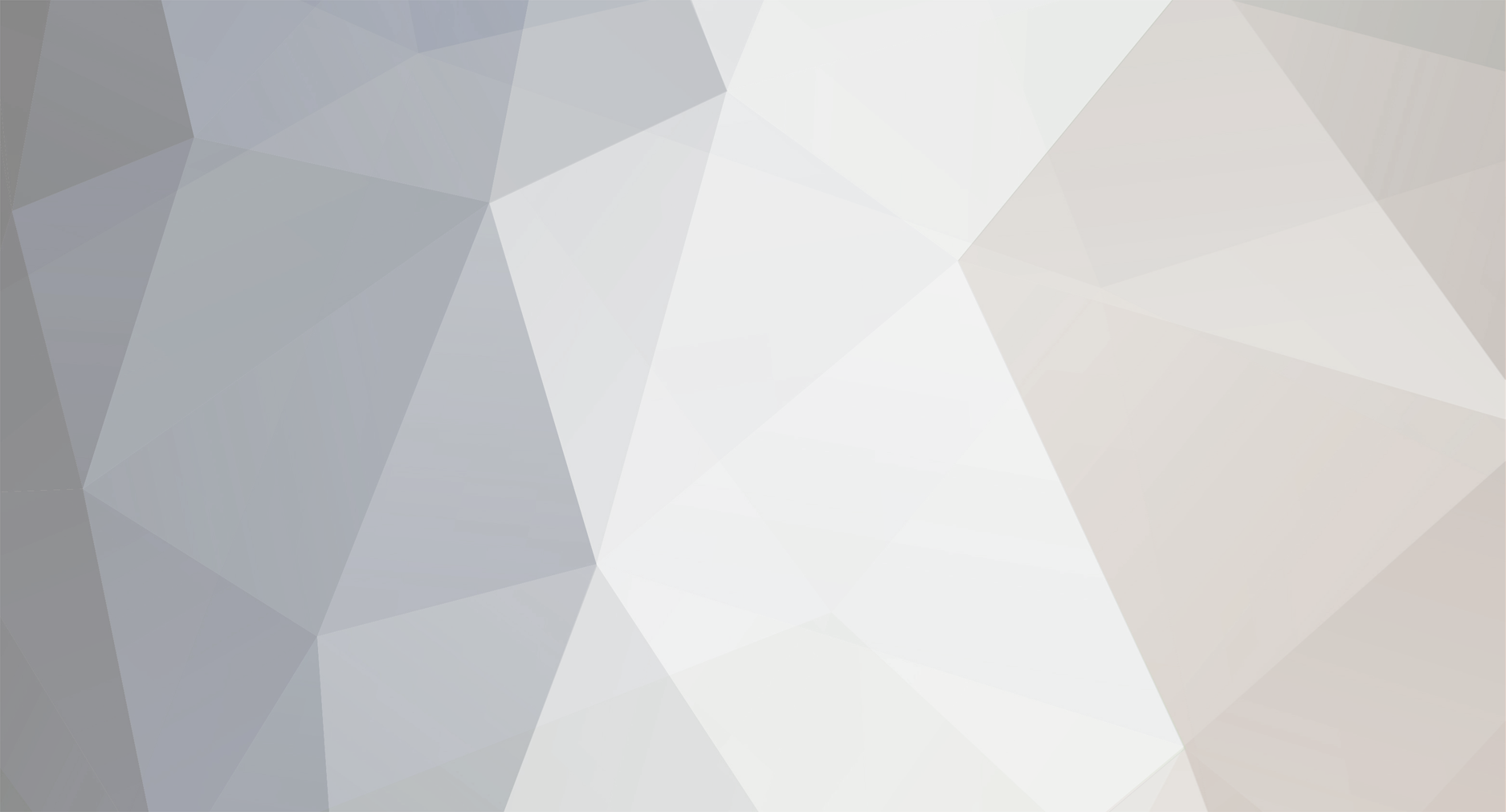
Wolphie
-
Posts
682 -
Joined
-
Last visited
Never
Posts posted by Wolphie
-
-
Yeah, as craygo said, always use error handling. I didn't realise you forgot mysql_error on the actual query, I assumed you did because you had it on the initial database connection.
-
Put this at the top of your script
error_reporting(E_ALL); ini_set('error_reporting', 'on');
Then let us know if you get any errors.
-
Use array_diff()
<?php $array1 = array("a" => "green", "red", "blue", "red"); $array2 = array("b" => "green", "yellow", "red"); $result = array_diff($array1, $array2); print_r($result); ?>
Result:
Array ( [1] => blue )
Manual: http://uk3.php.net/manual/en/function.array-diff.php
-
We're not here to write scripts for you, regardless of whether you're a beginner or not. We will however provide help and assistance if you have/need any help with the code you've written or don't understand. For us to help you, you need to show at leat some effort and commitment to some degree.
papaface provided you with your answer, google is the best place to find what you're looking for. If you really want someone else to write it for you then go to the Freelance forums.
-
It is possible to return HTML inside a PHP function yes, although I don't see why you would want to return an entire table in PHP. I can understand if you actually wanted to populate a table using PHP.
-
Have you actually tried viewing the pages source code? Considering that is a HTML comment.
-
If you want some advice, use a PHP framework, something of a MVC (Model, View, Controller) architecture. It should help you manage your code, and help seperate large blocks of your code from the initial design. My personal favorite is Zend Framework, or Drupals built-in framework.
-
This is not true, you don't have to 're-make' the page, you only need to refresh it, unless you use JavaScript, an in which case would allow you to use Ajax to automatically query the script every 5 minutes or something for new images.
A simple example:
<?php $dir = dir('images'); while (($file = $dir->read()) !== false) { if(($file != '.') || ($file != '..')) { print '<img src="' . $file . '" border="0" />'; } } $dir->close(); ?>
This is just a very basic example of how to list files in a directory. In order to dynamically resize an image to create the thumbnail, you would have to use GD Library, or ImageMagik.
You could also upload the images into a BLOB on a database, or just store the image paths. The possibilities are endless, it may be tough although it's worth learning!
-
What do you mean, without being generated by a software again?
-
Etiquette schmetiquette...
Just some basic decency should be enough.
Agreed. It just goes to show how a long and detailed explanation could be out weighed by a short and simple answer. I personally feel a sense of accomplishment also, and try to help as much as I can even when I don't fully understand the code, the explanation, the desired result etc..
-
Yes this is possible, although it would be done in JavaScript. In which case this is the wrong forum.
If you want to display an image stored in the database then you would use AJAX (JavaScript, XMLHttpRequest)
-
<?php $to = 'test@domain.co.za'; $email = $_POST['email']; $info = $_POST['message']; // this should be the same as the name on the form element $message = <<<MESSAGE <html> <head> <title>HTML E-mail!</title> </head> <body> <table border="1"> <tr> <td>$info</td> <td>This is an HTML e-mail!</td> <td>This is an HTML e-mail!</td> </tr> </table> </body> </html> MESSAGE; $subject = 'HTML E-mail!'; $headers = 'MIME-Version: 1.0' . "\r\n"; $headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n"; $headers .= 'From: ' . $email; mail($to, $subject, $message, $headers); ?>
-
This isn't a failsafe method. A lot of people have HTML formatting switched off for their e-mails, in which case if you were to send a HTML formatted e-mail they would get thoroughly confused. You should provide an option for your users to select which format the e-mail should be in. And in which case, if they make a mistake it's down to them. And you'll then be able to determine which format the e-mail should be sent in, either plain text or HTML.
But back to your original question:
<?php $to = 'example@domain.com'; $message = '<html><head><title>HTML E-mail!</title></head><body>This is an HTML e-mail!</body></html>'; $subject = 'HTML E-mail!'; $headers = 'MIME-Version: 1.0' . "\r\n"; $headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n"; $headers .= 'From: anotherexample@domain.com'; mail($to, $subject, $message, $headers); ?>
-
Could you please provide the code relevant to the question and the desired result? Without it, we can't help you.
-
Since you're running a mac, I would first suggest downloading and installing a useful IDE (intergrated development enviroment). For the mac I use either Xcode development tools (download from the apple developer connection website free, version 3.0 for OS X 10.5) or TextMate, which isn't free although it's better than Xcode in my opinion. With these you'll be able to create a range of different files.
But back to your original question, it isn't difficult. For example, when you open say notepad on windows and type something and then save it. It'll ask you to enter a name to save it as, and it'll have a .txt extension. You just change this .txt file extension to .php.
The best resource for learning PHP would be PHP.net
He has a point though, if you don't know how to do something in which a job requires then you don't need that job. Epically since there is probably vary highly qualified PHP programmers that need work **cough**me**
How is that relevant? If you're going to criticise people, at least offer a solution rather than just being an a** about it. At the end of the day, we were all beginners at some stage. None of us knew how to do any of this, until we put in the effort to learn and asked for guidance from more experienced people along the way.
-
You won't be able to prevent users from directly downloading videos unless you use a password protected directory or store it outside the web root as the previous posts have stated. Though generally, when you stream videos they're stored in the cache anyway.
-
What do you mean by 'delete the files when the page was uploaded?'?
-
Yes, this is possible. I quite often have to access our intranet from home through a VPN. Though unless you have very important documents on the intranet database, it really isn't worth the hassle.
-
Try running an update query by itself, without any predefined variables. And see if it updates the database. If that fails, try a regular update query without the MySQL class.
-
Have you tried to echo the parameters to see if the variables are even getting set before calling the function?
<?php function updateContent($pgname, $content, $id) { echo $pagname . '<br />'; echo $content . '<br />'; echo $id; $db = new sql(); // Always add error handling $result = $db->sql_query("UPDATE `content` SET `pagename` = '$pgname', `content` = '$content' WHERE `id` = '$id'") or trigger_error(mysql_error()); if($result) return $result; else return false; } // Then when calling your function use a condition with error handling if(updateContent($_POST['name'], $_POST['rte1'], $_GET['update'])) { echo 'Updating...'; else echo 'Could not update!'; ?>
-
You're not being very clear, but it's because you're still using parentheses.
Here's an example of how it should be done.
<?php $myVar1 = 1; // An integer $myVar2 = 1; // A string if($myVar1 == 1) { echo 'My variable is an integer, Wahoo!'; } if($myVar2 == '1') { echo 'My variable is a string, Wahoo!'; } ?>
They'll both display the message regardless of ' ' because PHP is a loosely typed language unlike C++ and stuff.
Yes, PHP is a loosely typed language. However, declaring and comparing variables should, in my opinion be done the way it's supposed to be done. In my experience, it makes debugging much easier, and should reduce the chances of problems such as declaration issues, syntactical/parse errors from occurring. But again, that's just my opinion. I'm pretty strict when it comes to writing clean and easy to read code.
-
You're not being very clear, but it's because you're still using parentheses.
Here's an example of how it should be done.
<?php $myVar1 = 1; // An integer $myVar2 = '1'; // A string if($myVar1 == 1) { echo 'My variable is an integer, Wahoo!'; } if($myVar2 == '1') { echo 'My variable is a string, Wahoo!'; } ?>
Also stick error handling on.
error_reporting(E_ALL);
Also use mysql_error() for your queries.
$query = mysql_query("query...") or trigger_error(mysql_error());
-
Or you could alternatively use PHP's built in templating engine.
<html> <head> <title><?=$website['title'];?></title> </head> <body> <? if($online) : ?> <!--Your regular page here.--> <? else : ?> <!--Website offline display message.--> <? endif; ?> </body> </html>
Personally I don't mind using shorthand PHP tags when it's inside a HTML document. I don't ever declare a PHP document with them though. Also, it doesn't comply with PEAR coding standards (at least I don't think so).
-
What kind of field is it? Is it an INT or a VARCHAR.
If it's an integer, then you shouldn't use quotes. It makes it a string. And vice versa.
Also you don't need the parentheses wrapped around the comparison.
[SOLVED] two button form
in PHP Coding Help
Posted
You first need to get the POST data from the form.
my_form.php