['m_Title']} <p> {$row['m_Review']} <br> DBRow; } // No need for mysql_close(). PHP automatically terminates all open connections when the script ends. ?>
-
Just try this and see if it gives you an error in regards to what the problem is.
$query = "INSERT INTO `account_activity` ( `userid`, `AccountSelector`, `amount_to_send` ) VALUES ( ' $afso_userid', '$AccountSelector', '$ammount' )"; $result = mysql_query($query) or trigger_error(mysql_error());
-
From what I understand, you want to return only a single result even if there are more results of the same criteria. I'm not really sure how you want to organize it; but there are plenty of SQL statements that can organize it by ID etc..
<?php $model = $_REQUEST['model']; if(isset($model)) { $query = sprintf("SELECT `mod_desc` FROM `module` WHERE `type` = '%s' LIMIT 1", mysql_real_escape_string($model)); $result = mysql_query($query) or trigger_error(mysql_error()); if(mysql_num_rows($query) > 0) { while($obj = mysql_fetch_object($query)) { print $obj->mod_desc; // You were calling it by the wrong name, dammit those 2 got there before me >.< } } else { print 'No results!'; } ?>
I'm not entirely sure what you are looking for, if this is not acceptable. Please try to make yourself clearer.
-
No offense dannyb785, but you're criticizing peoples code and their efforts to help this person. Yet what exactly have you contributed? Apart from idiotic criticism.
If you're going to do that, make sure it's constructive criticism which will help people better their programming skills. Not everybody has an extensive experience.
-
Just out of curiosity, have you checked out the highlight_file() function? It's a built-in function that highlights PHP code and formats it according to how you have written it.
http://uk2.php.net/manual/en/function.highlight-file.php
-
Your problem is this line:
$where = ' WHERE type = \'' . $model' . ''';
You'd make your life much easier if you were to enclose your query string in double quotes; you then wont have to worry about escaping the single quotes around values in the query:
$sql = "SELECT mod_desc FROM module WHERE type='$model'"; $result = mysql_query($sql) or trigger_error(mysql_error());
I agree with danny with regard to the fact that using 3 variables is pointless. Apart from anything else, it makes it harder to follow.
I also like to enclose database references in back quotes.
e.g.
$sql = "SELECT `mod_desc` FROM `module` WHERE `type` = '$model'"; $result = mysql_query($sql) or trigger_error(mysql_error());
I find it makes distinguishing between SQL code and PHP easier.
-
No I haven't tested my code. And I do not need to because I know it works. It will write the contents of any file you specify into the browser in plain text. However, it will NOT be formatted like it would when opening the file in a text editor. You will need to write additional code to accept the line breaks.
It won't display the output of the PHP file which is being read because that particular code isn't being parsed by the PHP Engine, the read code is.
-
its correct code.
just change file name to read.php.
dats all.
<?php $file = 'read.php'; $handler = fopen($file, 'r'); $contents = fread($handler, filesize($file)); print $contents; fclose($handler); ?>
Precisely, fopen() can read any kind of file. I use it often to read .torrent files and use my BEncode library I wrote to get the Meta-Info from it. However, you won't be able to write to this file without the necessary permissions on the server.
-
The only form of a goto command I've seen in PHP is continue. It can accept optional numeric arguments, however this is only used in loops to skip the current iteration.
Example:
<?php while (list($key, $value) = each($arr)) { if (!($key % 2)) { // skip odd members continue; } do_something_odd($value); } $i = 0; while ($i++ < 5) { echo "Outer<br />\n"; while (1) { echo " Middle<br />\n"; while (1) { echo " Inner<br />\n"; continue 3; } echo "This never gets output.<br />\n"; } echo "Neither does this.<br />\n"; } ?>
Reference: http://uk2.php.net/manual/en/control-structures.continue.php
-
<?php $file = 'somefile.txt'; $handler = fopen($file, 'r'); $contents = fread($handler, filesize($file)); print $contents; fclose($handler); ?>
I think that's what you're looking for.
-
I would agree with Daniel, however... If you can't afford a VPS or haven't got the knowledge to set one up yourself I would strongly recommend http://www.webfaction.com/
They're cheap, and have good plans. They allow PHP and Python and Ruby, PostgreSQL and MySQL. Also they provide almost complete DNS control and not only that you can pretty much have them install anything on the server for you if you require it.
-
If you want my honest opinion, I'm not really sure. I don't work with eCommerce at all. I don't really like working with sensitive information, especially credit-cards. And if that is the case, I would use PayPal as a gateway. But one thing is for sure, USE SSL. Also, try doing some research on google about how PayPal, and other payment gateways do it.
P.S. Could a moderator/administrator remove my second post above.
-
Wolphie, ... whoa.
Lol, huh, what?
br0ken: That isn't a really failsafe method. As you said, it needs the ability to be decrypted and in which case, would make it a perfect target for hackers. Seeing as it is *supposed* to be decrypted. If they get hold of the method you use, you're f***ed. Things that are encrypted aren't supposed to be decrypted, especially sensitive information like that. They should be sent to a secure e-mail address which makes use of SSL authentication.
-
Wolphie, ... whoa.
Lol, huh, what?
br0ken: That isn't a really failsafe method. As you said, it needs the ability to be decrypted and in which case, would make it a perfect target for hackers. Seeing as it is *supposed* to be decrypted. If they get hold of the method you use, you're f***ed. Things that are encrypted aren't supposed to be decrypted, especially sensitive information like that.
-
Why would they need to be decrypted again?
EDIT: Also bear in mind that when people submit forms, using POST or GET methods all data is sent in plain text any way unless the website has an SSL certificate and is SSL secured.
-
No, it doesn't work like that.
When a person registers, their password is encrypted and then written to the database. Then when they log in, the password is again encrypted using the same method and then compared to the one already in the database for validation. It will never appear in plain text again unless they had some kind of rainbow table (which people use to decrypt MD5 hashes).
-
Please in future use the code BB tags. And just out of curiosity, why not just create your own algorithm? Just use other encryption methods to create your own. That's what I do, then it's unlikely for someone to crack it unless they know exactly what method you use.
I use this for passwords:
function secure_password($password) { $password = $this->secure($password); $password = md5(sha1('!h6' . $password . substr($password, -1) . substr($password, 0, 2))); $password = sha1($password . '@^$' . md5($password)); $password = str_rot13(substr($password, 3) . 'zFq'); $password = sprintf('%s%x', $password, crc32($password)); return $password; }
-
I don't really see your problem..
if(($_GET['s'] == 'news') && (in_array($_GET['s], $array)) { // successful } else { // failed }
-
You should be using mysql_real_escape_string() especially when it comes to storing sensitive information. You want everything as secure as possible. I always avoid adding slashes regardless anyway.
But as the previous posts have stated, post your code.
Database Design Question
in Application Design
Posted
In terms of BOOLEANS for databases (including relational databases), numeric values are always best. 1 = true, 0 = false. Just like binary.
If it goes above 0 and 1, then the same rule still applies. Just use a switch statement.
I don't know if that's sufficient enough. But that's what I would do.
I would also construct the hospital table slightly differently too.
id
pid
kpid
wid
time_left
hp (Current hp (should rise in stages) in hospital)
I would also have a purchasing history to determine which player has which weapon.